Embedded systems C++ refers to the use of C++ programming language in designing and developing software that runs on dedicated hardware devices, often with constraints on resources like memory and processing power.
Here’s a simple example of controlling an LED using C++ in an Arduino embedded system:
#include <Arduino.h>
const int ledPin = 13; // Pin where the LED is connected
void setup() {
pinMode(ledPin, OUTPUT); // Set the LED pin as an output
}
void loop() {
digitalWrite(ledPin, HIGH); // Turn the LED on
delay(1000); // Wait for a second
digitalWrite(ledPin, LOW); // Turn the LED off
delay(1000); // Wait for a second
}
Understanding Embedded C++ Programming
What is Embedded C++ Programming?
Embedded C++ programming refers to the use of the C++ programming language tailored for embedded systems. Unlike standard C++, embedded C++ focuses on creating applications for constrained environments, such as microcontrollers and real-time applications. The primary objective is to utilize the capabilities of C++ while adhering to the memory and performance limitations typical of embedded systems.
Advantages of C++ for Embedded Systems
Choosing C++ for embedded systems brings several advantages:
-
Performance: C++ offers high-level programming constructs with low-level capabilities, which allows programmers to write efficient and optimized code. This efficiency can be crucial in applications where processing speed is vital.
-
Memory Management: With C++, developers have control over memory allocation, allowing them to use dynamic memory judiciously to reduce fragmentation, which is critical in resource-constrained environments.
-
Object-Oriented Features: C++'s object-oriented programming features, such as encapsulation, inheritance, and polymorphism, enable complex systems to be modeled more naturally and maintainably. This improves reusability and reduces development time.
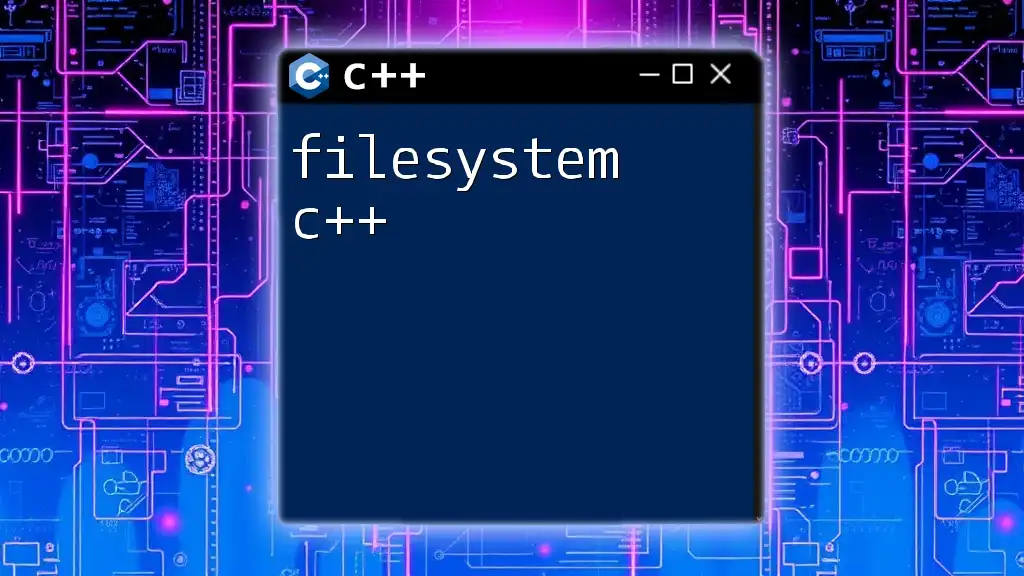
Essentials of C++ Programming for Embedded Systems
Setting Up Your Embedded C++ Development Environment
To start with embedded C++ programming, you need a robust development environment. Recommended IDEs for this purpose include:
- Eclipse with the CDT plugin
- Keil µVision
- CodeLite
Setting up involves configuring compilers and toolchains specifically designed for embedded systems, such as GCC for ARM or AVR-GCC. Ensure to include the relevant headers for your target microcontroller to access specific registers and peripherals.
Understanding the Basics: C++ Syntax and Features
To navigate embedded C++ programming, familiarize yourself with basic syntax and key features, including:
-
Classes and Objects: These help in modeling real-world entities, providing a clear structure to the code.
-
Inheritance and Polymorphism: These concepts allow developers to define simple interfaces and extend functionality without modifying existing code. This supports better code organization and reinforces the DRY (Don't Repeat Yourself) principle.
-
Operator Overloading: This feature allows the customization of how operators work with user-defined types, enhancing code readability.
