In C++, you can create tabs in your output by using the escape sequence `\t`, which inserts a horizontal tab character in your string.
Here's an example:
#include <iostream>
int main() {
std::cout << "Name:\tJohn Doe\nAge:\t30\n";
return 0;
}
Understanding Tabs in C++
What are Tabs?
Tabs are special characters used in coding to create horizontal space in the text. They help in aligning code elements and improving readability. In programming, especially in languages like C++, proper use of tabs can significantly contribute to the organization of code, making it easier for developers to interpret and modify.
Whitespace is crucial in programming as it defines structure and hierarchy. Without proper indentation, your code can become confusing, making it challenging for others to follow the program's logic.
The Role of Tabs in C++
In C++, tabs serve primarily as a method of indentation to visually separate code blocks, such as functions, control structures, and classes.
Using tabs versus spaces often incites debate among developers. Each has its advocates, but the most significant difference hinges on consistency and collaboration. Here’s a simple illustration of the difference between using tabs and spaces:
#include <iostream>
int main() {
std::cout << "Using Tabs\n"; // Using a tab character
std::cout << "Using\nSpaces\n"; // Using spaces for indentation
return 0;
}
The different spacing formats can lead to code that looks inconsistent. Thus, understanding how to properly use tabs helps maintain code readability.
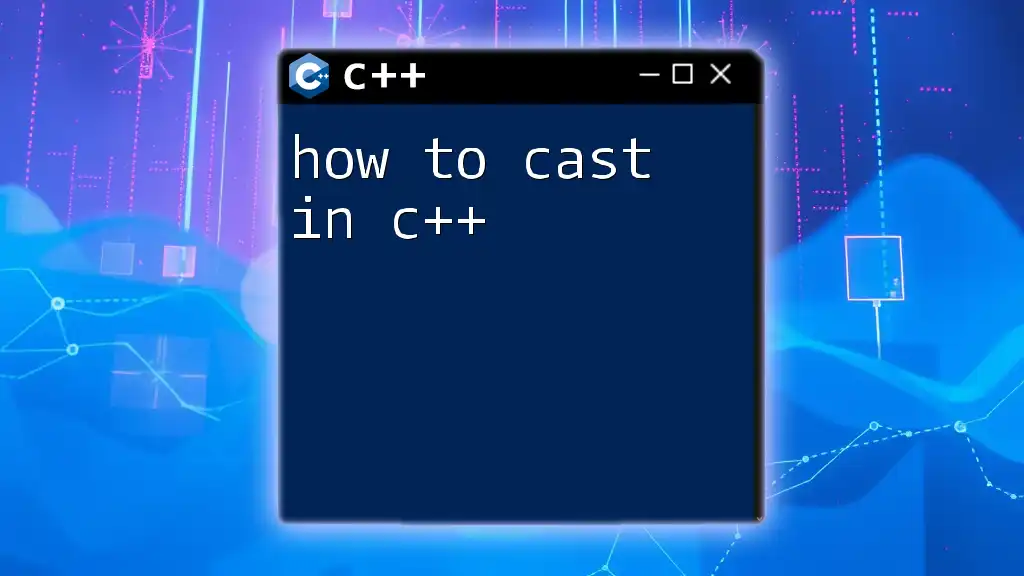
Configuring Tabs in Your Development Environment
Setting Up Tabs in Popular Text Editors
Each text editor offers distinct ways to configure tab settings according to personal preferences and project standards.
Visual Studio Code:
- Go to `Settings`.
- Type "Tab Size" in the search bar.
- Choose between `2`, `4`, or `8` spaces per tab and toggle between using tabs or spaces.
Code::Blocks:
- Navigate to `Settings > Editor > Code style`.
- Here you'll find options to set the tab width and determine if tabs should be used.
Eclipse:
- Locate `Preferences > C/C++ > Code Style > Formatter`.
- Customize tab width according to your coding style.
