In C++, a `const` expression represents a value that is known at compile time and cannot be changed during program execution, allowing for optimization and the use of such values in array sizes or template parameters.
Here’s a simple example of a `const` expression in C++:
#include <iostream>
const int maxSize = 100; // maxSize is a constant expression
int main() {
int arr[maxSize]; // Using const expression to define array size
std::cout << "Array size is: " << maxSize << std::endl;
return 0;
}
What is a Constant Expression?
A constant expression is an expression that can be evaluated by the compiler at compile time. These expressions possess specific characteristics that allow them to be determined during the compilation process rather than at runtime. In C++, expressions declared with the `constexpr` keyword are a prime examples of constant expressions.
For instance, simple arithmetic operations or literal values, such as:
constexpr int sum = 5 + 7;
can be evaluated at compile time. Similarly, more complex calculations that can be computed without parameters that change at runtime also qualify.
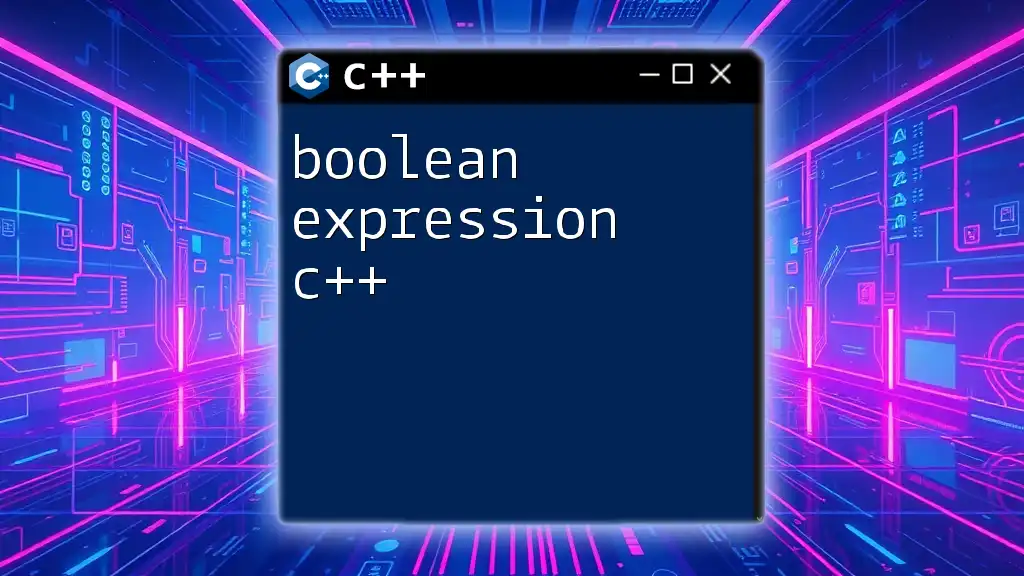
Why Use Constant Expressions?
Using constant expressions offers significant benefits in programming. Here are two primary advantages:
Enhancing Performance
One of the crucial reasons to utilize constant expressions is their ability to enhance performance. When expressions are evaluated at compile time, it eliminates the need for runtime evaluation, which can lead to faster execution of the program. This is especially beneficial for computations that are used multiple times.
For example:
constexpr int width = 10;
constexpr int height = 20;
constexpr int area = width * height; // Evaluated at compile-time
In this example, the computation of the area is resolved during compilation, meaning that when the program runs, it will use the pre-computed value instead of recalculating it.
Improving Code Readability
Using `constexpr` can improve code readability and maintainability. It allows you to express intent clearly and make your code easier to understand. The use of self-descriptive identifiers provides information about their purpose and constraints.
