To add a function in C++ comments, you can use single-line comments (`//`) or multi-line comments (`/* ... */`) to describe the function's purpose and usage without impacting the code execution.
Here's a code snippet demonstrating both comment types:
// This function adds two integers and returns the result
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
/*
This function multiplies two floats and returns the result.
Usage: float result = multiply(2.5, 4.0);
*/
float multiply(float x, float y) {
return x * y;
}
Understanding C++ Comments
What are Comments in C++?
In C++, comments are snippets of text within the source code that are ignored by the compiler. They serve as annotations intended for the programmer’s benefit, helping to document what the code does, clarify complex logic, or remind developers of future improvements. This documentation is especially significant when working as part of a team or when the code may need to be revisited later.
Types of Comments
C++ supports two primary types of comments:
-
Single-Line Comments: These begin with `//` and extend to the end of the line. They are typically used for brief explanations or notes.
Example:
// This comment explains the following line of code int x = 5;
-
Multi-Line Comments: Beginning with `/` and ending with `/`, these can span multiple lines, making them ideal for longer explanations or documentation.
Example:
/* This is a multi-line comment. The lines are useful for providing detailed explanations or notes. */

Why Commenting Functions is Crucial
Enhancing Code Readability
Comments play a vital role in making your code more readable. Instead of diving directly into the logic, comments allow developers to grasp the purpose of a function instantly. They bridge the gap between complex code and human understanding, minimizing the cognitive load involved in deciphering what each part does. This is especially crucial in collaborative environments where multiple developers work on the same codebase.
Facilitating Learning and Code Review
When someone new joins a project or when peer reviews are conducted, well-commented functions make the learning curve gentler. Comments provide context that might be missing from the code itself, thereby making it easier for new developers to understand and adopt the code effectively. Moreover, during code reviews, clear comments can help explain design decisions and highlight areas that may need more scrutiny.
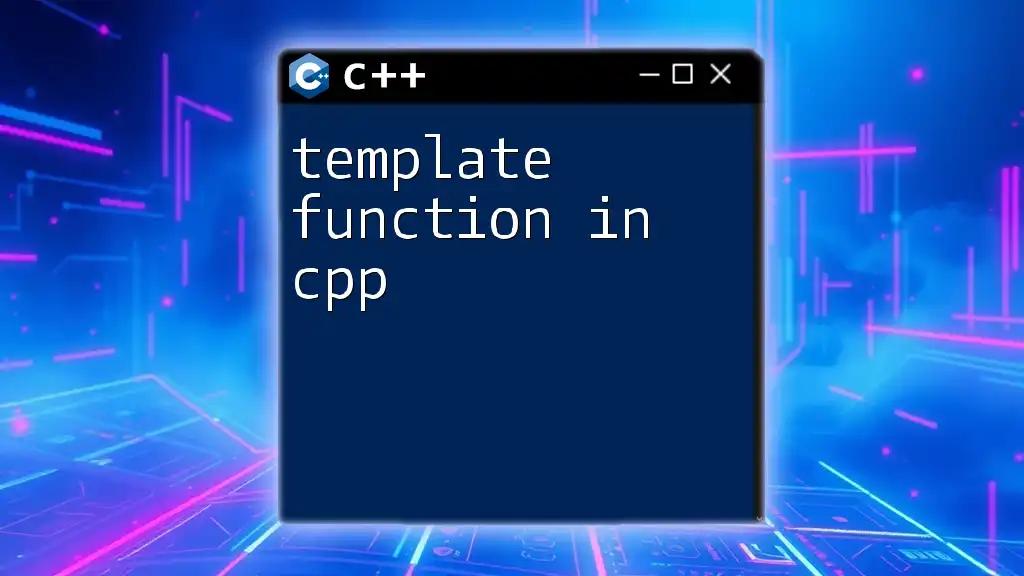
How to Use Comments for Functions
Commenting Function Definitions
A well-commented function begins with a clear description of its purpose, the parameters it accepts, and what it returns. This pattern helps anyone reading the code to quickly understand what to expect.
Here’s a practical example:
// Function to calculate the area of a rectangle
// Parameters:
// - width: Width of the rectangle (float)
// - height: Height of the rectangle (float)
// Returns:
// - Area of the rectangle (float)
float calculateArea(float width, float height) {
return width * height;
}
By organizing comments in this format, you provide all necessary information at a glance, making it easy for anyone to see the function's utility.
