To concatenate two vectors in C++, you can use the `insert` method to append the elements of one vector to another. Here's a code snippet to illustrate this:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
vec1.insert(vec1.end(), vec2.begin(), vec2.end());
for (int num : vec1) {
std::cout << num << " ";
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can hold a variable number of elements. Unlike traditional arrays, which have a fixed size, vectors can resize themselves automatically when new elements are added or removed. This flexibility makes vectors a powerful and convenient tool for developers, particularly when dealing with collections of data that may grow or shrink over time.
Here’s a simple example of how to declare and initialize a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
Basic Operations on Vectors
Vectors support a variety of operations that facilitate the manipulation of their elements:
-
Adding elements: You can use the `push_back()` function to add new elements to the end of a vector. For example:
numbers.push_back(6);
-
Accessing elements: You can access individual elements in a vector using the `[]` operator. For instance:
int firstElement = numbers[0]; // Accesses the first element (1)
Here's a code snippet demonstrating basic operations:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(1);
numbers.push_back(2);
numbers.push_back(3);
for (int i = 0; i < numbers.size(); ++i) {
std::cout << numbers[i] << " ";
}
return 0;
}
When executed, this code will output: `1 2 3`.
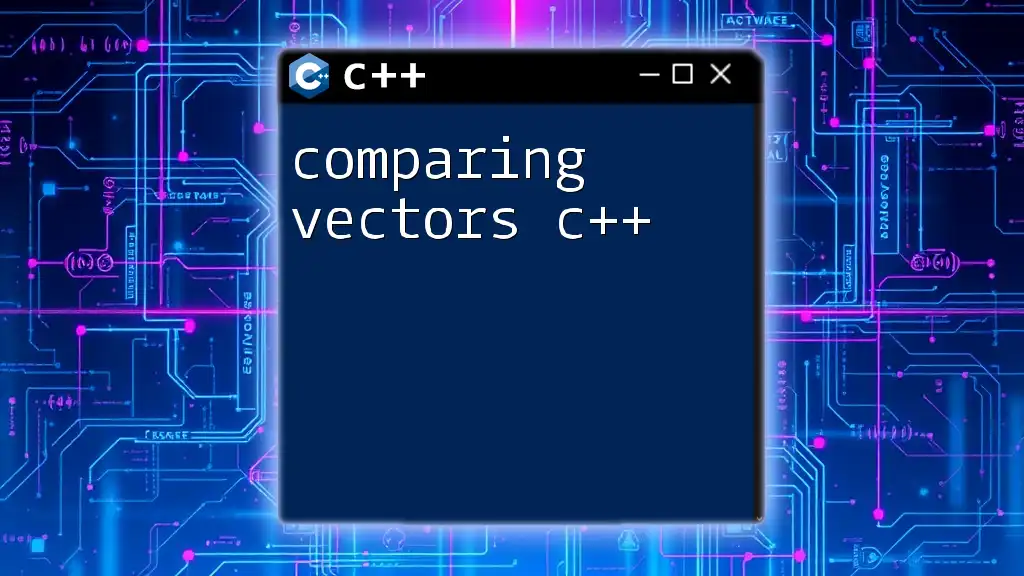
Methods to Concatenate Vectors
Concatenating vectors means merging two or more vectors into a single vector. There are several effective methods to concatenate vectors in C++.
Using `insert()` Function
One straightforward method to concatenate vectors is by using the `insert()` function. This function allows you to insert elements from one vector into another at a specified position.
Syntax: `vector.insert(position, first, last)`
- position: The point at which to insert the elements.
- first / last: The range of elements to insert.
Here’s a code example that demonstrates concatenation using `insert()`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
vec1.insert(vec1.end(), vec2.begin(), vec2.end());
// Output the concatenated vector
for (int i : vec1) {
std::cout << i << " ";
}
return 0;
}
In this example, `vec2` is concatenated to `vec1`, and the resulting output will be: `1 2 3 4 5 6`.
Using the `copy` and `back_inserter` from `<algorithm>`
Another popular method is to use the `copy` function in conjunction with `back_inserter()` from the `<algorithm>` header. This approach allows you to copy elements efficiently while maintaining a clean and concise syntax.
