In C++, the modulo operator `%` is used to find the remainder of the division between two integers.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a % b;
std::cout << "The remainder of " << a << " divided by " << b << " is " << result << std::endl;
return 0;
}
Understanding Modulo in C++
What is the Modulo Operator?
The modulo operator, denoted by the percentage sign (`%`), is a mathematical operator that returns the remainder of a division operation. It is commonly used in programming to perform calculations that involve cyclical patterns, even or odd checks, and more.
How Does the Modulo Operator Work?
In C++, the modulo operation follows a straightforward syntax: `a % b`, where `a` is the dividend and `b` is the divisor. When you perform `a % b`, you essentially calculate how many times `b` fits into `a` and return what remains.
For example, if `a` is 10 and `b` is 3, the operation `10 % 3` yields a remainder of 1 (since 3 goes into 10 three times, which is 9, leaving a remainder of 1).
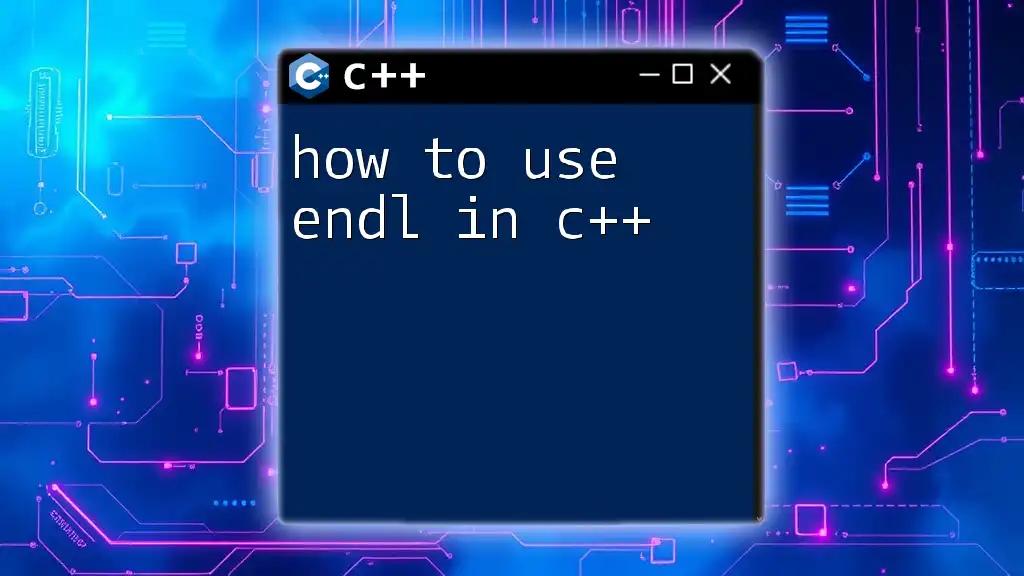
Using Mod in C++
Syntax of the Modulus Operator in C++
The basic syntax of the modulo operator is:
result = a % b;
Here, `result` will store the remainder after dividing `a` by `b`. It's essential to understand how both operands affect the outcome.
Examples of Using Modulus in C++
Example 1: Basic Modulo Calculation
Let’s start with a simple example to demonstrate the modulo operation:
#include <iostream>
using namespace std;
int main() {
int a = 10;
int b = 3;
cout << "10 % 3 = " << a % b << endl; // Output: 1
return 0;
}
In this example, the output will display 1, confirming our expectation as 10 divided by 3 leaves a remainder of 1.
Example 2: Even and Odd Number Check
A common application of the modulo operator is to check whether a number is even or odd. Here’s how it works:
#include <iostream>
using namespace std;
int main() {
int number = 6;
if (number % 2 == 0) {
cout << number << " is even." << endl;
} else {
cout << number << " is odd." << endl;
}
return 0;
}
In this case, since 6 is divisible by 2 without a remainder, the program outputs "6 is even." If you change `number` to an odd integer, like 7, it would output "7 is odd."
