The "GitHub Llama CPP" project focuses on leveraging the Llama language model implementations in C++, providing a framework for developers to effectively integrate and utilize these models in their applications.
Here's a simple code snippet illustrating how to use a Llama model in C++:
#include <llama.h>
int main() {
LlamaModel model("path/to/llama/model");
model.load();
std::string output = model.generate("Hello, Llama!");
std::cout << output << std::endl;
return 0;
}
Understanding LLaMA
What is LLaMA?
LLaMA (Large Language Model Meta AI) is a groundbreaking project designed to provide powerful and efficient language models for research and application in the field of natural language processing (NLP). Developed by Meta (formerly Facebook), LLaMA aims to enhance the understanding of language through advanced algorithms and large datasets. Its evolution reflects the rapid advancements in the AI ecosystem, making it a critical tool for developers and researchers alike.
LLaMA features a variety of model sizes tailored to different performance needs. This flexibility allows users to find the right balance between computational efficiency and output quality, making it suitable for diverse applications ranging from chatbots to complex data analysis.
Why Use LLaMA in C++ Applications?
Integrating LLaMA into C++ applications opens up numerous possibilities, particularly due to the performance advantages of C++. The language is known for its speed and efficiency, which can result in significantly faster model training and inference times compared to interpreted languages.
This can be particularly useful in scenarios requiring real-time processing, such as:
- Chatbots that need to generate quick responses.
- Data analytics tools that perform sentiment analysis on large datasets.
- Integration into gaming applications for dynamic character interactions.
Incorporating LLaMA with C++ can make your applications not only faster but also more responsive and capable of handling larger demands.
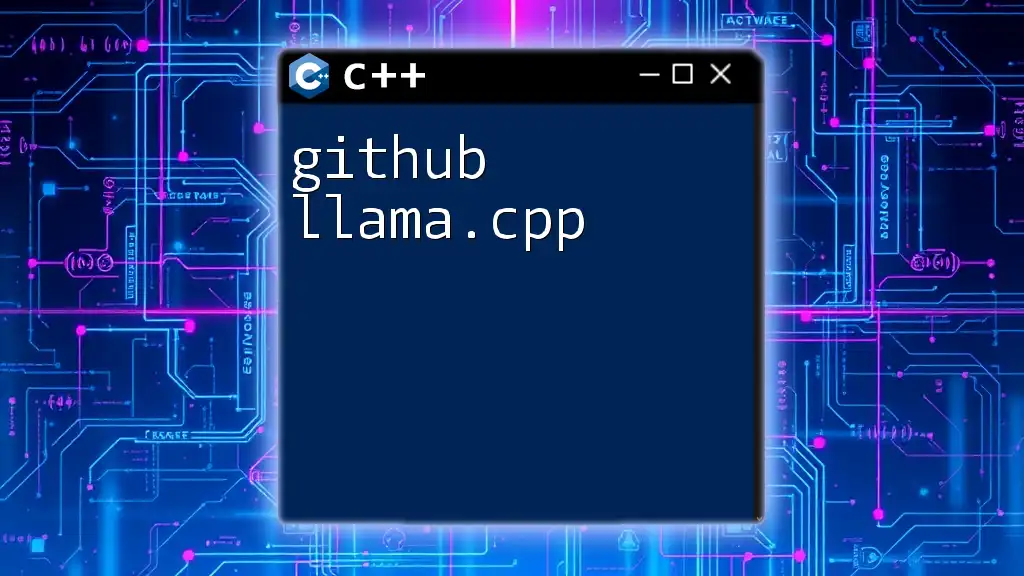
Getting Started with GitHub LLaMA CPP
Setting Up Your Environment
To get started with LLaMA in a C++ environment, you need to ensure you have the right tools. Here’s a checklist of required installations:
- C++ Compiler: A modern compiler like GCC or Clang enables you to compile your C++ code.
- Git: Essential for cloning the LLaMA repository from GitHub.
- CMake: This builds system is critical for managing the build process of the LLaMA project.
Cloning the Repository
To use the LLaMA codebase, the first step is to clone the repository from GitHub. You can do this easily by executing the following command in your terminal:
git clone https://github.com/facebookresearch/llama.git
This command will create a local copy of the LLaMA repository, giving you access to all the models, scripts, and resources provided by the developers.
