To call a void function in C++, simply use the function's name followed by parentheses, as shown in the example below:
#include <iostream>
// Define a void function
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
// Call the void function
greet();
return 0;
}
Understanding Void Functions
What is a Void Function?
A void function in C++ is a type of function that does not return a value. Unlike other function types that might return integers, floating-point numbers, or other data types, void functions perform operations but do not provide any output back to the calling function. They are particularly useful for performing tasks such as outputting data to the console, modifying global variables, or executing commands without needing to return data. This characteristic makes them essential for controlling program flow and encapsulating behaviors.
Syntax of Void Functions
To define a void function, you'll need to use the following structure:
- Return type: In this case, it is `void`.
- Function name: This should be descriptive as it represents the action.
- Parameters (optional): These are inputs the function can accept.
Here is an example of a simple void function:
void myFunction() {
// Function body
}
In this example, `myFunction` is defined without any parameters and does not return a value.
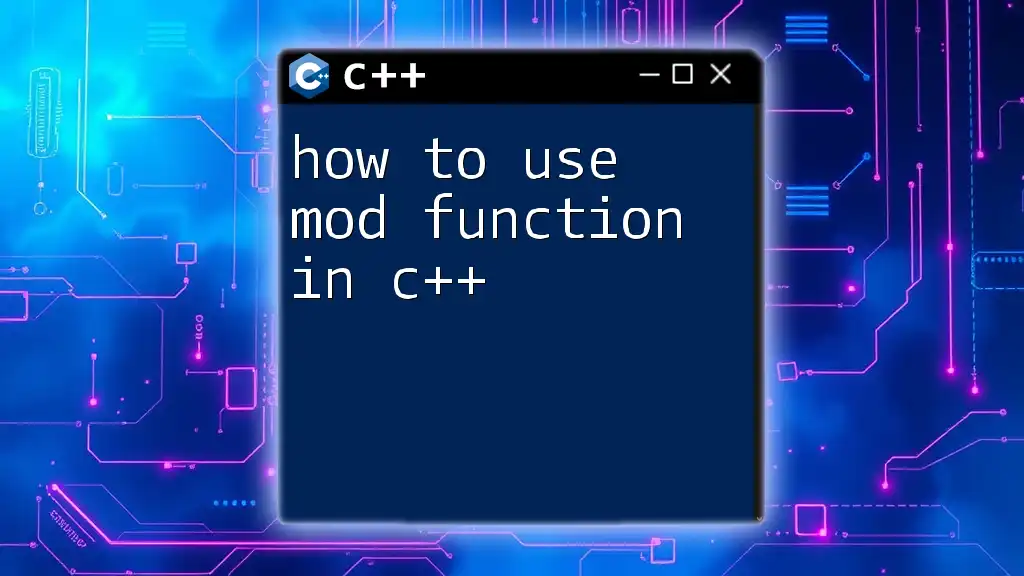
Calling Void Functions
Basics of Calling Functions
When you call a function, you are essentially instructing the program to execute the code within that function. For void functions, you simply need to state the function name followed by parentheses. If the function has parameters, you should provide arguments that match the expected data types.
Directly Calling a Void Function
Calling a void function directly is straightforward. Here's an example that illustrates this:
#include <iostream>
using namespace std;
void greet() {
cout << "Hello, world!" << endl;
}
int main() {
greet(); // Calling the void function
return 0;
}
In this code snippet, the `greet` function does not take any parameters. When we call `greet()` in the `main` function, the output will be Hello, world!. The process involves transferring control to the `greet` function, executing its body, and returning control to the calling location once finished.
Calling Void Functions with Parameters
What are Function Parameters?
Function parameters allow you to pass information to a function. They provide a mechanism for functions to perform operations based on input values, enabling the same function to handle different data.
Calling a Void Function with Parameters
Here’s an example of a void function that accepts a parameter:
void displayMessage(string message) {
cout << message << endl;
}
You can call this function from the `main` function as follows:
int main() {
displayMessage("Welcome to C++ programming!");
return 0;
}
When you call `displayMessage`, you pass the string "Welcome to C++ programming!" as an argument, and the program will output that message. It’s crucial to ensure that the argument provided matches the parameter type specified in the function declaration.
