`constexpr` functions in C++ are compile-time evaluated functions that enable improved performance and code optimization by allowing certain expressions to be calculated at compile time rather than at runtime.
constexpr int square(int x) {
return x * x;
}
int main() {
constexpr int result = square(5); // result is calculated at compile time
}
What are constexpr Functions?
Definition of constexpr
In C++, constexpr functions are designed to allow computation at compile time. The term constexpr stands for "constant expression," indicating that the function can be evaluated when the program is being compiled, rather than at runtime. This feature enables developers to optimize performance by performing calculations ahead of time, which can lead to reduced execution times and better efficiency.
Historical Context
constexpr was introduced in C++11 to enable compile-time calculations, but it had limitations. The C++14 standard relaxed some of these restrictions, allowing more complex operations within constexpr functions. With the release of C++17 and C++20, constexpr became even more powerful, expanding its functionalities, such as allowing dynamic memory allocation.
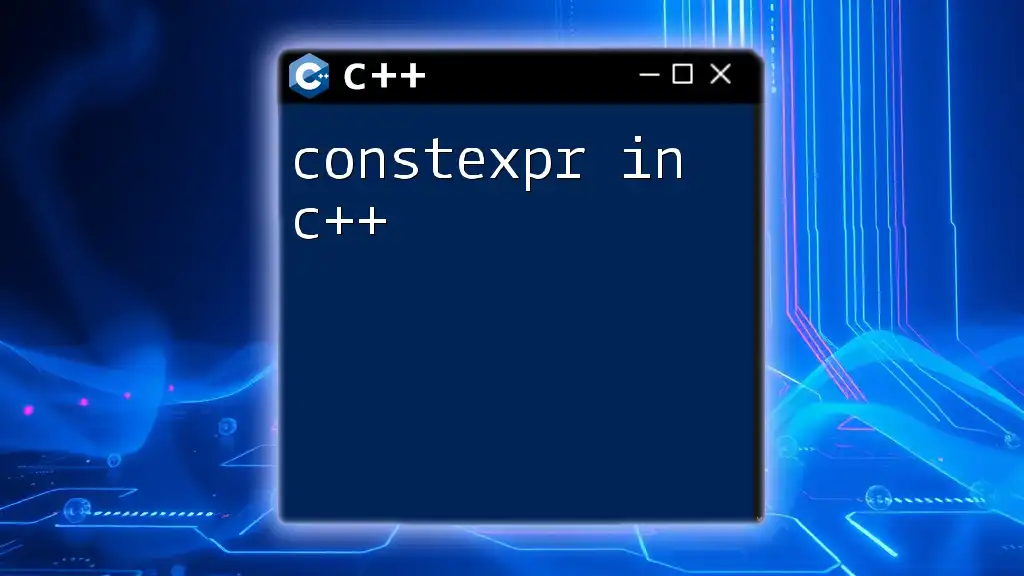
Syntax and Structure of constexpr Functions
Basic Syntax
The syntax for defining a constexpr function is straightforward, resembling that of regular functions. Here's a simple example:
constexpr int square(int x) {
return x * x;
}
In this code snippet, `square` is a constexpr function that computes the square of an integer `x`. Because it is marked as constexpr, the compiler can evaluate `square(5)` during compilation, allowing it to use the result directly in the final binary.
Return Type
It is important to note that constexpr functions must have a return type, such as an integral or non-integral type. Using invalid types, such as a class type without a proper constructor marked as constexpr, will result in a compilation error.
Implicit constexpr
C++ also allows constexpr in lambda expressions and constructors. This gives developers more flexibility. For instance:
auto add = [](int a, int b) constexpr { return a + b; };
In this case, the lambda `add` can be evaluated at compile time if it is invoked with constant parameters.
