A `stringstream` in C++ is a convenient way to perform input and output operations on strings, allowing you to manipulate string data as if it were a stream.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::stringstream ss;
ss << "Hello, " << "World!";
std::string str = ss.str();
std::cout << str; // Outputs: Hello, World!
return 0;
}
What is `stringstream`?
`stringstream` is a part of the C++ Standard Library found in the `<sstream>` header, serving as a core component for stream-based input and output operations. Streams can be thought of as abstractions that facilitate reading and writing in a flow-like manner, allowing for flexible interaction with strings.
Key Distinctions: It's important to understand that `stringstream` is a versatile tool, encompassing both `istringstream`, which is specifically designed for reading from strings, and `ostringstream`, which is focused on writing to strings. Each serves a unique purpose depending on your needs:
- `istringstream`: Primarily used for reading formatted data from strings.
- `ostringstream`: Designed for writing formatted data to strings.
- `stringstream`: Combines both functionalities, allowing for mixed operations.
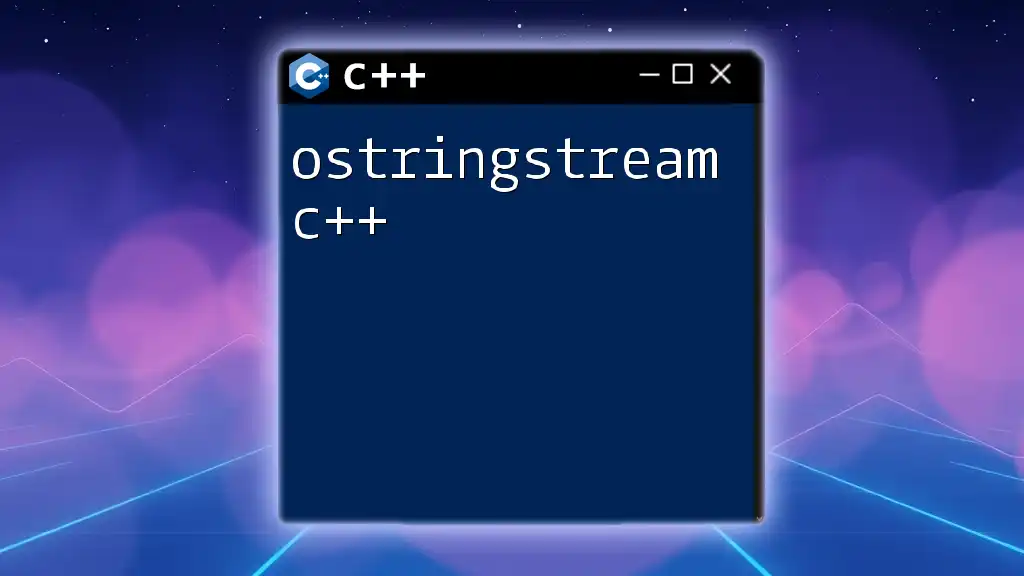
Setting Up `stringstream`
To utilize `stringstream` in your program, the first step is including the necessary header:
#include <sstream>
Once the header is included, you can declare a `stringstream` as follows:
std::stringstream ss;
This line creates an instance of `stringstream` ready for operations.