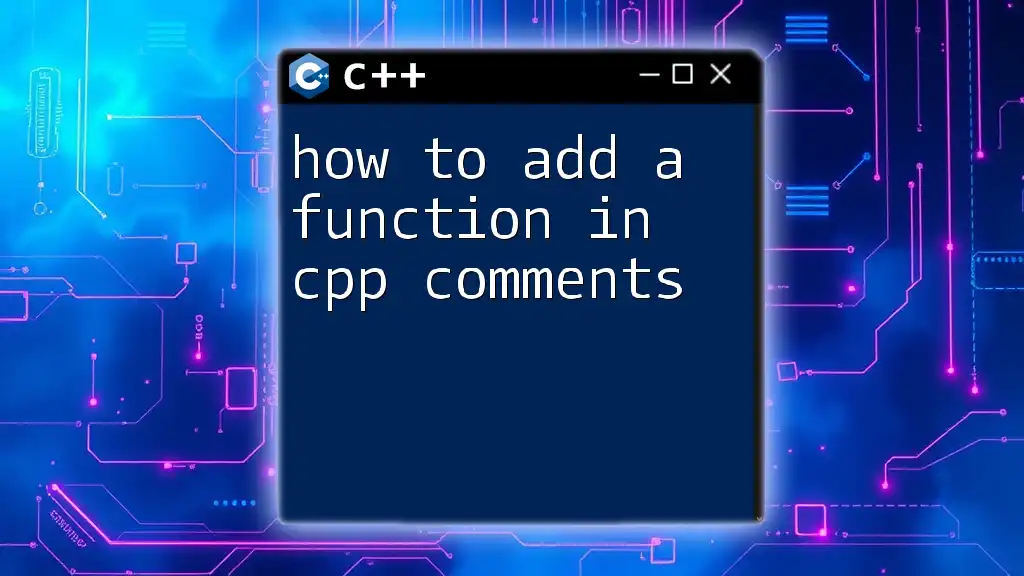
The Role of Overloading in Function Calls
What is Function Overloading?
Function overloading in C++ allows you to define multiple functions with the same name but with different parameter lists. This is particularly useful for void functions, as it enables the same function name to handle different data types or numbers of parameters without confusion.
Example of Overloaded Void Functions
Consider this example of overloaded void functions:
void display(int number) {
cout << "Number: " << number << endl;
}
void display(string text) {
cout << "Text: " << text << endl;
}
Calling Overloaded Void Functions
You can call these overloaded functions based on the type of argument you provide:
int main() {
display(10); // Calls the first version
display("Hello, overload!"); // Calls the second version
return 0;
}
The C++ compiler determines which function to call based on the argument types, showcasing the flexibility of overloading in managing function calls effectively.
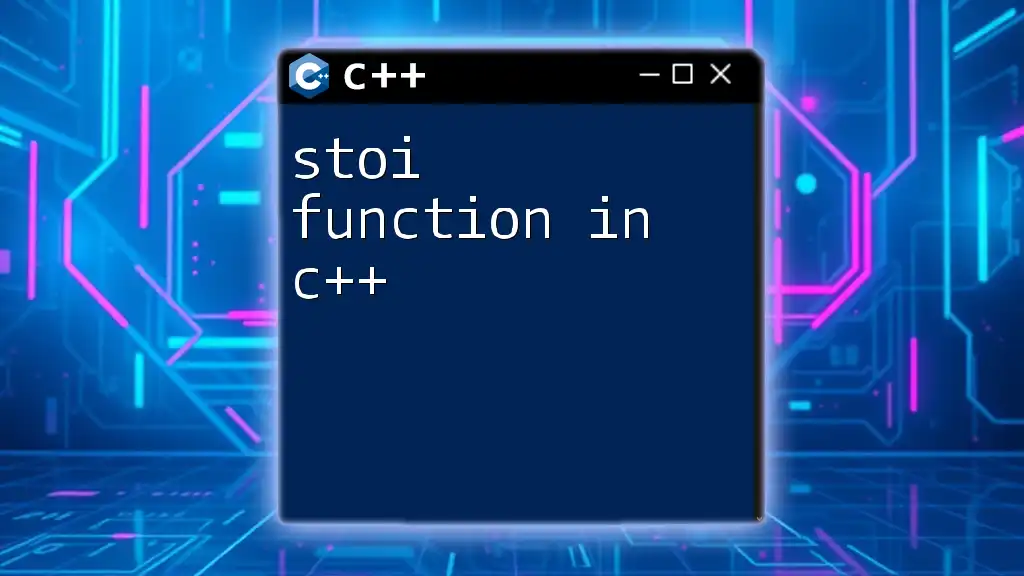
Error Handling when Calling Functions
Common Mistakes in Function Calls
When working with function calls, several common errors can arise:
- Mismatch of Parameters: If you do not provide the correct number or type of arguments, the compiler will throw an error.