Customizing the Look of Tabs
Setting the right tab size (commonly 4 spaces) enhances readability without compromising the compactness of the code. Consistent usage across your projects prevents confusion, especially in teams where differing tab settings can introduce hidden errors and visual inconsistencies.
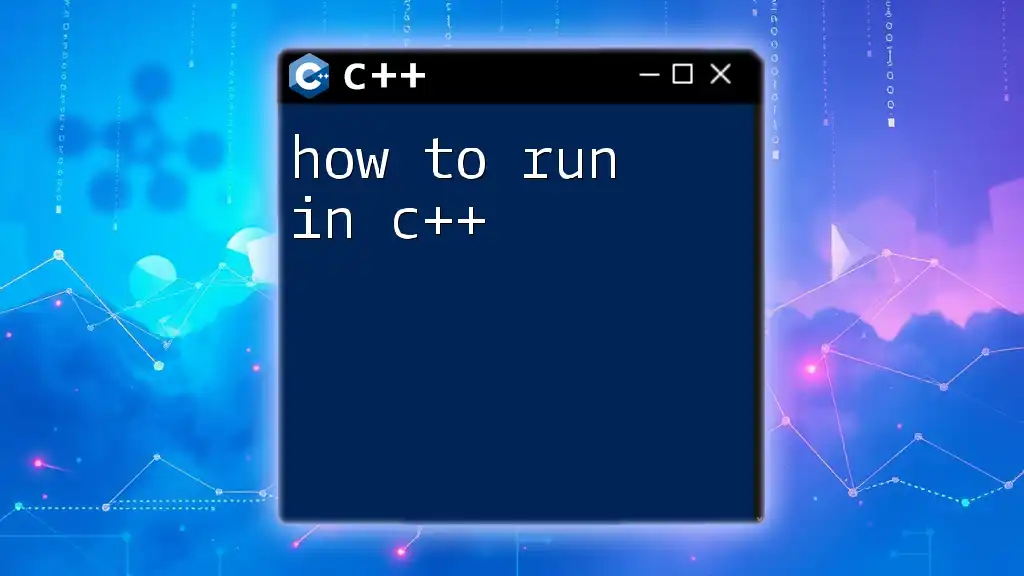
Using Tabs in C++ Code
Basic Syntax and Implementation
Creating Tab-Indents in Output
Tabs can be included in output streams with the escape character `\t`. This ensures neatly formatted output and better alignment across multiple output lines.
Here’s a practical example of how to use tab characters in output:
#include <iostream>
int main() {
std::cout << "Column1\tColumn2\n"; // Using tabs in output
std::cout << "Data1\tData2\n";
return 0;
}
In the above example, the output will show two columns aligned under their respective headers, thanks to the use of the tab character.
Handling Tabs in Input
When processing input, especially from users or files, tabs can have a significant impact. It’s essential to consider how tabs interact with parsed values.
For example:
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter data (tab-separated): ";
std::getline(std::cin, line);
// Example: Process input string using tabs
std::cout << "You entered: " << line << std::endl;
return 0;
}
This code snippet demonstrates how to read an entire line of data, potentially containing tab characters, which can be useful when dealing with structured data inputs.
Tab Expansion vs. Tab Compression
The difference between tab expansion and tab compression revolves around how tab characters are displayed versus how they are interpreted. Some editors expand tabs to a fixed number of spaces for consistency, while others may treat tabs as distinct characters based on user settings. Understanding this can avoid formatting issues when sharing code.
