The absolute value function in C++ can be implemented using the built-in `abs()` function, which returns the non-negative value of a given number.
#include <iostream>
#include <cstdlib> // For abs()
int main() {
int number = -10;
int absoluteValue = abs(number);
std::cout << "The absolute value of " << number << " is " << absoluteValue << std::endl;
return 0;
}
What is the Absolute Value Function?
The absolute value function is a mathematical function that transforms a number into its positive counterpart. This means that regardless of whether a number is positive or negative, its absolute value will always be non-negative. For instance, the absolute values of both 5 and -5 are the same, which is 5. In programming, particularly in C++, knowing how to efficiently utilize the absolute value function is essential in various mathematical computations and algorithms.
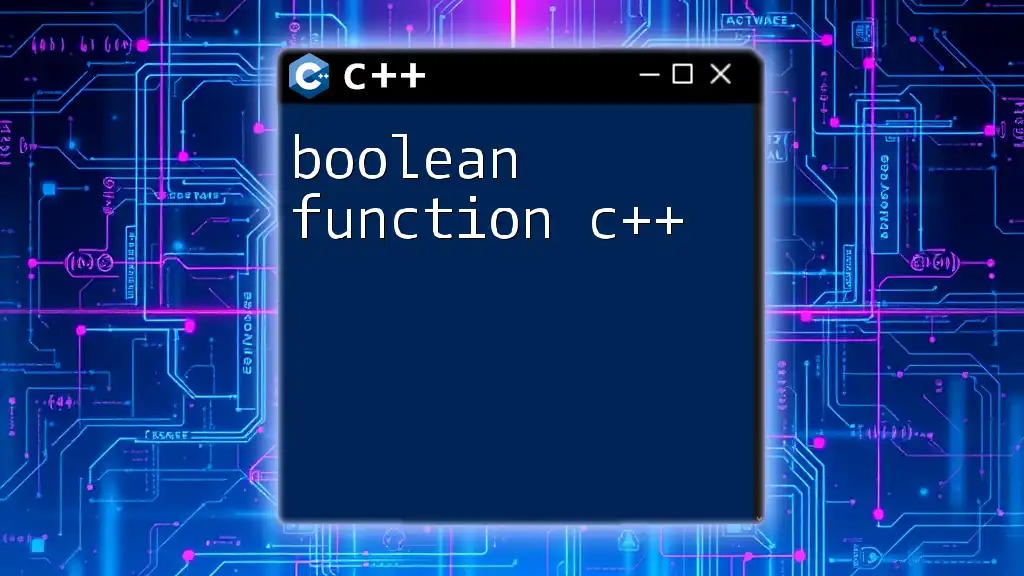
The `abs` Function in C++
In C++, the built-in `abs` function is a straightforward way to compute the absolute value of integers. It is defined in the `<cstdlib>` header file. For floating-point numbers, you should use `fabs`, which is included in the `<cmath>` header.
To include these functions in your program, add the following directives:
#include <cstdlib> // For integers
#include <cmath> // For floating-point numbers
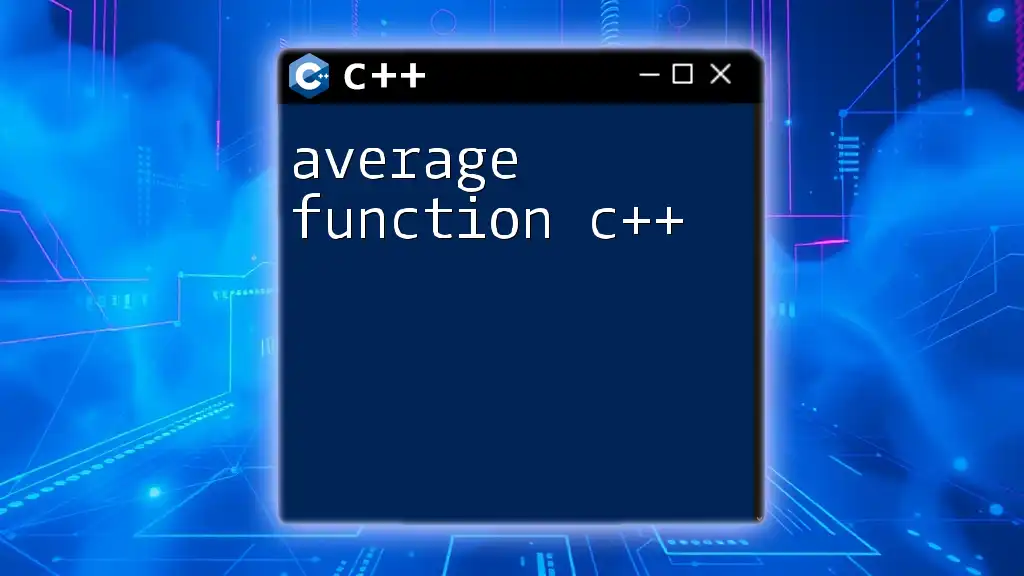
Usage of the `abs` Function in C++
Syntax
The syntax for using the `abs` function is simple:
abs(x);
Where `x` can be an integer, and the function returns the absolute value of `x`.