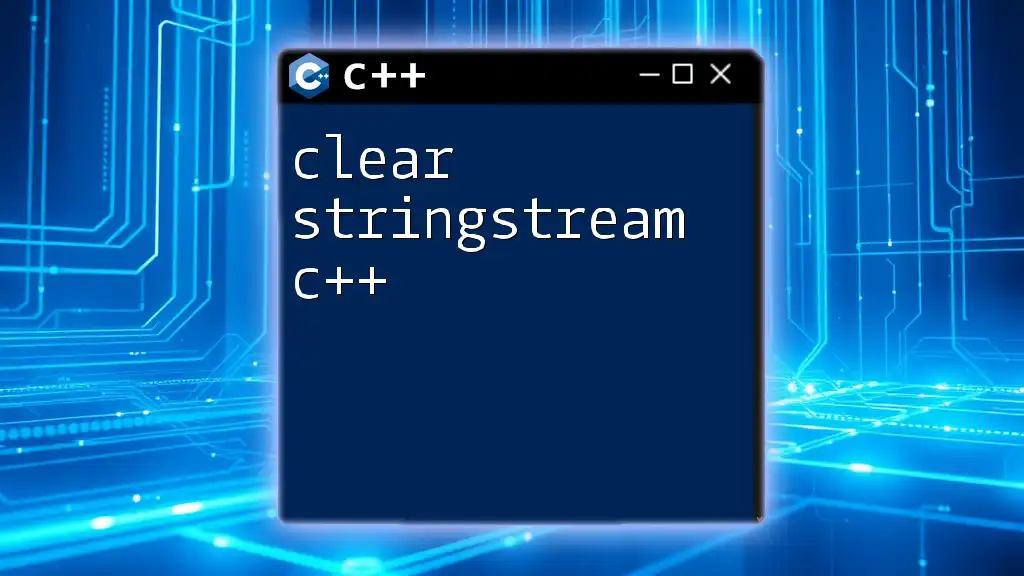
Common Use Cases
Converting Data Types
One of the most powerful features of `stringstream` is its ability to facilitate conversion between strings and various numeric types. Whether you need to extract a number from a string or convert a number to a string format, this utility excels.
For instance, here’s how to convert a string to an integer:
std::string numStr = "123";
int num;
std::stringstream(numStr) >> num; // Conversion
This code snippet will successfully parse the integer from `numStr`, storing the value in `num`.
Conversely, you can convert an integer to a string using:
int num = 123;
std::stringstream ss;
ss << num;
std::string numStr = ss.str(); // Conversion
Here, `ss.str()` retrieves the string representation of `num` from the `stringstream`.
String Manipulation
`stringstream` also shines in string building and manipulation. When you need to concatenate various data types into a single string, `stringstream` provides an elegant solution.
Consider the following example where we combine strings and numbers:
std::stringstream ss;
ss << "Hello, " << "World! " << 2023;
std::string result = ss.str(); // "Hello, World! 2023"
This example effectively constructs a string that incorporates both text and numerical data.
Parsing Input
Another significant application of `stringstream` is its capability to parse input strings, especially when dealing with delimited data. For instance, if you have a string containing comma-separated values, you can process it as shown below:
std::string data = "one, two, three";
std::stringstream ss(data);
std::string item;
while (std::getline(ss, item, ',')) {
// Process item...
}
This loop extracts individual items from `data`, allowing for customized processing of each separated value.
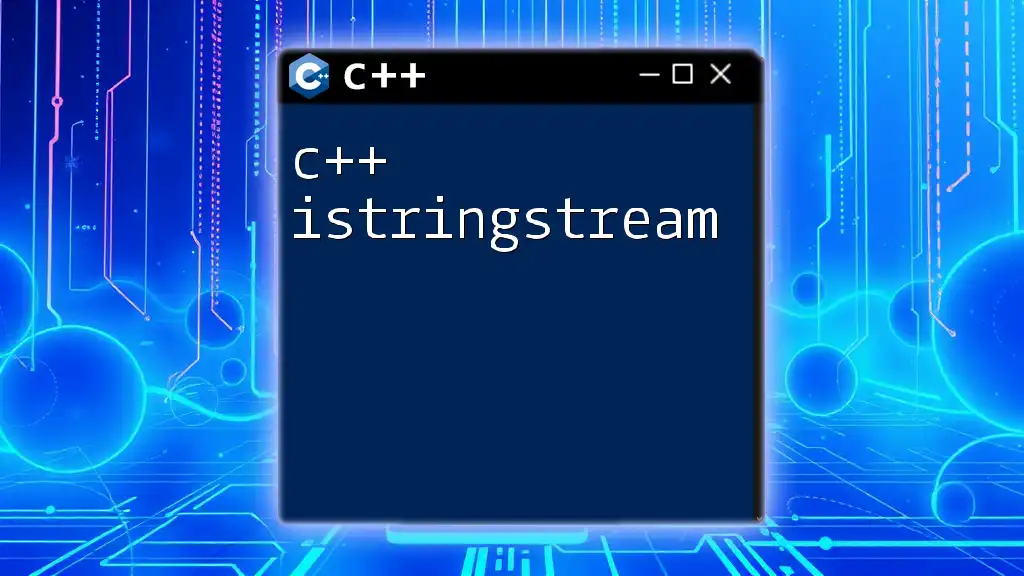
Performance Considerations
When it comes to performance, choosing between `stringstream` and traditional string manipulation methods can make a difference. Stream-based operations may offer more efficient handling of complex string manipulations compared to manual concatenations with `+`.
