In C++, the "expected expression" error generally indicates that the compiler encountered a situation where it was expecting a valid expression but found something else, often due to syntax errors or missing components in the code.
Here’s a simple example that triggers this error:
#include <iostream>
int main() {
int a = 5;
int b; // Missing initialization or assignment here causes an error
a + b; // This will cause the "expected expression" error if used incorrectly
return 0;
}
What is an Expression in C++?
In C++, an expression is a combination of variables, constants, operators, and function calls that computes a value. Expressions are essential as they form the basis of all C++ statements, allowing you to perform operations, evaluate conditions, and manipulate data.
Types of Expressions
Understanding the different types of expressions can help you effectively utilize them in your programs. Here are the main types:
-
Arithmetic Expressions: These involve arithmetic operators like `+`, `-`, `*`, and `/`. For example:
int a = 5; int b = 10; int sum = a + b; // sum is now 15
-
Relational Expressions: These use comparison operators such as `==`, `!=`, `<`, `>`, `<=`, and `>=`, and evaluate to `true` or `false`. For example:
int x = 10; bool isGreater = x > 5; // isGreater is true
-
Logical Expressions: These utilize logical operators like `&&` (AND), `||` (OR), and `!` (NOT) to combine boolean values. For instance:
bool condition1 = true; bool condition2 = false; bool result = condition1 && condition2; // result is false
-
Bitwise Expressions: These expressions manipulate bits and use operators like `&`, `|`, `^`, `<<`, and `>>`. For example:
int a = 5; // 0101 in binary int b = 3; // 0011 in binary int bitwiseAND = a & b; // bitwiseAND is 1 (0001 in binary)
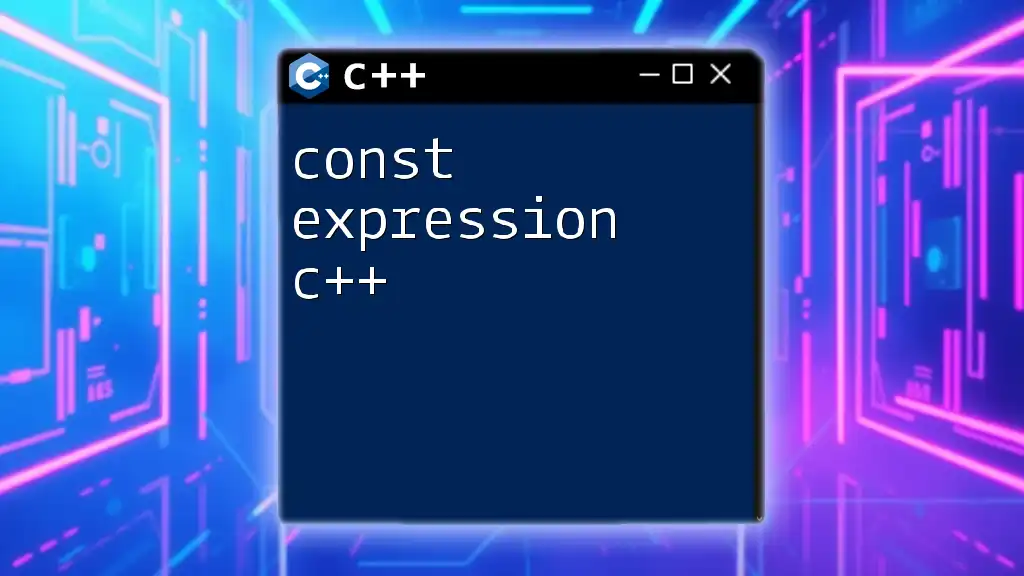
Understanding the "Expected Expression" Error
Definition of "Expected Expression" Error
The "expected expression" error in C++ indicates that the compiler encountered a point in your code where it anticipated an expression but did not find one. This error often leads to confusion as it can arise from different issues in your syntax.
