In C++, a switch statement is used to execute one block of code among multiple choices based on the value of a variable or expression.
Here's a simple example:
#include <iostream>
using namespace std;
int main() {
int day = 3;
switch (day) {
case 1: cout << "Monday"; break;
case 2: cout << "Tuesday"; break;
case 3: cout << "Wednesday"; break;
default: cout << "Invalid day";
}
return 0;
}
What is a Switch Statement in C++?
A switch statement in C++ is a control structure that allows you to execute a block of code based on the value of a variable. It is an alternative to using multiple if-else statements, offering a more readable and organized way to handle multiple conditions.
Understanding the Syntax of Switch Statements
The basic syntax of the switch statement includes several key components:
switch(expression) {
case constant1:
// code block
break;
case constant2:
// code block
break;
default:
// default code block
}
In this syntax:
- Expression is evaluated once and compared with the values of each case.
- Case labels are constant expressions that determine which block of code to execute.
- The break statement prevents the code from falling through to the next case.
- The default case executes if none of the specified cases match.
C++ Case Statement Syntax
The c++ case statement syntax is crucial for understanding how to use switch statements effectively. Each case must be a unique constant value or expression. If the expression evaluates to a case value, the associated code block executes until a break statement is encountered or the switch statement ends.
For example:
switch (color) {
case 'R':
cout << "Red";
break;
case 'G':
cout << "Green";
break;
case 'B':
cout << "Blue";
break;
default:
cout << "Unknown Color";
}
In this snippet, the program will output "Red" if the color variable is set to 'R'.