However, there are cases where `stringstream` might introduce overhead. Understanding your specific use case, especially regarding memory management, is crucial for optimizing performance. If you're performing frequent conversions or concatenations, benchmark each approach to determine which provides the best efficiency for your situation.
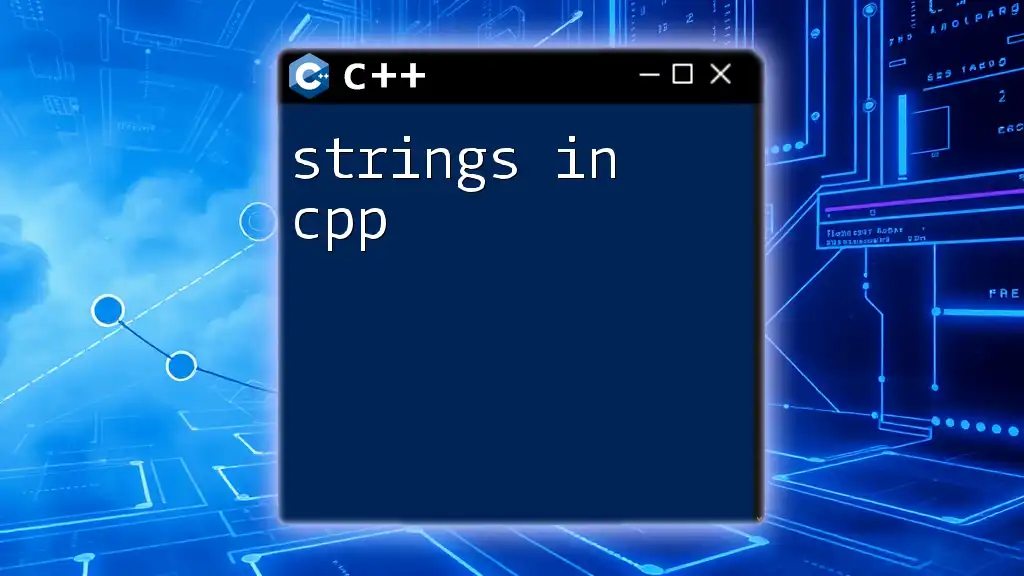
Practical Applications
Logging Information
In production applications, logging is essential for monitoring behaviors and handling errors. Leveraging `stringstream` for formatted logs allows you to construct detailed messages dynamically:
std::stringstream logStream;
logStream << "Error: " << errorCode << " at " << timestamp;
std::string logMessage = logStream.str();
This approach ensures that your log messages can flexibly incorporate varying runtime data in a readable format.
Formatting Output
When you need to present numerical data in a specific format, `stringstream` comes to the rescue once again. For example, if you want to control the decimal precision for floating-point numbers, you can do it like this:
#include <iomanip> // for std::fixed and std::setprecision
std::stringstream ss;
ss << std::fixed << std::setprecision(2) << 123.45678;
std::string formattedOutput = ss.str(); // "123.46"
Using manipulators like `std::fixed` and `std::setprecision`, you can format your output precisely to meet your requirements.
