A vector of vectors in C++ is a dynamic two-dimensional array implemented using the `std::vector` class, allowing for flexible storage of data in rows and columns.
Here's a code snippet demonstrating this concept:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
for (const auto& row : matrix) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
Understanding Vector of Vectors
What is a Vector of Vector in C++?
A vector of vectors in C++ is a two-dimensional dynamic array that allows you to create a collection of vectors. Unlike a single-dimensional vector, which can store a linear sequence of elements, a vector of vectors provides a more flexible structure for managing tabular data or matrices. It is especially useful when the dimensions of the data are variable or when you want to model relationships in a way that requires a complex structure.
The power of using a C++ vector of vectors lies in its ability to dynamically grow and shrink in size based on your needs. This characteristic is fundamental when dealing with real-world data that is unpredictable in terms of volume or organization.
Visual Representation of a Vector of Vectors
To better understand this concept, think of a vector of vectors as a table or matrix. Each row in the table corresponds to a vector, and each element within that vector represents a column in that row. This two-dimensional representation makes it intuitive for managing and accessing the data structured in rows and columns.
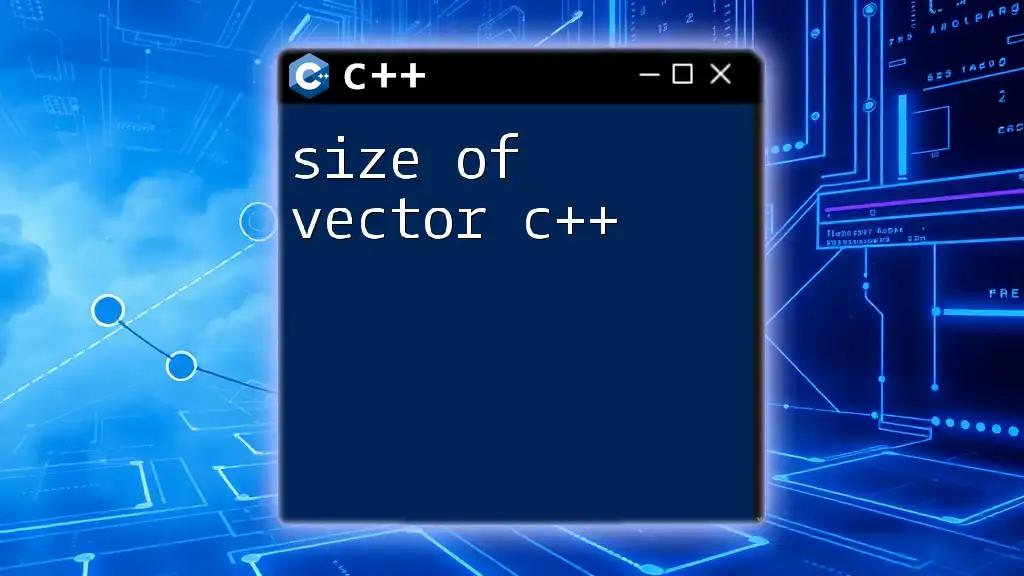
Creating and Initializing Vectors of Vectors
How to Declare a Vector of Vectors
To declare a vector of vectors in C++, you use the following syntax:
std::vector<std::vector<int>> matrix;
In this code, `matrix` is a 2D vector capable of storing integers. You can specify any data type based on your requirements.
Initializing with Values
You can also initialize a C++ vector of vectors with predetermined values. This allows for immediate population of the vector with data. For instance:
std::vector<std::vector<int>> matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Here, `matrix` represents a 3x3 table with specific integer values initialized. Each outer vector defines a row, and the inner vectors contain the corresponding elements of that row.
