To open a file in C++, you can use the `fstream` library along with an `ifstream` object for reading or an `ofstream` object for writing, as demonstrated in the code snippet below:
#include <fstream>
int main() {
std::ifstream inputFile("example.txt"); // For reading
std::ofstream outputFile("output.txt"); // For writing
return 0;
}
Understanding File Streams in C++
What are File Streams?
File streams are an essential component of C++ programming that enable efficient data input and output operations. They act as intermediaries between your program and data stored in files, allowing for various file manipulations like reading, writing, and appending data.
Types of File Streams
In C++, there are three primary types of file streams:
- Input File Stream (`ifstream`): Used for reading data from files.
- Output File Stream (`ofstream`): Used for writing data to files.
- Input/Output File Stream (`fstream`): Used for both reading and writing operations on files.
By understanding these file stream types, you will be prepared to deal with files effectively.
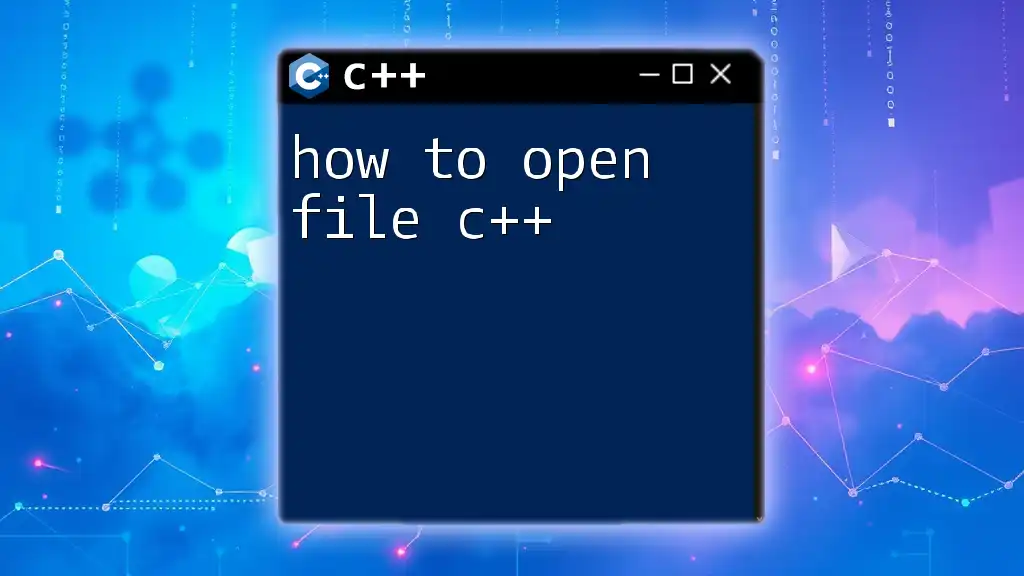
Opening Files in C++
The Basics of Opening a File in C++
To begin any file operation in C++, you must first open the file. This involves using the appropriate file stream and the `open()` method.
The syntax for opening a file generally looks like this:
std::ifstream inputFile("filename.txt");
In this example, you're opening a file named `filename.txt` in read mode. It's crucial to check if the file has opened successfully; otherwise, your program may encounter errors when trying to read from or write to a file.
Example: Basic File Opening
Here’s a simple code example that demonstrates how to open a file and check for successful opening:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (!file.is_open()) {
std::cerr << "Error opening file." << std::endl;
return 1;
}
std::cout << "File opened successfully!" << std::endl;
file.close();
return 0;
}
In this snippet, if the file fails to open, an error message is printed, and the program exits. Otherwise, it confirms that the file opened correctly.