Commenting Inside Functions
In addition to initial comments, the logic inside the function can often be complex. Comments here can clarify each step of the process.
For instance:
float calculateArea(float width, float height) {
// Check if width and height are positive
if (width <= 0 || height <= 0) {
// Invalid dimensions
return 0;
}
// Calculate area based on the provided dimensions
return width * height;
}
In this example, comments explain the validation process and provide context for why the area is calculated, enhancing the clarity for other developers.

Best Practices for Commenting Functions
Be Concise but Descriptive
When adding comments, strive to be concise yet descriptive. Avoid writing unnecessary information that doesn't add value. Focus on key points such as the functionality, inputs, and outputs instead of restating the code.
Use Consistent Formatting
Consistency is key in maintaining readability. Adopt a uniform comment style throughout your project to aid in comprehension. If you choose a specific format, such as using one-line comments for brief notes and multi-line comments for extensive explanations, stick to that format.
Regularly Update Comments
As the code evolves, comments must also be updated to ensure relevance. Outdated comments can result in confusion, leading to misunderstandings and errors. Make it a habit to review comments alongside the code they describe, deleting or updating any that have become obsolete.
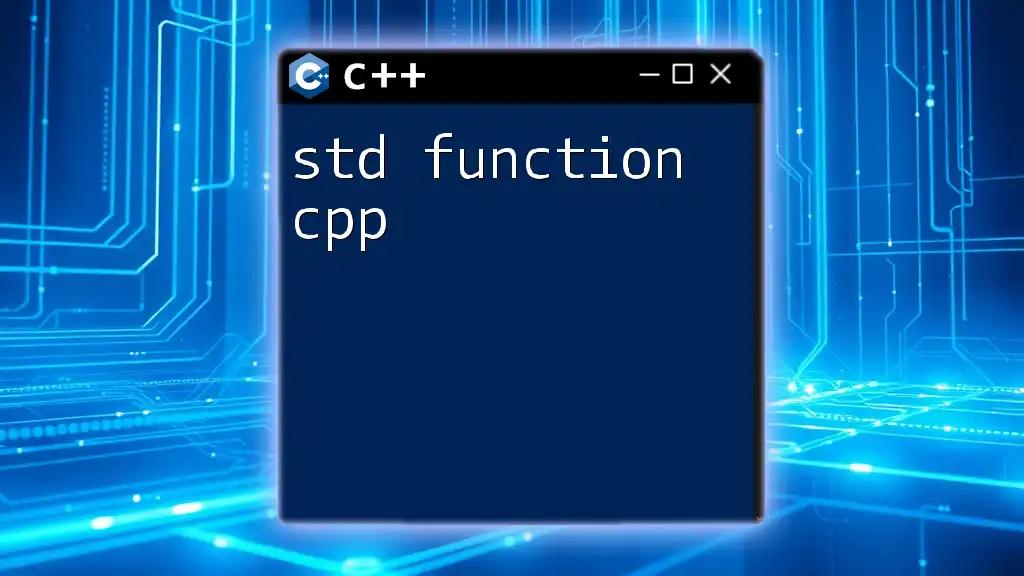
Examples of Well-Commented Functions
Example 1: Utility Function
Here’s a simple utility function with comprehensive comments:
// Function to convert Celsius to Fahrenheit
// Parameters:
// - celsius: temperature in Celsius (float)
// Returns:
// - Converted temperature in Fahrenheit (float)
float celsiusToFahrenheit(float celsius) {
return (celsius * 9.0 / 5.0) + 32;
}
This function has clear comments that describe its purpose, input, and output, making it user-friendly.
Example 2: Recursive Function
Here's an example of a recursive function that calculates the factorial of a number:
// Recursive function to compute factorial
// Parameters:
// - n: non-negative integer whose factorial is computed
// Returns:
// - Factorial of the number (int), returns 1 if n is 0
int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
The comments here delineate the function's parameters and what it returns, as well as provide clarity on the base case in the recursion.

Conclusion
In summary, knowing how to add a function in C++ comments is invaluable for enhancing code readability and facilitating better collaboration among developers. Whether you’re defining a function or documenting its logic, thorough and consistent comments can make a significant difference in how easily others (and you) can understand, maintain, and expand upon your work.
