In C++, you can round up a floating-point number to the nearest integer using the `ceil` function from the `<cmath>` header.
Here’s a code snippet demonstrating this:
#include <iostream>
#include <cmath>
int main() {
double number = 3.14;
double roundedUp = ceil(number);
std::cout << "Rounded up value: " << roundedUp << std::endl; // Output: 4
return 0;
}
Understanding Rounding Principles in C++
Rounding is an important concept in programming that helps manage numerical data effectively. In C++, rounding plays a crucial role in ensuring that numbers are handled accurately, whether for calculations, data processing, or output formatting. Understanding the differences between rounding down, rounding up, and rounding towards zero can significantly impact your code's behavior and outcomes.
Mathematical functions relevant to rounding in C++ provide the tools you need to manage these operations effectively. In this article, we will focus specifically on how to round up in C++, exploring built-in functions and custom solutions.
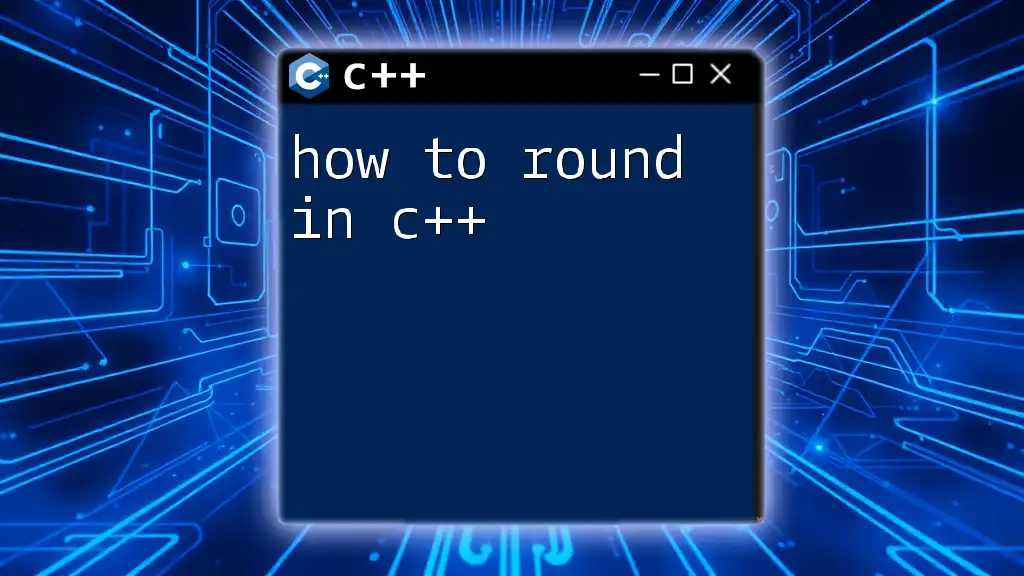
The Concept of Rounding Up
Rounding up refers to adjusting a number to the nearest whole number that is greater than or equal to it. For instance, rounding up 5.1 results in 6, while rounding up -1.7 results in -1. This concept is widely applicable in various fields, such as finance, statistics, and data analysis. When you round up, it assures that your results reflect a conservative estimate, crucial when dealing with quantities, prices, or statistical data that can't be fractional in certain contexts.
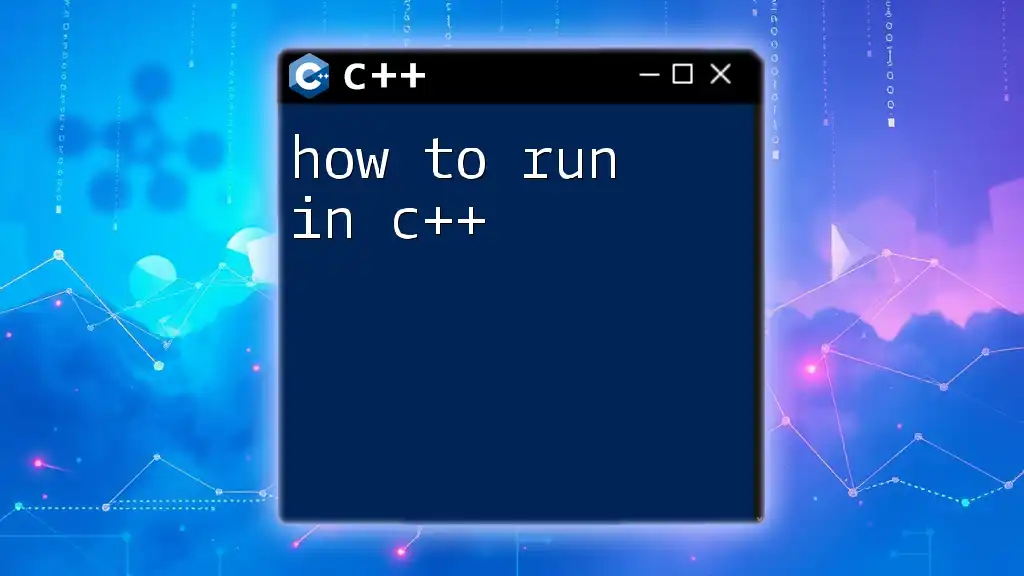
Rounding Up in C++ with Built-in Functions
Using the `ceil()` Function
The `ceil()` function in C++ is specifically designed to round up numbers. It takes a floating-point value as an argument and returns the smallest integral value that is greater than or equal to that value.