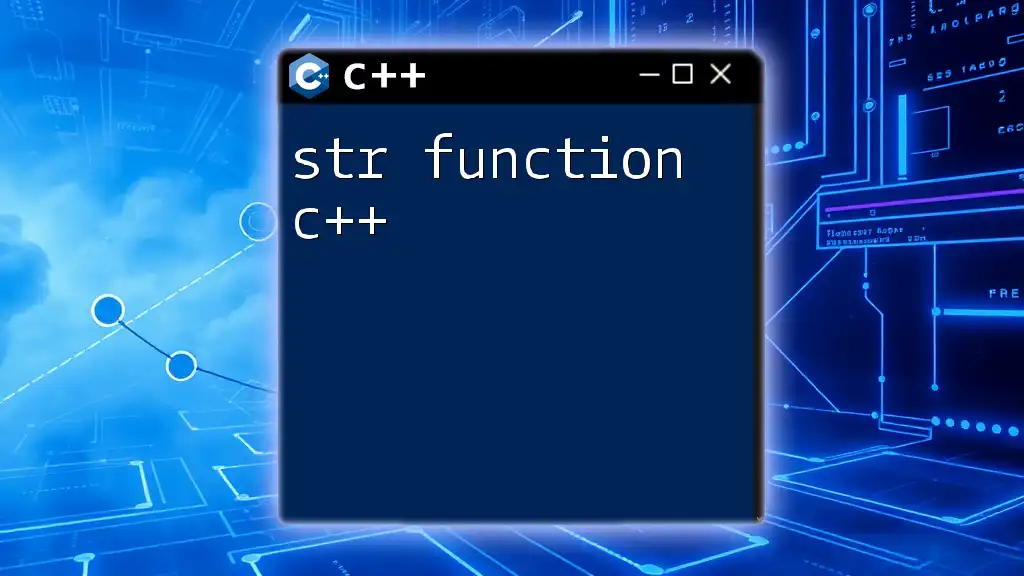
When to Use constexpr Functions
Use Cases
constexpr functions shine in scenarios where calculations can be predefined. This includes:
- Computing values that will be used as template parameters.
- Creating look-up tables that do not change at runtime.
- Any situation where the performance boost from compile-time evaluation is beneficial.
Practical Examples
To illustrate the power of constexpr functions, consider the following examples:
- Fibonacci calculation:
constexpr int fibonacci(int n) {
return (n <= 1) ? n : fibonacci(n - 1) + fibonacci(n - 2);
}
This constexpr function calculates Fibonacci numbers at compile time, allowing `fibonacci(5)` to be evaluated when the program is compiled, saving runtime resources.
- Using constexpr with data structures:
#include <array>
constexpr std::array<int, 5> createArray() {
return {1, 2, 3, 4, 5};
}
In this example, the array is created using a constexpr function, which can then be utilized without incurring additional runtime costs.
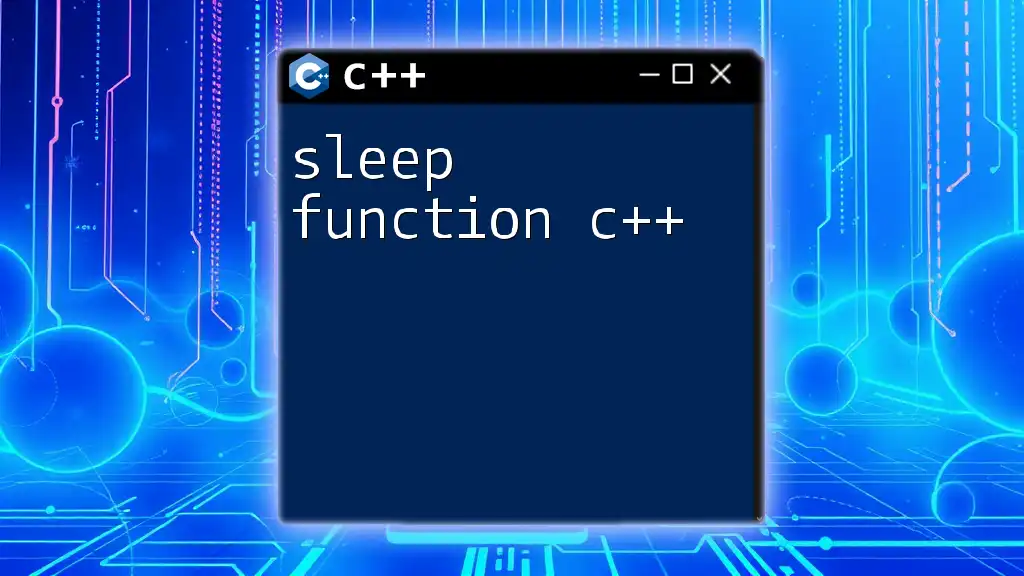
Guidelines for Writing constexpr Functions
Limitations and Constraints
While constexpr functions are powerful, they come with certain constraints:
- Return Type: Must explicitly define a return type.