Here’s how you can do this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::vector<int> result;
std::copy(vec1.begin(), vec1.end(), std::back_inserter(result));
std::copy(vec2.begin(), vec2.end(), std::back_inserter(result));
for (int i : result) {
std::cout << i << " ";
}
return 0;
}
This code will also output: `1 2 3 4 5 6`. The `back_inserter` function creates an insert iterator that appends elements to the end of the vector.
Using Range Constructor
You can also concatenate vectors using the range constructor, which allows you to create a new vector initialized with the contents of existing vectors.
Here's an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::vector<int> result(vec1.begin(), vec1.end());
result.insert(result.end(), vec2.begin(), vec2.end());
for (int i : result) {
std::cout << i << " ";
}
return 0;
}
In this case, we first initialize `result` with the elements of `vec1`, then insert elements from `vec2`. The output is the same: `1 2 3 4 5 6`. This method can be particularly useful when you want to concatenate multiple vectors into a new vector in one go.
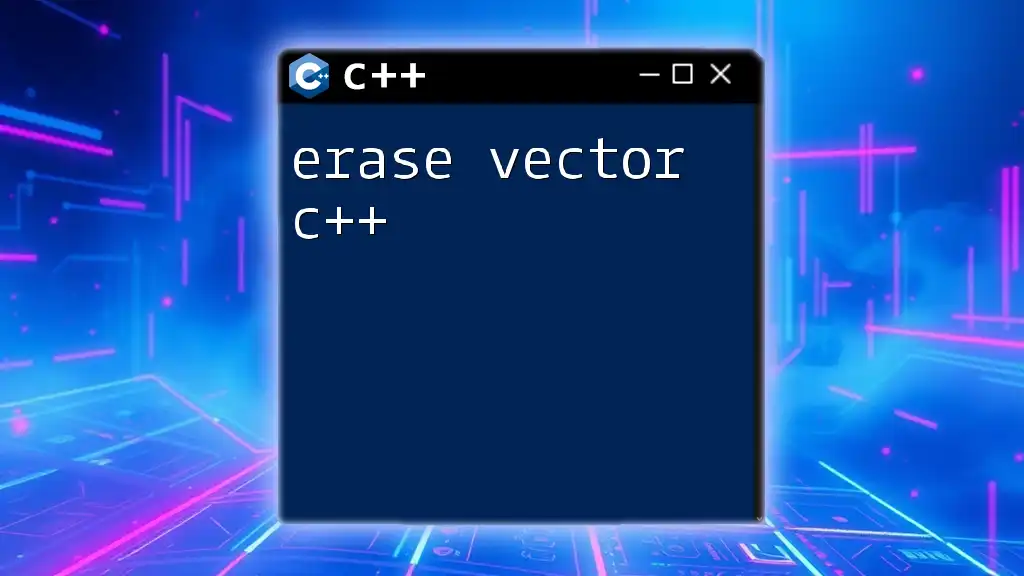
Performance Considerations
Time Complexity of Concatenation Methods
Each method of concatenating vectors has its own time complexity:
- Using `insert()` has a time complexity of O(n) because it may need to move elements to accommodate the new ones.
- The `copy` method with `back_inserter` also runs in O(n), as it effectively copies the elements one by one.
- Using the range constructor followed by `insert()` is similarly O(n).
When choosing a method, consider the size of the vectors and how often you’ll need to concatenate them. For large-scale applications, it's often worth benchmarking each approach based on your specific requirements.
Memory Management
Concatenating large vectors can lead to significant memory use, especially if the destination vector needs to be reallocated to accommodate the combined size. To optimize memory management, it’s helpful to reserve space for the new vector beforehand if you know its final size:
std::vector<int> result;
result.reserve(vec1.size() + vec2.size()); // Reserve space before insertion
This approach can minimize the number of reallocations and improve performance.