Syntax of `ceil()`:
double ceil(double x);
Code Snippet Example Using `ceil()`:
#include <iostream>
#include <cmath>
int main() {
double num = 5.1;
double roundedUp = ceil(num);
std::cout << "Rounded Up: " << roundedUp << std::endl; // Outputs: 6
return 0;
}
In the example above, the `ceil()` function successfully rounds up 5.1 to 6.
Key Points to Remember:
- The `ceil()` function returns a `double` type.
- It effectively handles both positive and negative floating-point numbers, rounding towards positive infinity.
Using the `round()` Function
While `round()` is not designed specifically for rounding up, it can be handy in certain scenarios. This function rounds to the nearest integer, resorting to `ceil()` when the decimal part is exactly 0.5 or more for positive numbers.
Syntax of `round()`:
double round(double x);
Code Snippet Example:
#include <iostream>
#include <cmath>
int main() {
double num = 4.6;
double roundedValue = round(num); // Rounds to nearest
std::cout << "Rounded Value: " << roundedValue << std::endl; // Outputs: 5
return 0;
}
Here, `round()` rounds 4.6 to 5.
Special Cases:
- When utilizing `round()`, it's important to understand that when the number is exactly halfway between two integers (e.g., 5.5), it will round to the nearest even number.
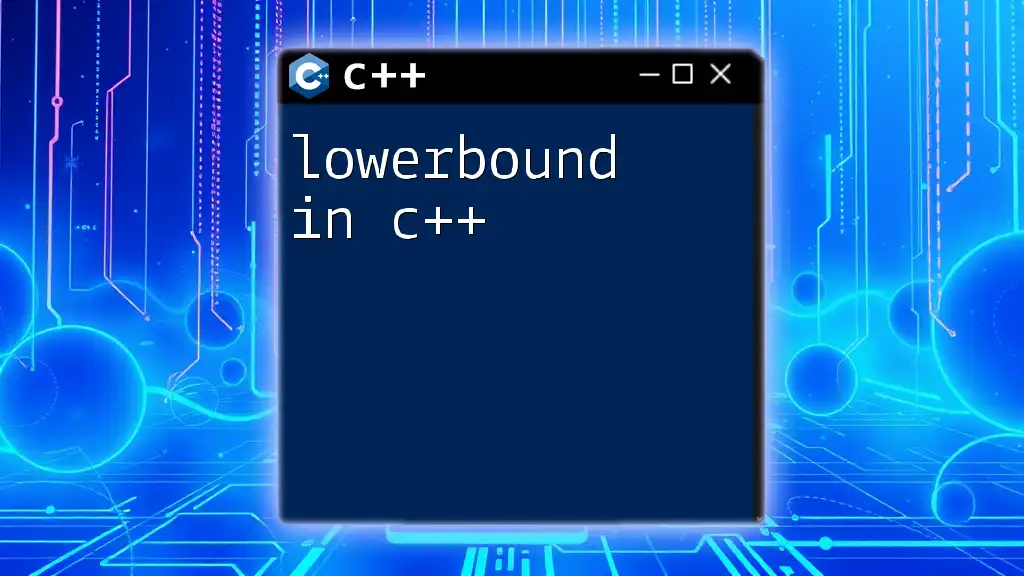
Implementing Custom Round-Up Functions
Creating Your Own Round-Up Function
There might be scenarios where built-in functions don't fulfill specific needs. In such cases, you can create your custom round-up function. This custom function can offer additional flexibility and control over how you manage rounding throughout your application.
