The modulus function in C++ is used to find the remainder of a division operation between two integers, and can be implemented using the `%` operator. Here's how you can use it in code:
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 3;
cout << "The remainder of " << a << " divided by " << b << " is " << a % b << endl; // Output: 1
return 0;
}
What is the Modulus Operator?
The modulus operator, represented by the symbol %, is a fundamental arithmetic operator in C++. It calculates the remainder when one integer is divided by another. Understanding the modulus operator is crucial for a variety of programming tasks, particularly in algorithm design and mathematical computations.
Basic Syntax
The basic syntax of the modulus operator in C++ is straightforward:
result = a % b;
In this expression, `a` is the dividend, `b` is the divisor, and `result` will hold the remainder of the division of `a` by `b`. For instance, if you have `a = 10` and `b = 3`, then:
int result = 10 % 3; // result will be 1
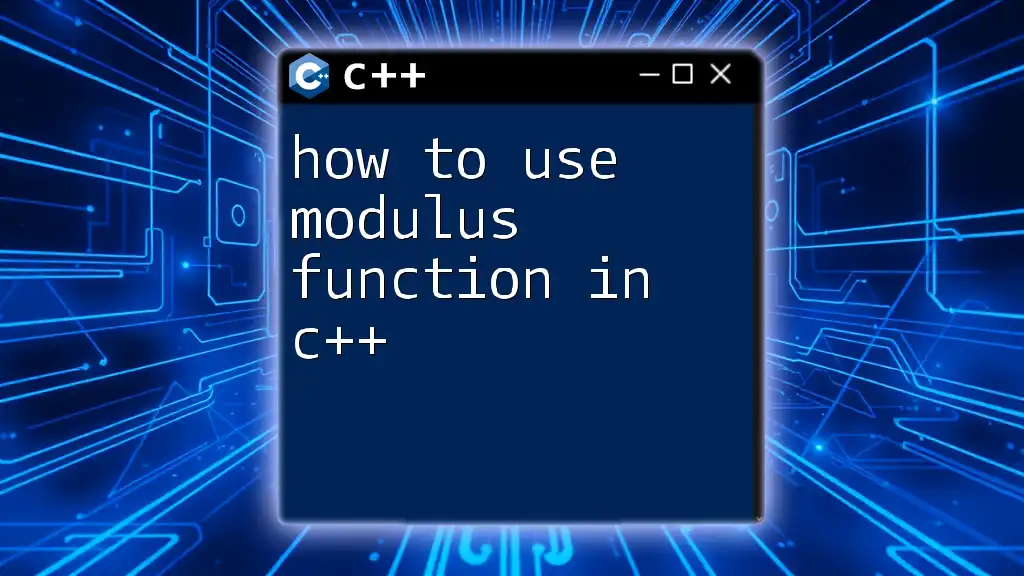
Practical Uses of the Modulus Operator
Checking Even or Odd Numbers
One of the most common uses of the modulus operator is to check whether a number is even or odd. Numbers can be classified based on their divisibility by 2: if a number divided by 2 has a remainder of 0, it is even. Otherwise, it is odd.
Here’s how you can implement this:
int number = 5;
if (number % 2 == 0) {
// Even number
std::cout << number << " is even." << std::endl;
} else {
// Odd number
std::cout << number << " is odd." << std::endl;
}
Divisibility Testing
You can also use the modulus operator to test whether one number is divisible by another. This is particularly useful in mathematical algorithms.
For example, if you want to check if `a` is divisible by `b`, you can do the following:
int a = 10, b = 5;
if (a % b == 0) {
// a is divisible by b
std::cout << a << " is divisible by " << b << std::endl;
}
In this case, since 10 divided by 5 leaves no remainder, the output will confirm that `a` is divisible by `b`.
Circular Array Indexing
The modulus operator is incredibly useful for implementing circular array indexing. This technique allows you to wrap around an array when accessing elements beyond its length.