For instance, consider the following code snippet without `constexpr`:
const int maxPlayers = 5; // This value is effectively constant during execution
In contrast, using `constexpr` makes it clearer that `maxPlayers` is meant to be a compile-time constant:
constexpr int maxPlayers = 5; // Clearly indicates compile-time constant
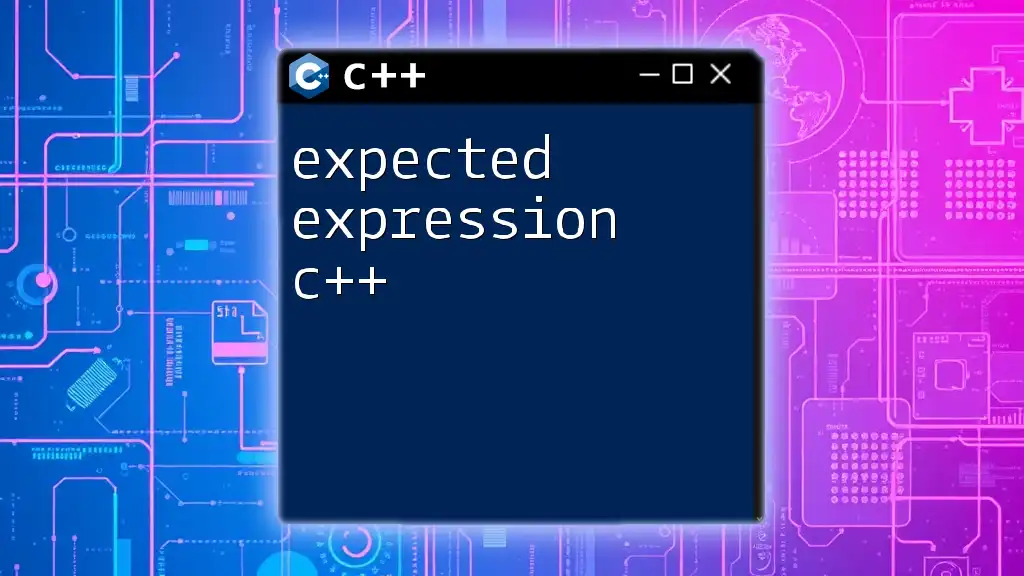
The `constexpr` Keyword
Definition and Purpose
The keyword `constexpr` is used to specify that a variable or function can be evaluated at compile time. It's important to distinguish between `const` and `constexpr`. While `const` variables cannot be modified after initialization, they are not necessarily evaluated at compile time. On the other hand, `constexpr` guarantees compile-time evaluation.
Syntax of `constexpr`
Declaring a variable with `constexpr` is simple and follows a straightforward syntax. Here’s a basic example:
constexpr int max_size = 100; // A compile-time constant
The above code declares `max_size` as a constant expression that can be utilized in scenarios where a compile-time constant is required, such as array sizes.
When to Use `constexpr`
Employing `constexpr` is particularly beneficial in scenarios that require constants for calculations or to define behaviors. For example, using `constexpr` for a function allows you to perform computations at compile-time:
constexpr int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
This function computes the factorial of a number at compile time. When you call `factorial(5)`, the compiler evaluates the result, allowing for more efficient code.
