To create a file in C++, you can use the ofstream class to open or create a file and write data to it, as shown in the following code snippet:
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
if (outFile) {
outFile << "Hello, world!" << std::endl; // Write to the file
outFile.close(); // Close the file
}
return 0;
}
Understanding File Streams in C++
What are File Streams?
In C++, file streams are essential for reading from and writing to files. They function similarly to the `cin` and `cout` streams used for console input and output. When working with files, we typically handle two types of streams:
- Input Stream (ifstream): Used to read data from files.
- Output Stream (ofstream): Used to write data to files.
The C++ Standard Library for File Handling
To leverage file handling capabilities in C++, the `<fstream>` header file is crucial. This library provides three main classes:
- ifstream: For reading files.
- ofstream: For writing files.
- fstream: For both reading and writing files.
Each of these classes uses the principles of stream I/O to interact with the filesystem seamlessly.
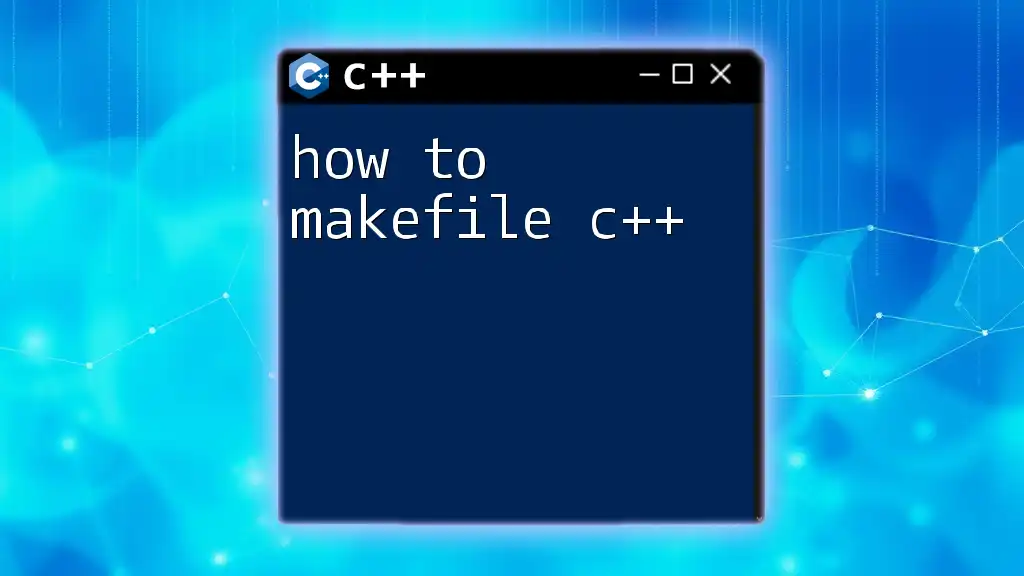
Step-by-Step Guide: Creating a File in C++
Setting Up the Environment
Before writing any code, ensure you have a working C++ development environment. Popular IDEs like Code::Blocks, Visual Studio, or online compilers can help streamline the coding process.
Writing Your First C++ Program to Create a File
To write a file in C++, you’ll primarily work with the `ofstream` class. Here is a simple example that demonstrates how to create a text file and write to it:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
if (!outFile) {
std::cerr << "Error creating file!" << std::endl;
return 1;
}
outFile << "Hello, World!" << std::endl;
outFile.close();
return 0;
}
Explanation:
- Including Libraries: The `#include <fstream>` directive includes the file stream functionality we need.
- Creating the ofstream Object: The line `std::ofstream outFile("example.txt");` creates a new file named `example.txt`. If it doesn't already exist, it will be created.
- Error Checking: It's essential to check if the file was created successfully. The condition `if (!outFile)` allows us to handle any errors that may arise during file creation.
- Writing to the File: `outFile << "Hello, World!" << std::endl;` writes the string to the file.
