In C++, classes are user-defined data types that encapsulate data and functions, enabling the creation of objects; the following example demonstrates a simple class definition and object instantiation:
#include <iostream>
class Dog {
public:
void bark() { std::cout << "Woof!" << std::endl; }
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Understanding Classes in C++
What is a Class in C++?
A class in C++ is a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. In essence, a class defines the properties (attributes) and behaviors (functions) that the objects will have.
Key Characteristics of a Class
- Attributes: These are the data members that define the state of the class.
- Methods: These are member functions that describe the behaviors or actions the class can perform.
Syntax of Class Declaration
The basic structure for declaring a class consists of the `class` keyword followed by the class name and a block that encloses the members.
class ClassName {
// Data members
// Member functions
};

Creating a C++ Class
How to Create a Class in C++
Creating a class in C++ involves defining its name, attributes, and behaviors. Let’s look at a simple example to illustrate this process.
Example of Class Creation
Suppose we want to create a class called `Car`.
class Car {
private:
string color;
string model;
public:
void setDetails(string c, string m) {
color = c;
model = m;
}
void showDetails() {
cout << "Color: " << color << ", Model: " << model << endl;
}
};
In this example, we have a `Car` class with two private attributes, `color` and `model`. We also have two public member functions: `setDetails` for setting the values of the attributes and `showDetails` for displaying them.
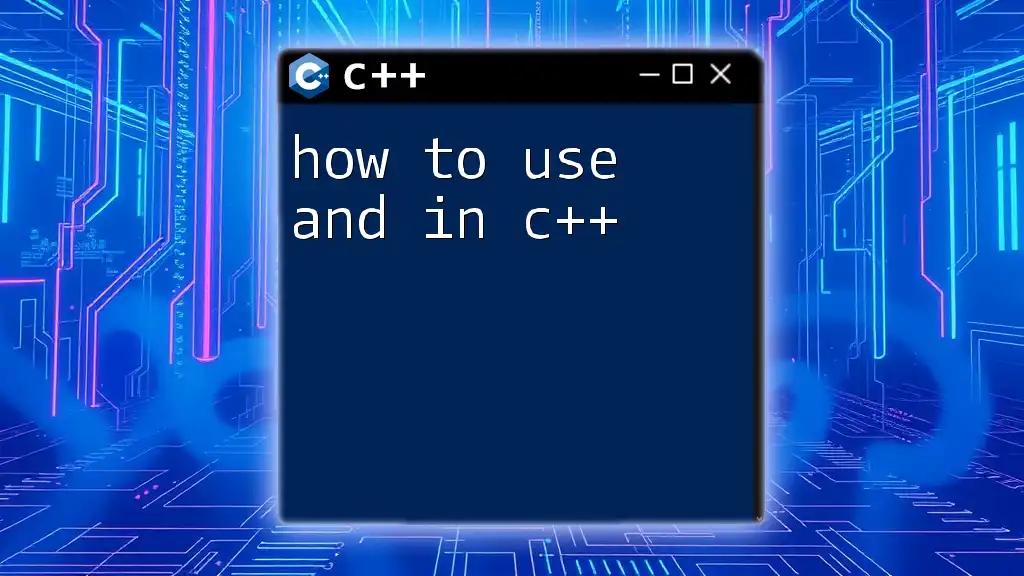
Making Classes in C++
Creating Objects from Classes
Once a class has been defined, creating an object from that class is straightforward. This is done using the class name followed by the object name.
Car myCar; // Creating an object
myCar.setDetails("Red", "Toyota");
myCar.showDetails();
In this example, we create an object `myCar` of type `Car` and use the member functions to set and display the details.