Example with Integer
Here’s how you can use the `abs` function with integers:
int main() {
int num = -5;
int absoluteValue = abs(num); // calls abs function
std::cout << "The absolute value of " << num << " is " << absoluteValue << std::endl;
return 0;
}
Explanation: In this example, the variable `num` is assigned a negative value (-5). The `abs` function processes this value, and since `-5` has an absolute value of `5`, the output will be:
The absolute value of -5 is 5
Example with Floating-Point Numbers
When working with floating-point numbers, the `abs` function can’t be used directly; instead, utilize `fabs`:
int main() {
double num = -3.14;
double absoluteValue = fabs(num); // calls fabs for floating-point
std::cout << "The absolute value of " << num << " is " << absoluteValue << std::endl;
return 0;
}
Explanation: The floating-point variable `num` is set to -3.14. The `fabs` function calculates the absolute value, resulting in:
The absolute value of -3.14 is 3.14
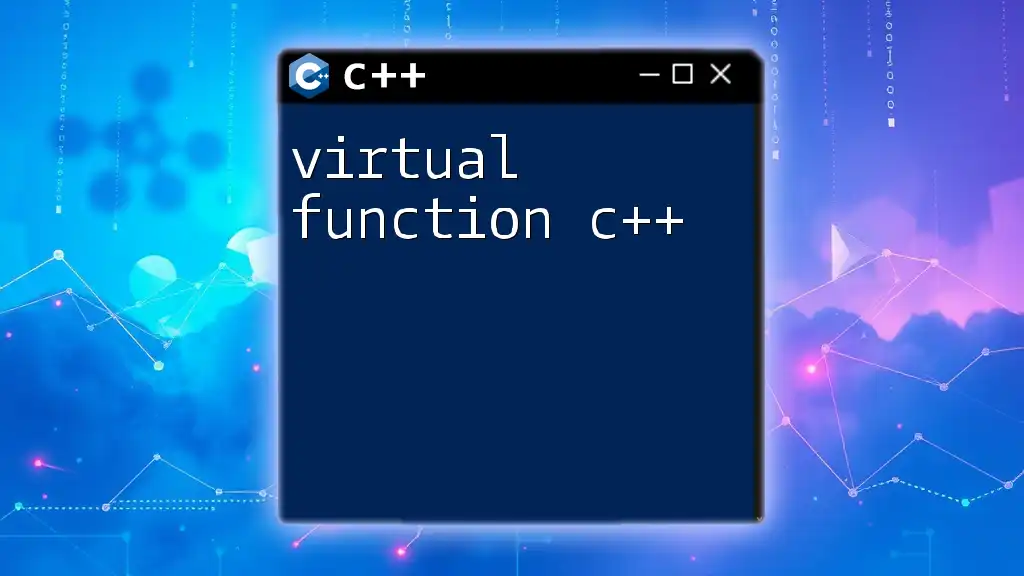
Creating a Custom Absolute Function
In some scenarios, it might be necessary to create your own implementation of an absolute value function. This can be useful for educational purposes or in specific contexts where you may require additional functionality.