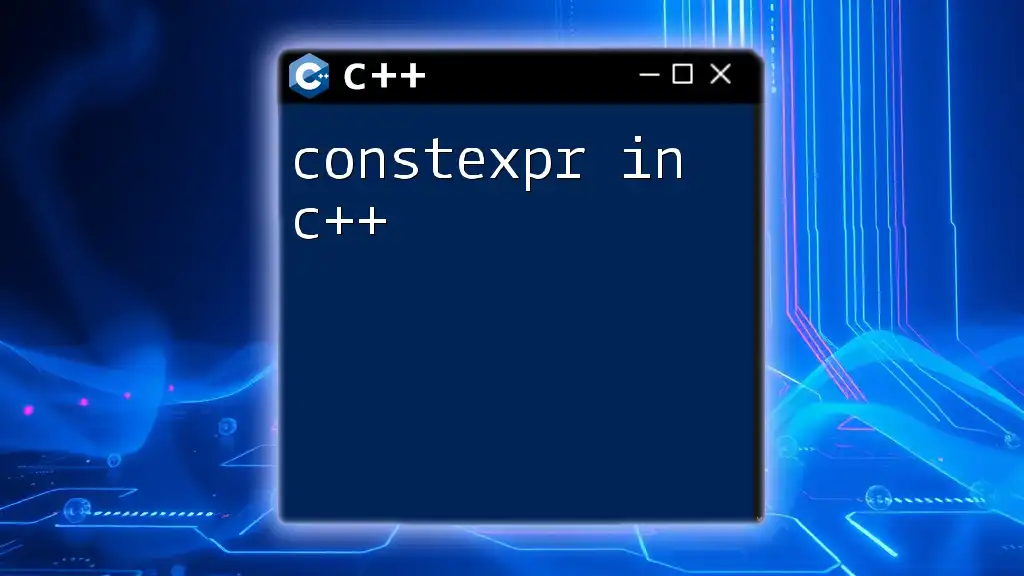
Constant Expressions in Functions
Defining `constexpr` Functions
A function can be marked as `constexpr`, indicating that it can be executed at compile time given appropriate input. Such functions must adhere to specific guidelines, including the absence of dynamic memory allocation.
Here’s an example of a simple `constexpr` function:
constexpr int square(int x) {
return x * x;
}
The `square` function can be used in contexts where compile-time evaluation is needed.
Limitations of `constexpr` Functions
Although `constexpr` allows for powerful compile-time computations, there are limitations to consider. Specifically, you cannot utilize certain constructs within `constexpr` functions, such as dynamic memory allocation or non-constant expressions.
For instance, the following code would result in an error:
constexpr int* allocate() {
return new int(5); // Error: Can't use dynamic memory
}
This example highlights the restriction that `constexpr` functions cannot perform actions that rely on runtime evaluation.
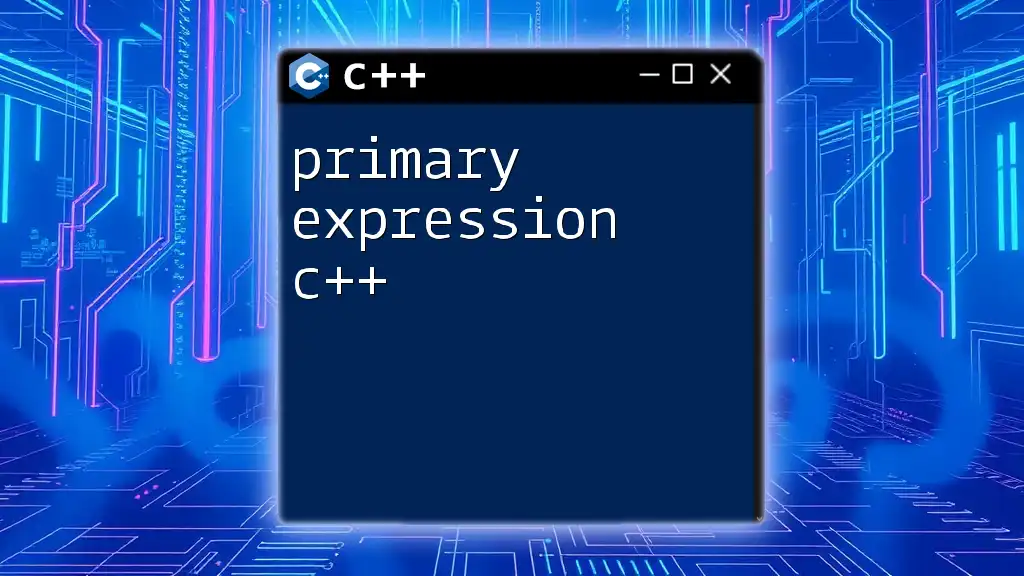
Constant Expressions in Classes
Using `constexpr` with Class Members
Within classes, `constexpr` can be used to define constant member variables and methods. This ensures that these elements can be evaluated at compile time.