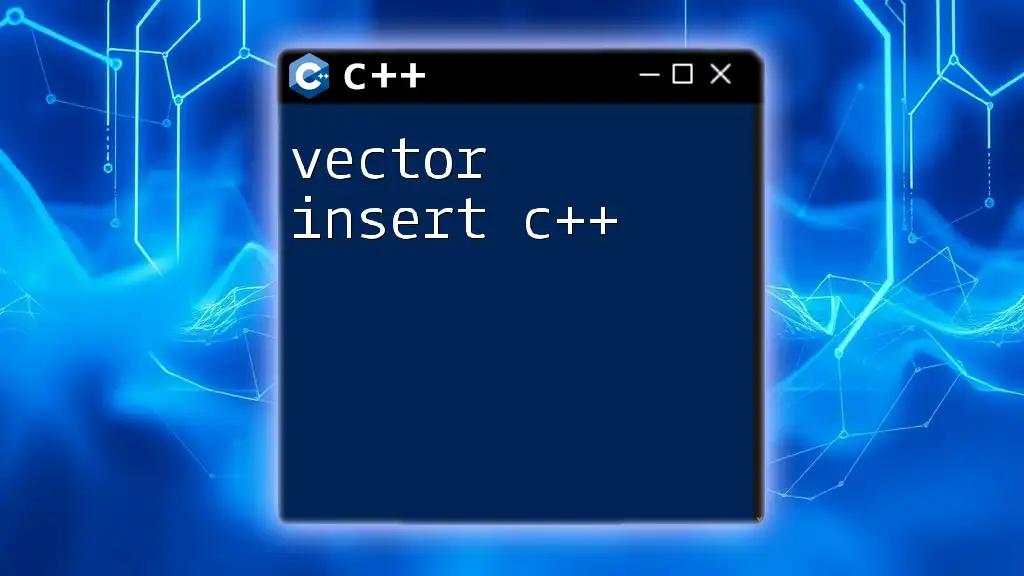
Accessing Elements in a Vector of Vectors
Indexing a Vector of Vectors
Accessing elements in a vector of vectors is straightforward. You can use two indices: the first for the row and the second for the column. For instance:
int value = matrix[1][2]; // Accessing the value at row 1, column 2
This retrieves the number `6` from our earlier defined `matrix`. Remember that in C++, indexing starts at `0`, meaning the first row is index `0`, the second is index `1`, and so forth.
Iterating Through a Vector of Vectors
Iterating through a vector of vectors is essential for accessing or manipulating each element contained within the structure.
Using Range-based for Loops
Range-based for loops provide a clean and readable way to iterate through the elements:
for(const auto& row : matrix) {
for(int val : row) {
std::cout << val << " ";
}
std::cout << std::endl;
}
This code snippet prints each element in `matrix`, separating them by spaces, and gives an output of each row in distinct lines.
Using Traditional For Loops
Alternatively, you can use traditional for loops, which allow for greater control over the iteration process:
for (size_t i = 0; i < matrix.size(); ++i) {
for (size_t j = 0; j < matrix[i].size(); ++j) {
std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
This method explicitly specifies the indices for accessing each element.
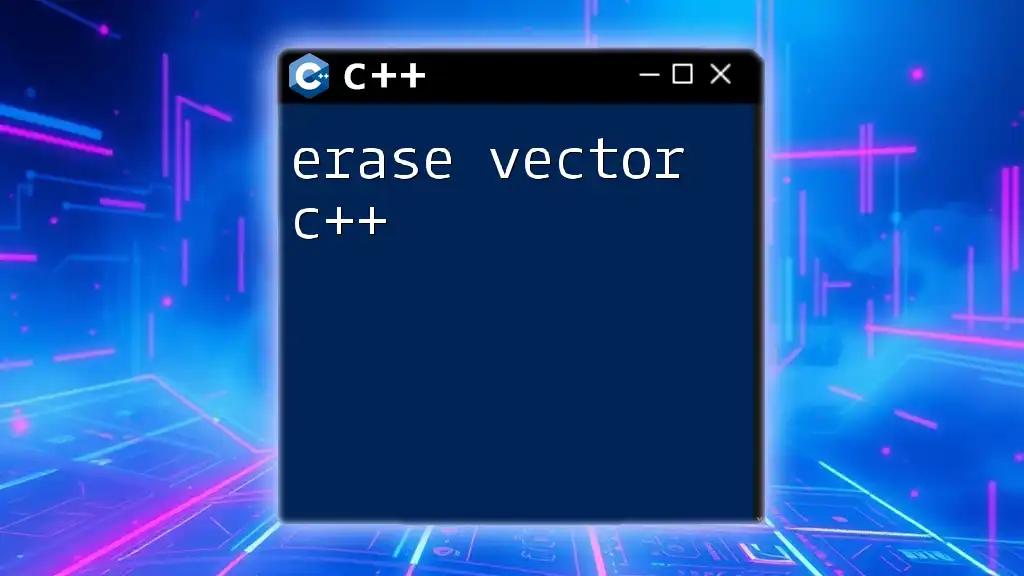
Modifying a Vector of Vectors
Adding Rows and Columns
Adding rows and columns to a vector of vectors is a significant advantage for managing dynamic data structures.
