To reverse an array in C++ using a for loop, you can swap the elements from the beginning and the end until you reach the middle of the array.
#include <iostream>
using namespace std;
void reverseArray(int arr[], int n) {
for (int i = 0; i < n / 2; i++) {
swap(arr[i], arr[n - i - 1]);
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
reverseArray(arr, n);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array in C++ is a collection of elements of the same type placed in contiguous memory locations. It allows you to store multiple values under a single name, making it easier to manage and manipulate data. Arrays are fundamental data structures that help in various programming tasks like sorting, searching, and, as we will discuss, reversing.
Declaring and Initializing Arrays in C++
To use an array in C++, you must first declare it by specifying the type of elements it will hold and its size. Here’s how you can declare and initialize an array:
int arr[5] = {1, 2, 3, 4, 5};
In this example, `arr` can hold five integers, and its elements are initialized to 1, 2, 3, 4, and 5, respectively. Accessing elements of an array is done using indices, starting at 0. For instance, `arr[0]` would refer to the first element, which is 1.

Methods to Reverse an Array
Several techniques exist for reversing an array in C++. While you might come across methods like using the Standard Template Library (STL) or recursive approaches, this article will focus specifically on using a for loop. This method is beneficial because it gives you more control over the process and is relatively straightforward.

How to Reverse an Array in C++ Using a For Loop
Step-by-Step Explanation
Reversing an array involves swapping elements from the start of the array with elements from the end. The for loop allows us to iterate through the first half of the array while simultaneously swapping with the corresponding elements from the second half.
Basic Algorithm to Reverse an Array
The basic algorithm can be summarized as follows:
- Start a for loop that runs from the beginning of the array to the halfway point.
- For each iteration, swap the current element with its corresponding element from the other end of the array.
Example: Reversing an Array
Here's an implementation to illustrate how to reverse an array using a for loop in C++:
#include <iostream>
using namespace std;
void reverseArray(int arr[], int n) {
for (int i = 0; i < n / 2; ++i) {
swap(arr[i], arr[n - i - 1]);
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
reverseArray(arr, n);
cout << "Reversed array: ";
for(int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
Explanation of the Code
- Function `reverseArray`: This function takes an array and its size as inputs. It uses a for loop to iterate through the first half of the array.
- Using `swap()`: The `swap` function from the `<algorithm>` header is used to exchange the values of the elements. For example, `swap(arr[i], arr[n - i - 1])` swaps the first element with the last, the second with the second-last, and so on.
- Iterating through the Array: The loop condition `i < n / 2` ensures that each element is swapped only once, avoiding redundancy.
Additional Considerations
Handling Edge Cases
When working with arrays, it’s important to consider edge cases:
- Empty Arrays: An empty array should not cause any errors; the function should simply exit.
- Single Element Arrays: A single-element array remains unchanged when reversed.
Example code to handle these cases could look like this:
if (n <= 1) {
return; // No need to reverse
}
Time Complexity Analysis
The time complexity of reversing an array using a for loop is O(n), where n is the number of elements in the array. This is efficient because each element is visited exactly once. This efficiency is illustrated by the big-O notation.
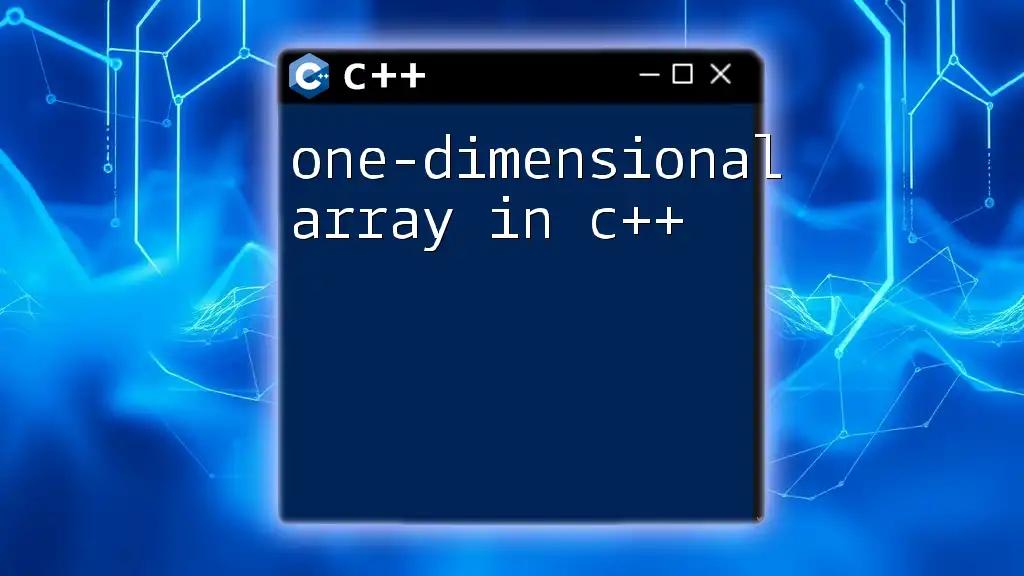
Common Issues and Debugging Tips
Common Mistakes When Reversing an Array
- Off-by-One Errors: Ensure that your loop correctly iterates to the halfway mark to avoid unnecessary operations.
- Pointer Mismanagement: Always check that your indices do not go out of bounds, which can cause runtime errors.
How to Troubleshoot Your Code
- Insert Print Statements: This helps track the values of your arrays at different stages.
- Use a Debugger: C++ IDEs typically come with debugging tools that can step you through your code.

