In C++, a vector is a dynamic array that can resize itself automatically when elements are added or removed, providing an easy way to manage collections of data.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Add an element to the end
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
What is a Vector?
A vector is a dynamic array that can grow and shrink in size, making it a flexible data structure for various programming needs. Unlike fixed-size arrays, vectors allow for efficient memory management and automatic resizing.
Characteristics of Vectors
Vectors in C++ are part of the Standard Template Library (STL) and exhibit several key characteristics:
- They store elements in contiguous memory locations, which allows for easy access via indexing.
- Their size can change during runtime, accommodating scenarios where the number of elements is not known in advance.
- Vectors manage their own memory, which simplifies programming complexity.
Comparison with Arrays
While both arrays and vectors are used to store collections of elements, there are notable distinctions:
- Size Flexibility: Arrays require a predefined size, while vectors can grow or shrink dynamically.
- Memory Management: Vectors handle memory allocation and deallocation automatically. In contrast, arrays necessitate more manual management.
- Functionality: Vectors come with numerous member functions for manipulation, which arrays lack.
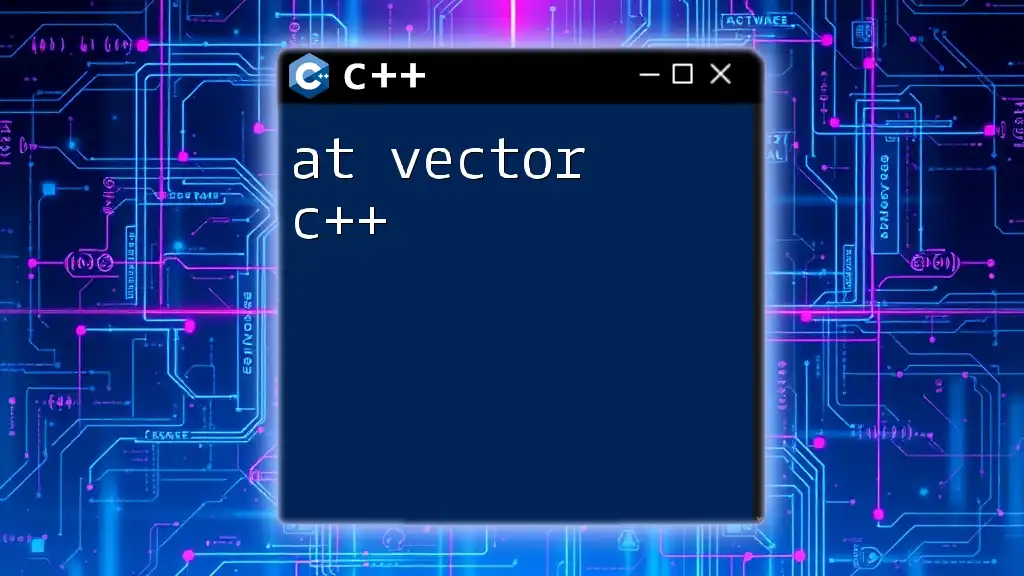
How to Include Vectors in Your Program
Including the Necessary Header
To utilize vectors, include the relevant header at the top of your C++ program:
#include <vector>
Creating a Vector
Creating a vector involves defining its type and optionally providing initial values. Here’s the basic syntax:
std::vector<int> myVector;
You can also initialize a vector with default values:
std::vector<int> myVector(5, 0); // Creates a vector of size 5 with all elements set to 0
For custom initialization, you can provide a list of values:
std::vector<int> myVector = {1, 2, 3, 4, 5};
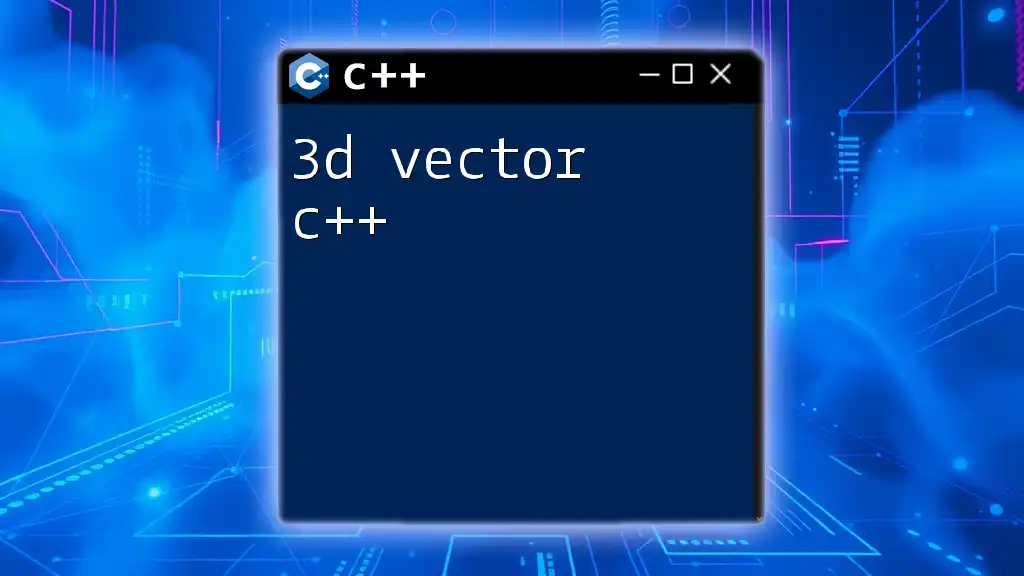
Common Operations on Vectors
Adding Elements
