The modulus operator in C++ is used to find the remainder of a division operation between two integers, represented by the percentage symbol (%).
Here’s a simple example:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a % b; // result will be 1
std::cout << "The remainder of 10 divided by 3 is: " << result << std::endl;
return 0;
}
Understanding the Modulus Operator
What is the Modulus Operator?
The modulus operator, represented by the symbol `%`, is a fundamental arithmetic operator in C++. It allows you to find the remainder of a division between two integers.
For instance, when you divide 5 by 2, the problem can be expressed as follows:
5 divided by 2 equals 2 with a remainder of 1.
Thus, `5 % 2` results in 1, showcasing how the modulus operator helps us in determining the remainder.
Basic Concept of Modulus
The modulus operator is defined mathematically as:
remainder = dividend % divisor
This operation is crucial in various programming tasks, such as determining whether a number is even or odd.
Example
A simple example using small integers can clarify the usage:
#include <iostream>
int main() {
int a = 10, b = 3;
int result = a % b;
std::cout << "10 % 3 = " << result; // Output: 1
return 0;
}
In this example, `10 % 3` confirms that the remainder of dividing 10 by 3 is indeed 1.
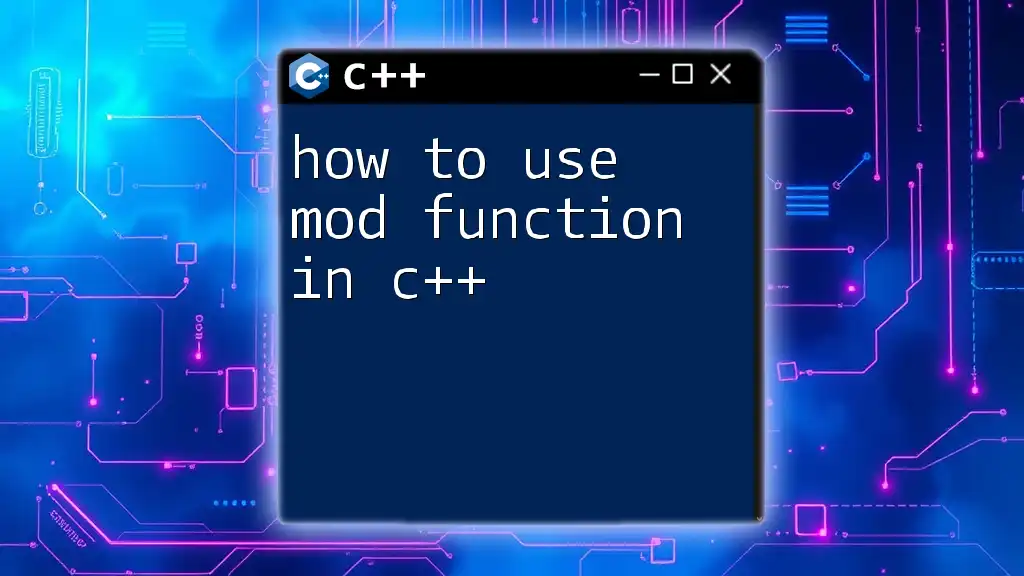
How to Use the Modulus Operator in C++
Syntax of the Modulus Operator
The basic syntax for using the modulus operator is as follows:
remainder = dividend % divisor;
Each component serves a specific role:
- `dividend`: This is the number you want to divide.
- `divisor`: This is the number by which you want to divide the dividend.
Examples of Modulus in Action
Simple Use Cases
You can see how to apply this in a practical scenario:
#include <iostream>
int main() {
int a = 10, b = 3;
int result = a % b;
std::cout << "10 % 3 = " << result; // Output: 1
return 0;
}
This confirms our earlier example, clearly demonstrating the core functionality of the modulus operator.
Practical Scenarios
Another practical use for the modulus operator is checking for even or odd numbers. Here’s how you can implement this:
#include <iostream>
int main() {
int number = 7;
if (number % 2 == 0) {
std::cout << number << " is even.";
} else {
std::cout << number << " is odd."; // Output: 7 is odd.
}
return 0;
}
In this case, `7 % 2` gives us a remainder of 1, confirming that 7 is odd.
