A pointer to a pointer in C++ is a variable that stores the address of another pointer, allowing for multiple levels of indirection.
#include <iostream>
int main() {
int value = 42; // Original integer
int* ptr = &value; // Pointer to the integer
int** ptrToPtr = &ptr; // Pointer to the pointer
std::cout << **ptrToPtr; // Accessing the original integer via pointer to pointer
return 0;
}
Understanding Pointers
What Are Pointers?
In C++, a pointer is a variable that stores a memory address, primarily the address of another variable. This allows for direct manipulation of memory, which can be very powerful for performance and control. Pointers serve as a conduit between programs and hardware, enabling dynamic memory allocation and efficient array manipulation.
Understanding Pointer Syntax
To declare a pointer, you use the asterisk (`*`) symbol, which denotes that the variable is a pointer type. For instance, if you want to declare a pointer to an integer, you would write:
int* ptr;
To initialize a pointer, you assign it the address of a variable using the address-of operator (`&`). Dereferencing a pointer (accessing the value stored at the address it points to) is done using the asterisk again:
int value = 10;
ptr = &value; // ptr now holds the address of value
int dereferencedValue = *ptr; // dereferencedValue will be 10
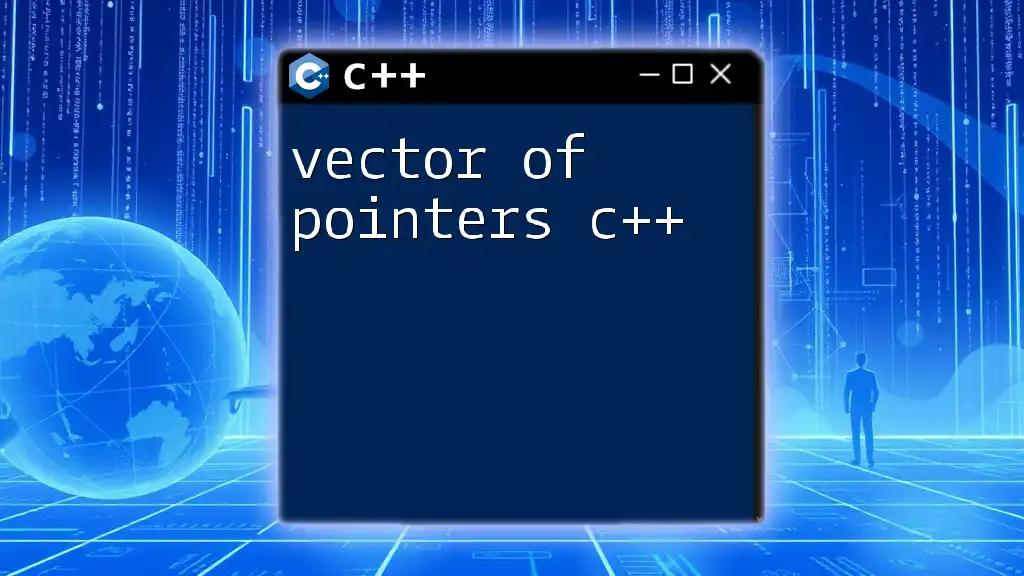
Diving Deeper: Pointer of a Pointer
What is a Pointer of a Pointer?
A pointer of a pointer is simply a pointer that points to another pointer. This concept expands the relationship between pointers, allowing you to manage pointers more flexibly. Why would you need such a structure? It can be particularly useful for multidimensional arrays and dynamic memory management strategies.
Syntax of Pointer of a Pointer
The syntax for a pointer to a pointer is relatively straightforward. For instance, to declare a pointer to a pointer of type `int`, you write:
int** ptrPtr;
Here, `ptrPtr` is a pointer that stores the address of another pointer, which in turn points to an integer.
