To calculate the square root in C++, you can use the `sqrt()` function from the `<cmath>` library.
Here's a simple example:
#include <iostream>
#include <cmath>
int main() {
double number = 16.0;
double result = sqrt(number);
std::cout << "Square root of " << number << " is " << result << std::endl;
return 0;
}
What is Square Root?
Definition of Square Root
The square root of a number \( x \) is a value \( y \) such that \( y^2 = x \). In simpler terms, the square root answers the question: "What number multiplied by itself gives me \( x \)?"
Real-world applications of square root include calculating the length of the sides of geometric shapes, analyzing statistical data, and solving quadratic equations. Understanding square roots is essential for programmers who work in fields like gaming, physics simulations, or finance.
The Importance of Square Root in C++
In programming, square roots are frequently used in algorithms for computational geometry, physics calculations, and financial models. Whether you are calculating Euclidean distances or implementing advanced graphics transformations, knowing how to square root in C++ is crucial.
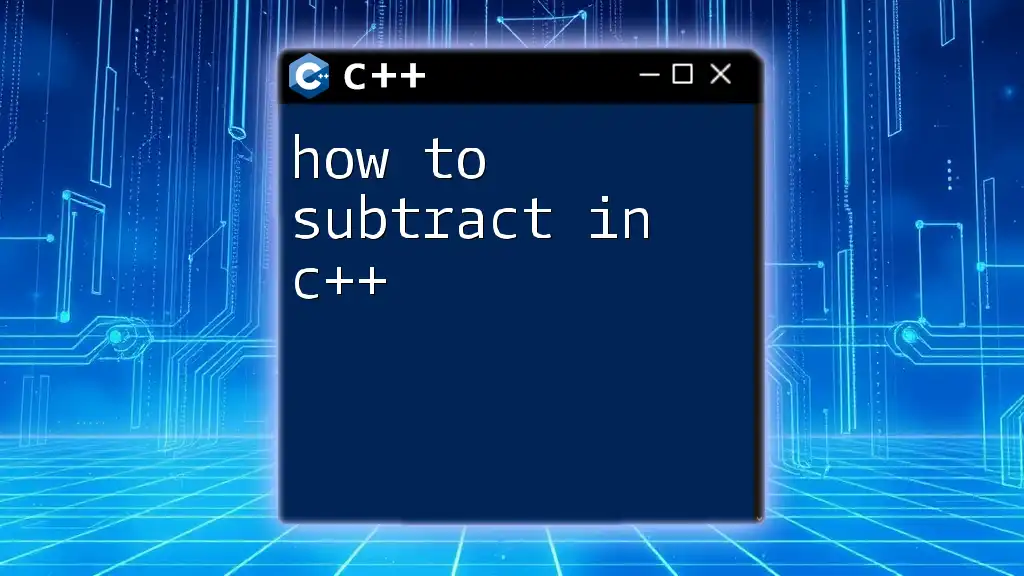
Understanding Square Root Function in C++
Introduction to the `sqrt` Function
C++ provides a built-in function `sqrt` for calculating the square root of a number. To utilize this function, you need to include the header file `<cmath>` at the beginning of your program.
Syntax of `sqrt`
The basic syntax of the `sqrt` function is as follows:
double sqrt(double x);
- Parameters: `x` - This is the number for which the square root is to be calculated. It must be a non-negative value.
- Return Type: The function returns a `double` that represents the square root of the specified number.
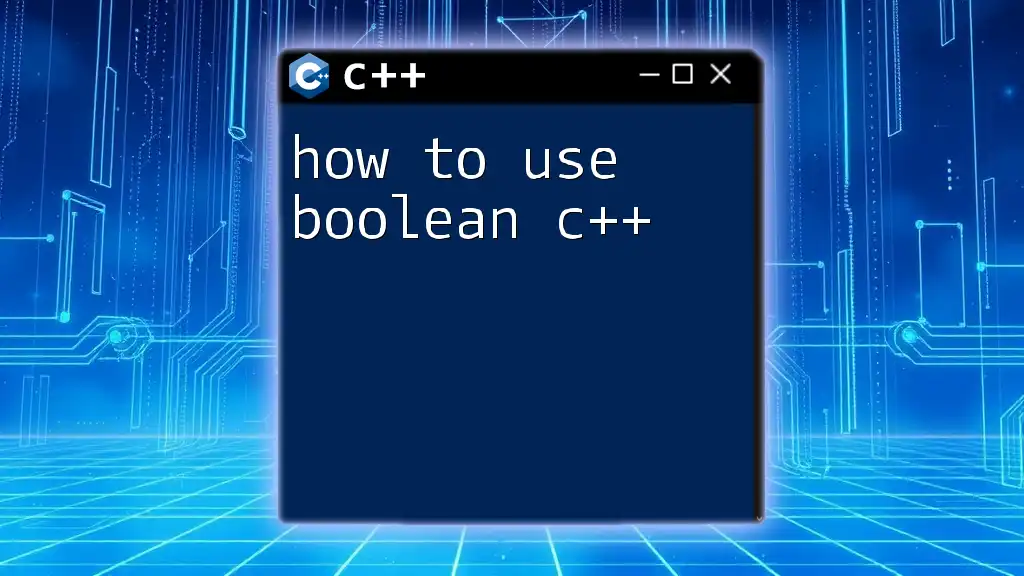
How to Do Square Root in C++
Step-by-Step Implementation
Step 1: Include the Required Header To access the `sqrt` function, you'll need to include the `<cmath>` library along with the `<iostream>` library for input and output operations.