How to Use a Switch in C++
When to Use a Switch
Switch statements are particularly useful when you have a single variable with numerous potential values to evaluate. They make code easier to read and manage. A switch is often preferable when the number of cases is known and fixed, allowing for clear structured decision-making.
Step-by-Step Example of Switch Statement in C++
Here’s a simple example demonstrating a switch statement:
#include <iostream>
using namespace std;
int main() {
int day = 3;
switch(day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
case 3:
cout << "Wednesday";
break;
default:
cout << "Invalid day";
}
return 0;
}
In this example:
- The expression is day, and it evaluates the value of the variable.
- If day equals 3, it matches the case and outputs "Wednesday."
- The use of break ensures that the program exits the switch block after executing the matching case.
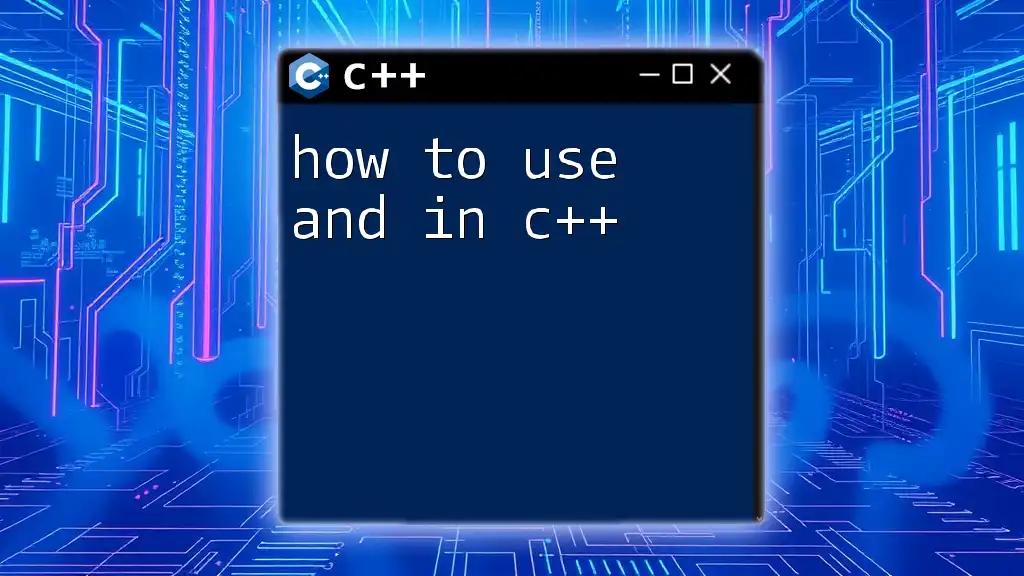
Switch Case in C++ Example
Example with Multiple Cases
Switch statements can handle multiple potential cases, as shown in the following example:
#include <iostream>
using namespace std;
int main() {
int day = 5;
switch(day) {
case 1: // Fall through
case 2:
case 3:
cout << "Weekday";
break;
case 4:
case 5:
cout << "Weekend";
break;
default:
cout << "Invalid day";
}
return 0;
}
In this code snippet:
- Days 1 through 3 classify as "Weekday," and case 4 and 5 classify as "Weekend."
- Notice how there isn’t a break statement between the first three cases. This allows for fall-through, meaning if the day is 1, 2, or 3, it outputs "Weekday."
Understanding Fall-Through Behavior
The absence of a break statement can be beneficial, but it can also lead to unintentional behavior if not handled carefully. Developers should know when to use and avoid fall-through to prevent bugs.
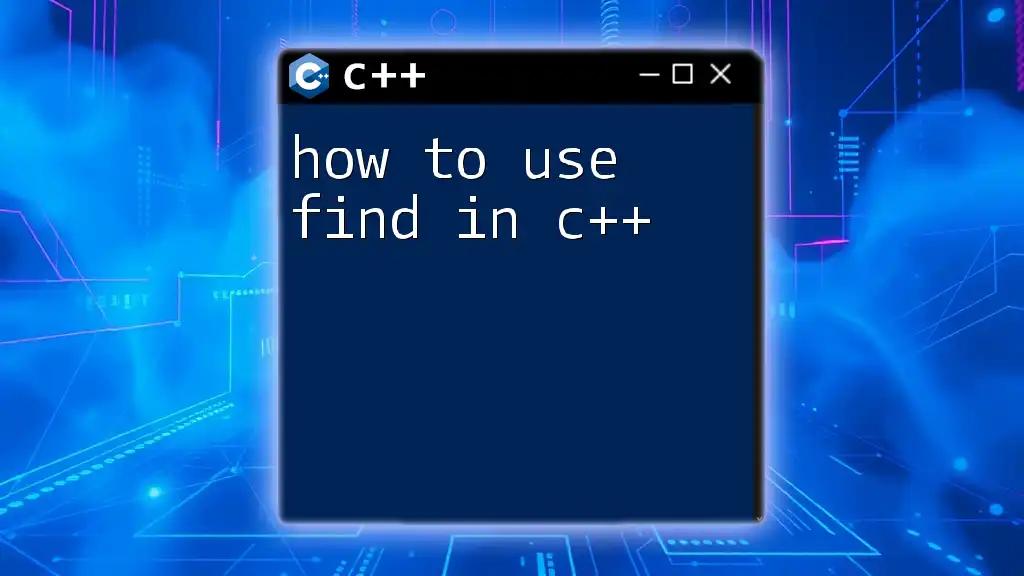
Switch Function in C++
Creating a Function That Uses a Switch Statement
A switch statement can also be encapsulated in a function for clarity and reusability. Here’s an example:
#include <iostream>
using namespace std;
void printDay(int day) {
switch(day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
case 3:
cout << "Wednesday";
break;
case 4:
cout << "Thursday";
break;
case 5:
cout << "Friday";
break;
case 6:
cout << "Saturday";
break;
case 7:
cout << "Sunday";
break;
default:
cout << "Invalid day";
}
}
int main() {
printDay(2);
return 0;
}
In this function, the printDay function modularizes the switch statement, improving code organization and reusability. Whenever this function is called with a valid day, it outputs the corresponding weekday.
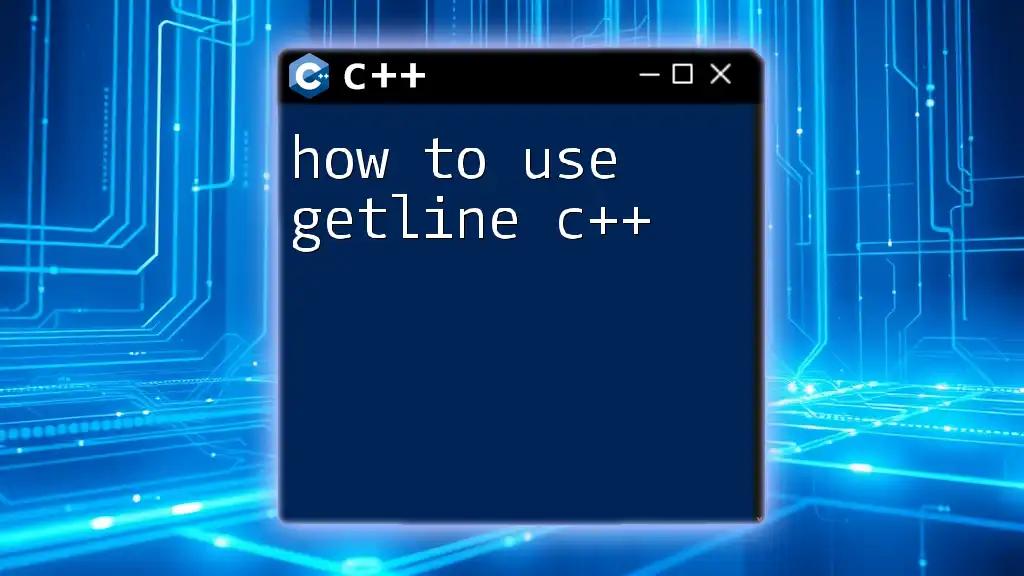
Advanced Concepts in Switch Statements
Nested Switch Statements
You can also create nested switch statements for more complex control flow. Consider the following example:
switch (outerVariable) {
case 1:
switch (innerVariable) {
case 1:
cout << "Outer 1, Inner 1";
break;
case 2:
cout << "Outer 1, Inner 2";
break;
}
break;
case 2:
cout << "Outer 2";
break;
}
In this structure, the outer switch evaluates outerVariable, and a nested switch evaluates innerVariable. This structure allows handling complex scenarios effectively.
Limitations of Switch Statements
Despite their utility, switch statements come with limitations. They can only evaluate integral types (char, int, etc.) and cannot accept conditions or ranges. Be mindful when choosing between switch and other control structures.

Conclusion
In summary, understanding how to use a switch in C++ is essential for creating clean and efficient code. The switch statement provides a clear and manageable way to control the flow of your program based on variable values. With practice and experimentation, you can master this important aspect of C++ programming, enhancing your ability to write organized and logical code.

Additional Resources
- Consult the [C++ documentation](https://en.cppreference.com/w/) for in-depth reference.
- Engage in hands-on practice with projects that implement switch statements to solidify your understanding and skills.