Code Example
Here’s a simplistic approach to creating a custom absolute function:
int customAbs(int number) {
return (number < 0) ? -number : number;
}
int main() {
std::cout << "Custom Absolute Value: " << customAbs(-10) << std::endl;
return 0;
}
Explanation: The `customAbs` function checks if the provided integer is less than zero. If so, it returns the negative of that number to convert it into a positive value; otherwise, it returns the number itself. The output for `customAbs(-10)` will be:
Custom Absolute Value: 10
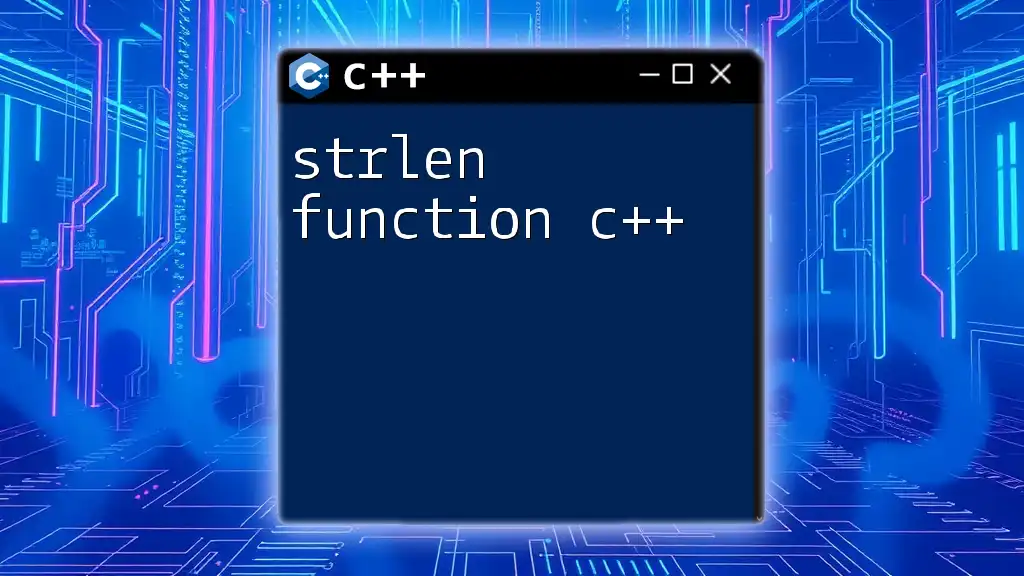
Performance Considerations
When working with absolute values, it’s generally more efficient to use the built-in functions (`abs` for integers and `fabs` for floats) rather than writing your own. Built-in functions are optimized for performance and include various checks that ensure they handle edge cases more gracefully.
Best Practices
- Use Built-in Functions: Whenever possible, default to using the built-in functions to maintain code simplicity and efficiency.
- Think About Type: Always ensure you’re using the correct function (`abs` vs. `fabs`) for the data type you are dealing with.
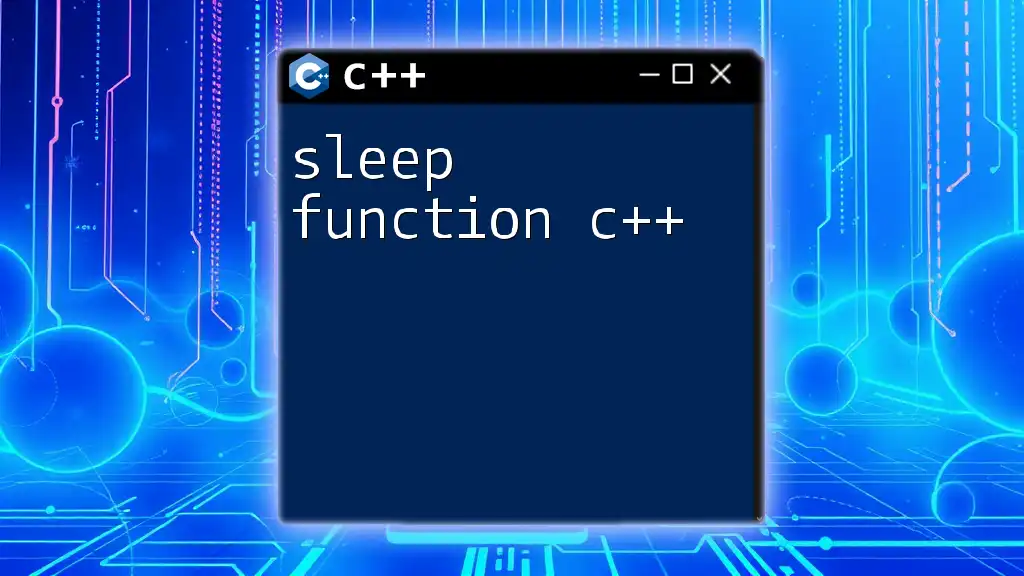
Common Mistakes When Using Absolute Value Functions
- Forgetting the Include Directive: Always add the required header files before calling absolute value functions.