Consider the following code:
int arr[] = {10, 20, 30, 40};
int size = sizeof(arr) / sizeof(arr[0]);
int index = 5;
int circularIndex = index % size; // Results in 1
std::cout << "Element at circular index " << circularIndex << " is: " << arr[circularIndex] << std::endl;
In this example, `index` is greater than the size of the array. By applying the modulus operator, we get a valid index that wraps around, allowing us to access elements seamlessly.
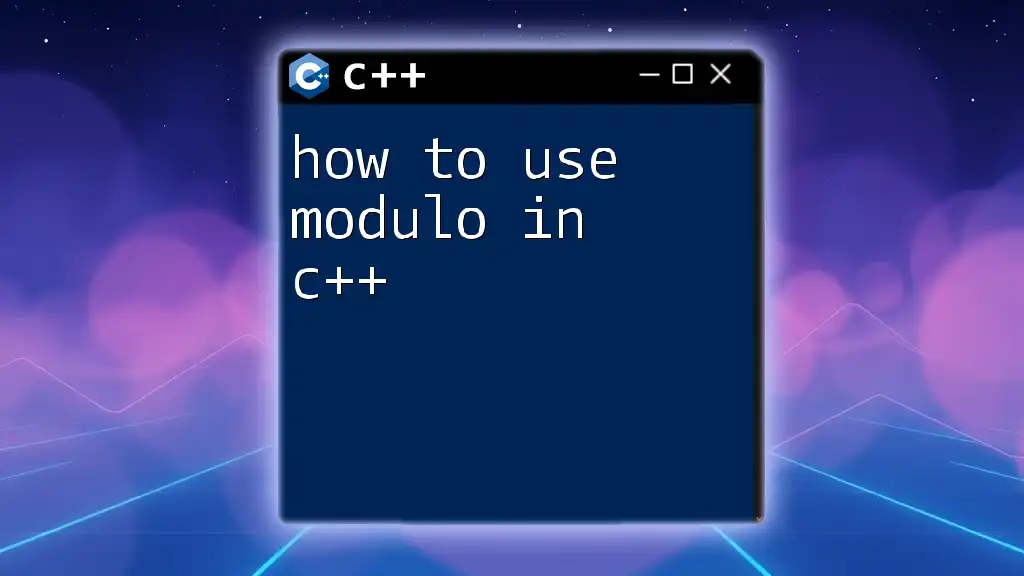
Edge Cases and Considerations
Modulus with Negative Numbers
When using the modulus operator with negative numbers, it is essential to understand how C++ handles such cases. The result of the modulus operation retains the sign of the dividend.
For example:
int a = -5;
int b = 3;
int result = a % b; // result will be -2 in C++
std::cout << "-5 % 3 = " << result << std::endl;
This behavior might vary in other programming languages, so it's crucial to be mindful of this detail when switching contexts.
Modulus with Zero
Dividing by zero is undefined in mathematics and will lead to a runtime error in C++. You need to safeguard code that uses the modulus operator to prevent attempting to divide by zero. Here’s how you can handle this:
int a = 10, b = 0;
if (b != 0) {
int result = a % b;
std::cout << "Result: " << result << std::endl;
} else {
std::cout << "Division by zero is not allowed!" << std::endl;
}
This snippet checks if `b` is not zero before performing the modulus operation, thus preventing any runtime exceptions.