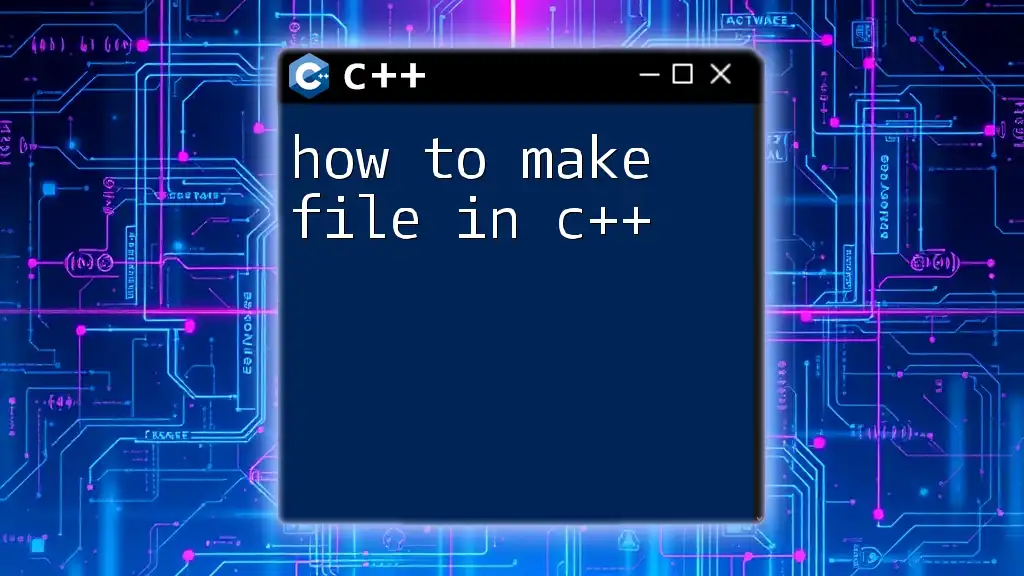
Different Modes to Open a File in C++
Reading from a File
When you want to read data from a file, you would use the input file stream. The most basic way to open a file in read mode is:
std::ifstream file("example.txt", std::ios::in);
This tells C++ that you intend to read from `example.txt`.
Writing to a File
To write data to a file, you would use `ofstream`. Here's how to do it:
std::ofstream file("output.txt", std::ios::out);
This opens `output.txt` for writing. If the file does not exist, it will be created.
Appending to a File
If you want to add data to an existing file without overwriting its current contents, you can open it in append mode like this:
std::ofstream file("output.txt", std::ios::app);
This way, any new data you write will be added to the end of the file.
Combination of Modes
If you need both reading and writing capabilities, you can use `fstream` as follows:
std::fstream file("example.txt", std::ios::in | std::ios::out);
This allows you to read from and write to the file in a single operation.
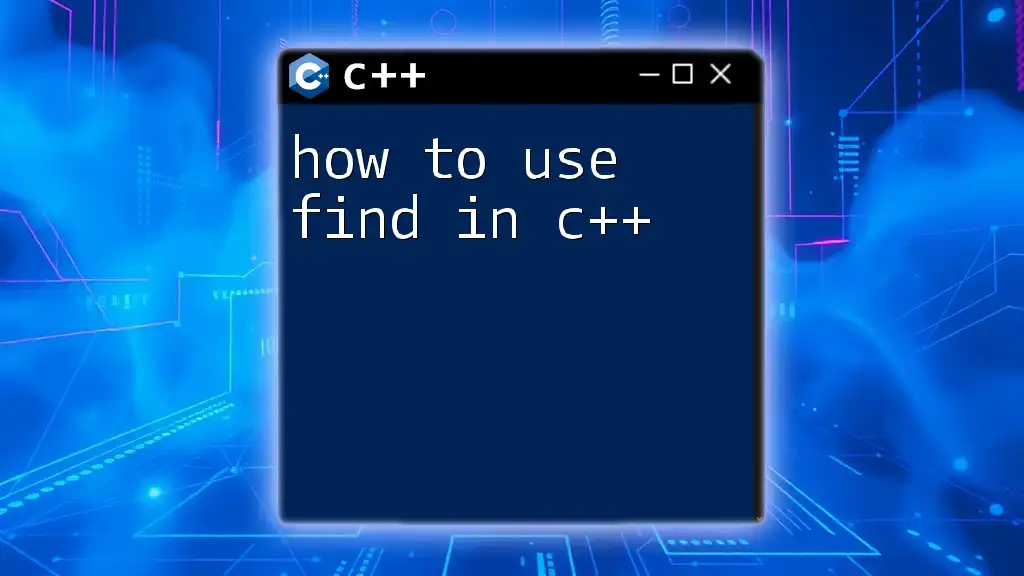
Error Handling When Opening Files in C++
Common Errors and Solutions
Opening files can sometimes lead to errors. Common issues might include:
- File Not Found: The specified file does not exist in the given path.
- No Permission: The program does not have permission to access the file.
- Disk Space Issues: Insufficient disk space may prevent writing to a file.
Best Practices for Error Handling
When handling files, it’s good practice to include error checking. Use `std::cerr` for capturing error messages and implement validation checks right after attempting to open a file. Here’s an example:
std::ifstream file("example.txt");
if (!file) {
std::cerr << "Error: File could not be opened." << std::endl;
}
This snippet ensures that you are promptly informed if the file cannot be opened.