- Memory Operations: Cannot allocate or deallocate dynamic or static memory.
- Side Effects: Should have no side effects and must be pure functions.
These rules ensure that constexpr functions can be evaluated at compile time without unexpected behavior.
Best Practices
To maximize the utility of constexpr functions, consider the following best practices:
- Simplicity: Keep logic simple to ensure compilers can reliably optimize it.
- Constexpr and Consteval: Utilize `consteval` for functions that must always be evaluated at compile-time.
- Refactoring: Regularly ensure any changes maintain constexpr integrity.
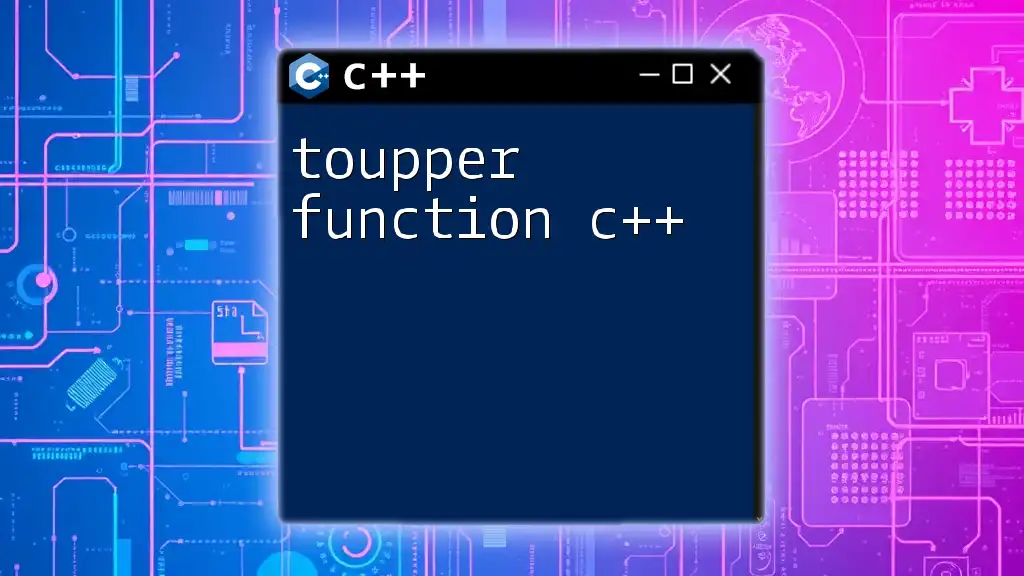
constexpr in Templates
Using constexpr with Template Functions
constexpr functions can also be integrated with template functions, allowing for increased flexibility and efficiency.
template <typename T>
constexpr T max(T a, T b) {
return (a > b) ? a : b;
}
In this template example, a constexpr function determines the maximum value of two parameters at compile time.
Example of Template Specialization with constexpr
Template specialization can also take advantage of constexpr functions.
template <typename T>
struct Checker {
static constexpr bool isIntegral = false;
};
template <>
struct Checker<int> {
static constexpr bool isIntegral = true;
};
Here, `Checker<int>` will yield a compile-time constant indicating whether the type is integral.