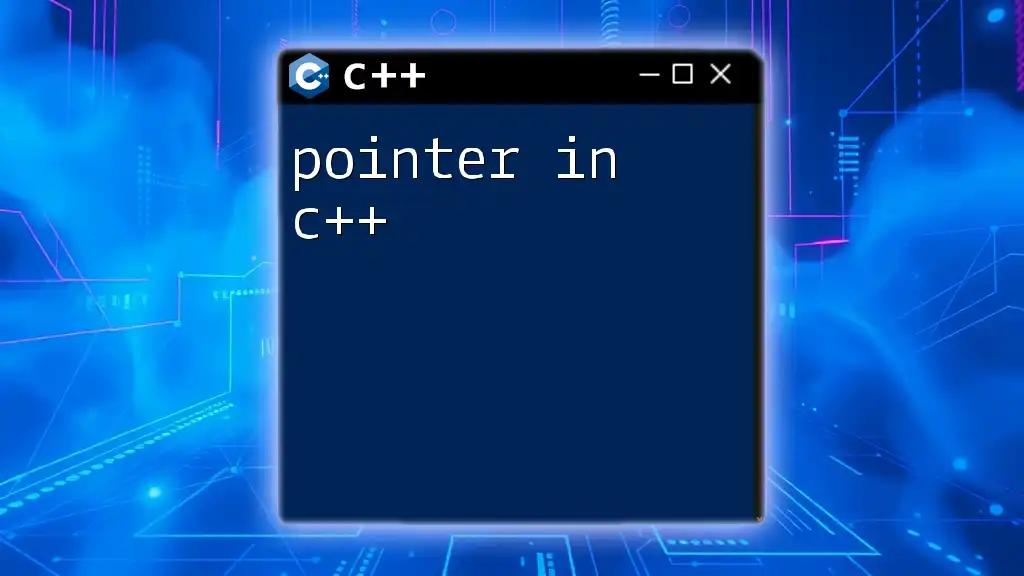
How Does a Pointer of a Pointer Work?
Memory Allocation and Addressing
Understanding how a pointer of a pointer operates requires a grasp of how memory is structured in C++. When you declare multiple levels of pointers, your program allocates separate memory for each pointer involved. The first level points to the address of the second level pointer, which in turn stores the address of the actual variable.
Accessing Values through Pointer of a Pointer
To access a value using a pointer of a pointer involves dereferencing twice. Here's a complete example:
int val = 5;
int* ptr = &val; // Pointer to an int
int** ptrPtr = &ptr; // Pointer to a pointer
// Accessing the value stored in 'val' through ptrPtr
int accessedValue = **ptrPtr; // accessedValue is now 5
Practical Use Cases
Dynamic Memory Allocation
Pointer of a pointer proves essential when dealing with dynamic memory allocation, especially for arrays of pointers. Here’s an example demonstrating how to dynamically allocate memory for a 2D array:
int** arr = new int*[3]; // Allocation for an array of 3 int pointers
for (int i = 0; i < 3; ++i) {
arr[i] = new int[5]; // Each int pointer points to an array of 5 ints
}
This code snippet effectively creates a 3x5 two-dimensional array where each row can be independently accessed through pointers.
Managing Arrays of Strings
Using a pointer of a pointer is particularly useful in handling two-dimensional character arrays (arrays of strings). The implementation looks like this:
const char** names = new const char*[3];
names[0] = "Alice";
names[1] = "Bob";
names[2] = "Charlie";
Here, `names` is a pointer that points to an array of string pointers. Each pointer in the array can be used to access a string.

Memory Management with Pointer of a Pointer
Proper Deallocation
When using pointers of pointers, it's crucial to free the allocated memory properly to avoid memory leaks. Incorrect memory management can lead to program instability and unexpected behaviors.
Example of Safe Cleanup
For the previously defined `arr`, cleaning up the allocated memory is essential:
for (int i = 0; i < 3; ++i) {
delete[] arr[i]; // Deallocate each row
}
delete[] arr; // Deallocate the array of pointers
By following this approach, you ensure that each level of dynamically allocated memory is appropriately freed.

Common Mistakes to Avoid
Dereferencing Errors
One common mistake with pointers of pointers is dereferencing a null or uninitialized pointer. Always ensure that a pointer has been assigned a valid address before dereferencing it, or you may encounter program crashes.
Memory Leaks
C++ does not provide automatic garbage collection, which means forgetting to delete dynamically allocated memory can create memory leaks. With pointer of pointers, keeping track of allocation and deallocation is vital to prevent such issues.