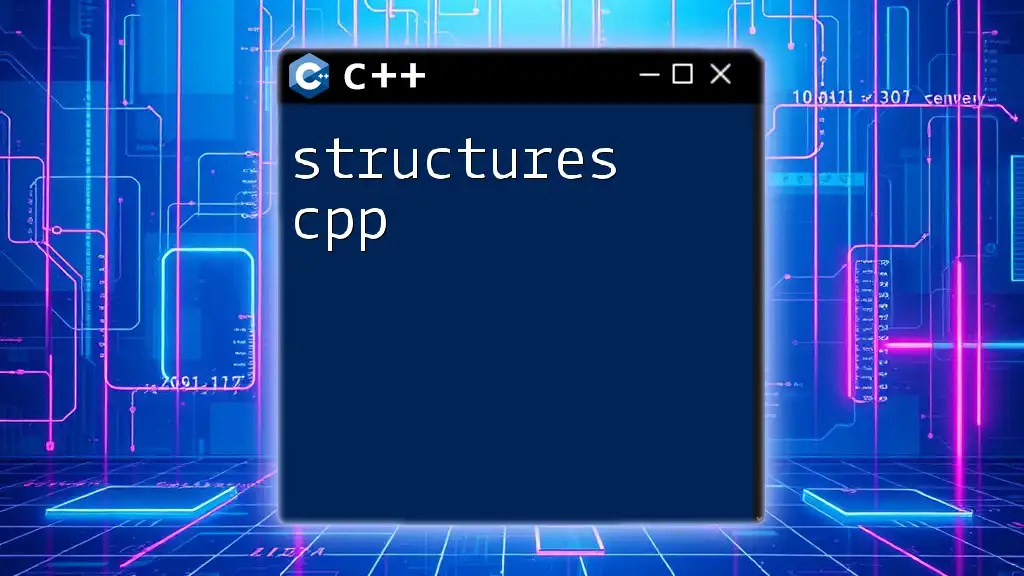
Error Handling
When working with `stringstream`, it's crucial to implement appropriate error handling, especially during data conversions. If the input string is invalid (e.g., contains non-numeric characters), the conversion may fail. Here's how to account for that:
std::string numStr = "abc"; // Invalid input
int num;
std::stringstream ss(numStr);
if (!(ss >> num)) {
std::cerr << "Conversion failed!" << std::endl;
}
This code checks whether the conversion succeeded and outputs an error message if it did not, providing a safer and more reliable programming environment.
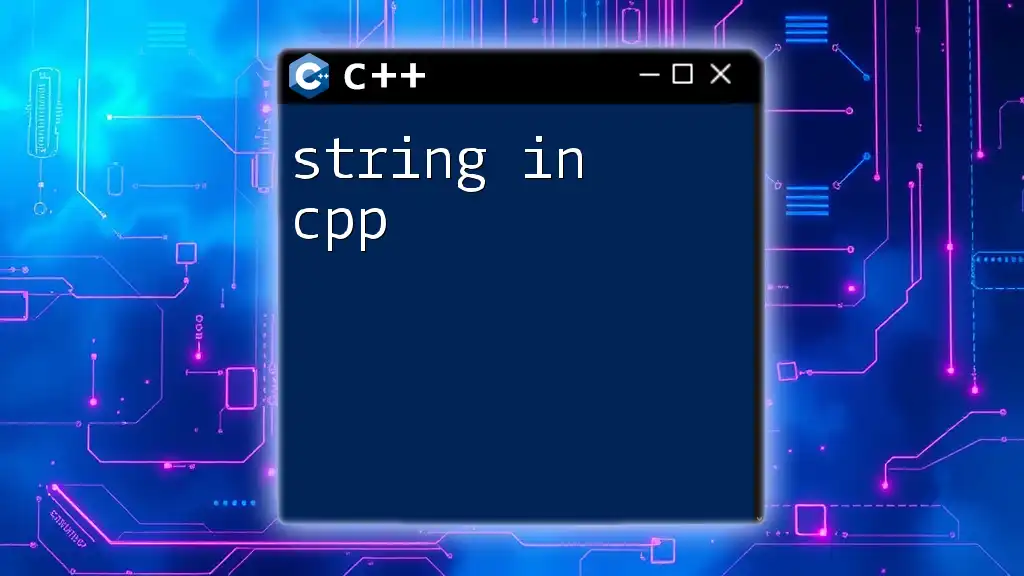
Best Practices
To maximize your effectiveness with `stringstream`, adhere to these best practices:
- Be Mindful of Memory Usage: Avoid excessive allocations and manage your streams appropriately.