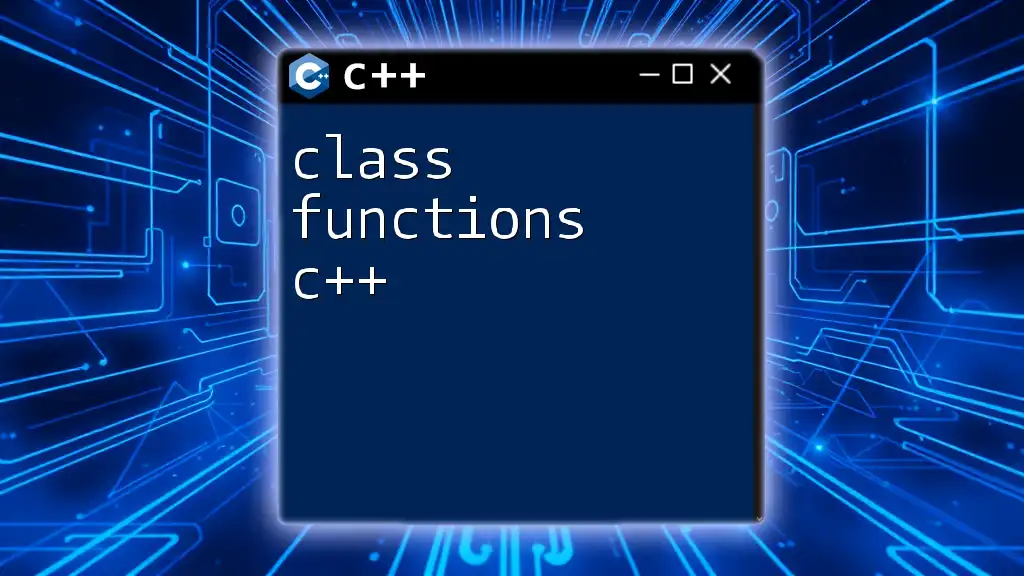
Debugging and Error Handling
Common Errors with constexpr
While using constexpr functions, developers may encounter several errors, including:
- Attempting to utilize non-constant expressions.
- Assigning values to variables that are non-constant or mutable.
These errors usually manifest during compilation, giving immediate feedback.
Tools and Techniques
Debugging constexpr functions can be performed using:
- Compiler warnings: Pay attention to warnings about constant evaluations.
- Static analysis tools that can inspect your code for constexpr related correctness.
Employing unit tests that invoke constexpr functions can also help catch edge cases and ensure functionality remains intact.
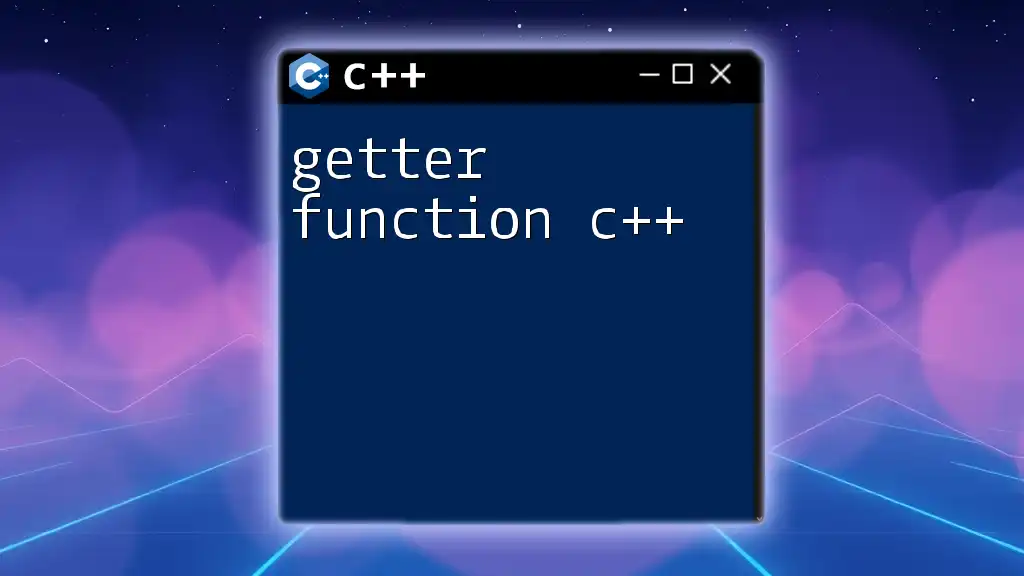
Frequently Asked Questions
Can constexpr functions be overloaded?
Yes, constexpr functions can be overloaded as long as their signatures differ. This means you could have multiple constexpr functions with the same name but different parameter types or counts.
What happens if a constexpr function cannot be evaluated at compile time?
If a constexpr function cannot be evaluated at compile time (due to non-constant inputs, for example), it falls back to being a regular function, and the computation occurs at runtime, which may impact performance.
How to mix constexpr with runtime values?
You can mix constexpr with runtime computations by calling a constexpr function within a runtime context. However, you must ensure it still yields a constant evaluation when possible:
constexpr int base = 10;
int value = base + runtime_variable; // runtime_variable is not constexpr
In this example, `base` is a constant, while `runtime_variable` is computed at runtime.
