To create a vector in C++, you can use the `std::vector` class from the Standard Template Library (STL), which provides a dynamic array functionality.
#include <vector>
std::vector<int> myVector; // Creates an empty vector of integers
Understanding Vectors in C++
A vector in C++ is a part of the Standard Template Library (STL) that provides a dynamic array, which can grow and shrink in size. Unlike traditional arrays, vectors allow you to manage memory more efficiently, accommodating varying amounts of data. They offer numerous advantages, such as automatic resizing, ease of element access, and built-in functions for manipulation. Vectors are particularly useful when the number of elements is not known in advance, enabling greater flexibility in managing collections of items.
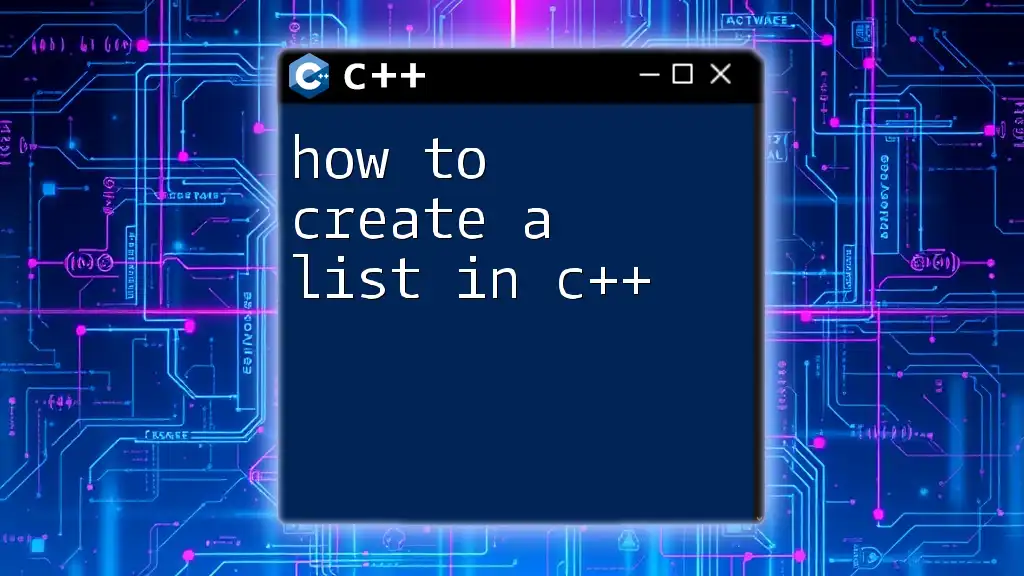
How to Create a Vector in C++
Vector Declaration in C++
To start working with vectors, you must first declare one. The declaration process defines the type of elements the vector will hold. Here is how a vector is declared in C++:
#include <vector>
std::vector<int> myVector; // Declaring an empty vector of integers
How to Declare a Vector in C++
Using the vector Header File
Before you can declare a vector, it is essential to include the `<vector>` header file, which provides the necessary functionalities to use vectors:
#include <vector> // Important for using vectors
Different Data Types for Vector Declaration