Adding elements to a vector can be performed using the `push_back()` function. This method appends an element to the end of the vector:
std::vector<int> vec;
vec.push_back(10);
vec.push_back(20);
Alternatively, `emplace_back()` can be used to construct elements in place, which can be more efficient for user-defined types:
vec.emplace_back(30);
Accessing Elements
To access elements in a vector, you can use the index operator `[]`, which provides direct access:
int firstElement = vec[0]; // Accesses the first element
The `at()` function offers bounds-checking, preventing out-of-bounds access:
int secondElement = vec.at(1); // Accesses the second element
Modifying Elements
You can change values stored in a vector by assigning new values to specific indices. For instance:
vec[0] = 100; // Modifies the first element to 100
This modification has implications for algorithms that depend on the data being in a specific form, so always consider the context in which modifications are made.
Removing Elements
To remove elements from a vector, you can use `pop_back()` to remove the last element:
vec.pop_back(); // Removes the last element
For removing an element at a specific position, use `erase()`:
vec.erase(vec.begin() + 1); // Removes the element at the second position
You can also clear all elements in the vector using `clear()`:
vec.clear(); // Removes all elements
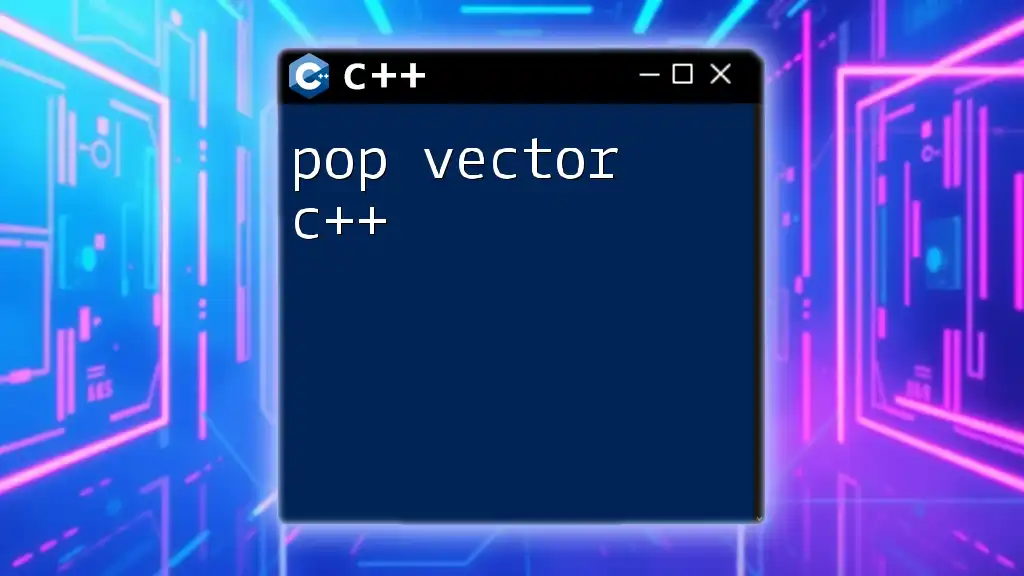
Advanced Vector Features
Iterating Through Vectors
Iterating through vectors can be done using a traditional for loop:
for (int i = 0; i < vec.size(); i++) {
std::cout << vec[i] << " ";
}
A more modern approach is to use a range-based for loop for readability:
for (const auto& elem : vec) {
std::cout << elem << " ";
}
You may also use iterators to traverse the vector:
for (auto it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " ";
}
Sorting and Manipulating
Vectors support standard algorithms from the `<algorithm>` library. For example, you can sort a vector using `std::sort()`:
std::sort(vec.begin(), vec.end()); // Sorts elements in ascending order
This makes vectors a powerful choice for tasks that involve collections of data that need sorting or other manipulations.