Adding New Rows
To add a new row, you can use the `push_back` method of the outer vector:
matrix.push_back({10, 11, 12});
This statement adds a new row with the values `10`, `11`, and `12` at the end of `matrix`.
Adding New Columns
Adding a new column requires iterating through each row and using `push_back` again:
for (auto& row : matrix) {
row.push_back(0); // Adding a new column with an initial value of 0
}
This adds an additional column initialized to `0` to each row of `matrix`.
Removing Rows and Columns
Managing the removal of rows and columns is just as crucial in a vector of vectors.
Removing a Row
To remove a specific row, you can use the `erase` function:
matrix.erase(matrix.begin() + 1); // Removes row at index 1
This line removes the second row of the `matrix`.
Removing a Column
Removing a column is more involved because it requires modifying every row:
for (auto& row : matrix) {
row.erase(row.begin() + 1); // Removes column at index 1
}
This will effectively remove the values in the second column from every row.
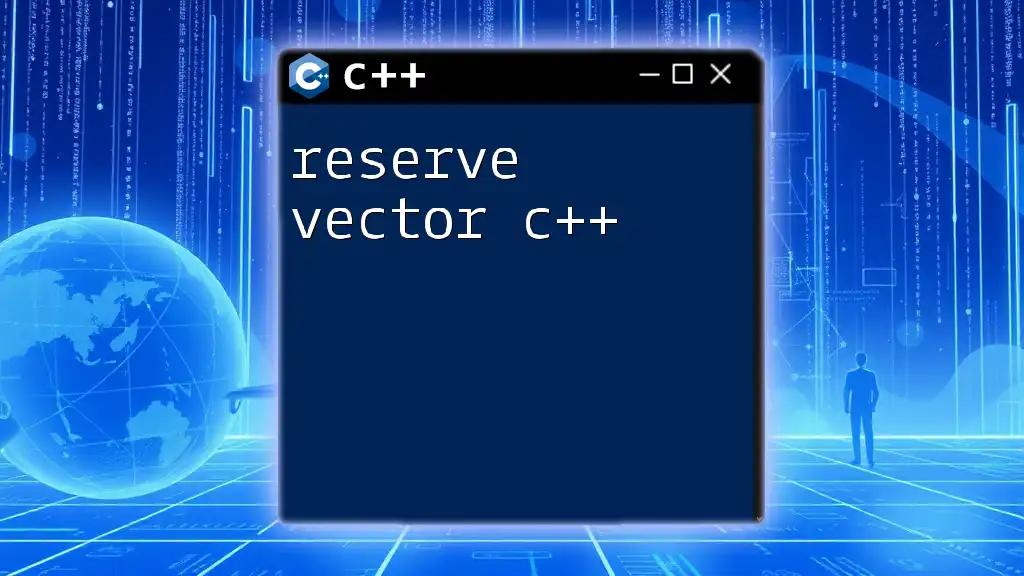
Use Cases for Vectors of Vectors
Applications in Real-World Scenarios
Vector of vectors is particularly useful in a variety of scenarios, including but not limited to:
- Graph representation: Using adjacency matrices to represent graphs.
- Image representation: Storing pixel values for an image in a 2D matrix structure.
- Game maps: Utilizing grids to map out spaces within video games.
These applications highlight the versatility of a C++ vector of vectors in complex data handling.
