A vector of pointers in C++ allows you to create a dynamic array that holds pointers to objects, providing flexibility in managing object lifetimes and memory efficiently.
#include <iostream>
#include <vector>
int main() {
// Create a vector of pointers to integers
std::vector<int*> vec;
// Dynamically allocate and add integers to the vector
for (int i = 0; i < 5; ++i) {
vec.push_back(new int(i));
}
// Access and print the values
for (const auto& ptr : vec) {
std::cout << *ptr << " ";
}
// Clean up allocated memory
for (auto& ptr : vec) {
delete ptr;
}
return 0;
}
Understanding Vectors in C++
Understanding Vectors in C++
In C++, a vector is a dynamic array that can resize itself to accommodate new elements. Unlike traditional arrays, which have a fixed size, vectors provide greater flexibility and ease of use when managing collections of data. The key advantages of using vectors include automatic memory management, easy insertion and deletion of elements, and integration with C++'s Standard Template Library (STL).
What are Pointers in C++?
A pointer is a variable that stores the memory address of another variable. Pointers are essential for tasks such as dynamic memory allocation, passing large structures or classes to functions efficiently, and implementing complex data structures like linked lists.
To declare a pointer, you use the `*` operator, indicating that the variable is a pointer type. For example:
int* p; // p is a pointer to an integer
Pointers can be dereferenced to access or modify the data they point to, which is a fundamental aspect of pointer usage in C++.
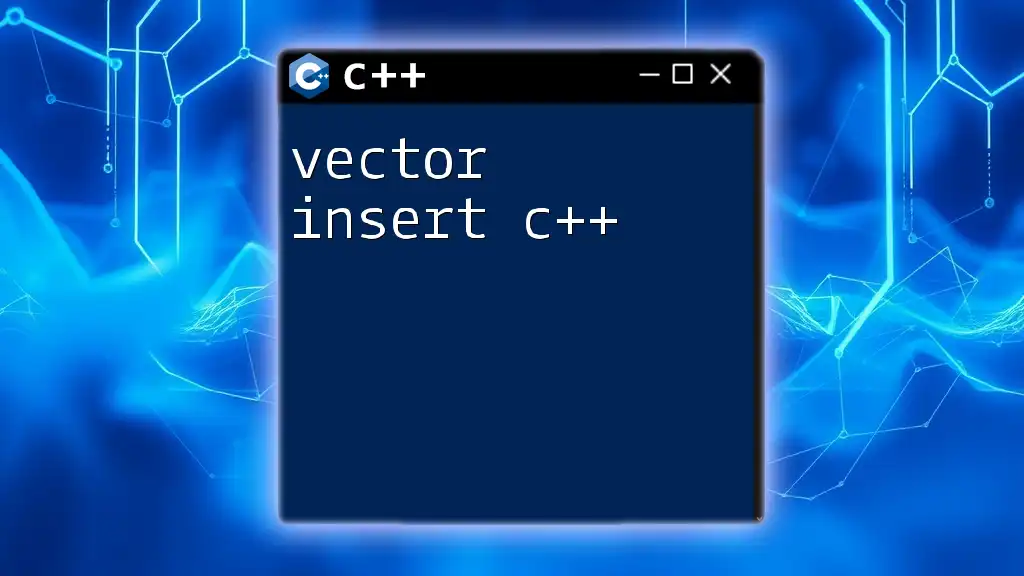
The Concept of a Vector of Pointers
What is a Vector of Pointers?
A vector of pointers in C++ is a vector that holds pointers to objects instead of the objects themselves. This allows you to store multiple pointers to dynamically allocated data, giving you a powerful mechanism for managing complex data structures.
For example, if you have a vector of integers but you want to store pointers to those integers, you can do so using a vector of pointers. This setup is especially beneficial when you want to manage resources efficiently or represent large datasets.
Benefits of Using a Vector of Pointers
Using a vector of pointers offers several key benefits:
- Memory Management: Vectors of pointers allow you to allocate memory dynamically for the elements, which can be particularly useful for large datasets.
- Flexibility and Scalability: Since you can insert and delete pointers dynamically, vectors of pointers provide a more adaptable structure when working with changing data sizes.
- Enhanced Performance: In certain scenarios, using pointers can enhance performance, such as when working with large objects, as it avoids unnecessary copying of data.