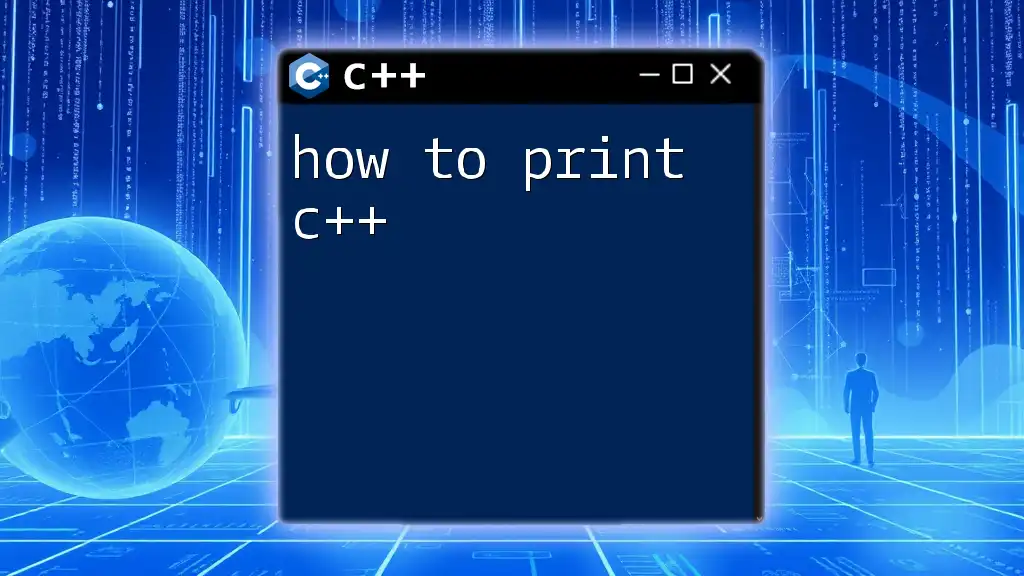
Best Practices for Using Tabs in C++
The Tab vs. Space Debate
The ongoing discussion about whether to use tabs or spaces revolves around maintainability and adherence to team coding standards. One side argues that tabs allow for user preference in personal display settings, while the other side insists that spaces ensure uniform presentation across all environments.
Consistency is Key
Regardless of the choice made between tabs and spaces, consistency is crucial. Maintaining a uniform style throughout your codebase fosters better collaboration and reduces misunderstandings. To enforce consistency, consider using tools like linters or formatters, which help validate code standards automatically.
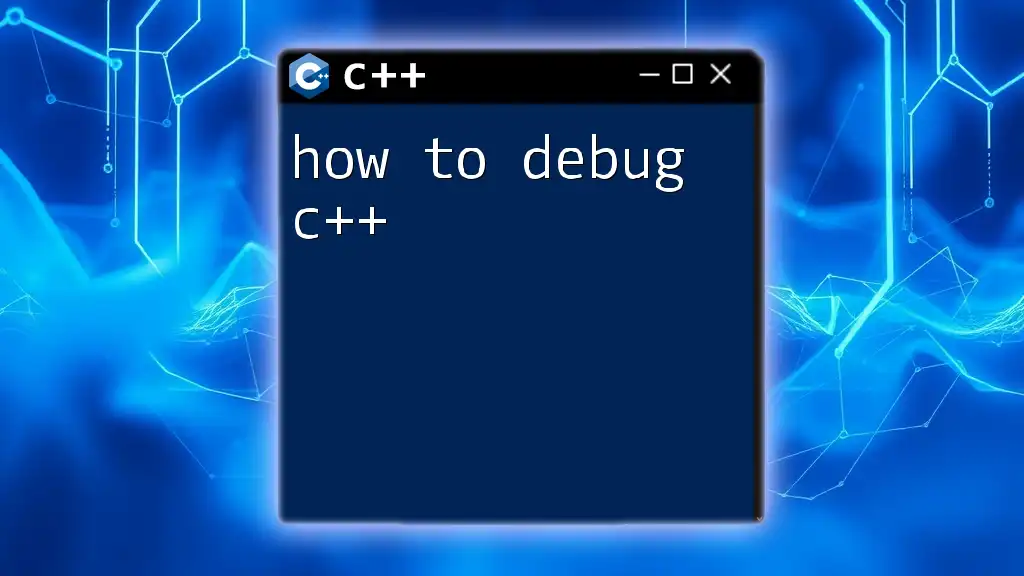
Advanced Tab Usage in C++
Custom Tab Handling
Creating reusable functions for handling tab characters can enhance your code's maintainability. It allows you to abstract formatting logic away from your main codebase. Here’s how you can format output with tabs through a simple function:
#include <iostream>
void printWithTabs(const std::string& data1, const std::string& data2) {
std::cout << data1 << "\t" << data2 << std::endl;
}
int main() {
printWithTabs("Name", "Age");
printWithTabs("John", "25");
return 0;
}
This function encapsulates the tabbed output, which you can use throughout your program to keep your formatting consistent and clear.