- Using Incorrect Function for Type: Using `abs` for floating-point types can lead to unexpected behavior. Remember to use `fabs` for doubles or floats.
- Overcomplicating with Custom Functions: Unless you have a specific need, avoid reinventing the wheel when built-in functions already exist.
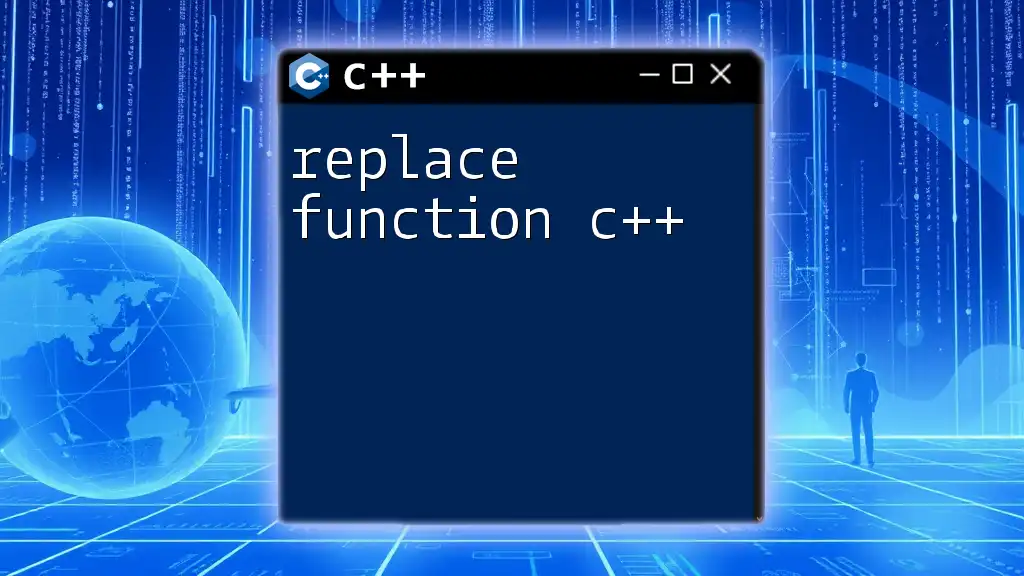
Alternatives to Absolute Value Functions
If you are unable or choose not to use the built-in functions, you can perform a manual check to achieve the same result. Here’s a manual method:
int manualAbs(int number) {
return (number < 0) ? -number : number;
}
This example replicates what the built-in `abs` function does, demonstrating that while alternatives exist, they may not always be as efficient or straightforward.