- Closing the File: Always remember to close the file using `outFile.close();`. This action ensures that all data is flushed and resources are released.
Error Handling When Creating a File
Handling errors is a critical part of working with files. You might encounter several potential issues, such as:
- Insufficient permissions: Ensure you have permission to create files in the directory you are working in.
- Existing file conflicts: Be aware that creating a file with the same name as an existing file will overwrite it.
Using `std::cerr` helps display error messages in the console, making debugging easier.
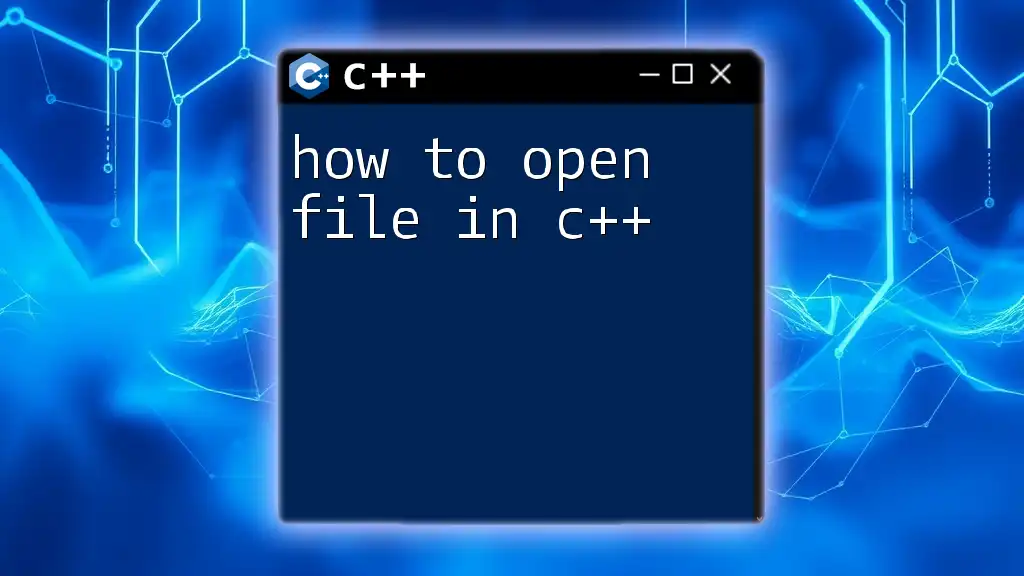
Advanced File Creation Techniques
Creating Binary Files in C++
Binary files store data in a format that is readable by computers but not necessarily by humans. Using binary files can offer efficiency, especially for larger data sets.
Here’s an example of how to write to a binary file:
#include <iostream>
#include <fstream>
struct Data {
int id;
float value;
};
int main() {
std::ofstream binFile("data.bin", std::ios::binary);
Data data = {1, 23.45f};
binFile.write(reinterpret_cast<char*>(&data), sizeof(data));
binFile.close();
return 0;
}
Explanation:
- Binary Stream: `std::ofstream binFile("data.bin", std::ios::binary);` opens a file for binary writing.
- Data Structure: We define a `struct` called `Data` to demonstrate how to handle complex types.
- Writing Data: The line `binFile.write(reinterpret_cast<char*>(&data), sizeof(data));` writes the binary representation of the `data` object to the file. The use of `reinterpret_cast<char*>` allows us to treat the `Data` structure as raw bytes.
Reading Files in C++
Reading data from a file is just as simple as writing it. Here’s a code example to read from a text file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inFile("example.txt");
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
Explanation:
- ifstream Object: We initialize `std::ifstream inFile("example.txt");` to open the file for reading.
- Reading Lines: The loop `while (std::getline(inFile, line))` reads the file line by line, allowing us to process the contents as needed.
- Output the Content: Each line is printed to the console using `std::cout`.
- Closing the File: Just like when writing, don’t forget to close the file after reading.