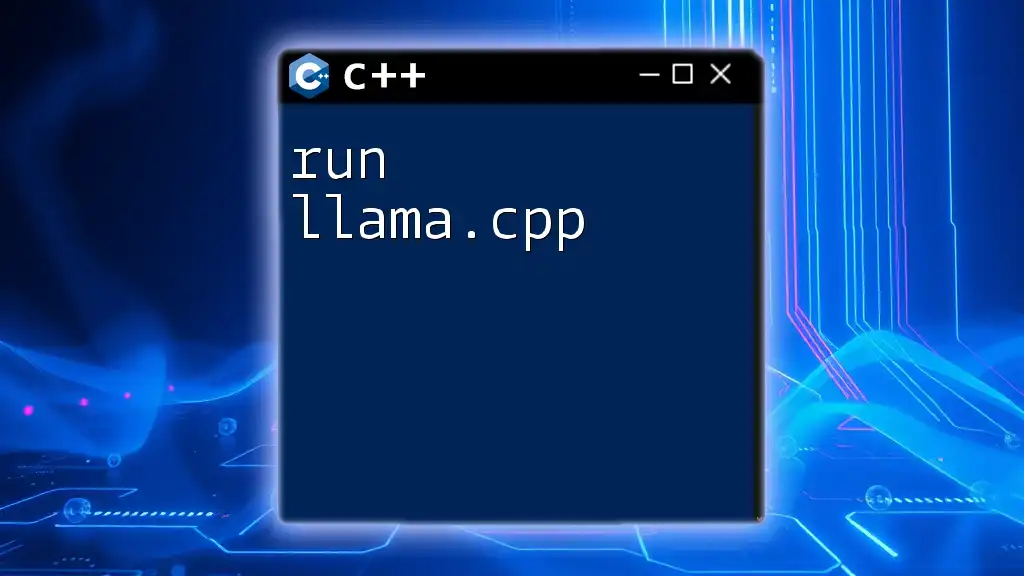
Understanding the Project Structure
Overview of the Repository
Once you've cloned the repository, it’s essential to understand its structure. The typical layout includes directories for models, data loaders, training scripts, and documentation to guide your development process. Assessing this structure allows you to navigate the project efficiently.
Important Components
The most noteworthy components within the repository include:
- Model Architectures: These define the configuration and workings of the various LLaMA models.
- Data Loaders: Scripts within this directory handle the preprocessing of datasets, which is crucial for training and evaluation.
- Training Scripts: These are used to train LLaMA models on your specific datasets.
For example, to initialize a model in C++, you may use:
LLM model = LLM("model-path");
This line of code demonstrates the simplicity with which you can create a model instance once the repository is compiled and integrated into your C++ application.
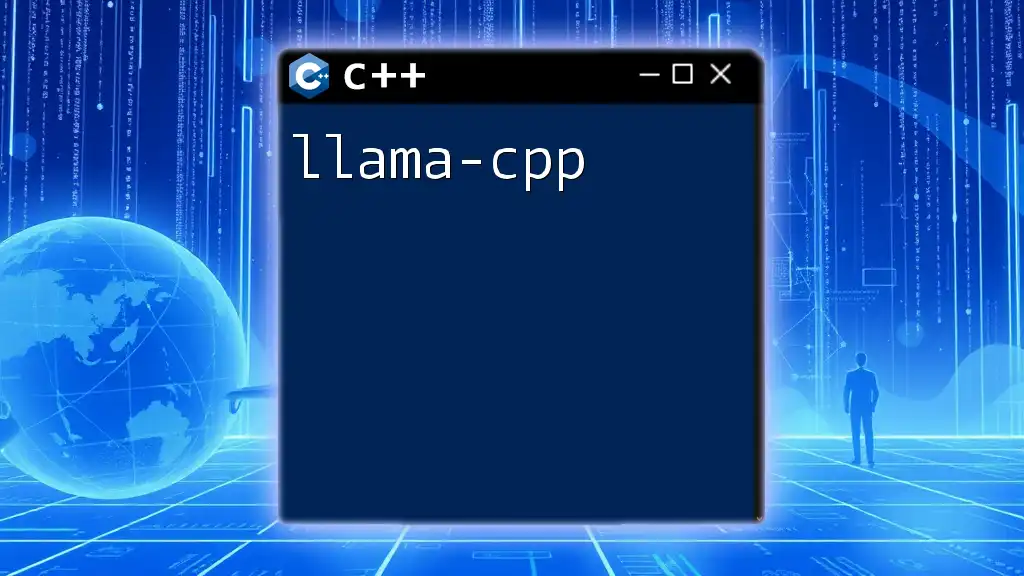
Using C++ Commands with LLaMA
Basic CPP Commands
C++ offers a variety of commands that can significantly enhance your interaction with LLaMA. Basic input/output operations and data management commands allow you to efficiently handle data while utilizing LLaMA's capabilities.