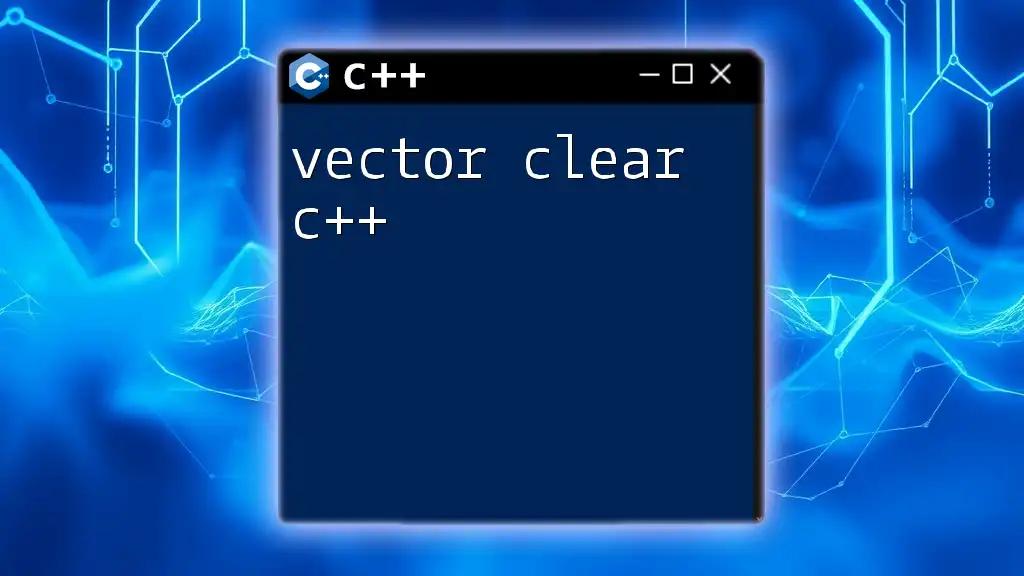
Best Practices for Using Vectors of Vectors
Performance Considerations
While vectors of vectors are flexible, they can introduce performance overhead due to multiple levels of pointers. It is essential to consider the cost of memory management when dealing with larger datasets. Generally, it is recommended to use this structure when the dimensions are expected to change significantly.
Avoiding Common Pitfalls
When working with vector of vectors, developers may often encounter issues related to out-of-bounds access or memory leaks if not managed properly. It is crucial to check the size of vectors before accessing elements to prevent run-time errors.
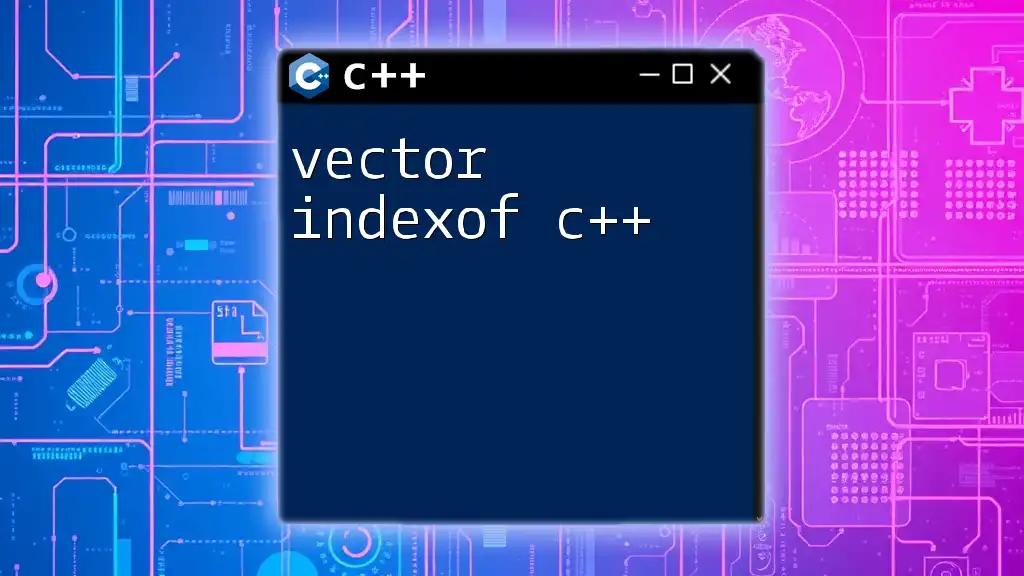
Conclusion
Understanding how to work with a vector of vector C++ is an invaluable skill for any C++ developer. By mastering this data structure, you can efficiently manage complex datasets that require a dynamic and flexible approach. Whether it’s through initialization, iteration, or modification, vectors of vectors give you the tools you need to handle data in a robust manner. We encourage you to practice implementing this powerful feature in your projects for a deeper understanding!