Vectors can hold any data type, including primitive types and user-defined types. Below are examples of declaring vectors with various data types:
std::vector<float> floatVector; // Vector of floats
std::vector<std::string> stringVector; // Vector of strings
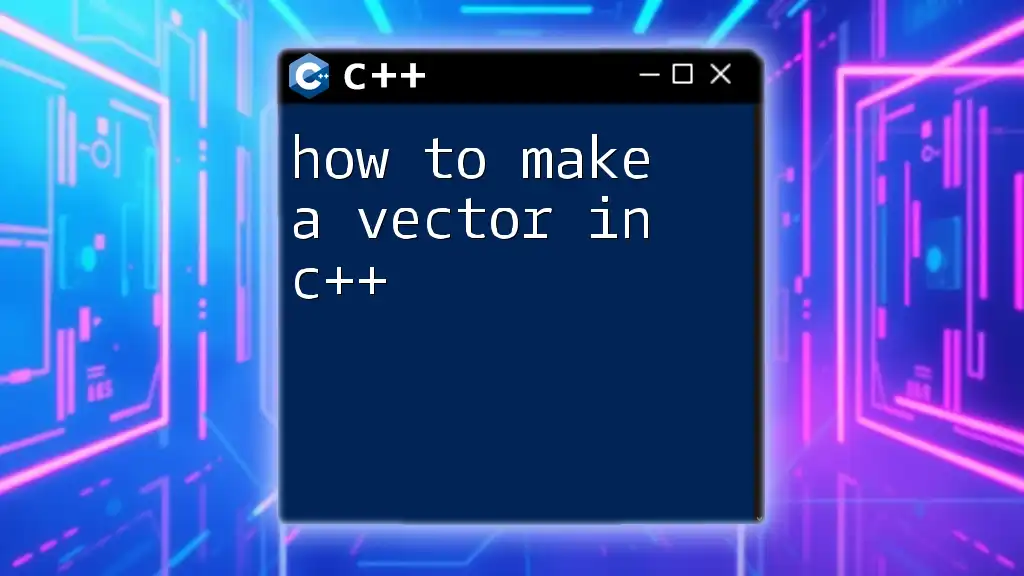
Creating a Vector with Initial Values
Initializing a Vector
To create a vector with specified initial values, you can use the constructor that allows you to define both the size and the default value for each element. Here’s an example:
std::vector<int> initVector(5, 10); // Vector of size 5, initialized with 10s
In this example, `initVector` is created with five elements, each assigned an initial value of `10`.
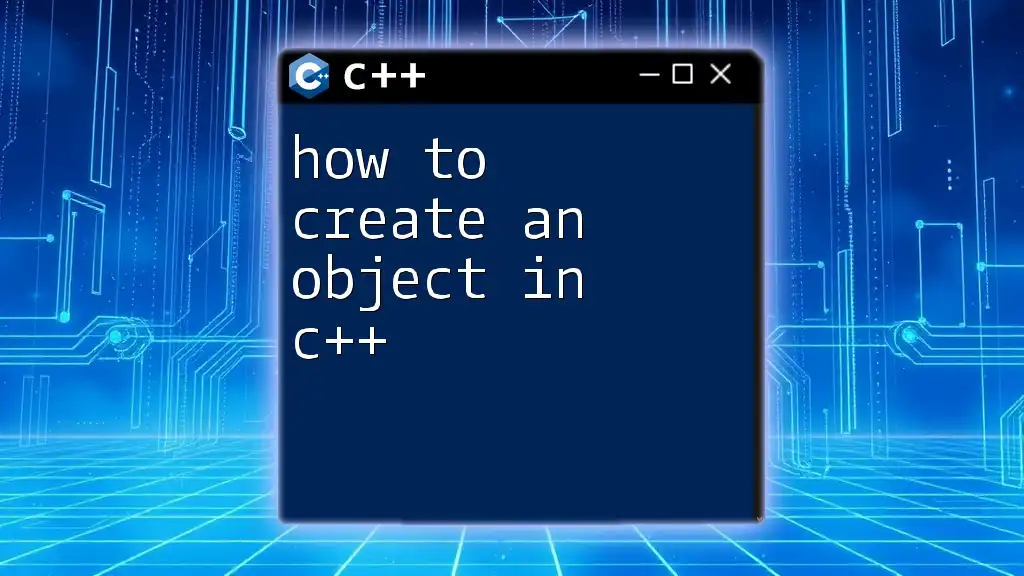
Adding Elements to a Vector
Using push_back()
One of the most common ways to add elements to a vector is through the `push_back()` method, which appends a new element at the end of the vector. Here’s how it works:
myVector.push_back(1);
myVector.push_back(2);
In this case, the integers `1` and `2` are dynamically added to `myVector`.
Insert Elements
If you need to insert elements at specific positions, the `insert()` function is your go-to method. Here’s an example:
myVector.insert(myVector.begin() + 1, 3); // Inserts 3 at index 1
In the example above, the number `3` is inserted at the second position of `myVector`, pushing other elements to the right.