For instance, if you need to read a text file containing input data for the model, you could do:
#include <fstream>
#include <string>
std::ifstream inputFile("input.txt");
std::string inputText;
if (inputFile) {
std::getline(inputFile, inputText);
}
In this snippet, we read from a file and store it in a string for processing.
Advanced CPP Commands
As your application grows in complexity, leveraging advanced C++ commands becomes necessary. Techniques like multi-threading can significantly accelerate data processing or model inference.
Here's an example of employing multi-threading:
#pragma omp parallel for
for (int i = 0; i < num_tasks; i++) {
// Perform task
}
Using OpenMP, you can distribute tasks to multiple threads, resulting in faster execution times especially useful for large-scale tasks or batch processes.
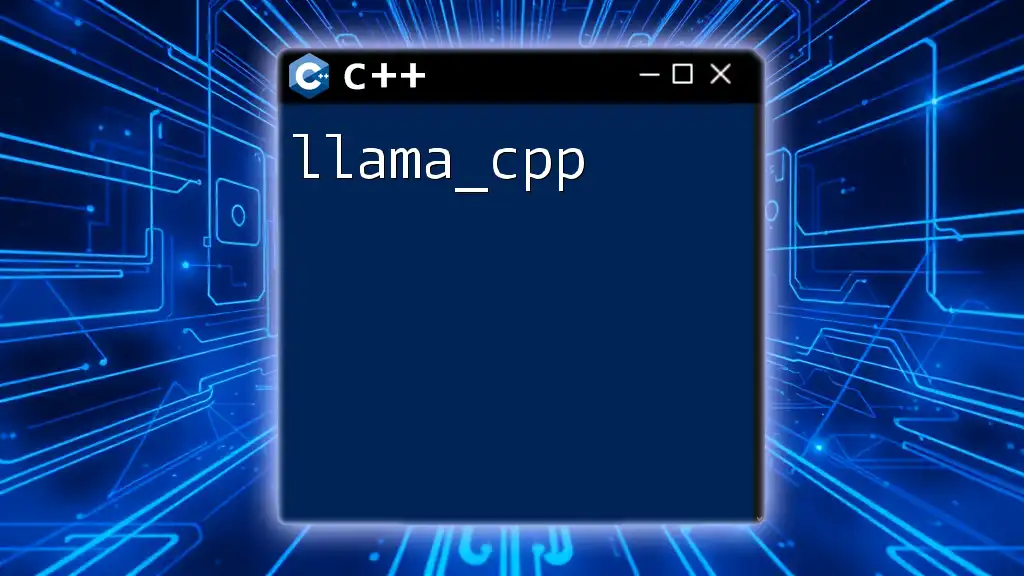
Building and Compiling the LLaMA Project
Preparing the Build System
CMake is instrumental for structuring the build process. Creating a `CMakeLists.txt` file specifically for your project will help streamline the compilation. It should define the required libraries, include directories, and build configurations.
Compiling the Code
After setting up your environment and preparing your CMake files, compiling the LLaMA project is straightforward. You can execute the following commands:
mkdir build
cd build
cmake ..
make
The `make` command will compile the code and produce the necessary binaries. During this process, you may encounter common compilation errors. Familiarize yourself with these issues and their potential solutions to enhance your debugging skills.