#include <iostream>
#include <cmath>
Step 2: Get User Input You will need to take user input so that your program can calculate the square root of any arbitrary number provided by the user.
double number;
std::cout << "Enter a number: ";
std::cin >> number;
Step 3: Calculate Square Root Invoke the `sqrt` function to compute the square root of the input number.
double result = sqrt(number);
Step 4: Display the Result Finally, you will output the result to the user to inform them of the computed square root.
std::cout << "The square root of " << number << " is " << result << std::endl;
Complete Example Code
Here is a full example of a program that calculates and displays the square root:
#include <iostream>
#include <cmath>
int main() {
double number;
std::cout << "Enter a number: ";
std::cin >> number;
if (number < 0) {
std::cout << "Square root of negative number is not defined in real numbers." << std::endl;
} else {
double result = sqrt(number);
std::cout << "The square root of " << number << " is " << result << std::endl;
}
return 0;
}
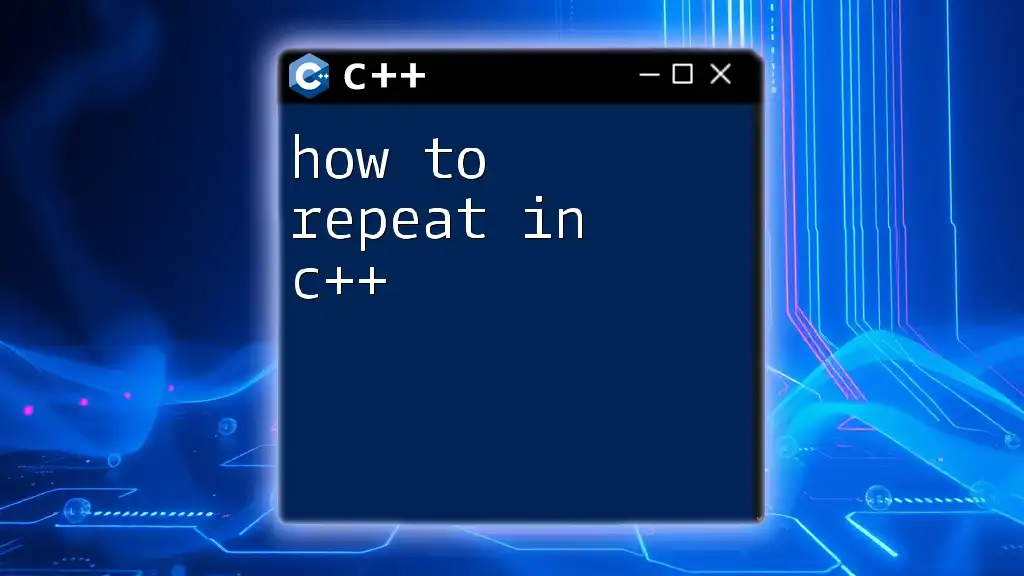
Common Mistakes when Using Square Root in C++
Input of Negative Numbers
One common mistake developers make is attempting to calculate the square root of a negative number. The `sqrt` function will return a NaN (Not a Number) value in such cases, as square roots of negative numbers are not defined in the realm of real numbers. Always validate inputs to ensure they are non-negative before performing the calculation.
Floating-Point Precision
When using floating-point numbers, precision can be an issue. It's crucial to be aware that operations involving `float` and `double` can lead to precision errors. Consider using `double` wherever high precision is needed, especially when dealing with mathematical functions like square roots.