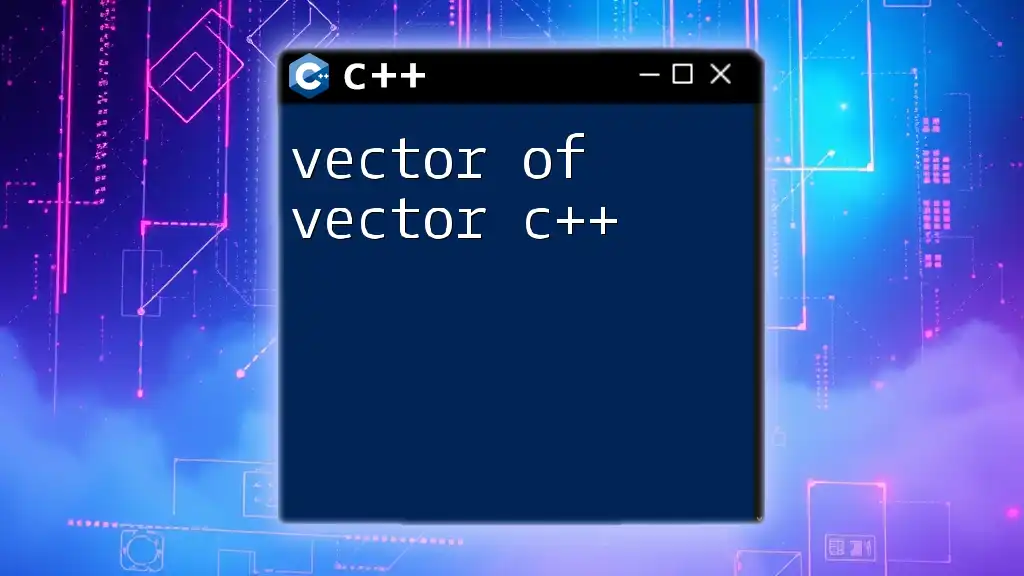
Working with Vectors of Pointers
Declaring a Vector of Pointers
To declare a vector of pointers, you can use the syntax:
#include <vector>
std::vector<int*> vec; // A vector that will hold pointers to integers
This line creates an empty vector that will eventually hold pointers to integer objects.
Adding Elements to a Vector of Pointers
To add elements to this vector, you often dynamically allocate memory using `new`. Here’s how you can do it:
vec.push_back(new int(10)); // Adds a pointer to a newly allocated integer with value 10
vec.push_back(new int(20)); // Adds another pointer to an integer with value 20
Each call to `new` allocates memory on the heap for an integer and returns a pointer to that memory, which you then store in the vector.
Accessing Elements in a Vector of Pointers
Accessing elements in a vector of pointers is straightforward. You can access the pointed data by dereferencing the pointer:
std::cout << *vec[0]; // Accesses and prints the value of the first pointer (prints 10)
*vec[1] = 30; // Modifies the value of the second pointer to 30
In this example, the first line retrieves the value stored at the memory location pointed to by the first pointer in the vector, while the second line assigns a new value to the integer at that location.
Iterating Over a Vector of Pointers
To iterate through a vector of pointers, you can use a range-based for loop:
for(auto ptr : vec) {
std::cout << *ptr << " "; // Prints the values of all integers pointed to
}
This loop allows you to access each pointer in the vector and dereference it to obtain the value it points to, effectively enabling you to perform operations on each element in one concise command.
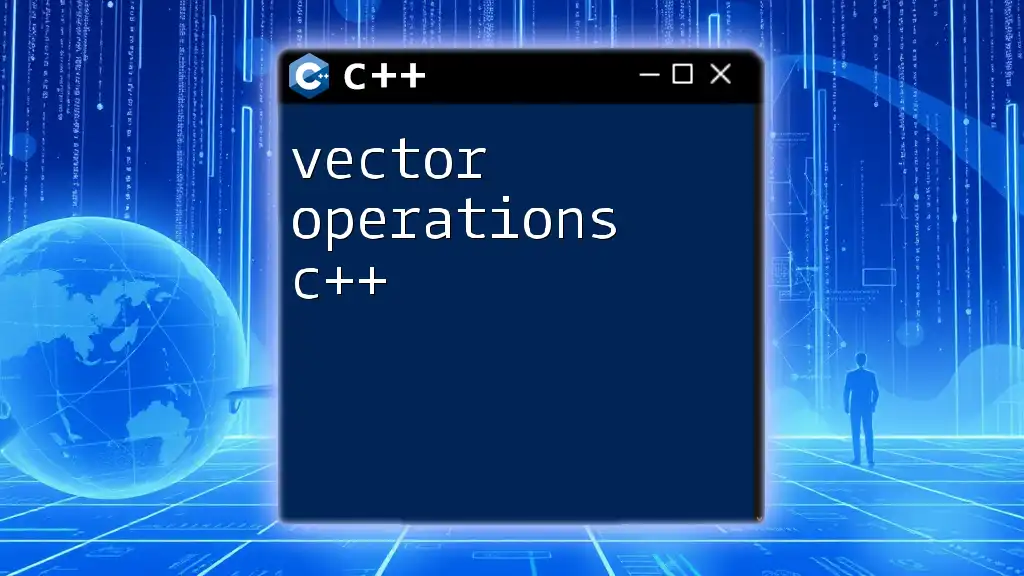
Memory Management in Vectors of Pointers
Dynamic Memory Allocation
Dynamic memory allocation is essential when using vectors of pointers. You must remember to release the dynamically allocated memory using `delete` to avoid memory leaks:
for(auto ptr : vec) {
delete ptr; // Frees the allocated memory for each integer
}
Failure to delete pointers will result in unused memory remaining allocated, which can slow down your program and eventually lead to exhaustion of available memory.