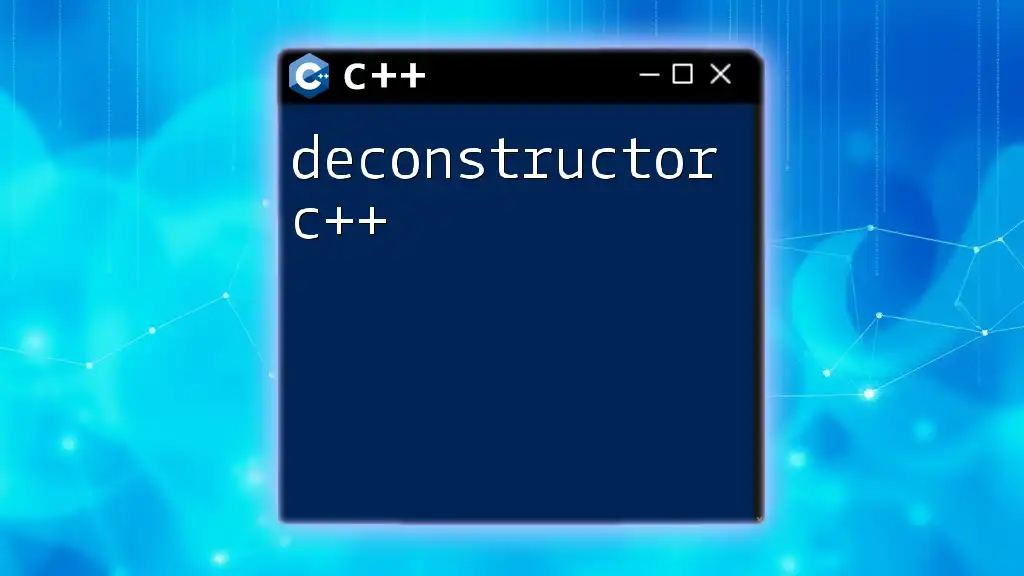
Common Errors and Troubleshooting
Common Issues During Concatenation
While concatenating vectors may seem straightforward, common pitfalls can occur. Issues might arise from:
- Out-of-bounds access: Be cautious when accessing elements.
- Using uninitialized vectors: Ensure vectors are initialized before attempting to concatenate.
Best Practices for Concatenating Vectors
To maintain quality code while performing vector concatenation:
- Opt for efficiency by reserving space when possible.
- Choose the method that best fits your performance needs and coding style.
- Handle exceptions properly to avoid crashes due to memory issues during dynamic memory allocation.
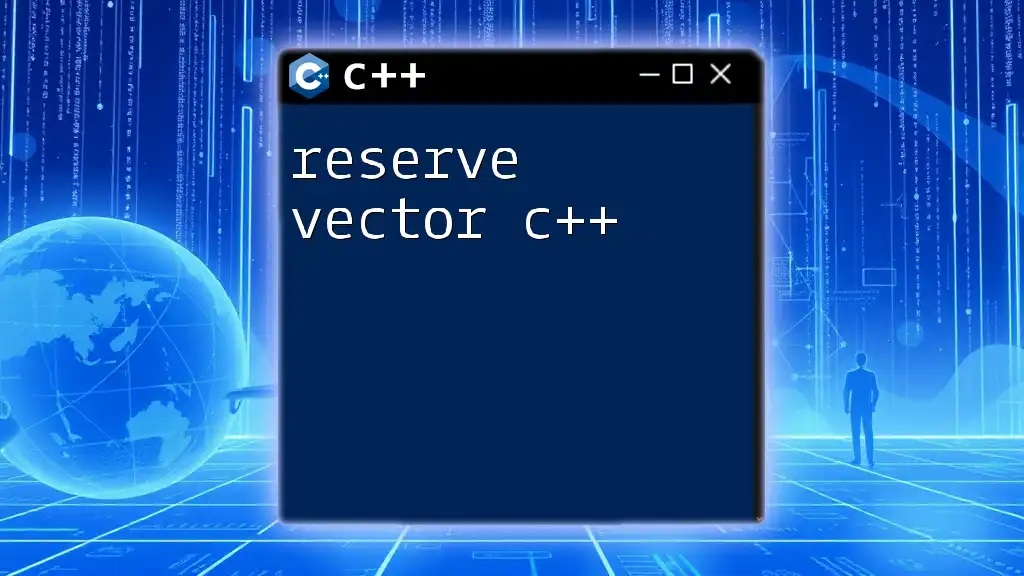
Conclusion
Understanding how to concatenate vectors in C++ is essential for managing collections of data effectively. The methods discussed, such as using `insert()`, `copy` with `back_inserter`, and the range constructor, provide flexible options for merging vectors. Each approach has its strengths, and by considering time complexity and memory management, you can optimize your code for better performance.
Experiment with the provided examples, try out different methods, and explore the capabilities of vectors further. As you gain experience with these operations, your proficiency in using C++ will grow, enabling you to tackle more complex data management challenges confidently.