Common Causes of the Error
Several common mistakes can lead to this error:
-
Missing Variable: If you reference a variable that hasn't been declared, the compiler will throw an "expected expression" error. For example:
int a = x + 5; // x is undeclared -> error
-
Syntax Errors: Failing to follow C++ syntax rules, like missing semicolons, can trigger this error. For instance:
int a = 5 // Missing semicolon here -> error
-
Incorrect Function Calls: Not providing all required arguments when calling a function can result in this error. Example:
int add(int x, int y) { return x + y; } int result = add(5); // Missing second argument -> error
-
Misplaced Operators: Using operators inappropriately, such as placing them where the compiler expects an expression, can lead to confusion and errors. For example:
int a = 5 +; // Incorrect use of the + operator -> error
Example Scenarios
Consider the following code snippets that result in the "expected expression" error:
-
Missing Variable
int total = quantity + 100; // 'quantity' was never declared
-
Syntax Error
if(x > 0) // Missing the opening brace int y = 10; // Error detected at this line
-
Incorrect Function Call
std::cout << add(5); // Correct: add(int, int) requires two arguments
In each case, the compiler halts processing and signals an "expected expression" error, often accompanied by a line number for context.
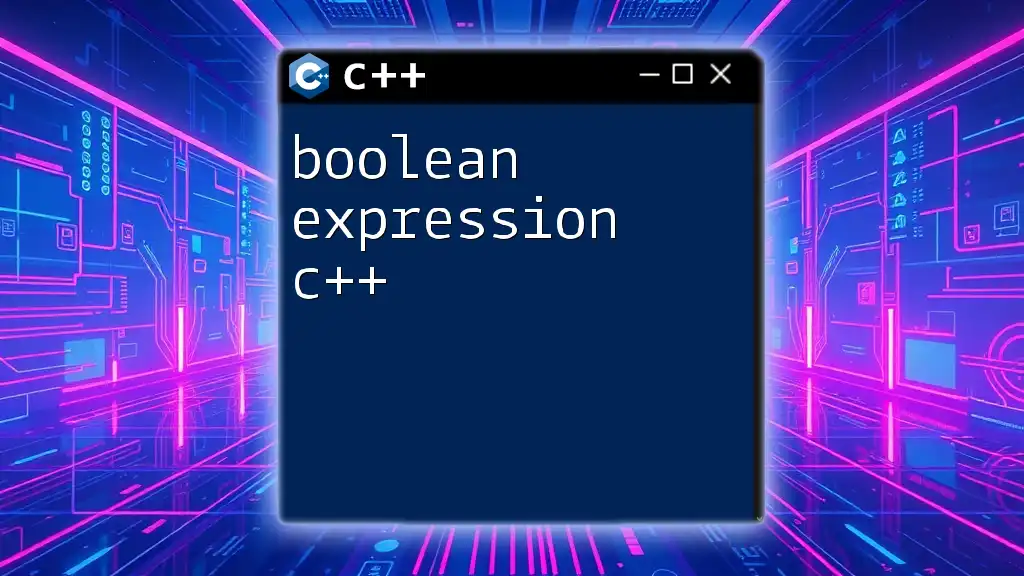
Fixing the "Expected Expression" Error
Identifying the Error Location
Using debugging tools and features in your IDE can help you pinpoint where this error arises. Many modern IDEs highlight the line of code causing the issue, making it easier to address.
Steps to Resolve "Expected Expression" Error
When you encounter the "expected expression" error, consider these steps to resolve it:
-
Step 1: Read the Error Message Carefully
Understanding the error message is crucial. It usually provides insight into where the compiler got confused. -
Step 2: Check for Syntax Errors