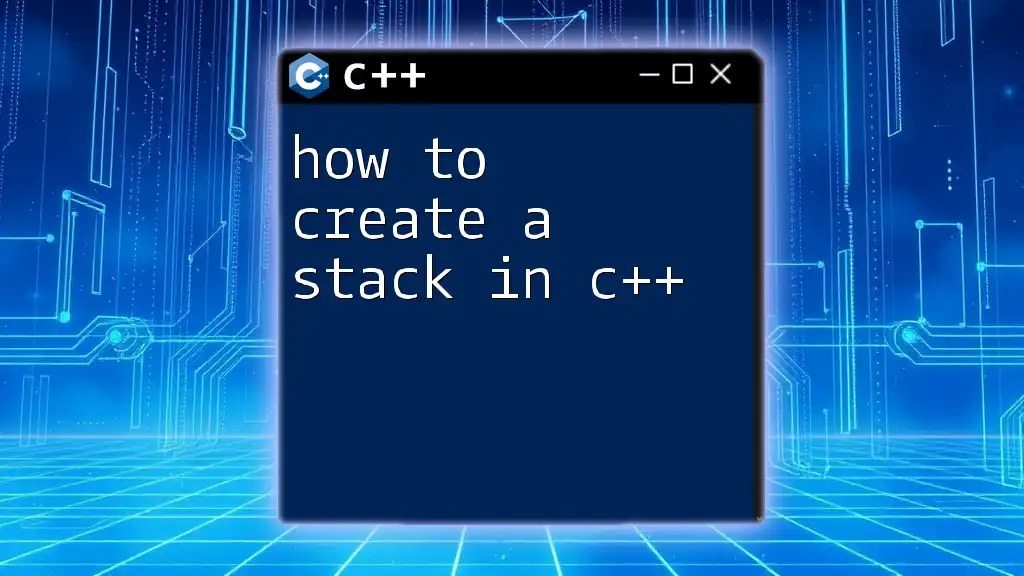
Accessing Vector Elements
Using Indexing
Accessing elements within a vector can be accomplished easily through indexing, similar to arrays. Here's how you can access the first element:
std::cout << "Element at index 0: " << myVector[0] << std::endl;
Using Iterators
For more flexibility, especially when needing to traverse all elements, you can utilize iterators. Here’s a sample of how to iterate over a vector:
for (auto it = myVector.begin(); it != myVector.end(); ++it) {
std::cout << *it << " ";
}
Using iterators allows you to access each element without needing to know the size of the vector in advance.
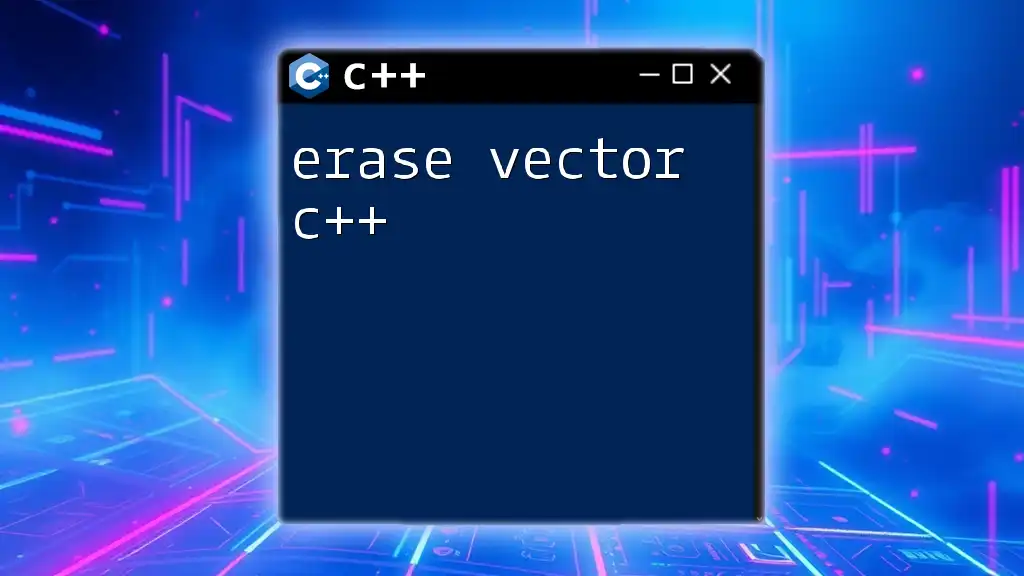
Resizing and Reserving Vectors
Resizing a Vector
As your data changes, you may need to resize your vector. The `resize()` function is quite handy for this:
myVector.resize(10); // Resizes the vector to hold 10 elements
In this situation, if the vector initially contained fewer than ten items, default-initialized values would fill the new slots.