Alternative Methods to Reverse an Array
Using Standard Template Library (STL)
C++ provides a convenient way to reverse arrays through the STL. The `std::reverse` function can reverse any array or collection easily. Here’s how to use it:
#include <algorithm>
std::reverse(arr, arr + n);
This line of code will reverse the entire array without needing to write your swap logic.
Using Recursion to Reverse an Array
If you prefer a recursive approach, you can create a function that reverses an array using the call stack:
void recursiveReverse(int arr[], int start, int end) {
if (start >= end) return; // Base case
swap(arr[start], arr[end]);
recursiveReverse(arr, start + 1, end - 1);
}
This method systematically swaps the elements towards the center of the array.
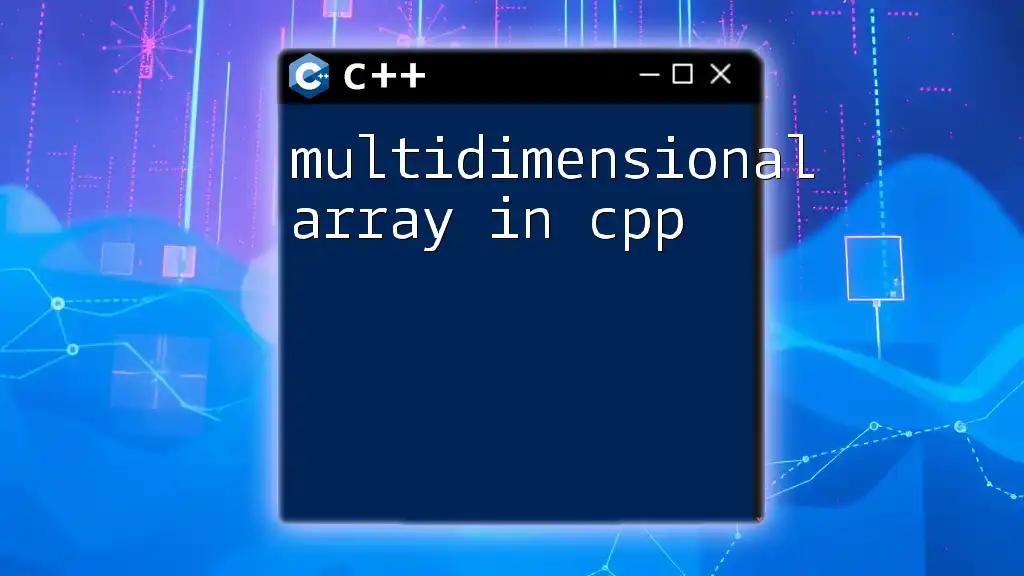
Summary
In this article, we explored how to reverse an array in C++ using a for loop. This method allows for a straightforward and efficient way of switching elements to achieve the desired result. Understanding the fundamentals of arrays and manipulation techniques will significantly enhance your coding capabilities in C++.

Call to Action
Now that you understand how to reverse an array using a for loop, it's time to put this knowledge into practice. Try writing your own examples and manipulating arrays in various ways. For more insights into array manipulations and other C++ concepts, consider exploring further through our services!

Conclusion
Reversing an array is a crucial skill in C++ programming. Mastering array manipulations not only helps in understanding data structures but also enhances your problem-solving abilities as a programmer. Don't hesitate to experiment with different methods and share your experiences with others!