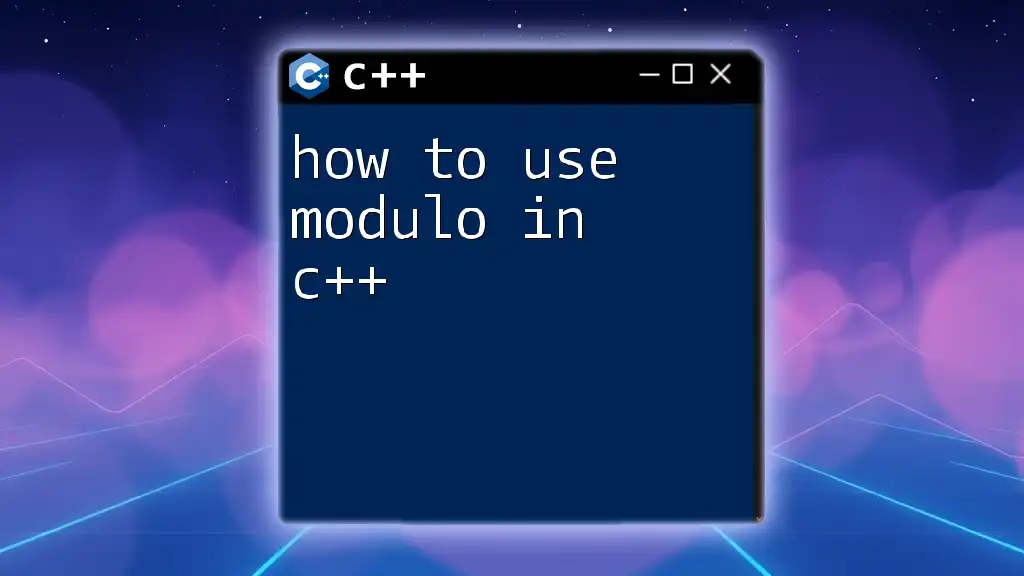
Advanced Applications of Modulus
Modulus with Negative Numbers
Using the modulus operator with negative numbers can be somewhat counterintuitive. Here’s how it behaves:
#include <iostream>
int main() {
int a = -10, b = 3;
int result = a % b;
std::cout << "-10 % 3 = " << result; // Output: -1
return 0;
}
In this example, the result is -1. It’s crucial to understand how negative dividends affect outcomes, as this is often a source of confusion.
Modulus in Loops
Using Modulus for Iteration Control
The modulus operator can be instrumental in loop iterations when you need to cycle through a set of values. Consider the following example that uses modulus to produce a repeating sequence:
#include <iostream>
int main() {
for (int i = 0; i < 10; ++i) {
std::cout << i << " modulo 3 = " << i % 3 << "\n";
}
return 0;
}
The output will show how the remainder of each iteration when divided by 3 produces a repeating pattern, valuable in arrays or circular structures.