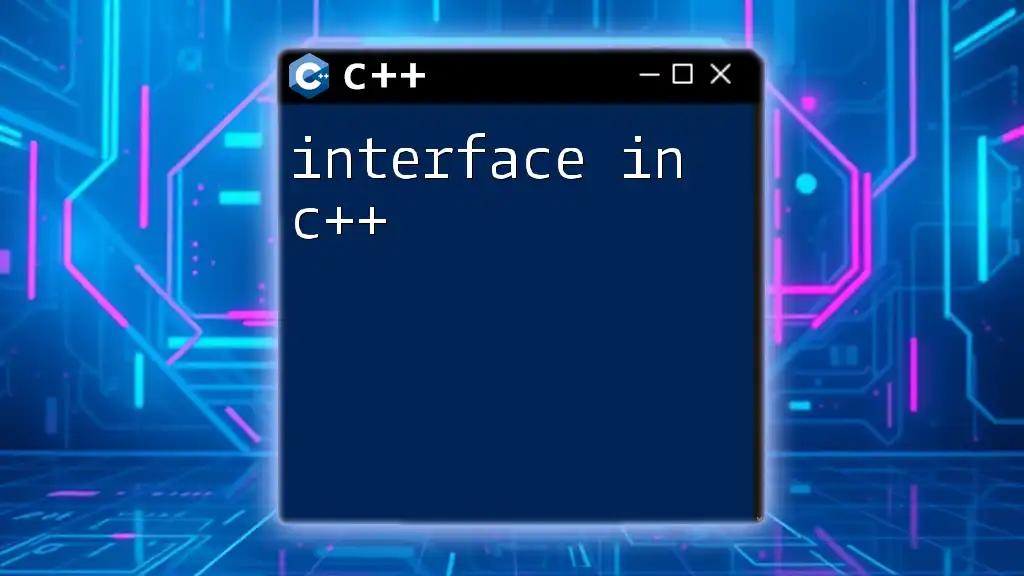
Conclusion
Recap of Key Takeaways
Understanding pointer of a pointer in C++ enhances your ability to manage complex data structures and dynamic memory allocation. They are crucial for advanced programming tasks, especially when dealing with multidimensional arrays and linked data structures.
Additional Resources
To deepen your understanding of pointers and memory management in C++, consider exploring the following resources:
- C++ programming books like "Effective C++" by Scott Meyers
- Online courses focusing on advanced C++ concepts
- Debugging tools to manage pointer-related issues effectively

FAQs about Pointer of a Pointer in C++
What is the difference between a single pointer and a pointer of a pointer?
A single pointer points to a data type, while a pointer of a pointer points to another pointer. This additional level of indirection allows you to manage more complex data structures and offers more flexibility.
When should I use a pointer of a pointer?
You should consider using a pointer of a pointer when working with multidimensional arrays or when you need to manage dynamic memory for a complex data structure, such as lists of lists.
Are there any performance implications?
While the use of pointers of pointers adds complexity, they do not inherently slow down a program. However, improper management of these pointers, like excessive dynamic allocation, could negatively impact performance. Always ensure your memory handling is efficient.
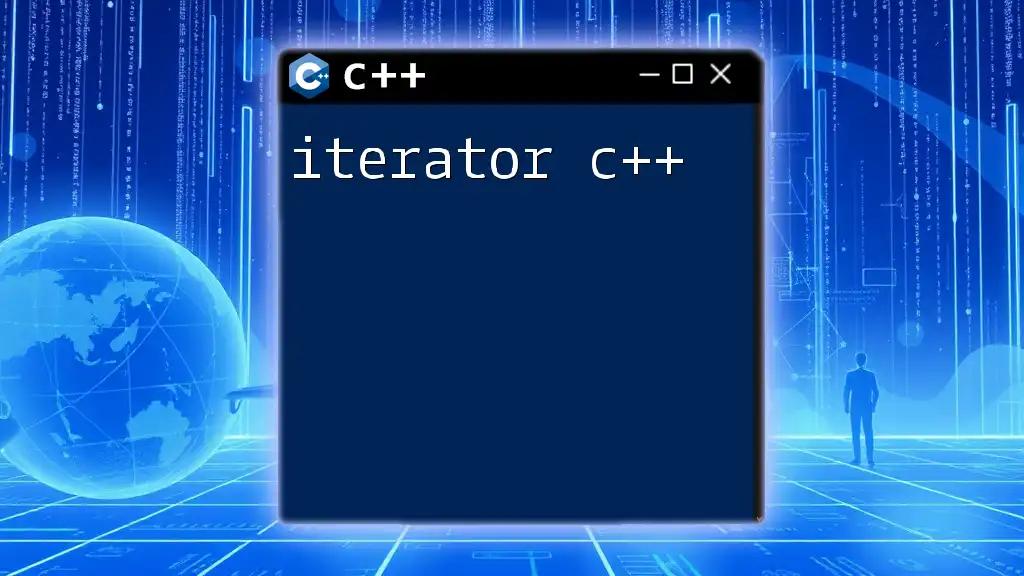
Call to Action
Now it's time to practice what you've learned! Dive into coding exercises with pointers of pointers and explore the intricacies of C++. For more in-depth studies or structured learning, check out our courses and materials designed specifically for aspiring C++ programmers.