Reserving Space
To optimize performance and reduce unnecessary reallocations as new elements are added, you can use the `reserve()` function. This preallocates space without changing the size of the vector:
myVector.reserve(20); // Reserves space for 20 elements
This method can significantly improve the performance of your code when you know in advance the number of elements to be inserted.
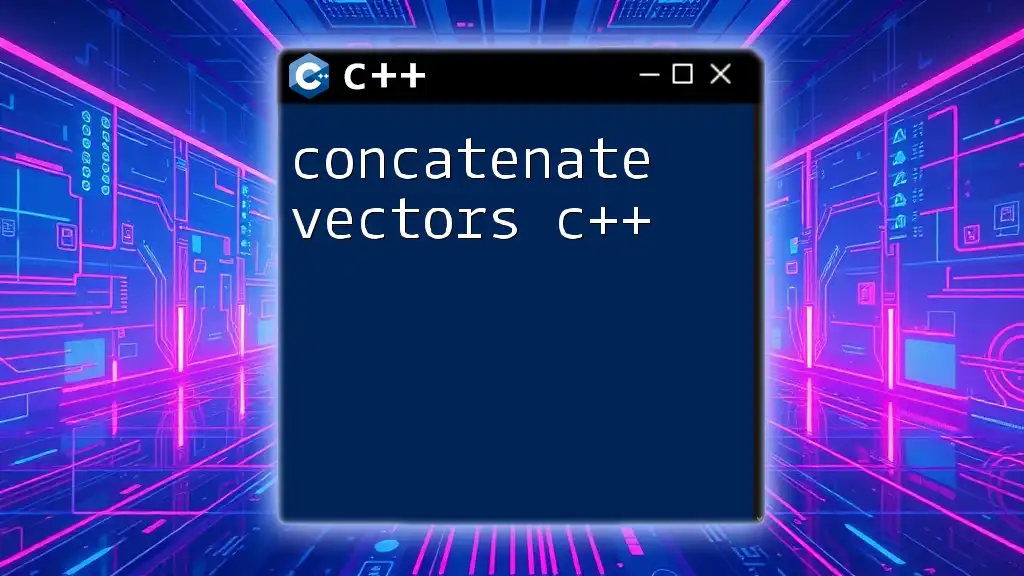
Common Practices and Tips
When working with vectors in C++, it’s crucial to follow certain best practices for optimal performance and reliability:
- Memory Management: Keep in mind that vectors manage memory dynamically, which can lead to overhead. Use `reserve()` if you anticipate many insertions.
- Avoiding Out-of-Bounds Access: Be cautious while accessing elements. Accessing indices beyond the vector's current size leads to undefined behavior. Always check the size beforehand.
Best Practices for Using Vectors
- Favor vectors over arrays when dealing with collections of data whose size may change.
- Leverage built-in functions like `clear()`, `swap()`, and `emplace_back()` for managing data efficiently.