Best Practices for Memory Management
To ensure robust memory management:
- Always `delete` pointers when you're done using them to prevent memory leaks.
- After deleting a pointer, you should set it to `nullptr` to avoid dangling pointers:
ptr = nullptr; // Preventing dangling pointer access
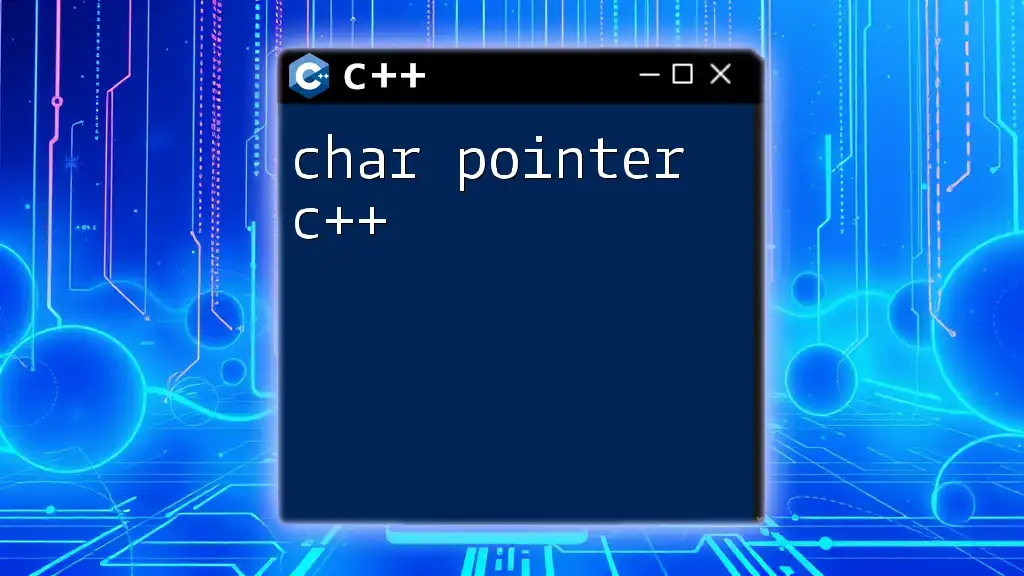
Common Use Cases for Vectors of Pointers
Storing Objects Using Vectors of Pointers
Vectors of pointers are especially useful for storing objects. For instance, you might have a class called `Student`:
class Student {
public:
std::string name;
int id;
Student(std::string n, int i) : name(n), id(i) {}
};
// Later, in your main function
std::vector<Student*> students;
students.push_back(new Student("Alice", 1));
students.push_back(new Student("Bob", 2));
This example demonstrates how you can create a vector that holds pointers to `Student` objects. Each student object is dynamically allocated, allowing you to efficiently manage their lifetimes.
Managing Large Datasets Efficiently
When working with large datasets, vectors of pointers can be advantageous. For example, if you're handling a large collection of images or large user-generated content, using pointers allows you to store and process them without creating large copies, saving both memory and time during execution. By maintaining a vector of these pointers, you can efficiently manage the data without overwhelming system resources.