- Limit Complex Logic in Streams: Keep operations straightforward to improve code readability.
- Use Clear Variable Names: Naming consistency helps maintain comprehension, especially when dealing with multiple streams.
By following these guidelines, you'll reduce common pitfalls and enhance the maintainability of your code involving `stringstream`.
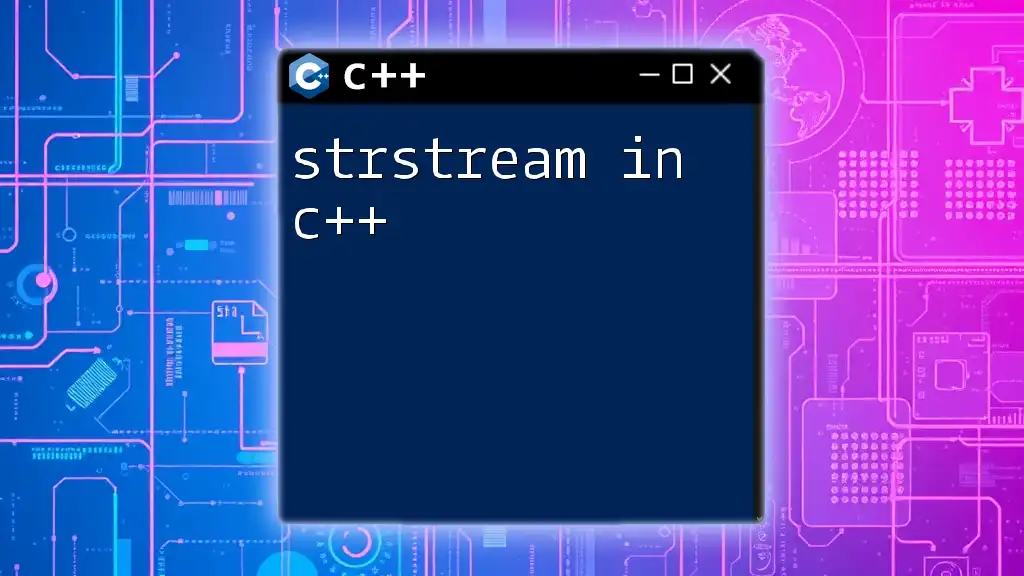
Conclusion
`stringstream cpp` is a powerful tool for converting types, manipulating strings, and parsing input with remarkable flexibility. Mastering its functionalities not only streamlines data handling but also enhances the overall quality of your C++ programming projects. Experiment with the examples provided to see firsthand how `sstream` can elevate your coding practices.