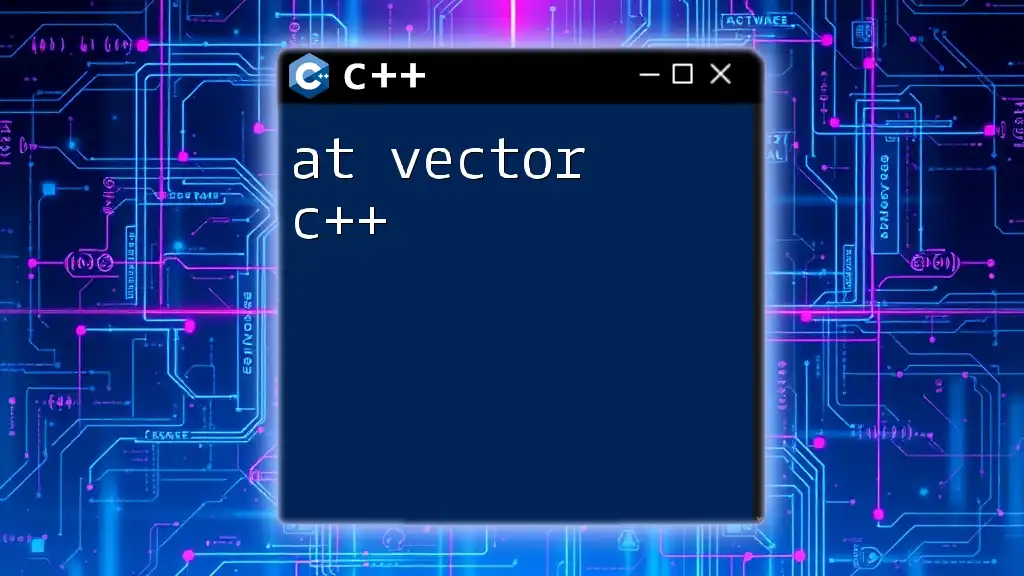
Additional Resources
For those looking to expand their C++ knowledge beyond vector concatenation, consider the following resources:
- Books and online tutorials on C++ STL (Standard Template Library)
- Documentation for the C++ standard library functions
- Community forums and programming challenges focused on data structures
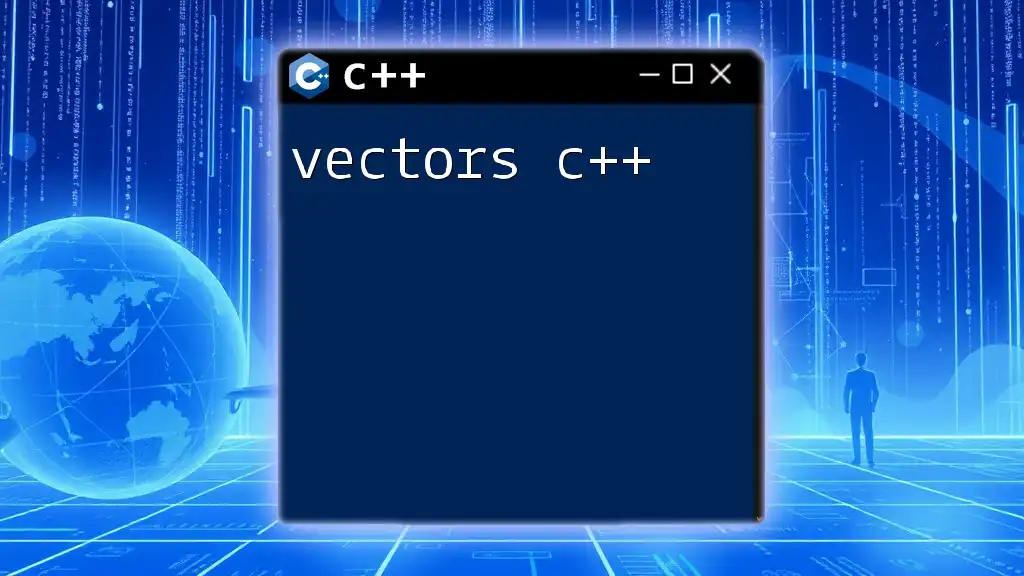
Call to Action
Feel free to share your experiences or questions in the comments below! If you're interested in learning more about C++ programming, sign up for our newsletter to stay updated with the latest guides and tips for beginners and advanced programmers alike.