Class Members
Access Specifiers in C++ Classes
C++ provides three access specifiers to define the accessibility of class members: `private`, `public`, and `protected`.
- Private Members: These members are accessible only within the class itself. They are crucial for encapsulation as they restrict access to sensitive data.
- Public Members: These can be accessed from outside the class and provide a means to interact with object data.
- Protected Members: These are similar to private members but can also be accessed by derived classes.
Example of Member Access
Here is how access control works in a class:
class Sample {
private:
int secret; // Private member
public:
void setSecret(int s) {
secret = s; // Can access private member
}
int getSecret() {
return secret; // Can access private member
}
};
// Example usage
Sample obj;
obj.setSecret(42);
cout << obj.getSecret(); // Accessing private member via public method

Constructors and Destructors
What are Constructors and Destructors?
Constructors are special member functions that initialize objects when they are created. Destructors, on the other hand, are responsible for cleaning up resources before an object is destroyed.
Creating Constructors in C++
There are generally two types of constructors: default and parameterized.
class Book {
public:
Book() { /* Default constructor */ }
Book(string title) { /* Parameterized constructor */ }
};
In this example, the class `Book` has both a default constructor and a parameterized constructor that takes a title as an argument.
Destructors in C++
Just as constructors can perform setup, destructors can handle cleanup.
class Example {
public:
~Example() {
// Destructor logic here
}
};
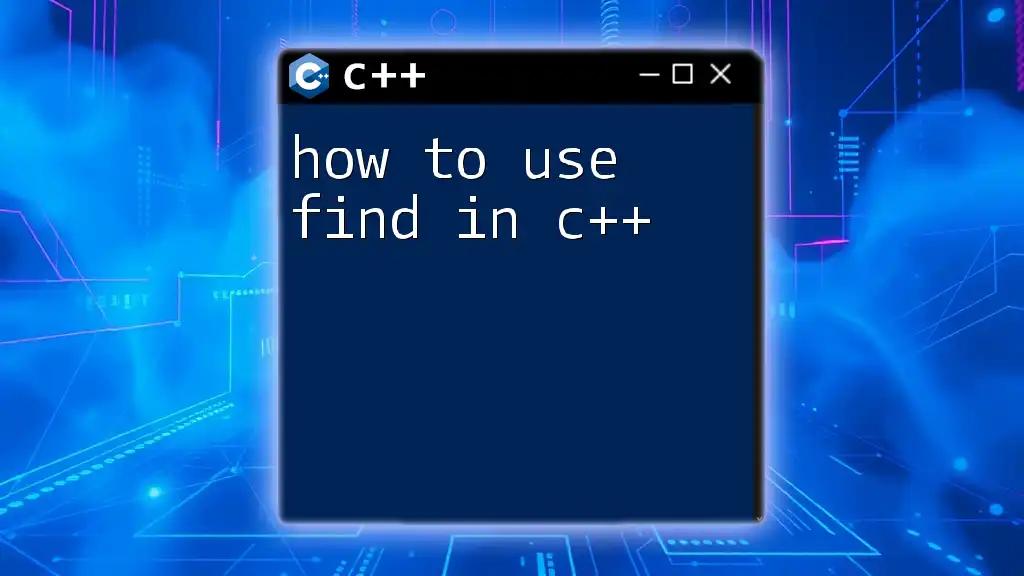
Features of Classes in C++
Inheritance
Inheritance allows a class (derived class) to inherit properties and methods from another class (base class). This promotes code reusability.
Polymorphism
Polymorphism lets classes define methods that can be overridden in derived classes. It enables a program to decide at runtime which method should be invoked.