- Failure to Declare Functions: If you call a function that hasn't been declared, you'll encounter a linking error.
Debugging Tips
To troubleshoot such issues:
- Double-check the parameters being passed to ensure they match the expected types and quantities.
- Use simple, isolated test cases to ensure individual functions work as intended before integrating them into larger programs.
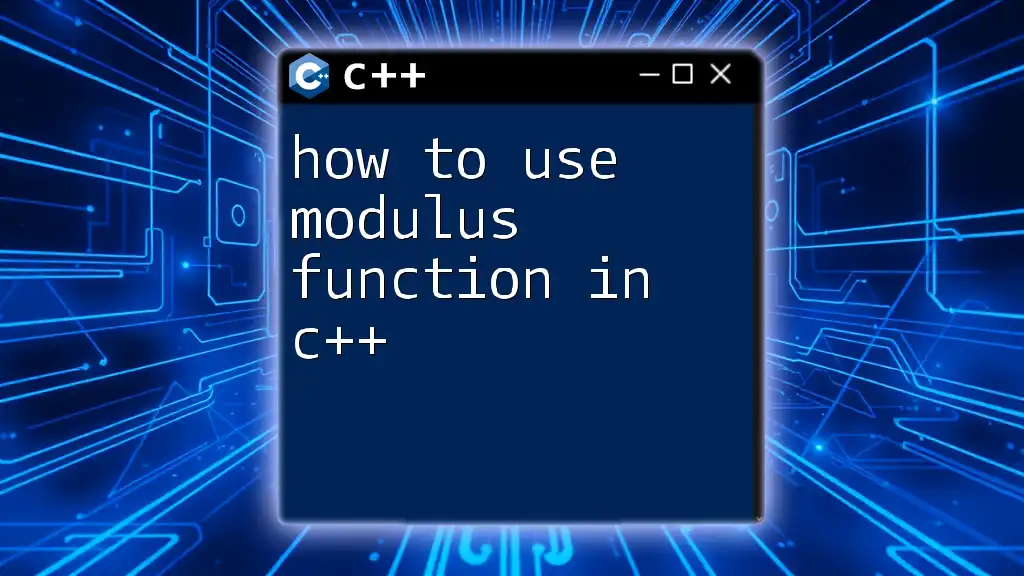
Conclusion
In this guide, we've explored how to call a void function in C++, covering definitions, syntax, parameter passing, and function overloading. Understanding these concepts is fundamental in writing effective, organized, and functional code in C++.
I encourage you to practice calling void functions in various scenarios to solidify your understanding. Begin implementing what you've learned today as you explore the depths of C++ programming!