Consider the following class example:
class Circle {
public:
static constexpr double pi = 3.14159; // Static constant member variable
constexpr Circle(double r) : radius(r) {}
constexpr double area() const { return pi * radius * radius; }
private:
double radius;
};
In this instance, `pi` is a static constant, and the `area` method is defined to be evaluated at compile time when possible.
Static Data Members and `constexpr`
Static data members in a class can also be declared with `constexpr`, reinforcing their immutability and compile-time evaluation. Here’s an example:
class Math {
public:
static constexpr int max_value = 1000; // Static constant data member
};
This definition ensures that `max_value` is available for use as a compile-time constant, enhancing performance and readability.
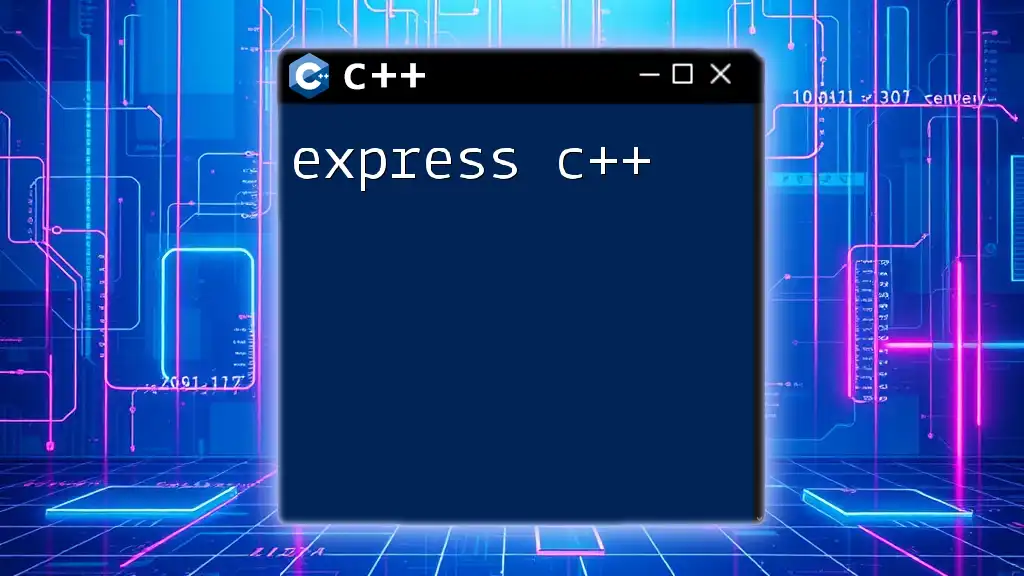
Practical Use Cases of Constant Expressions
Compile-Time Computation
Constant expressions shine particularly in template programming and metaprogramming. They provide mechanisms for performing calculations at compile time, enabling more efficient algorithms and structures.
Here is an example demonstrating compile-time calculations through templates:
template<int N>
struct Factorial {
static constexpr int value = N * Factorial<N - 1>::value;
};
template<>
struct Factorial<0> {
static constexpr int value = 1; // Base case for recursion
};
In this case, the template structure `Factorial` calculates the factorial of a number at compile time.
Use in Standard Library
Many constructs in the C++ Standard Library utilize constant expressions extensively. For instance, sizes of fixed arrays or template parameters often rely on `constexpr` for performance and optimization.