Resizing Vectors
To change the size of a vector dynamically, use the `resize()` function:
vec.resize(10); // Resizes the vector to hold 10 elements
If expanded, new elements will be initialized to their default values. This sometimes can lead to performance overhead due to memory reallocations.
Capacity and Size
Understanding the concepts of `size()`, `capacity()`, and `max_size()` is crucial for optimal vector usage:
- size() returns the number of elements currently stored in the vector.
- capacity() gives the amount of space allocated for elements, which can be greater than or equal to `size()`.
- max_size() returns the maximum number of elements the vector can hold.
Knowing these properties can help you efficiently manage memory and performance.
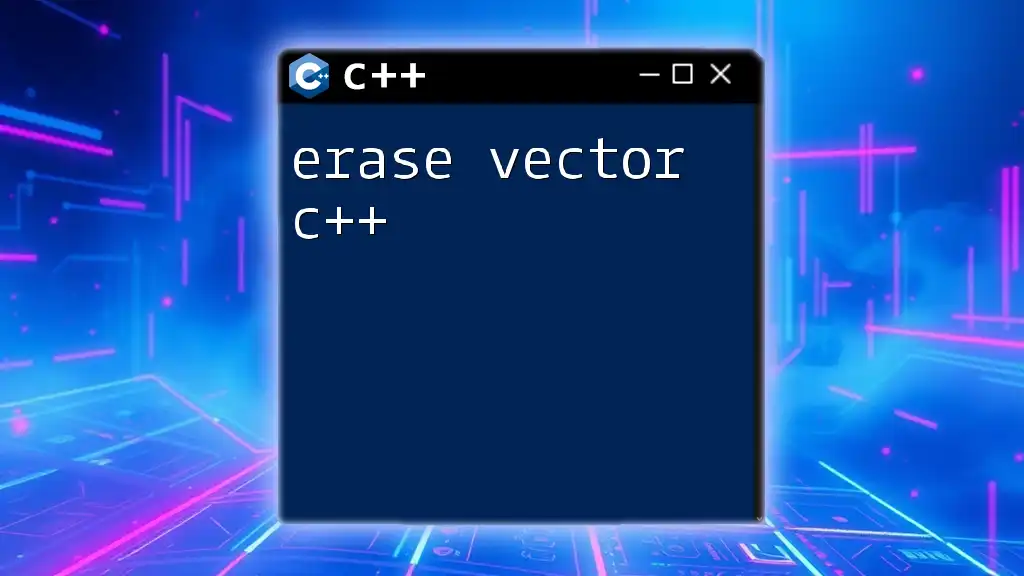
Common Use Cases for Vectors
Dynamic Arrays
Vectors are an excellent choice for dynamic arrays when the number of elements isn't known ahead of time. Their ability to grow and shrink on demand allows for flexible program design while maintaining performance.
Storing Objects
Vectors can also store instances of custom objects. This is extremely useful in object-oriented programming. Here’s an example:
class Person {
public:
std::string name;
Person(const std::string& name) : name(name) {}
};
std::vector<Person> people;
people.emplace_back("John");
people.emplace_back("Jane");
This allows you to create collections of complex data types while leveraging the features of vectors.