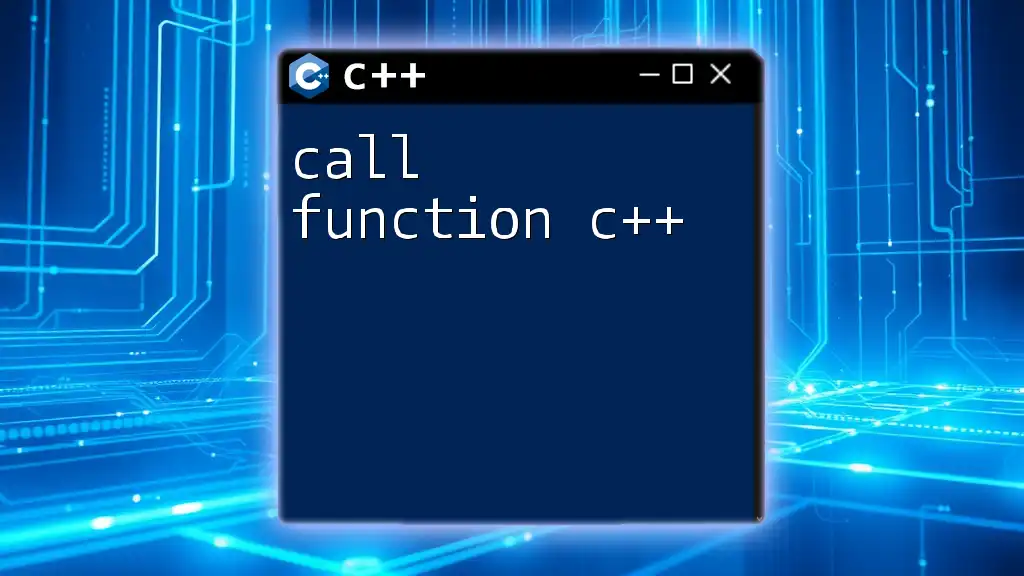
Additional Resources
Books and Tutorials
- Look for recommended literature on C++ programming for deeper insights.
Online Courses
- Explore different platforms offering structured C++ courses for comprehensive learning.
Community Forums
- Join C++ programming forums or communities where you can share knowledge, ask questions, and seek support as you continue your learning journey.
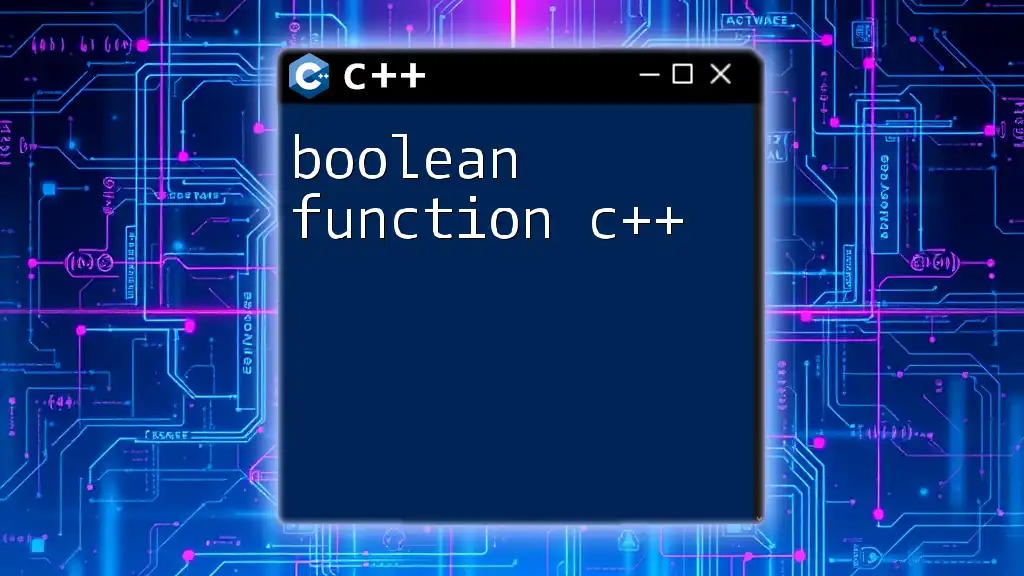
FAQs
What happens if I call a void function without necessary arguments?
If a void function requires parameters and you attempt to invoke it without providing the necessary arguments, the compiler will generate an error during the compilation phase. It’s essential to match the function call with the expected parameter signature.
Can I return a value from a void function?
No, you cannot return a value from a void function. Attempting to do so will result in a compilation error. Best practice dictates that you should clearly define whether a function should return a value or purely perform an action. If you need to return a value, consider changing the return type from void to the appropriate type for your needs.