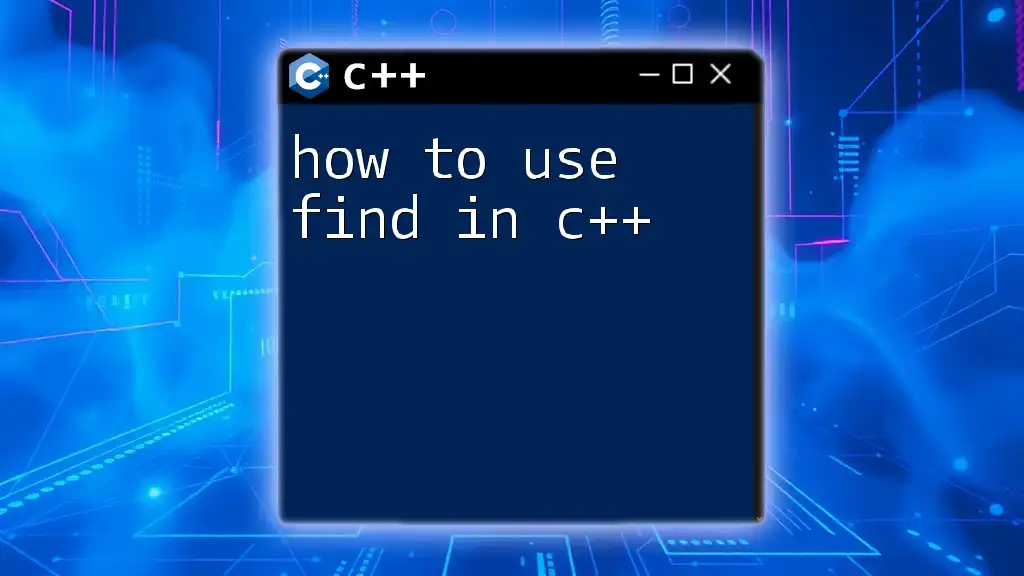
How to Make a Makefile for C++ Projects
What is a Makefile?
A Makefile simplifies project management by defining how to compile and link the code, making the build process more efficient and manageable. This file contains rules and dependencies for building different parts of your program.
Sample Makefile for Your C++ Project
Here's a simple Makefile to demonstrate how to build your C++ project:
CC=g++
CFLAGS=-c -Wall
SOURCES=main.cpp functions.cpp
OBJECTS=$(SOURCES:.cpp=.o)
EXECUTABLE=my_program
all: $(SOURCES) $(EXECUTABLE)
$(EXECUTABLE): $(OBJECTS)
$(CC) $(OBJECTS) -o $@
.cpp.o:
$(CC) $(CFLAGS) $< -o $@
Explanation:
- Variables: The `CC` variable specifies the compiler (`g++` in this case), and `CFLAGS` holds the compilation flags. The `SOURCES` variable lists the C++ source files.
- OBJECTS: `$(SOURCES:.cpp=.o)` automatically converts source file names to their corresponding object file names.
- Targets and Dependencies:
- The `all` target specifies that building the executable depends on its source files.
- The `$(EXECUTABLE)` target tells make how to create the executable by linking object files.
- The `.cpp.o:` rule specifies how to compile `.cpp` files into `.o` object files.