Carefully review the syntax around the reported line. For example, if there's a missing semicolon:int num = 10 // Correct with a semicolon int sum = num + 5;
-
Step 3: Verify Function Calls
Make sure all required parameters are provided in function calls and functions are being utilized correctly.int multiply(int x, int y) { return x * y; } int total = multiply(5, 2); // Correct function call
-
Step 4: Review Operator Usage
Ensure that operators are used appropriately. An improper operator placement could lead to semantic confusion.int count = 10 + ; // Remove the misplaced `+`
Code Correction Examples
Here are a few before-and-after examples demonstrating how to correct the "expected expression" error:
-
Before: Missing Declaration
int total = value + 10; // 'value' not declared
After: Declare Variable
int value = 5; int total = value + 10; // Now correct
-
Before: Syntax Error
if(x > 5) // Missing braces int y = 10; // Error detected here
After: Fix Syntax
if(x > 5) { int y = 10; // Now correct }
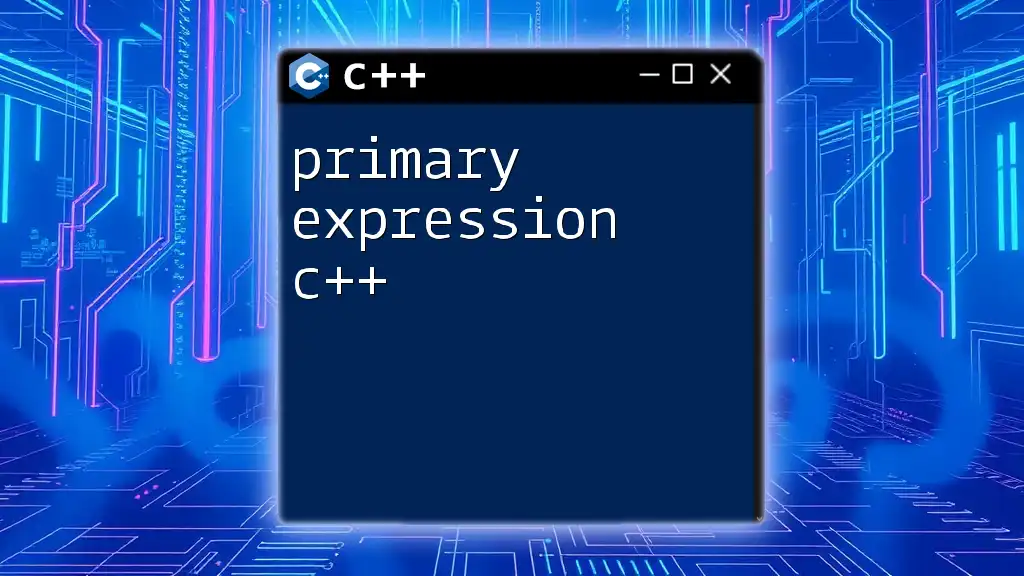
Best Practices to Avoid the "Expected Expression" Error
Regular Code Reviews
Conducting regular code reviews helps catch issues like the "expected expression" error early. This practice promotes a culture of collaborative problem-solving and learning.
Use of Comments and Documentation
Incorporating comments within your code aids clarity and can help you and others understand complex expressions more easily.
IDE Features and Tools
Leverage tools provided by IDEs to highlight potential syntax issues. Code suggestions and syntax highlighting can minimize errors before runtime.
Consistent Code Formatting
Maintaining consistent formatting practices not only makes your code more readable but also helps prevent careless mistakes that lead to errors like these.
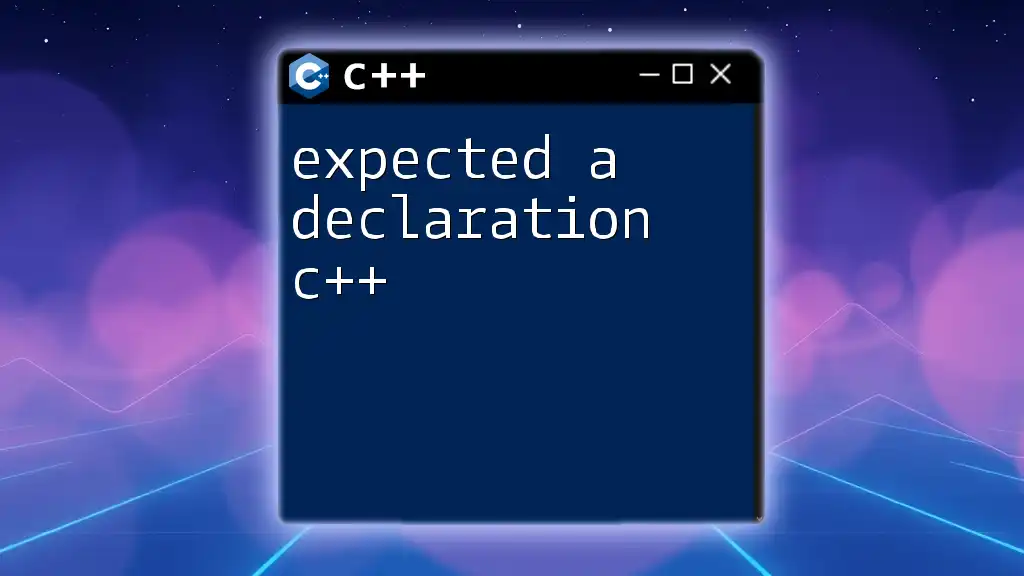
Conclusion
Understanding expressions in C++ and the factors that lead to the "expected expression" error is crucial for any programmer. By recognizing common pitfalls and utilizing best practices, you can write more efficient, error-free C++ code.