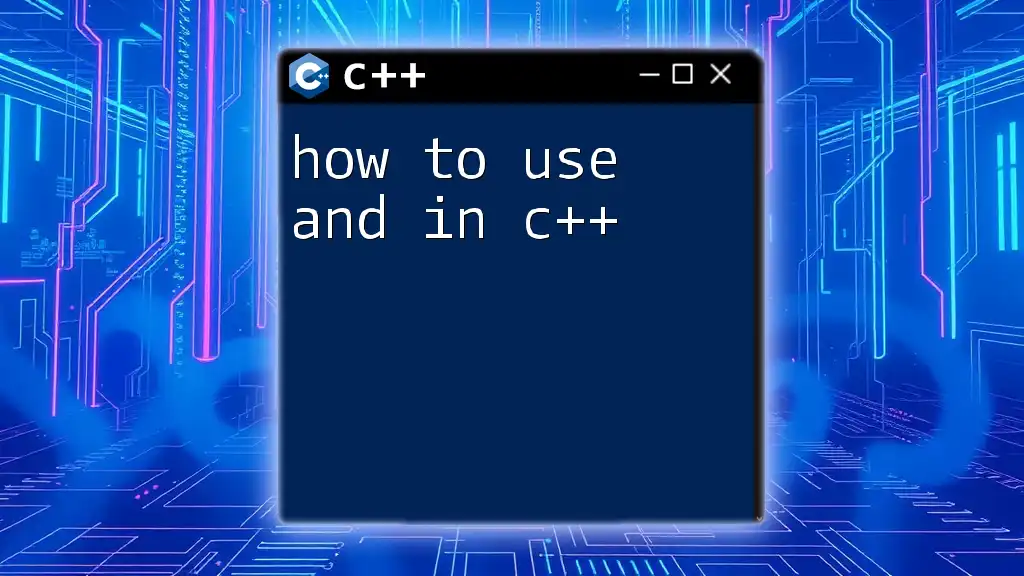
Additional Tips for Using Square Root in C++
Performance Considerations
Ensure that square root calculations are done judiciously, especially within loops or performance-critical applications. If you are performing frequent calculations, look into algorithmic optimizations to avoid redundant computations. You might also use approximate methods for very large datasets where exact precision is not crucial.
Alternative Methods
While the built-in `sqrt` function is efficient, you can also implement your own algorithm for finding square roots, such as Newton's method. This iterative method can provide an understanding of the underlying mathematics and allows for learning opportunities in algorithm design.
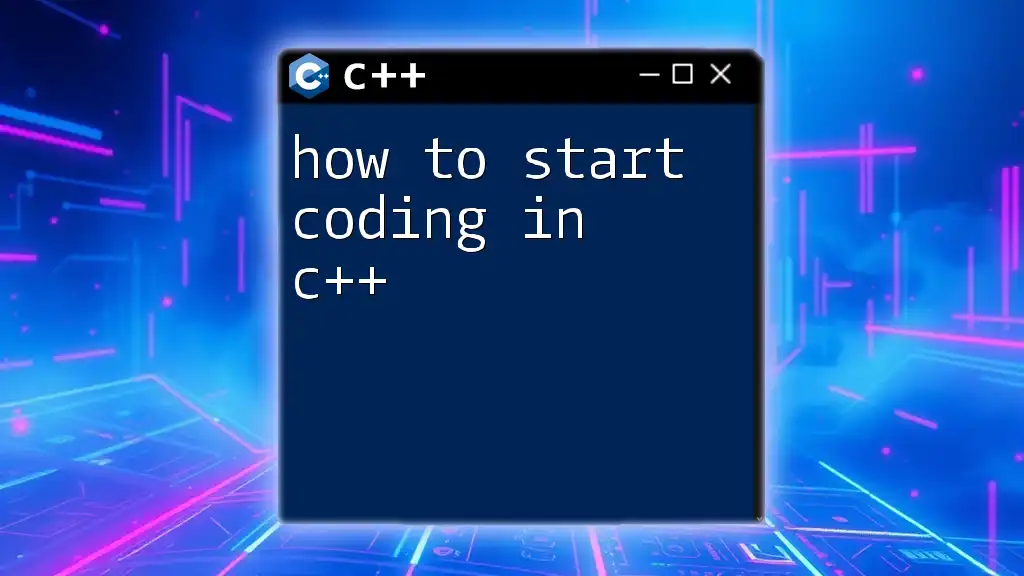
Conclusion
Recap of Key Points
In this article, we've covered how to square root in C++ effectively by utilizing the built-in `sqrt` function through a series of straightforward steps. We discussed the importance of validating inputs and maintaining precision while performing calculations.
Call to Action
Now that you have the tools needed to work with square roots in C++, why not implement this functionality in your own projects? Delve deeper into C++ and explore more mathematical functions to expand your programming capabilities!