Sample Code for Custom Round Up:
#include <iostream>
int roundUp(double num) {
return (int(num) + (num > int(num) ? 1 : 0));
}
int main() {
double value = 7.3;
std::cout << "Custom Rounded Up: " << roundUp(value) << std::endl; // Outputs: 8
return 0;
}
Here, the custom function `roundUp` checks if the decimal part of the number is greater than zero. If it is, it adds 1 to the integer part of the number, effectively rounding it up.
Testing and Validation: Testing your custom function ensures it meets specific requirements. Be sure to examine various inputs, including negative numbers and edge cases.
Handling Edge Cases
It’s vital to appropriately handle edge cases when creating custom round-up functions.
- Negative Numbers: Ensure your function rounds correctly. For example, -2.1 should yield -2, while -2.5 should yield -2.
- Very Small Numbers: For numbers close to zero, like 0.0001, rounding up should yield 1.
- Precision Issues: Be cautious of floating-point precision issues, which can lead to unexpected behavior. Make sure to consider using appropriate data types to maintain precision during computations.
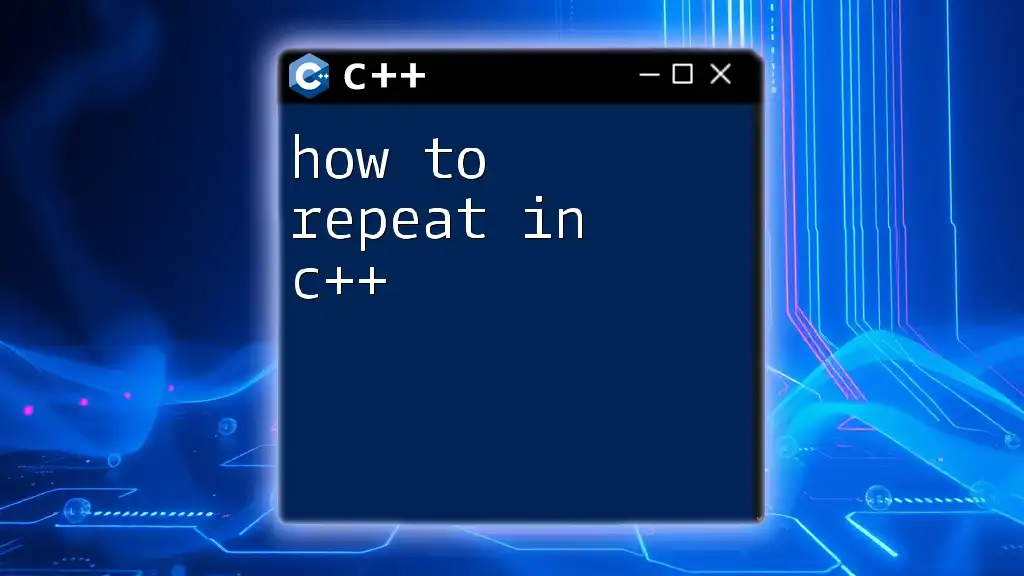
Practical Applications of Rounding Up in C++
Financial Calculations
Rounding up is particularly critical in financial contexts, such as transactions or pricing strategies. For example, when calculating tax or determining total charges, it is often necessary to round up to ensure that the customer pays an appropriate amount. Here's a simple example:
#include <iostream>
#include <cmath>
double calculateTotal(double price, double tax) {
double total = price + (price * tax);
return ceil(total); // Use ceil to ensure we round up
}
int main() {
std::cout << "Total Price: " << calculateTotal(19.99, 0.07) << std::endl; // Outputs: 22
return 0;
}
User Input and Data Validation
When dealing with user inputs, rounding can help validate and correct values. If users input fractional values when whole numbers are expected, using `ceil()` can ensure the value is correctly adjusted to meet requirements.
Code Snippet for Validating and Rounding User Inputs:
#include <iostream>
#include <cmath>
int main() {
double userInput;
std::cout << "Enter a number: ";
std::cin >> userInput;
double roundedUp = ceil(userInput);
std::cout << "Rounded Up Input: " << roundedUp << std::endl;
return 0;
}
In this example, if the user enters a number like 3.2, the program will round it up to 4, ensuring that it fits the expected criteria for further processing.