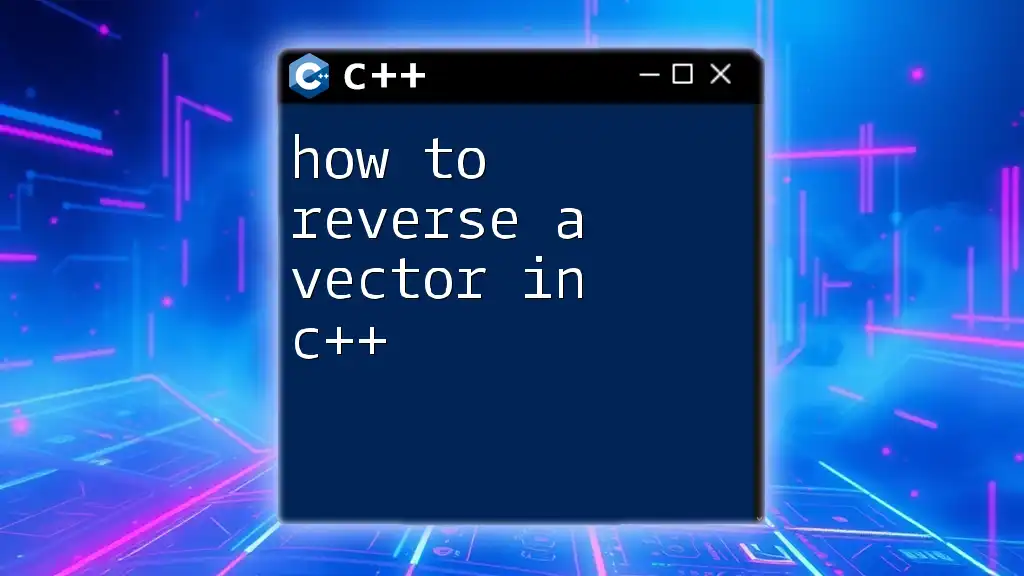
Conclusion
In summary, understanding how to create a vector in C++ is fundamental for effective programming. Vectors provide significant flexibility and functionalities that static arrays cannot offer. By mastering vector declarations, initializations, and proper data management techniques, you will elevate your C++ programming ability and harness the full power of the Standard Template Library.
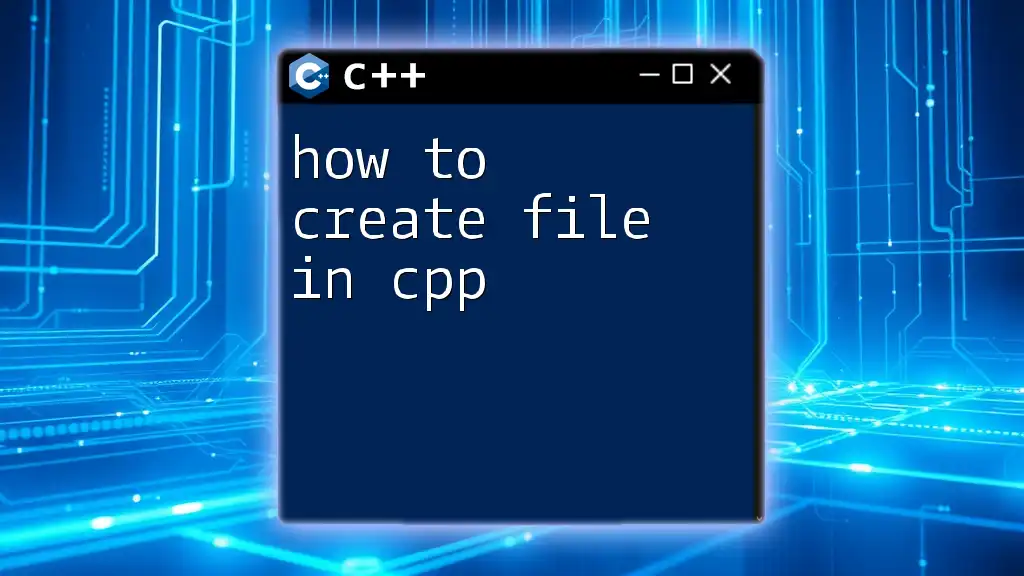
Call to Action
We encourage you to put these concepts into practice by creating vectors with various data types. Share your experiences and discoveries in working with vectors, and stay tuned for more insights and tips on improving your C++ programming skills!