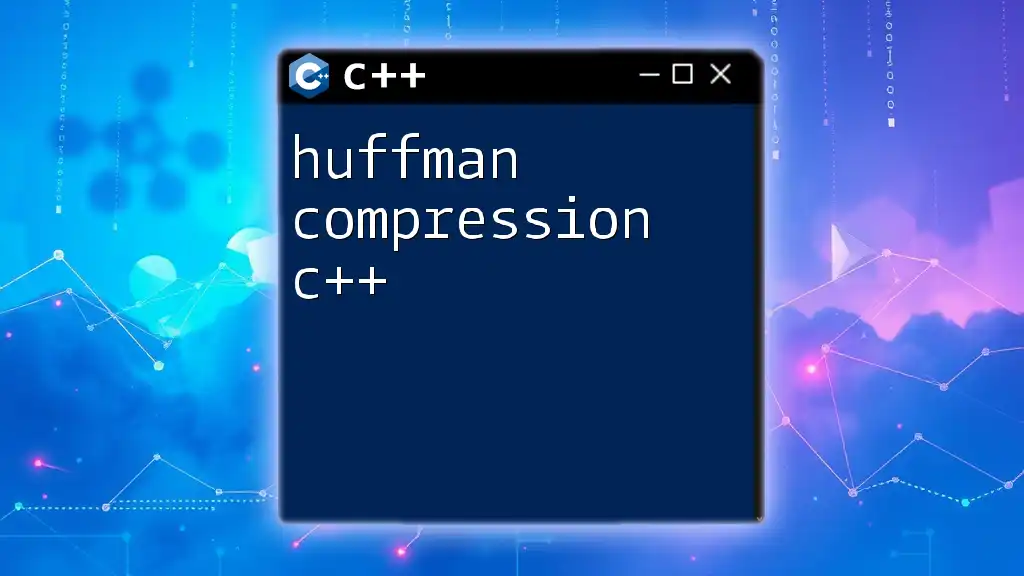
Common Mistakes with Constant Expressions
When working with constant expressions, it is crucial to avoid certain common pitfalls, such as misusing `constexpr` in the wrong context or trying to declare non-constant expressions as `constexpr`. Remember that only functions and expressions that can be evaluated at compile time are eligible for `constexpr`.
Moreover, be mindful of the scope and visibility of `constexpr`. Variable scope can affect whether a value is genuinely constant throughout your code.
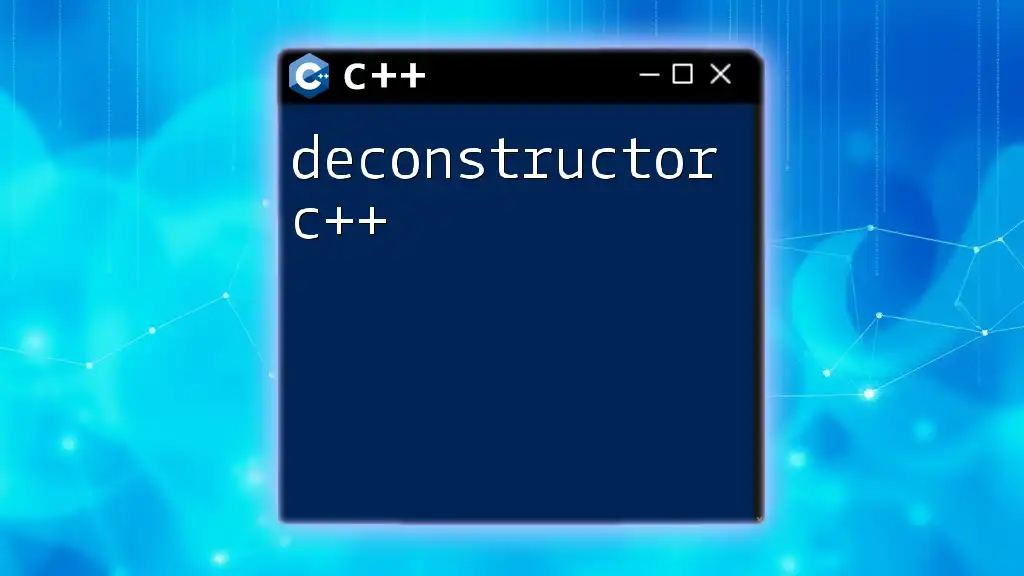
Conclusion
Constant expressions in C++ are invaluable tools that enhance both performance and code clarity. By leveraging the `constexpr` keyword, developers can optimize their applications significantly. As you continue to code in C++, consider the numerous benefits that constant expressions provide and integrate them into your programming practices. Practicing the use of `constexpr` will not only refine your skills but also lead to robust, efficient code.