Real-World Applications
How to Use C++ Classes in Real Projects
Classes are pivotal in organizing code efficiently. By encapsulating related data and functions, they make the code more modular and easier to maintain. For instance, in a banking application, you might have classes for `Account`, `Customer`, and `Transaction`, each with their attributes and methods.
Sample C++ Class Implementation
Here’s a practical example involving a simple calculator class.
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
};
// Example usage
Calculator calc;
cout << "Sum: " << calc.add(5, 3) << endl;
cout << "Difference: " << calc.subtract(5, 3) << endl;
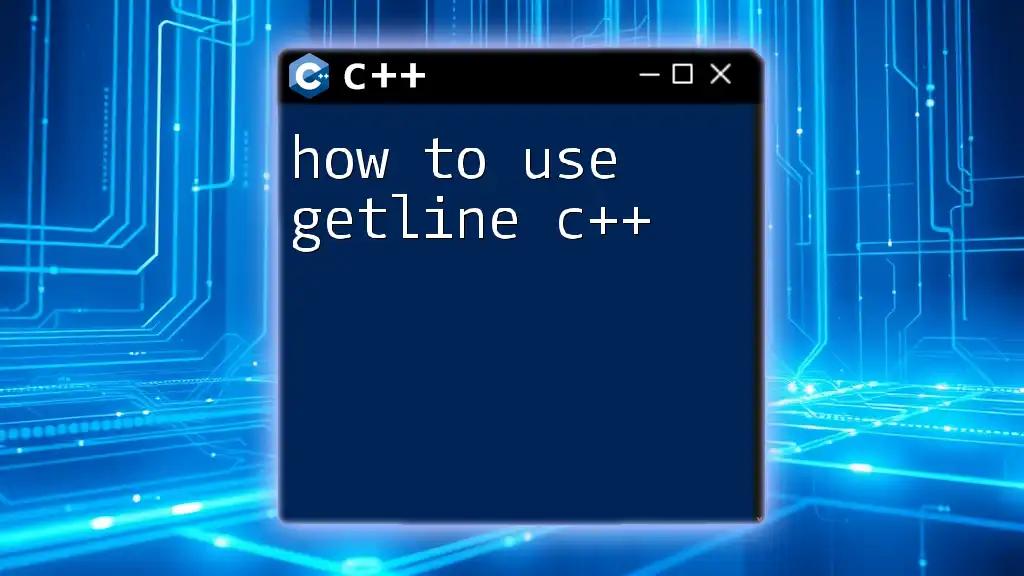
Debugging and Best Practices
Common Mistakes When Using Classes
- Forgetting to initialize members properly, leading to garbage values.
- Making too many members public, which compromises encapsulation.
- Overcomplicating the inheritance hierarchy.
Best Practices for Creating Classes in C++
- Use private members for sensitive data and expose them through public member functions.
- Keep your classes focused; one class should serve a single responsibility.
- Write clear and descriptive class and method names to improve code readability.
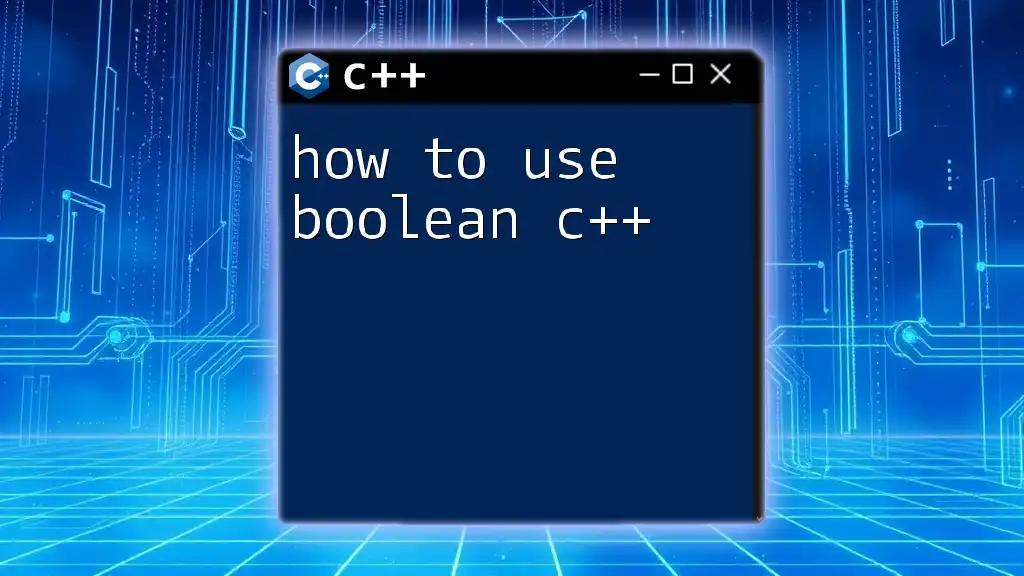
Conclusion
In summary, understanding how to use classes in C++ opens up tremendous opportunities to improve code structure and maintainability. By defining clear and efficient classes, you can enhance the functionality of your C++ programs. Embrace the principles of object-oriented programming, and you’ll find your coding experience both enjoyable and productive.

Additional Resources
For further learning, refer to reputable C++ documentation, tutorials, and books that offer in-depth insights into classes and OOP practices. Consider exploring advanced topics such as template classes, operator overloading, and the Standard Template Library (STL) to expand your C++ knowledge.