C++ Standard Library in Embedded Systems
While the C++ Standard Library provides many useful features, it often comes with a significant memory and performance overhead. In embedded systems, it’s advisable to use only those components of the library that are lightweight and necessary. Developers may choose to implement simpler alternatives for collections, strings, and I/O operations to ensure that they remain within resource constraints.
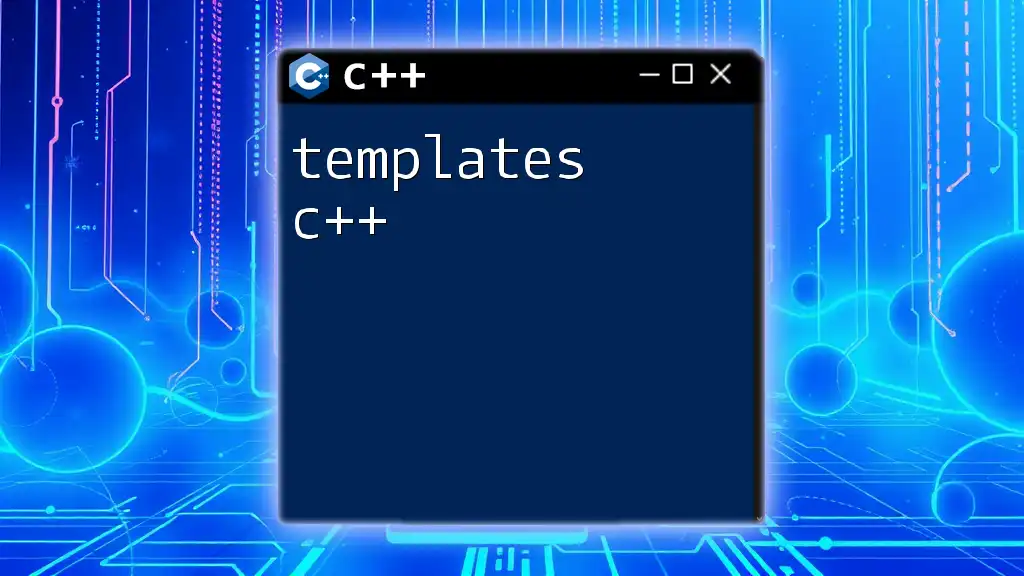
Specialized Topics in C++ Embedded Programming
Writing Efficient C++ Code for Embedded Systems
Efficiency is vital in embedded programming. Here are some strategies for writing optimized C++ code:
-
Minimize Memory Usage: Utilize fixed-size arrays and avoid using STL containers that might dynamically allocate memory.
-
Inline Functions: Use inline functions to eliminate function call overhead, which can be beneficial in performance-critical scenarios.
-
Profile Your Code: Analyze which parts of the code consume the most resources and optimize accordingly.
Real-Time Operating Systems (RTOS) and C++
Real-Time Operating Systems (RTOS) are critical in many embedded applications. They manage hardware resources to ensure timely task completion. C++ can be effectively used in conjunction with an RTOS, enabling developers to structure application logic while handling multitasking and scheduling effectively.
For example, when using FreeRTOS, you might create tasks as follows:
#include <Arduino.h>
#include <FreeRTOS.h>
void vTask1(void *pvParameters) {
while (1) {
// Task code
}
}
void setup() {
xTaskCreate(vTask1, "Task1", 1000, NULL, 1, NULL);
vTaskStartScheduler();
}
void loop() {
// Empty. RTOS handles everything.
}
This structure illustrates how C++ can handle multitasking in an embedded system context.
Handling Interrupts and Timers
Interrupt handling is crucial for responsive embedded applications. Understanding the differences between blocking and non-blocking calls is essential. Non-blocking calls ensure that the program can continue running while waiting for an event, which can be implemented using interrupts.