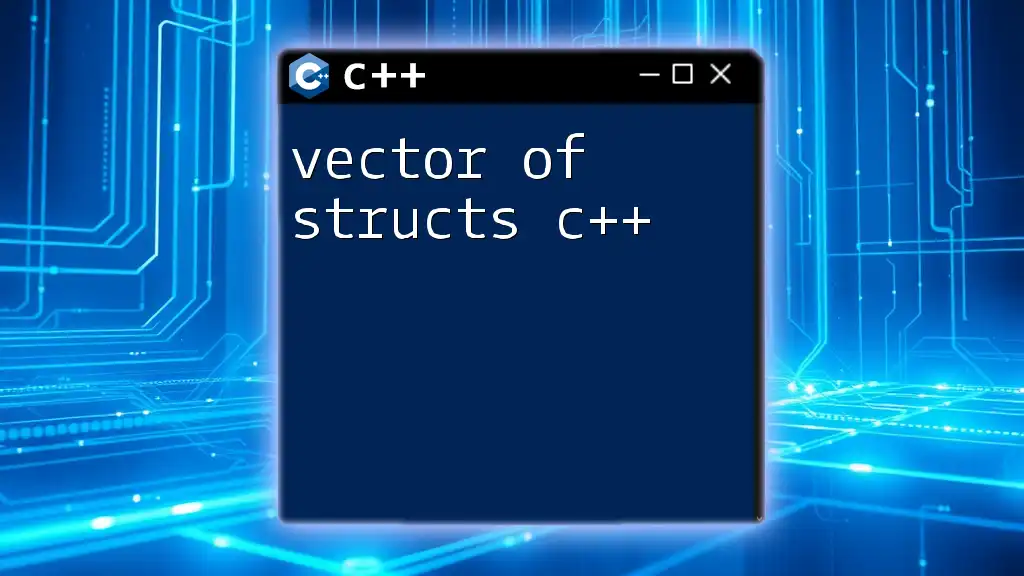
Conclusion
Recap of Key Points
Throughout this article, we explored the concept of a vector of pointers in C++, detailing the benefits of using this powerful construct. We discussed how vectors provide automatic memory management, flexibility, and efficient access to dynamically allocated data. You learned about declaring vectors of pointers, adding and accessing their elements, and the importance of proper memory management practices.
Encouragement for Practice
To fully grasp the concepts covered, it's important to practice coding with vectors of pointers. Experimentation will help solidify your understanding and skills. Whether by creating simple programs or tackling larger projects, consider focusing on implementing vectors of pointers to manage your data effectively.
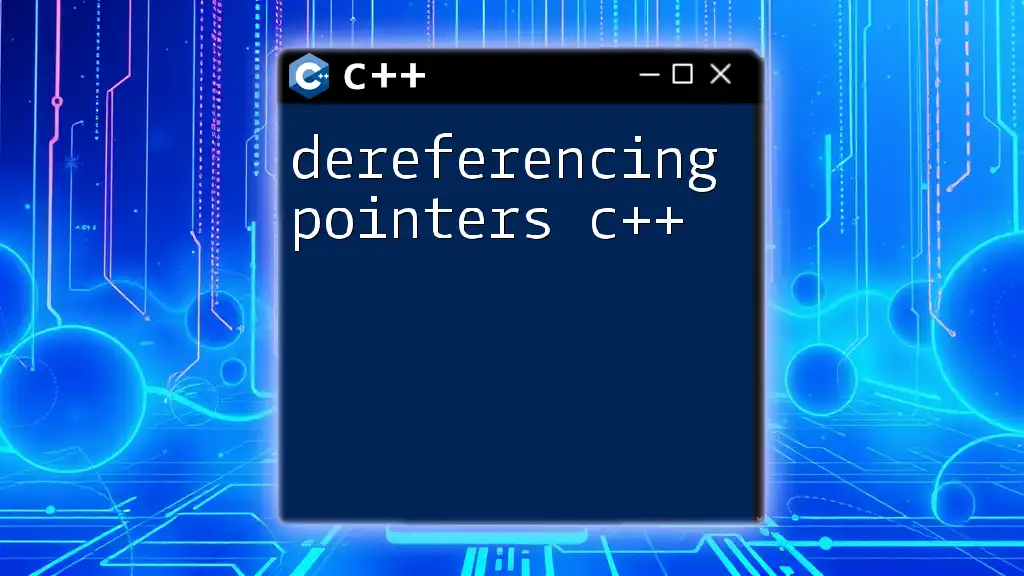
Additional Resources
Recommended Reading and Tutorials
For further learning, I recommend checking out the official C++ documentation, C++ reference books, and online courses dedicated to dynamic memory management and the Standard Template Library. Engaging with coding practice platforms will also provide valuable hands-on experience that complements your learning journey.