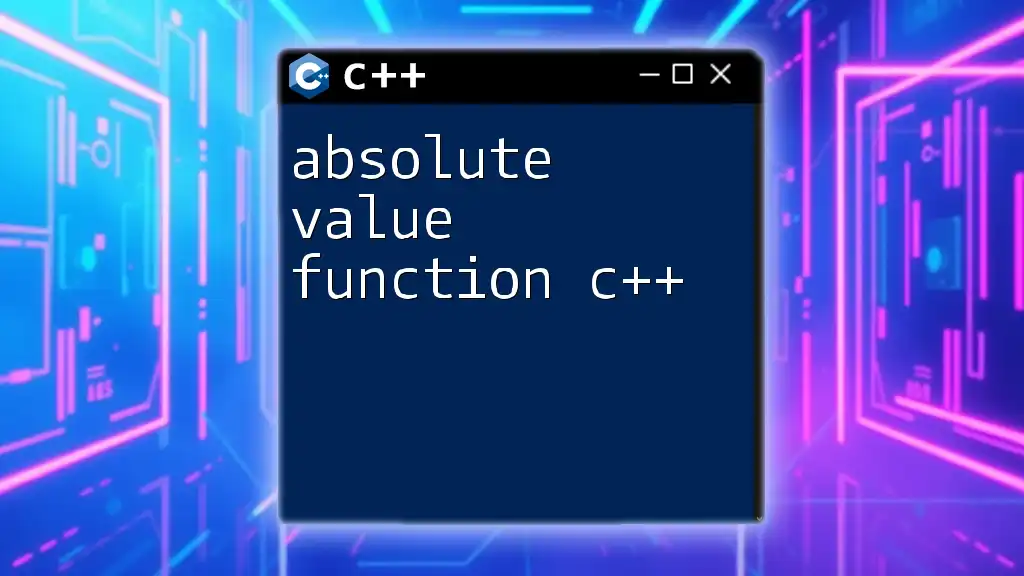
Common Pitfalls and Misunderstandings
Misusing Modulus with Zero
One of the most critical concepts to grasp is that dividing by zero causes an error, which we must avoid. Here’s a quick illustration:
#include <iostream>
int main() {
int a = 10, b = 0;
// int result = a % b; // Uncommenting this will cause an error
std::cout << "Attempting division by zero.";
return 0;
}
In this case, attempting to perform `10 % 0` will lead to a runtime error, making it essential to check the value of the divisor before applying the modulus operator.
Floating Point Numbers and Modulus
While the modulus operator is primarily designed for integers, if you wish to work with floating-point numbers, you can use the `fmod()` function from the `<cmath>` library to achieve a similar result:
#include <iostream>
#include <cmath>
int main() {
double x = 5.5, y = 2.2;
double result = fmod(x, y);
std::cout << "5.5 fmod 2.2 = " << result; // Output: 1.1
return 0;
}
It’s important to note that while `fmod()` provides similar functionality for floating-point numbers, its behavior and rounding nuances can differ from integer modulus operations.
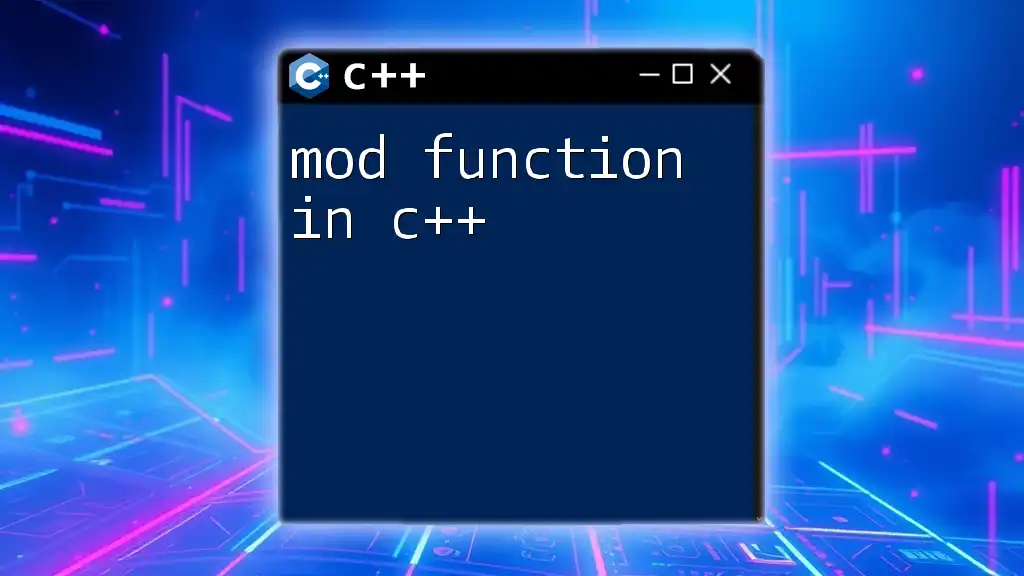
Conclusion
The modulus operator is a powerful tool in C++ programming. Understanding how to use the modulus function in C++ can significantly enhance your coding capabilities, enabling you to solve various arithmetic and logical problems efficiently.
By practicing its applications and being aware of common pitfalls, you can master the use of the modulus operator and integrate it effectively into your projects. Don't hesitate to experiment with your own examples or explore additional resources for deeper learning!