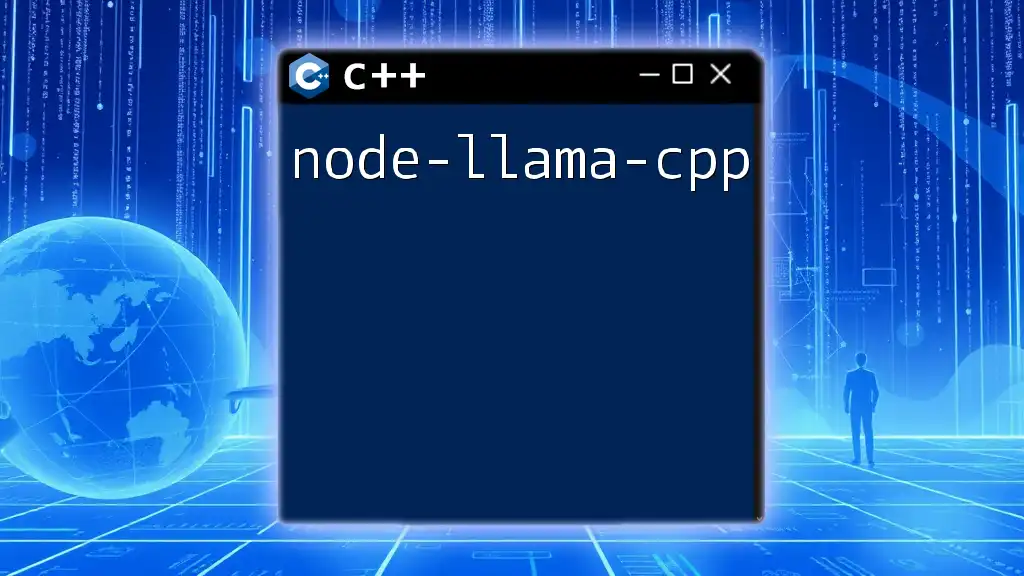
Integrating LLaMA in Your Application
Creating a C++ Application with LLaMA
Integrating LLaMA into an existing C++ application involves a few key steps. After building the project successfully, you can include LLaMA headers in your application code to enable its functionalities.
For example, initializing a model can be as simple as:
#include "llama.h"
int main() {
LLM model("model-path");
model.runInference("input-text");
return 0;
}
This straightforward implementation allows you to quickly generate outputs based on the inputs provided to the LLaMA model.
Handling Input and Output
Preparing input data for LLaMA models is crucial. Text needs to be tokenized and often transformed into a suitable format before being fed into the model. Conversely, after obtaining the output from the model, it requires deserialization and interpretation to extract useful information.
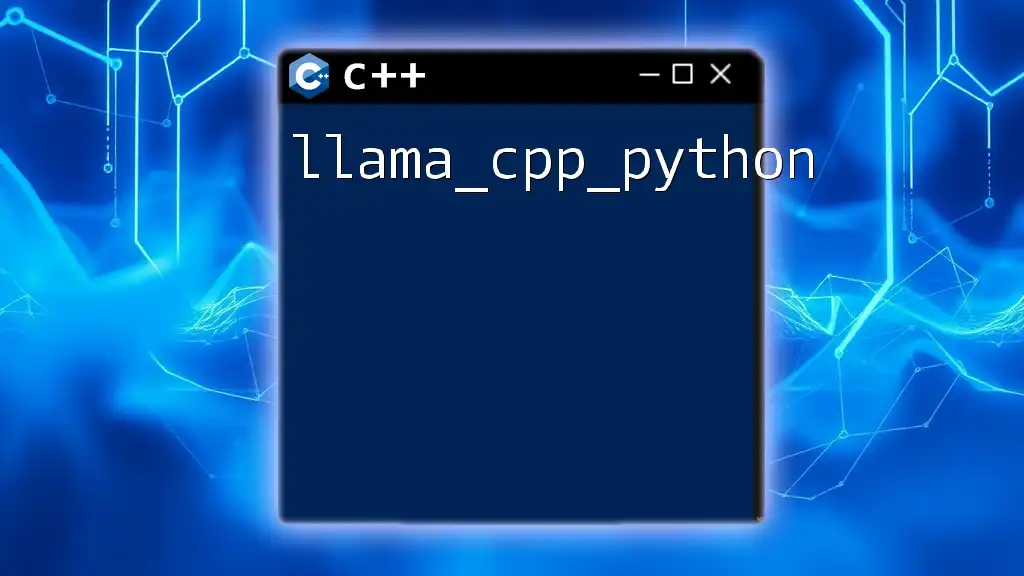
Best Practices for Using GitHub LLaMA with C++
Optimization Tips
Performance optimization in C++ is essential, especially when applying machine learning models like LLaMA. Here are some techniques to consider:
- Use smart pointers to manage memory efficiently.
- Implement inline functions where beneficial to reduce function call overhead.
- Employ appropriate data structures that align with your algorithm requirements to ensure swift access and manipulation.
Testing and Validation
Testing your application is crucial for maintaining code quality. Implement unit tests to validate the functionality of various components within your application. Use frameworks like Google Test for conducting thorough and systematic testing of your models and their integrations.