For instance, here’s a simple code snippet for handling interrupts:
volatile bool buttonPressed = false;
void setup() {
pinMode(2, INPUT);
attachInterrupt(digitalPinToInterrupt(2), isr, RISING);
}
void isr() {
buttonPressed = true;
}
void loop() {
if (buttonPressed) {
// Respond to button press
buttonPressed = false;
}
}
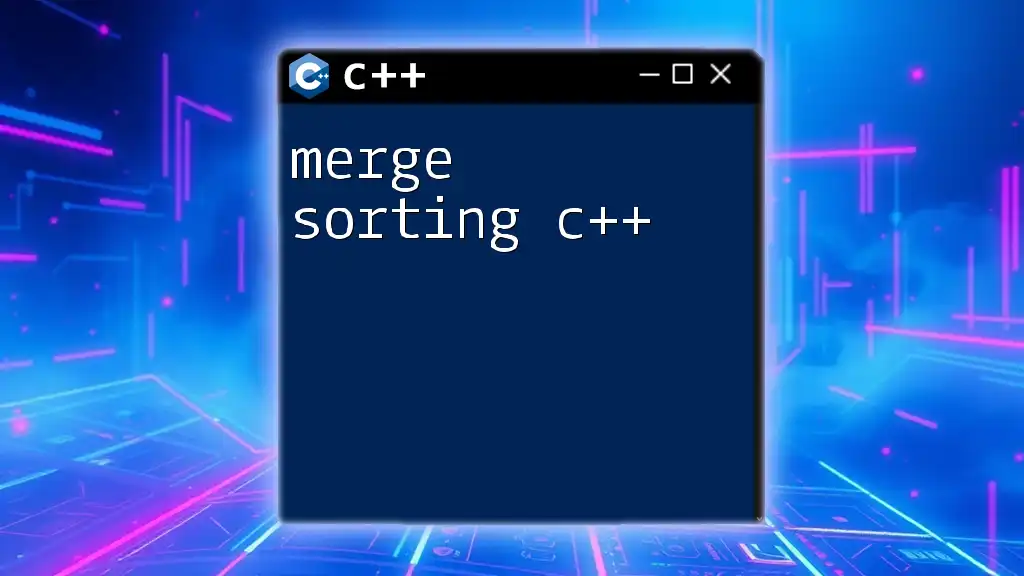
Best Practices in Embedded Programming with C++
Code Organization and Standards
Organizing code logically and adhering to coding standards is vital in any programming environment, and it becomes critically important in embedded systems where multiple developers might be involved. Use clear naming conventions, modular functions, and consistent formatting. Examples include following the MISRA C++ guidelines, which help to prevent undefined behavior and improve code quality.
Debugging Techniques in Embedded C++
Debugging embedded systems can be challenging due to limited visibility into the running code. Effective debugging techniques include:
-
Using Debuggers: Tools like GDB (GNU Debugger) allow stepping through code and inspecting variables at runtime.
-
Logging: Implement logging through serial outputs to observe system behavior and pin down issues.
Example of logger implementation:
void log(const char *message) {
Serial.println(message);
}
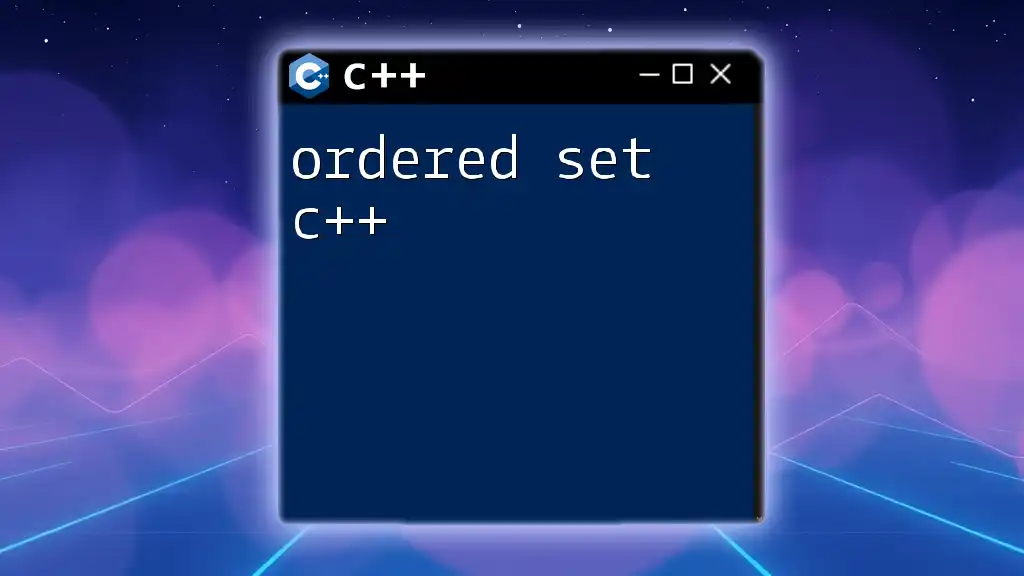
Case Studies and Real-World Applications
Successful Use Cases of C++ in Embedded Systems
C++ has been successfully implemented in various embedded applications. For instance:
-
Automotive Systems: Many modern cars use C++ in engine control units (ECUs) due to its performance and object-oriented capabilities.
-
IoT Devices: With the rise of smart devices, C++ has proven essential in building responsive and efficient IoT solutions that interface with hardware.
Challenges of Using C++ in Embedded Systems
Despite its advantages, using C++ presents challenges:
-
Complexity: The language's advanced features can introduce complexity, making code harder to manage in some cases.
-
Memory Usage: If not optimized, C++ code could lead to significant memory consumption. Developers need to be careful when using features like exception handling or RTTI (Run-Time Type Information).