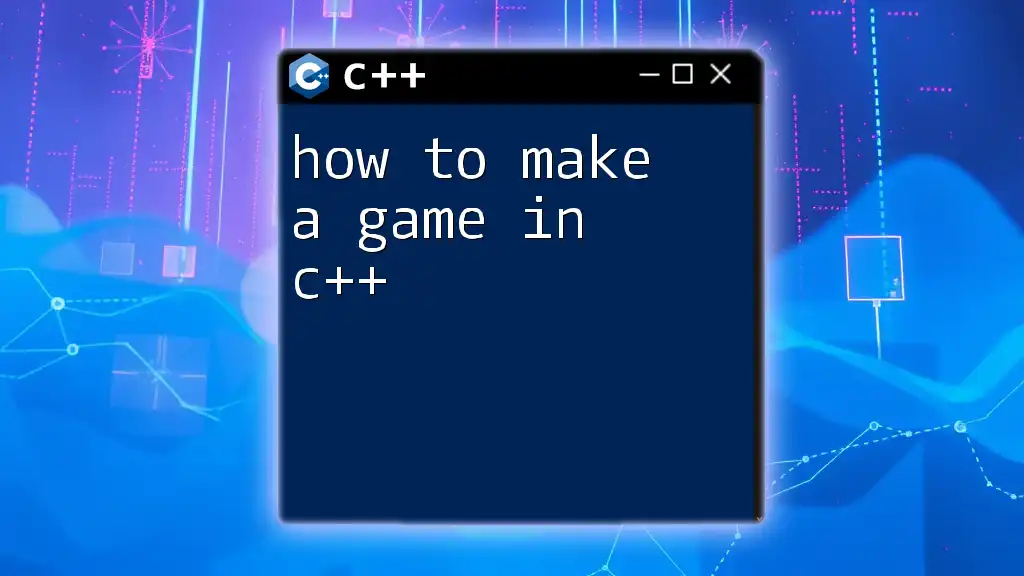
Best Practices in C++ File Handling
Tips for Efficient File Management
- Successful File Opening: Always check whether the file opened successfully by using conditional statements.
- Use Exceptions: For larger applications, consider using exceptions for better error handling.
- Close Files: Always close files explicitly to free up system resources.
Avoiding Common Pitfalls
- Overwriting Files: Be cautious when creating files to avoid inadvertently overwriting existing ones.
- Text vs. Binary: Understand the implications of working with binary files versus text files, as the methods of handling them differ substantially.
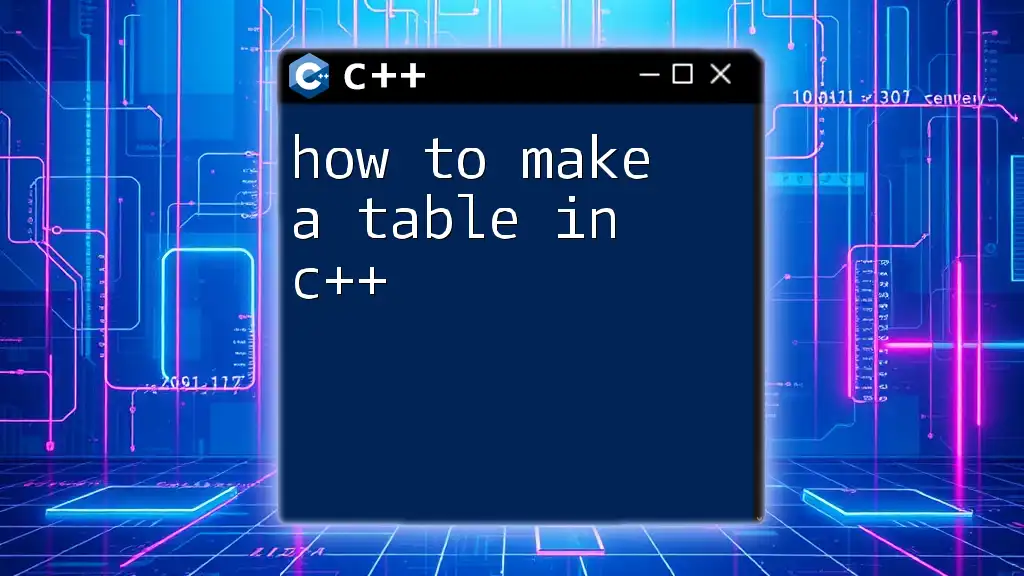
Conclusion
You’ve now learned how to make a file in C++ through straightforward examples and best practices. File creation and management are foundational skills in programming, enhancing your ability to handle data efficiently. As you continue to practice these concepts, you’ll be better equipped to integrate file operations into your projects confidently. Happy coding!