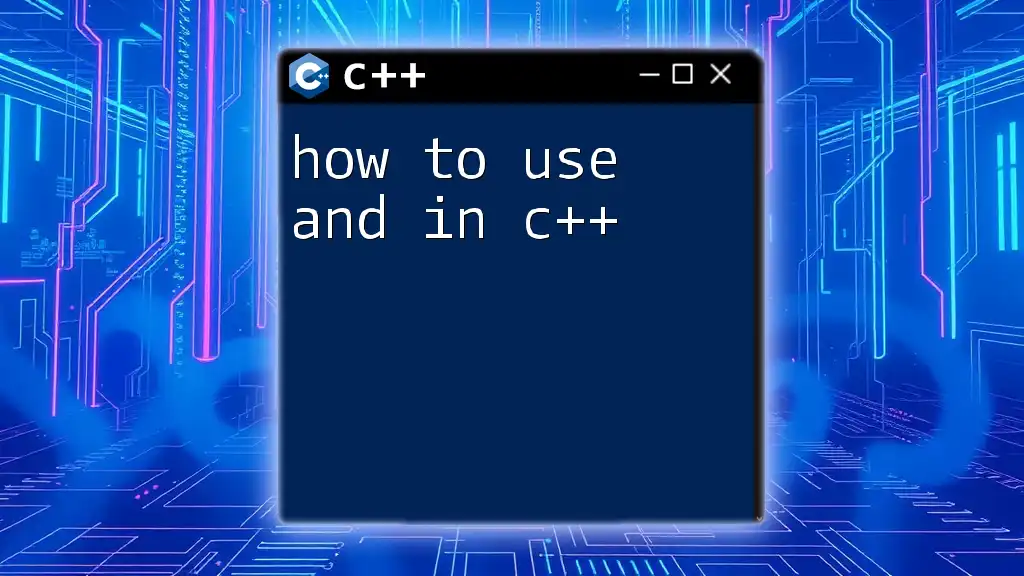
Common Use Cases of Modulus in C++
Calculating Remainders
The modulo operator has various practical applications beyond basic division. It can be particularly useful for calculating remainders in programming-related tasks, such as implementing algorithms that need to manage divisions and repeated patterns.
Working with Circular Structures
Another prominent use case for the modulo operator is in managing circular data structures, like arrays. The modulo operator allows you to wrap around indices and recycle them when they exceed defined boundaries.
Example: Circular Array Access
Here’s how you can utilize the modulo operator to access elements in a circular array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr)/sizeof(arr[0]);
int index = 7; // 7 % 5 = 2
cout << "Element at index " << index << " is " << arr[index % size] << endl; // Output: 3
return 0;
}
In this example, the array has five elements, and we attempt to access the index 7. Using `7 % 5` gives us 2, effectively retrieving the third element of the array—3.
Game Development Applications
In game development, the modulo operator is vital for maintaining score systems or managing game loops. It helps enforce constraints that keep values within certain limits, ensuring smoother and more predictable behavior during gameplay.
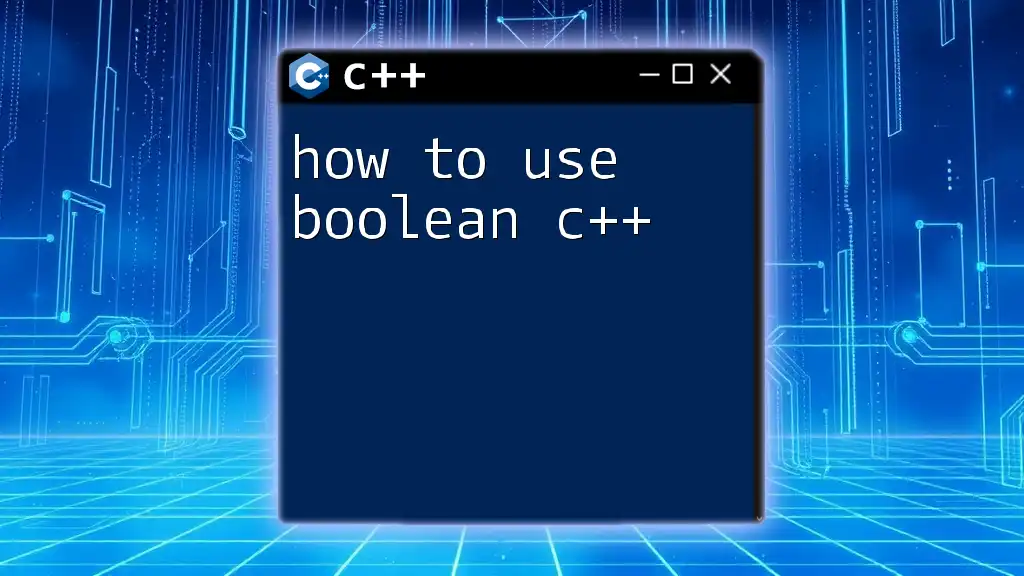
Advanced Concepts in Using Modulo
Negative Numbers and Modulo
One aspect of the modulo operator that programmers often find confusing is its behavior with negative numbers. In C++, the result of a modulo operation involving negative integers can differ from that in other programming languages.
Example: Negative Modulo Operation
#include <iostream>
using namespace std;
int main() {
int a = -10;
int b = 3;
cout << "-10 % 3 = " << a % b << endl; // Output: -1
return 0;
}
Here, `-10 % 3` results in -1. This behavior can be significant, especially when you expect a non-negative remainder. Understanding how C++ handles negative modulos can prevent unintended errors in your code.
Modulo with Floating Point Numbers
While C++ primarily deals with integers using the modulo operator, it is also possible to work with floating-point numbers. However, C++ does not directly support the modulo operator for floats. Instead, you can achieve similar results using functions like `fmod()` from the `<cmath>` library.