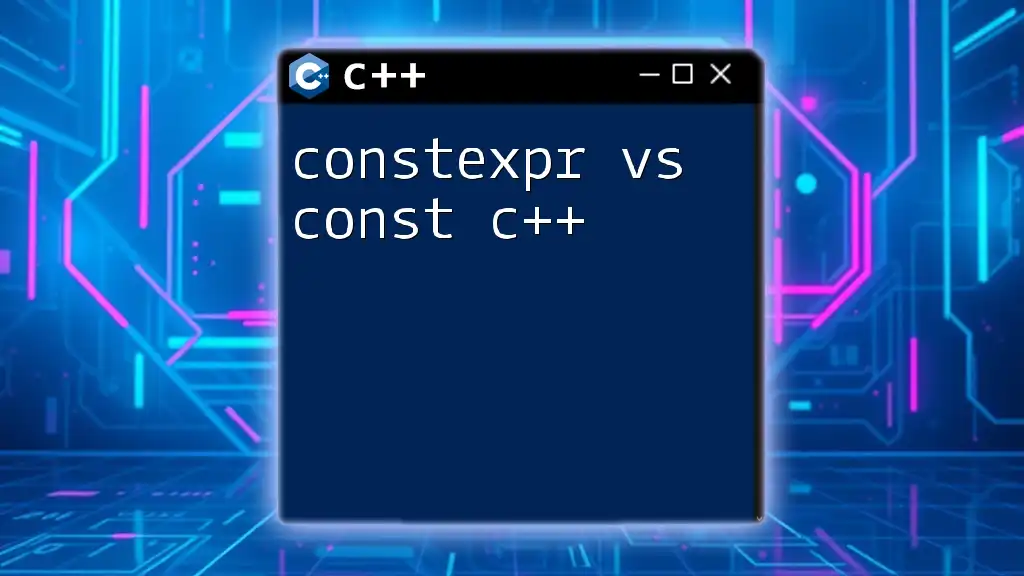
Conclusion
The use of constexpr functions in C++ opens up avenues for optimization by allowing calculations to be done at compile-time. By understanding their syntax, applications, and best practices, developers can harness the full potential of constexpr functions to enhance the efficiency and performance of their C++ programs. Implementing these techniques will lead to cleaner, faster code, and ultimately improve your software's overall quality.
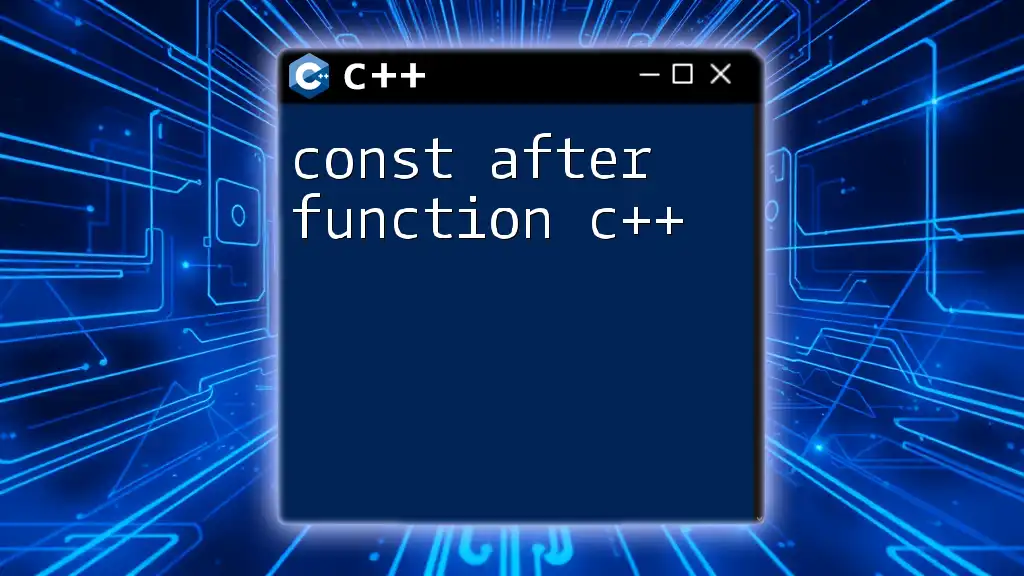
Call to Action
Share your experiences with constexpr functions in C++! What cool projects have you applied them to? Subscribe for more concise C++ tutorials and insights!