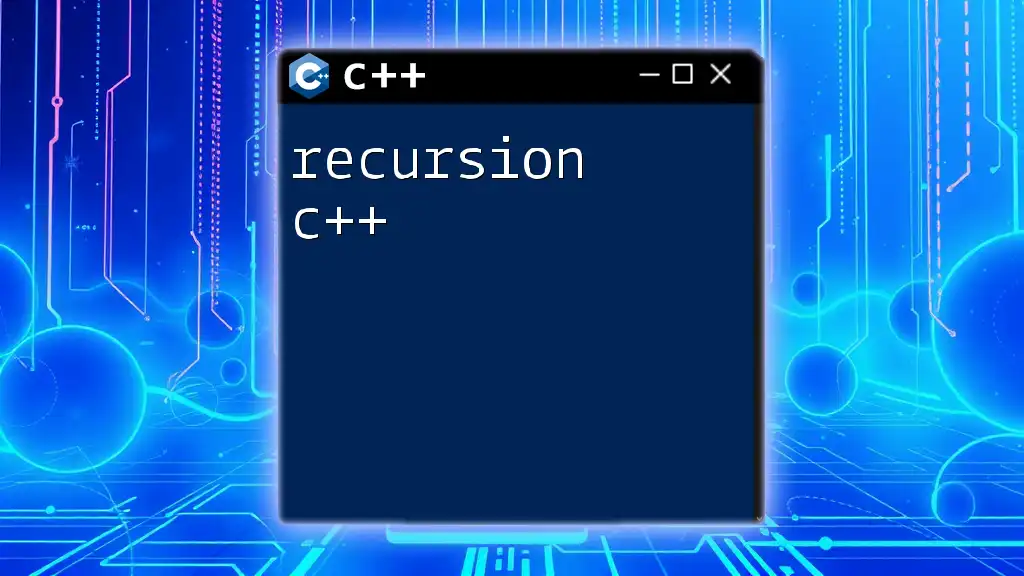
Additional Resources
Books
- C++ Primer by Stanley Lippman
- Effective C++ by Scott Meyers
Online Courses
- Coursera's C++ for C Programmers
- Udacity's C++ Nanodegree Program
Forums and Communities
Engage in C++ programming communities such as Stack Overflow and the C++ subreddit for advice, troubleshooting, and learning opportunities.
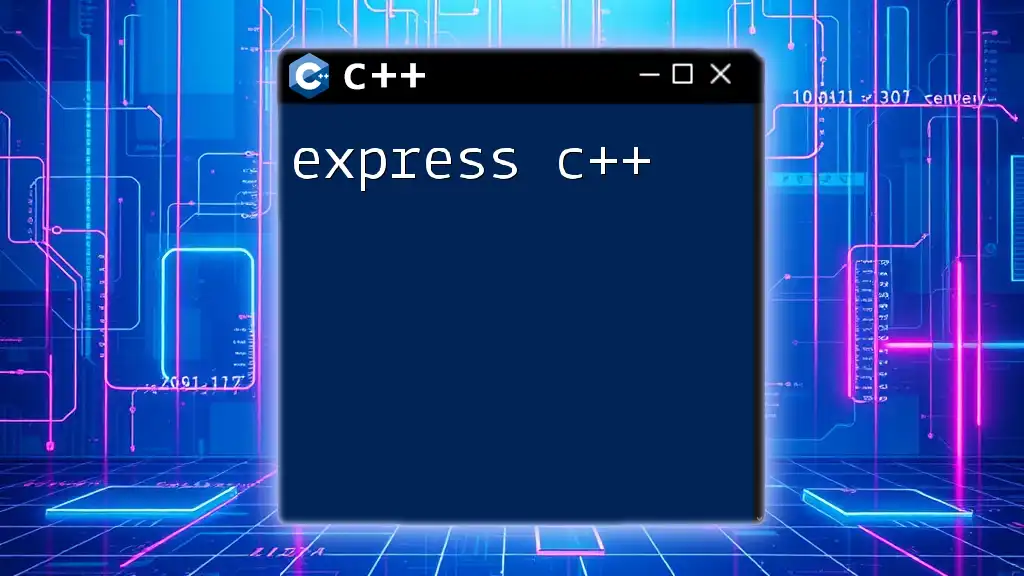
Call to Action
Share your experiences or challenges with the "expected expression" error in the comments below. Consider signing up for our courses to get concise, efficient training on C++ commands and best practices!