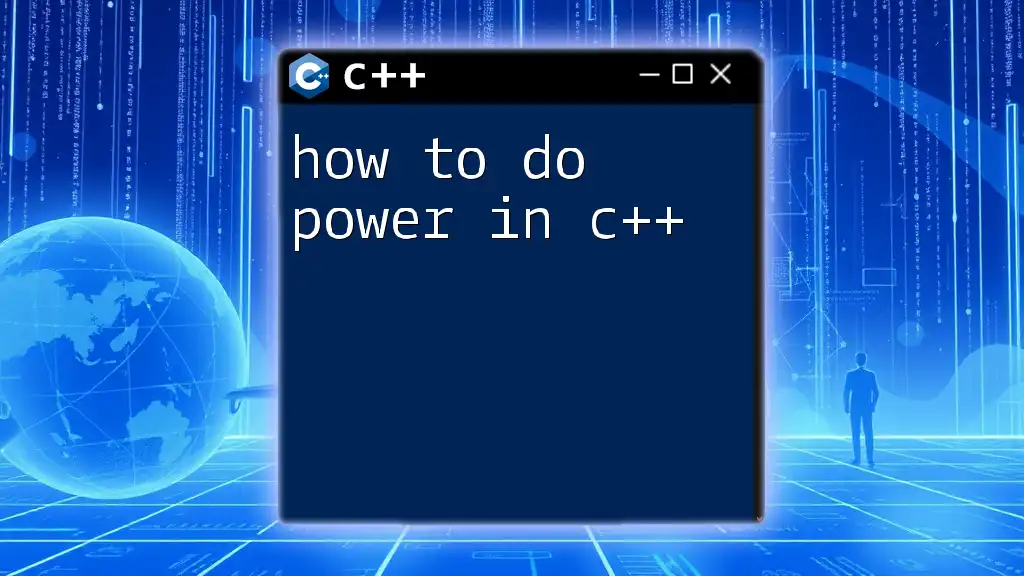
Common Mistakes to Avoid
When rounding up in C++, it's crucial to avoid common pitfalls:
- Misuse of `ceil()`: Remember that `ceil()` will always round towards positive infinity, even for negative numbers.
- Forgetting to Include `<cmath>`: The `ceil()` and `round()` functions are housed within the `<cmath>` library, and forgetting to include this header will result in compilation errors.
- Confusing Rounding Functions: It's important to differentiate between `ceil()`, `floor()`, and `round()` to ensure you employ the correct function for your specific needs.
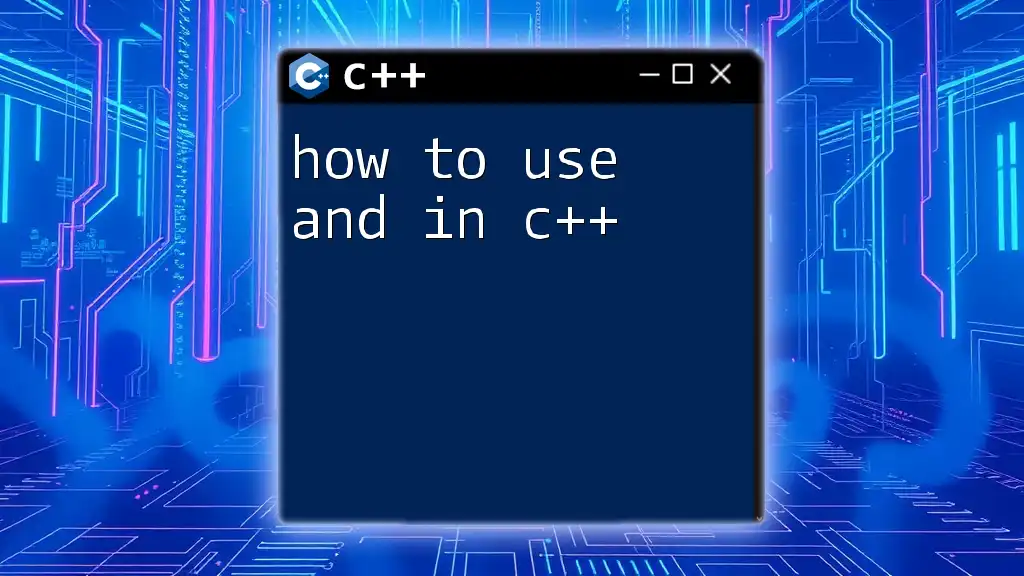
Conclusion
Rounding up is a fundamental operation in C++ that has significant implications across various applications. Understanding how to round up using built-in functions like `ceil()` and `round()`, alongside the ability to create custom rounding solutions, empowers developers to manage data effectively. As you delve deeper into C++, practice these principles through real-world projects and challenges, enhancing your programming expertise.
By continually refining your understanding of rounding techniques, you will pave the way for more robust and efficient code in your C++ endeavors.