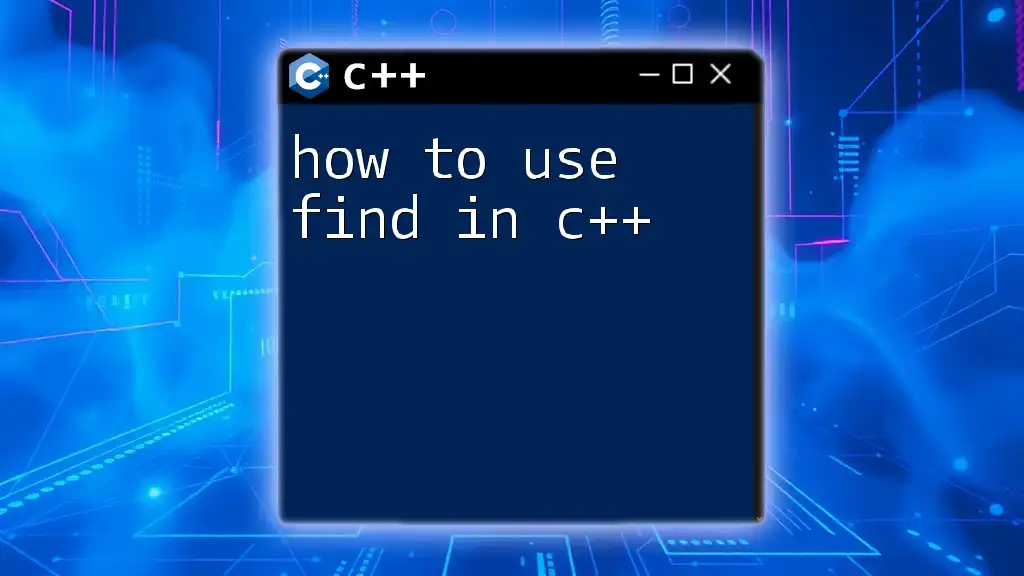
Best Practices When Using Modulo
Performance Considerations
While the modulo operator is simple, its use in large computations can lead to performance issues—especially within loops. Being conscientious about how often and where you use it can optimize your program’s efficiency.
Common Pitfalls
When utilizing the modulo operator, keep an eye out for common pitfalls such as:
- Misunderstanding its behavior with negative numbers.
- Forgetting to check for divisibility by zero.
- Not taking care of unintended infinite loops in your code that involve modulo.
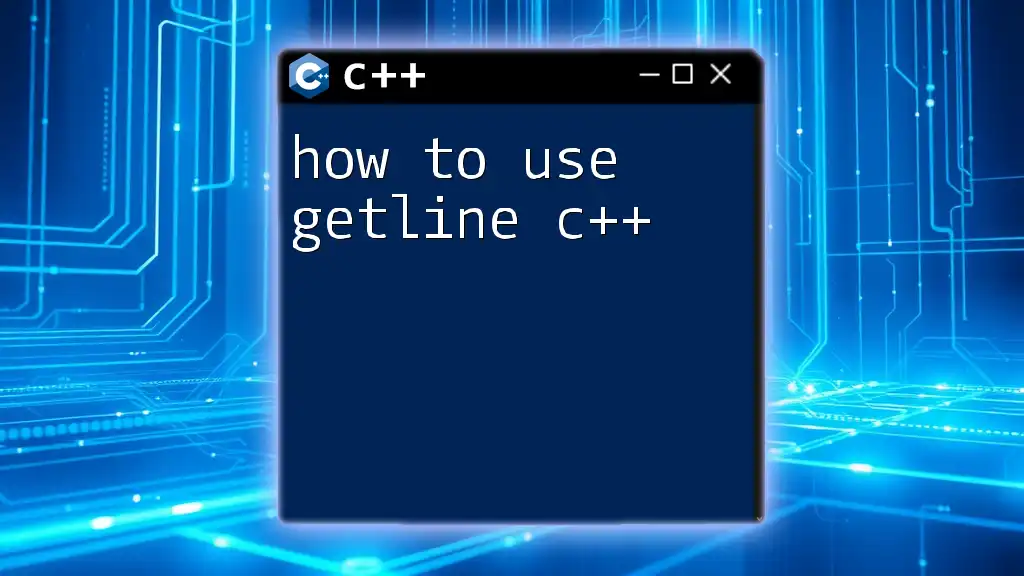
Conclusion
The modulo operator is a powerful tool in C++. It provides functionality for managing cycles, calculating remainders, and enforcing constraints on values. Mastering how to use modulo in C++ is essential for efficient programming and solving various coding challenges. By practicing the concepts discussed here, you'll harness this skill in meaningful and practical ways.
Further Reading and Resources
For those eager to enhance their understanding of C++ and the modulo operator, numerous online resources and guides dive deeper into advanced usage and nuanced behavior. Happy coding!