Using Tabs in File I/O
Tab-separated values (TSV) are an essential format for file input and output. You can effectively read from and write to files that contain tab-separated data. Here’s an example illustrating reading from such a file:
#include <fstream>
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> readTabSeparatedFile(const std::string& filename) {
std::ifstream file(filename);
std::string line;
std::vector<std::string> data;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string item;
while (std::getline(ss, item, '\t')) {
data.push_back(item);
}
}
return data;
}
In this code, the `readTabSeparatedFile` function reads from a file, separating values based on tabs, and stores them in a vector. Understanding how to work with tab-separated values can significantly impact data handling strategies in applications where structured data input/output is required.
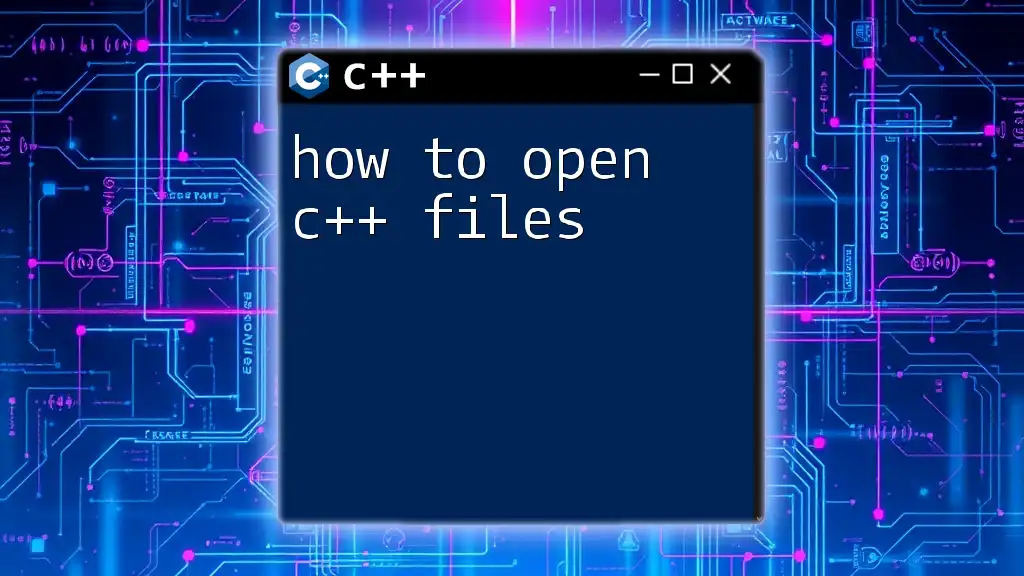
Conclusion
Recap of Key Points
In summary, understanding how to tab in C++ is fundamental for enhancing code readability and ensuring effective collaboration among developers. The format and consistency of your tabs directly influence the quality of your programming.
Further Resources
Explore online documentation to deepen your understanding of coding standards regarding whitespace. Embrace various resources for enhancing your practices in using tabs effectively in C++.
Encouragement to Practice
Challenge yourself to incorporate tabs into your own projects, experimenting with output formatting and data handling strategies. By applying these concepts, you'll not only polish your coding skills but also improve your overall programming practices.