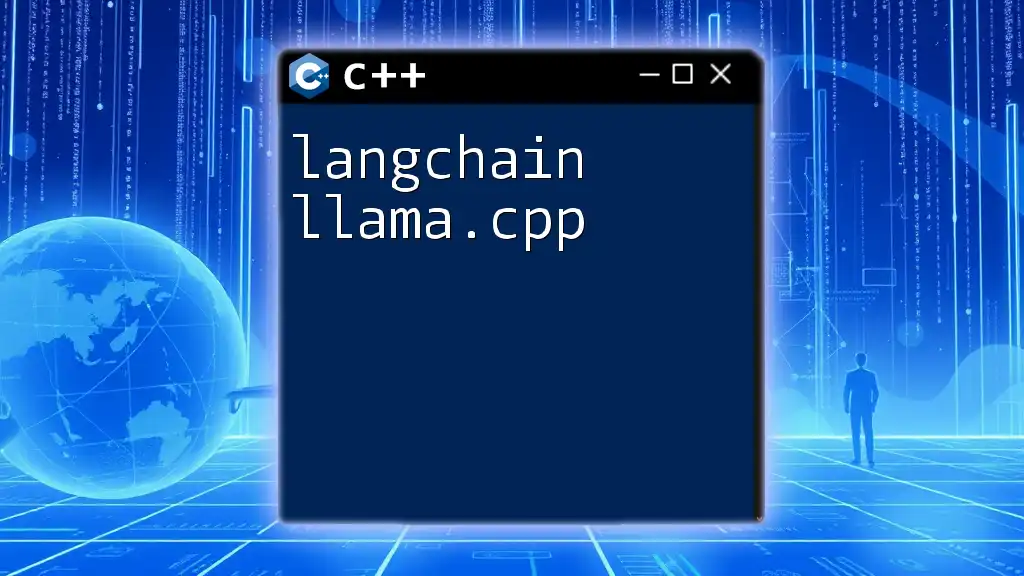
Community and Contributions
Engaging with the LLaMA Community
Becoming part of the LLaMA community can greatly enhance your understanding and experience. The community often shares valuable resources, coding tips, and troubleshooting advice. Engaging on platforms like GitHub issues or relevant forums can also lead to collaboration opportunities.
Future of LLaMA and C++ Integration
The intersection of machine learning and C++ is a rapidly evolving frontier. Keeping an eye on emerging trends, such as developments in hardware acceleration and new algorithms, can help you stay at the forefront of innovation. Continued enhancements to the LLaMA project will likely expand its capabilities and applications.
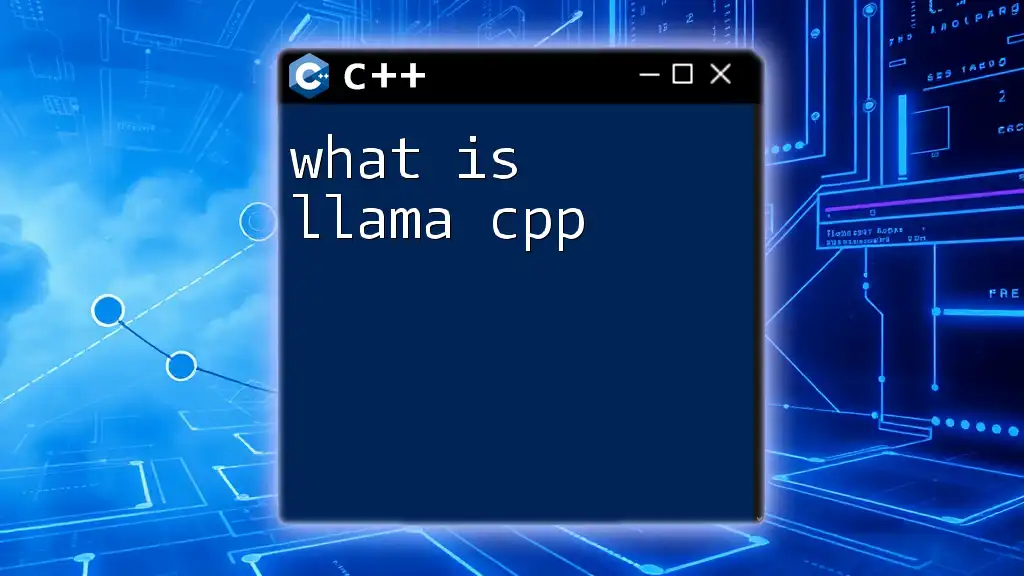
Conclusion
In this guide, we explored the fundamentals of integrating GitHub LLaMA CPP into C++ applications. By understanding its architecture, optimizing performance, and leveraging community resources, developers can create efficient and powerful programs. Dive into the world of LLaMA with C++—the possibilities are endless!