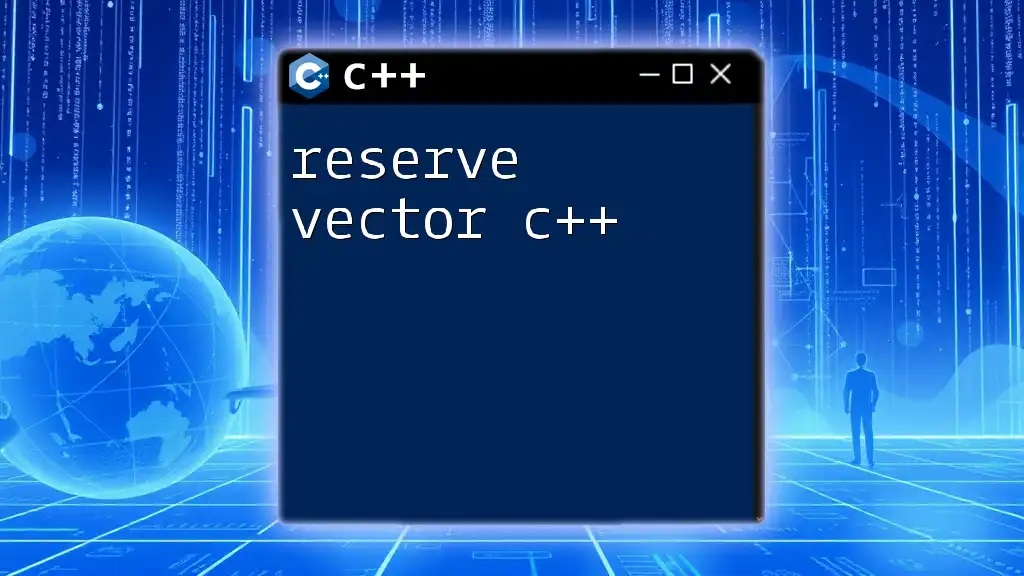
Best Practices for Using Vectors
Memory Management Tips
To avoid memory leaks, it is crucial to manage vector closures properly. Using scoped variables as vectors automatically calls the destructor when they go out of scope, preventing leaks. Additionally, `shrink_to_fit()` can reduce memory allocation after significant deletions.
Performance Considerations
When frequently adding elements to a vector, consider reserving space using `reserve()` to minimize reallocations and optimize performance:
vec.reserve(100); // Reserves space for 100 elements
With this pre-allocation, your vector won't need to expand as elements are added, leading to improved efficiency during operations.
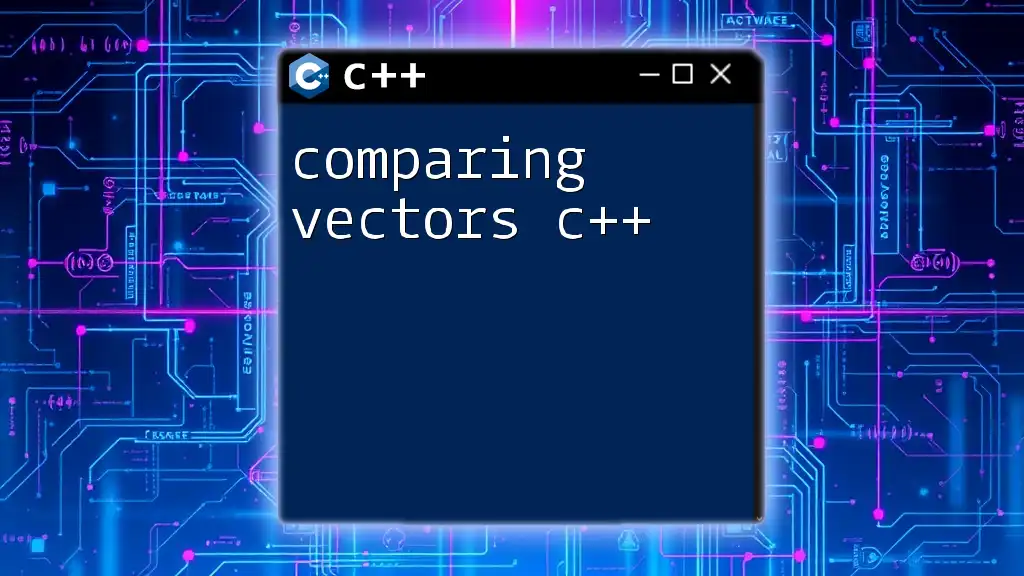
Conclusion
In summary, vectors are a fundamental component of C++ programming, offering dynamic sizing and extensive functionality. With the ability to easily add, access, and manipulate elements, vectors provide a versatile solution ideal for many programming scenarios.
Embracing vectors can significantly enhance your coding practices and lead to more efficient solutions. Whether you're handling dynamic arrays or collections of custom objects, the benefits of using vectors in C++ cannot be overstated.
Now, it's time to practice using vectors in your projects and see how they can simplify your data management! Join our classes for in-depth discussions and hands-on exercises on developing your C++ skills further.