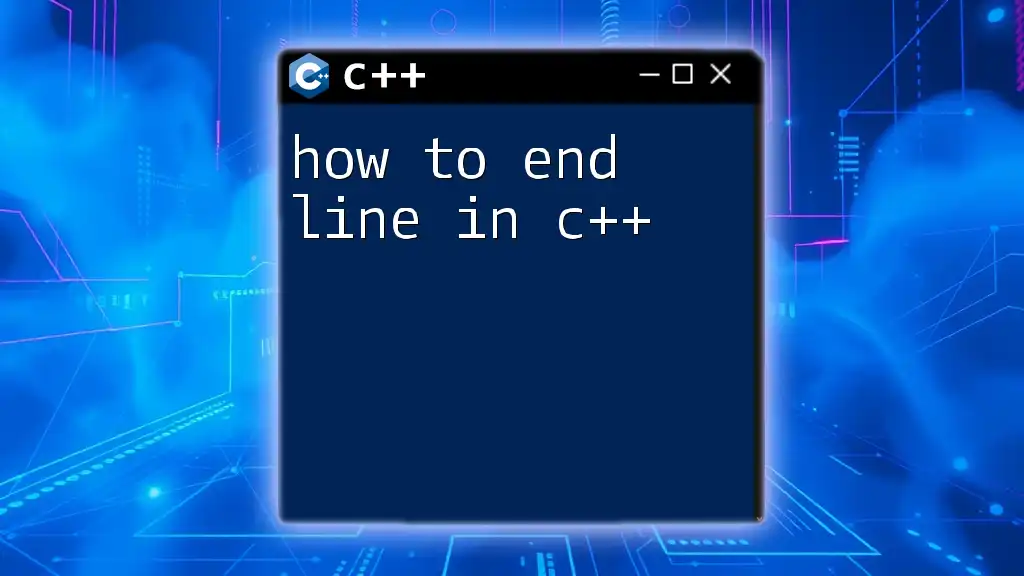
Closing a File in C++
Importance of Closing Files
After you finish your file operations, it's crucial to close the file to prevent data loss and free up system resources. Leaving files open can lead to memory leaks and file corruption.
How to Properly Close a File
Closing a file is straightforward in C++. Simply call the `close()` function on your file stream object:
file.close();
Always remember to close your files to maintain the integrity of your program.
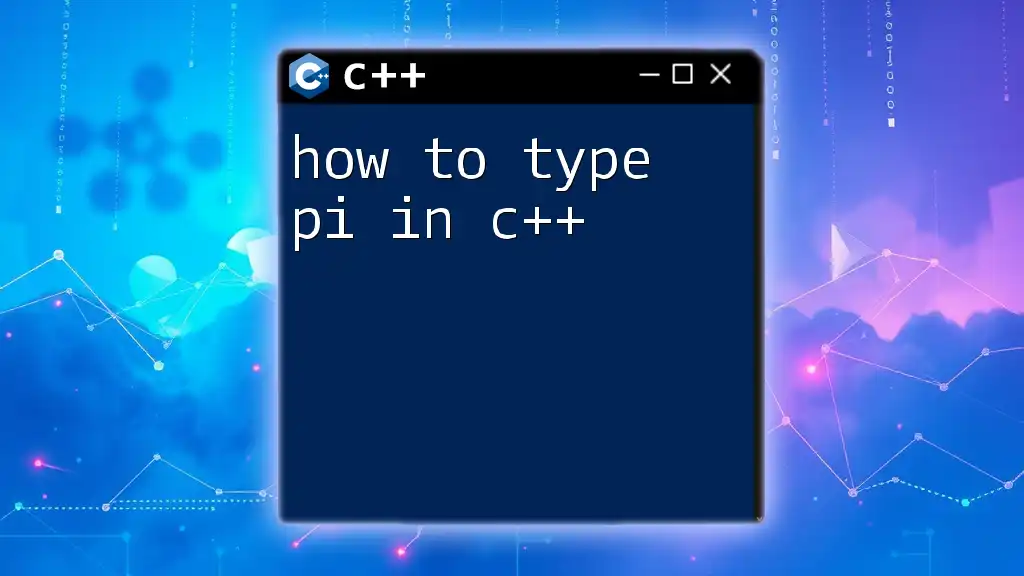
Conclusion
Understanding how to open a file in C++ is foundational for effective file handling within your applications. By mastering the types of file streams, different modes for opening files, and error handling best practices, you'll be well-equipped to manage file operations efficiently.
With practice, you can further explore advanced file operations and stream manipulations to enhance your C++ programming skills!