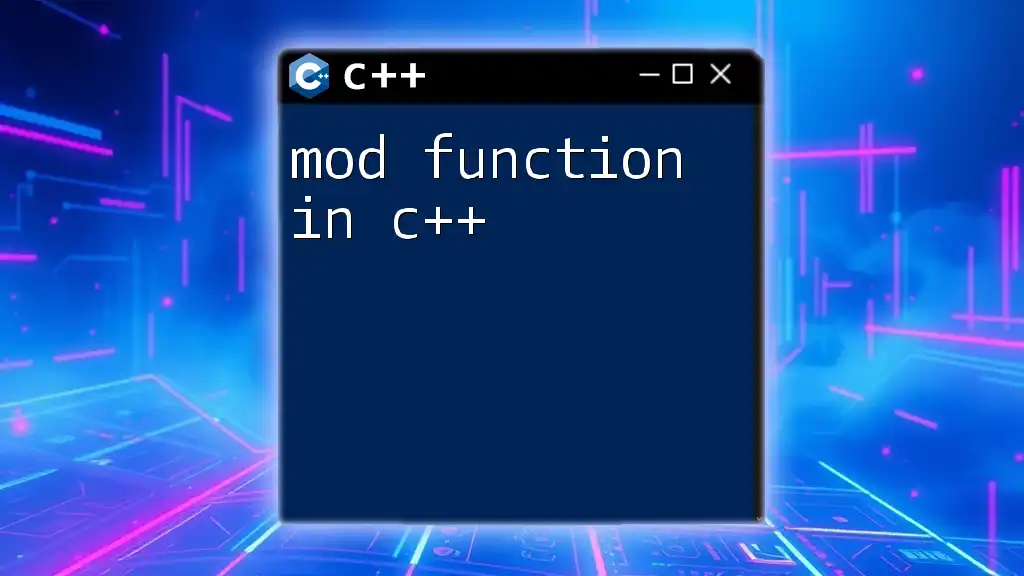
Performance Considerations
While the modulus operator is efficient for small amounts of data, it can become a performance bottleneck in high-volume applications. If you use modulus frequently in iterations, consider using alternate techniques where applicable. For example, when working with powers of two, you can use bitwise operations for enhanced performance:
int a = 10;
int powerOfTwo = 4; // 2^2
// Use bitwise AND for modulus with a power of two
int result = a & (powerOfTwo - 1); // Equivalent to a % powerOfTwo
This approach can significantly reduce computation time in scenarios where performance is critical.
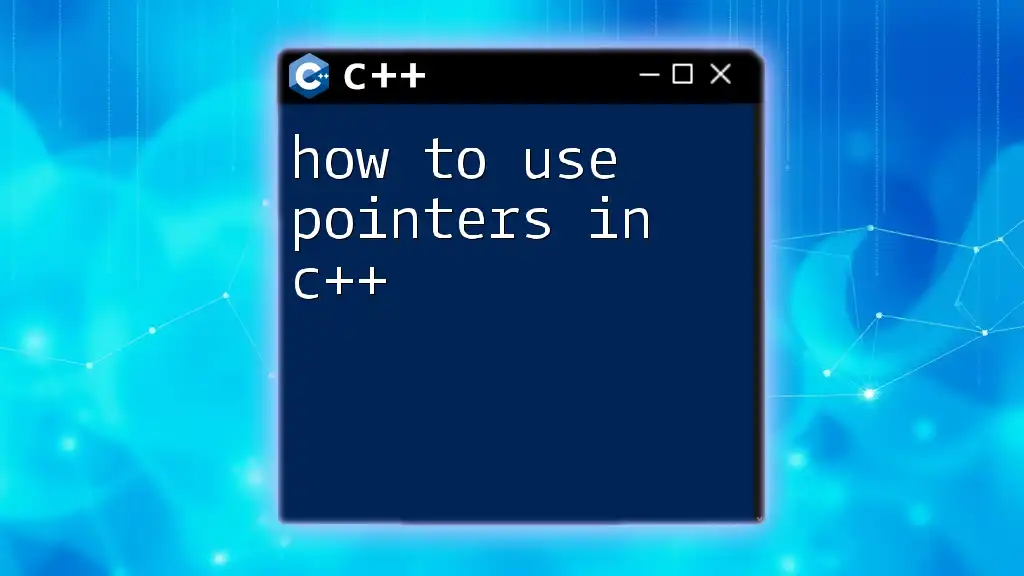
Conclusion
Understanding how to use the mod function in C++ is essential for a wide array of programming tasks. From checking evenness and oddness to handling circular indexing and ensuring safe operations, the modulus operator is a versatile tool. By being aware of its edge cases and performance implications, you can leverage it effectively in your coding endeavors. Experiment with the examples provided to reinforce these concepts and enhance your understanding of this critical operator in C++.