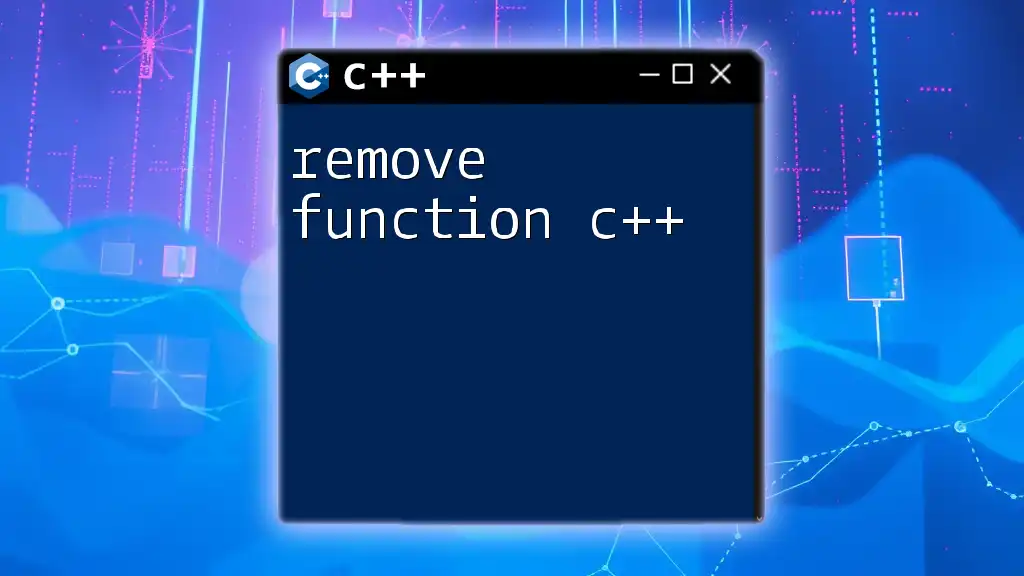
Conclusion
Understanding the absolute value function in C++ is fundamental to performing robust mathematical operations within your programs. By leveraging built-in functions like `abs` and `fabs`, you can maintain your code's efficiency and clarity while effectively managing numerical values. Experimenting with both built-in and custom functions will deepen your grasp of C++ programming and offer invaluable experience in handling different data types.
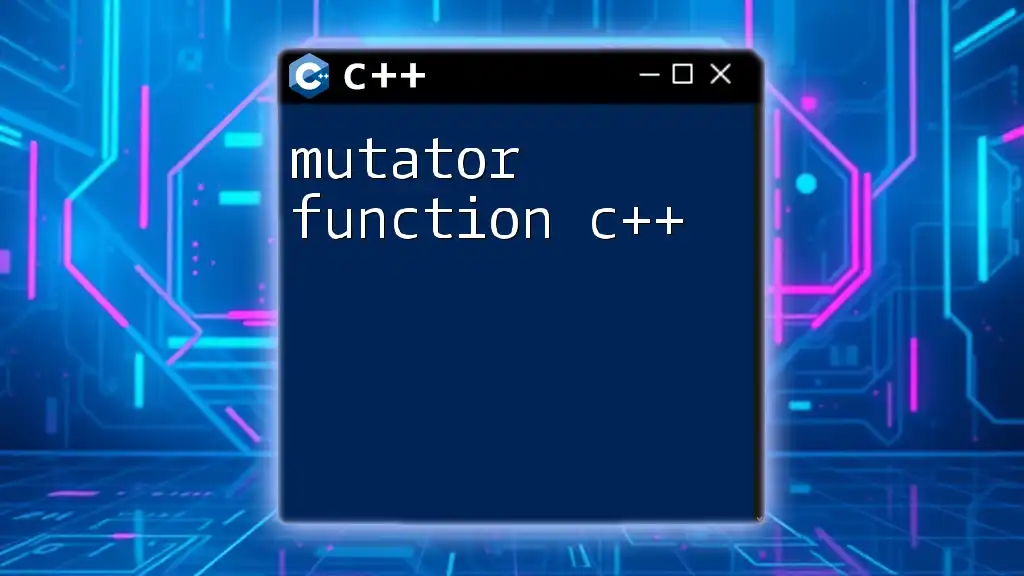
Additional Resources
For those looking to further expand their knowledge, consider exploring books on C++ programming, following online tutorials, or participating in C++ programming communities where you can share insights and ask questions. Engaging with the community will accelerate your learning and provide practical insights that enhance your coding proficiency.