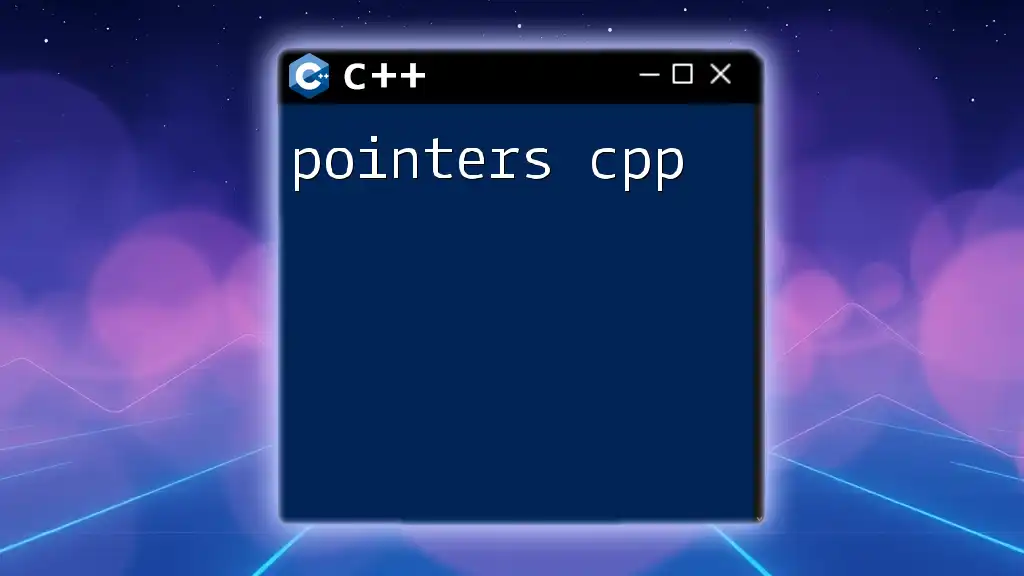
Additional Resources
For further learning, you can reference the official C++ documentation and explore recommended books and tutorials that delve into more advanced topics in C++ programming.
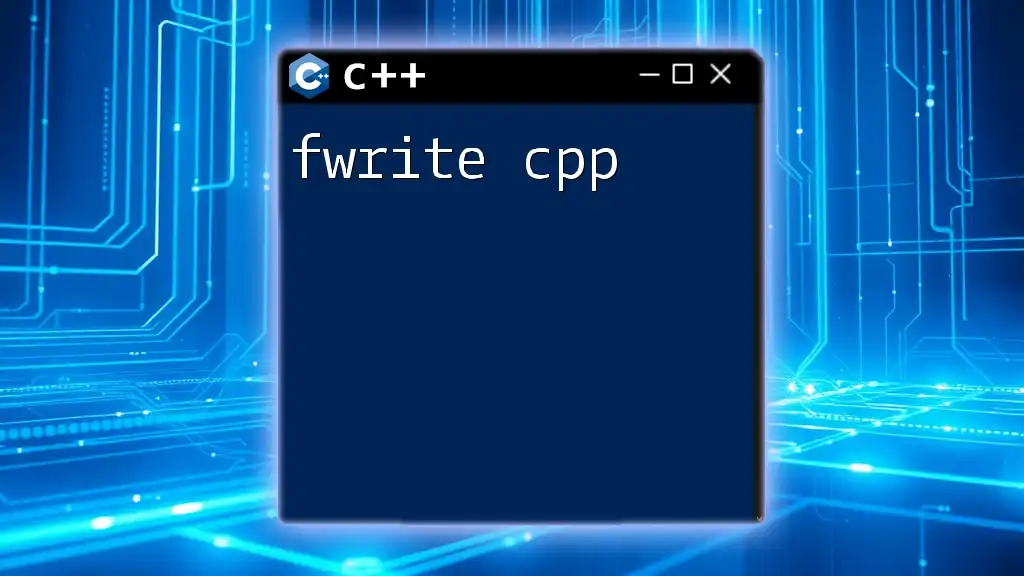
Call to Action
What has your experience with `stringstream` been like? Feel free to share your insights or challenges in the comments. Be sure to subscribe for more concise C++ tutorials and tips!