-
Pros and Cons of Using C++ vs. C: While C++ offers more powerful abstractions, C might sometimes be preferred for more straightforward embedded tasks due to its simplicity and smaller footprint.
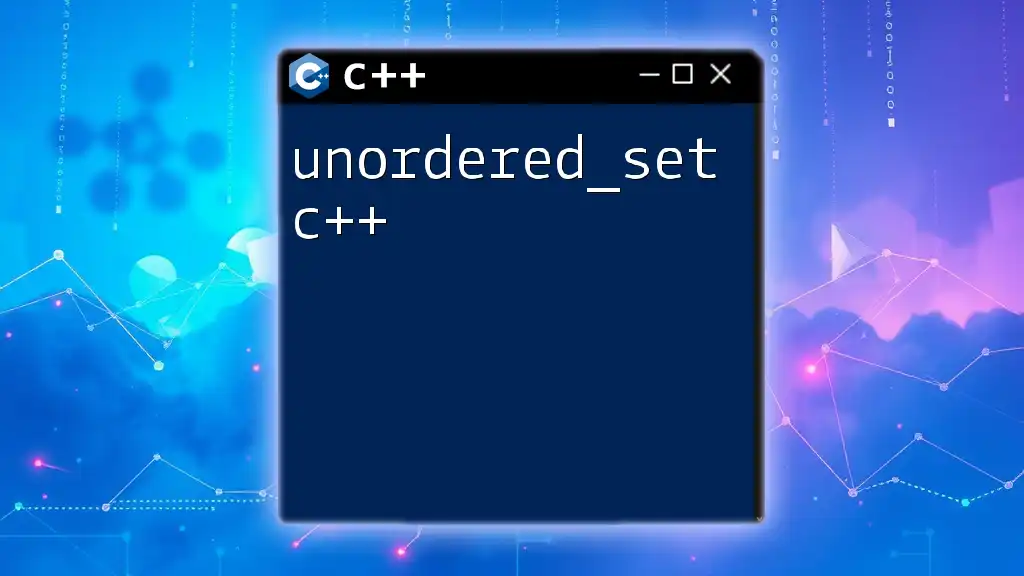
Conclusion
In conclusion, embedded systems C++ offers a powerful toolset for developing sophisticated applications. By leveraging the strengths of C++, developers can create efficient, maintainable, and high-performance code suitable for resource-constrained environments. As technology advances, the role of C++ in embedded systems is likely to become even more significant. Embracing learning and practice in this domain will prepare developers for the challenges ahead.
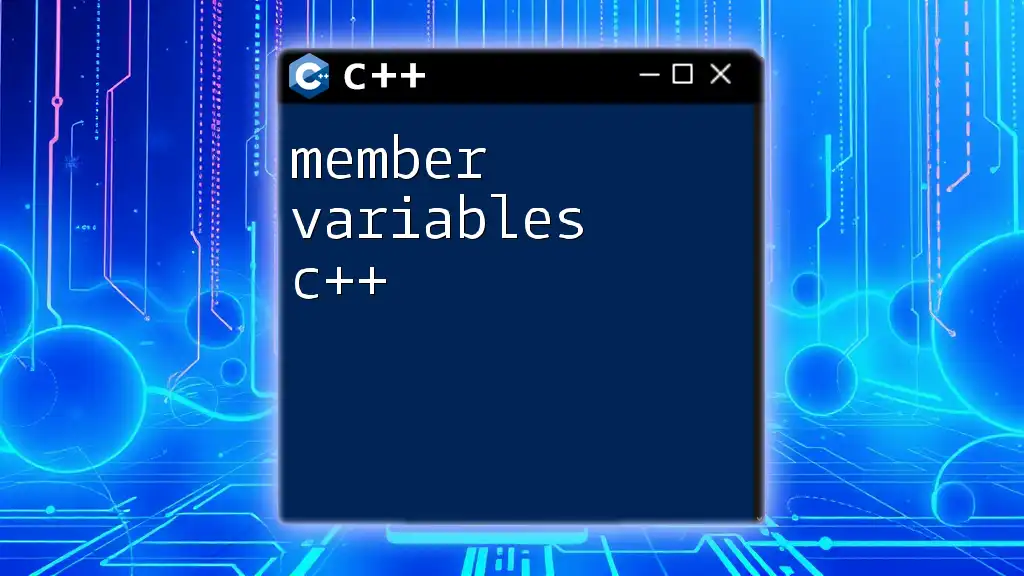
Additional Resources and References
For further exploration of embedded C++ programming, consider exploring additional resources such as specialized books, online courses, and community forums. Engaging with others in the field can provide valuable insights and further enhance your skills.