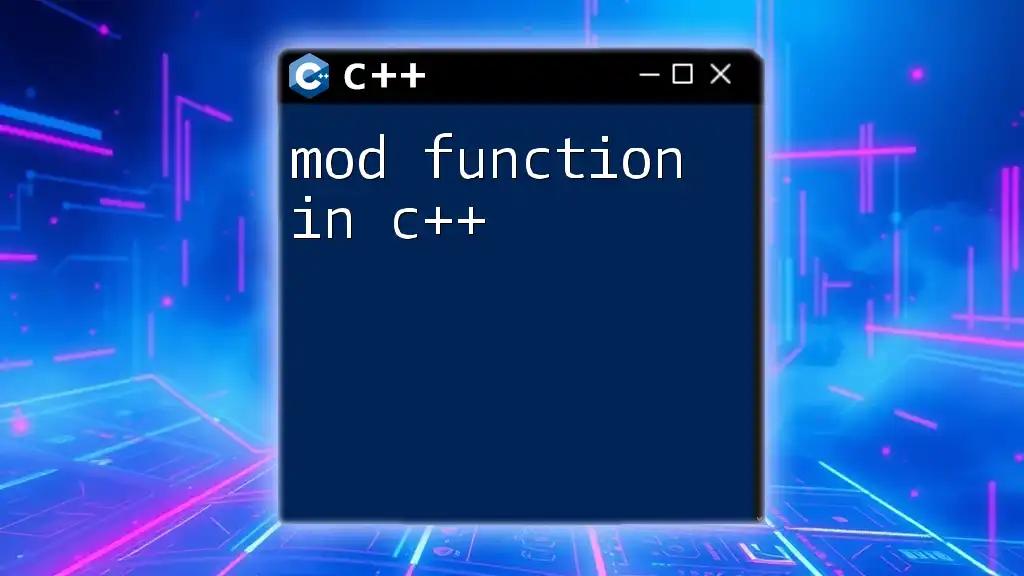
Call to Action
Take the initiative to practice commenting your functions thoughtfully. Review your existing code and enhance it with meaningful comments. Stay tuned for more insightful C++ tips and lessons to refine your coding skills!
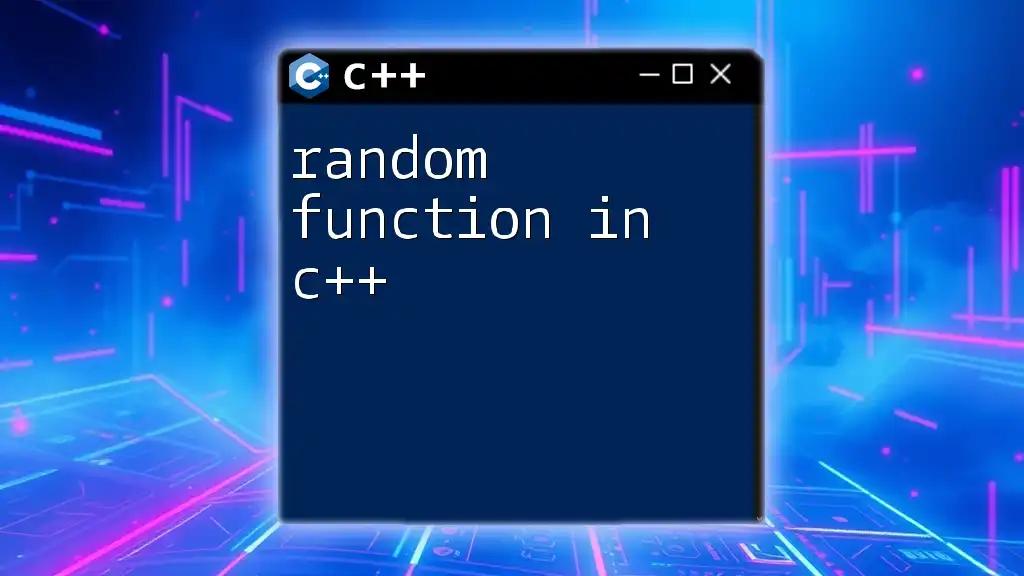
Additional Resources
For further reading, consider exploring style guides and best practices, such as the Google C++ Style Guide. These resources will deepen your understanding of effective commenting techniques and help you write clearer, more maintainable code.