To create a dynamic array in C++, you can use the `new` keyword to allocate memory for the array on the heap, allowing for a flexible array size determined at runtime.
Here's an example code snippet:
#include <iostream>
int main() {
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
int* dynamicArray = new int[size]; // creating dynamic array
// Example usage: filling the array
for (int i = 0; i < size; ++i) {
dynamicArray[i] = i + 1; // assign values
}
// Print the array
for (int i = 0; i < size; ++i) {
std::cout << dynamicArray[i] << " ";
}
delete[] dynamicArray; // freeing the allocated memory
return 0;
}
Understanding Dynamic Arrays
What is a Dynamic Array?
A dynamic array is a data structure that can grow or shrink in size during the runtime of a program. Unlike static arrays, which have a fixed size determined at compile time, dynamic arrays allow you to allocate memory as needed, providing greater flexibility in handling data.
Characteristics of dynamic arrays include:
- Variable Size: You can allocate and deallocate memory as required.
- Heap Memory Allocation: Memory is allocated from the heap, allowing the array to exceed the limits of the stack.
Advantages of dynamic arrays are:
- They can adapt to changing data needs, accommodating additional elements without requiring a predefined size.
- You can work efficiently with large datasets that might not fit into static arrays.
However, some disadvantages to consider include:
- Complexity in memory management, including the need to manually handle memory allocation and deallocation.
- Potential fragmentation of the heap memory, leading to inefficient memory use.
When to Use Dynamic Arrays
Dynamic arrays are particularly useful in scenarios where the amount of data is not known in advance. Common use cases include:
- User-Driven Input: Situations where the size of the array is determined by user input, such as collecting data from various sources.
- Data Processing Applications: Programs that handle datasets of varying sizes, like dynamic lists of records in a database or image processing applications that vary based on input.
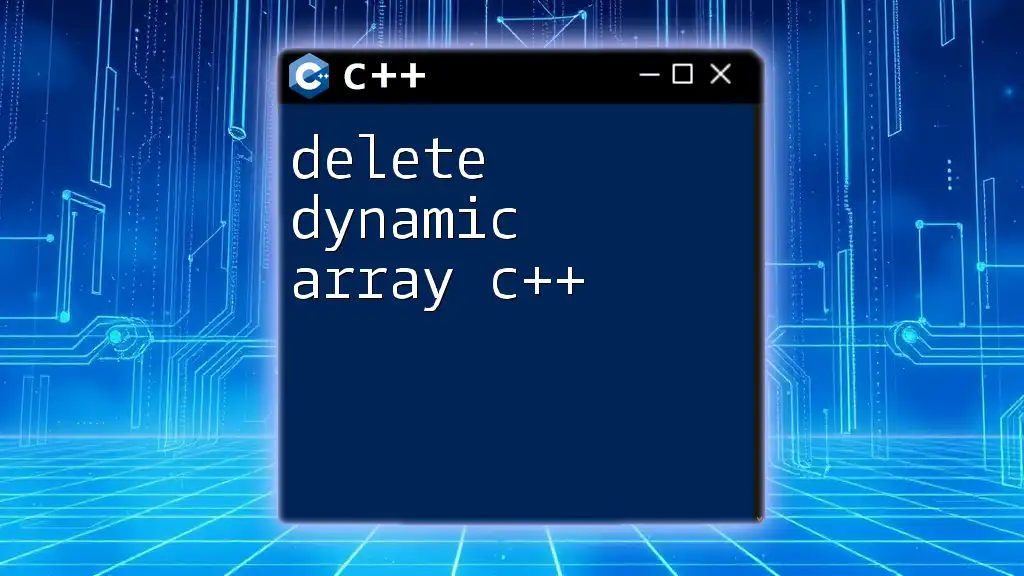
How to Create a Dynamic Array in C++
Memory Management in C++
Proper memory management is crucial in C++ programming. While local variables are allocated on the stack, which automatically frees up memory when they go out of scope, dynamic arrays utilize heap memory, allowing for more control.
Understanding the difference between stack and heap memory is essential, as heap memory must be manually managed using operators like `new` and `delete`. Failure to do so can lead to memory leaks, where allocated memory is never freed.
Using the `new` Operator
The `new` operator in C++ is pivotal in creating dynamic arrays. It allocates memory on the heap and returns a pointer to the first element of the array.
Syntax:
data_type* pointer_name = new data_type[array_size];
For instance, to create a dynamic array of integers, you would write:
int* dynamicArray = new int[size];
Example: Creating a Dynamic Integer Array
Here’s a step-by-step implementation of how to create a dynamic integer array:
#include <iostream>
using namespace std;
int main() {
int size;
cout << "Enter the size of the array: ";
cin >> size;
int* dynamicArray = new int[size]; // Dynamic array creation
for (int i = 0; i < size; ++i) {
dynamicArray[i] = i * 10; // Initializing elements
}
// Displaying the array
for (int i = 0; i < size; ++i) {
cout << dynamicArray[i] << " ";
}
// Memory cleanup
delete[] dynamicArray;
return 0;
}
Explanation of the code:
- The program prompts the user for the size of the array.
- It allocates the array dynamically using `new`.
- A loop initializes the elements of the array.
- Another loop displays the content of the array.
- Finally, memory is freed using `delete[]` to prevent memory leaks.
Allocating Memory for Various Data Types
Dynamic arrays can be created for various data types, such as `double`, `char`, or even complex data structures. The method remains the same:
double* doubleArray = new double[size];
char* charArray = new char[size];
Initialization techniques may vary slightly based on requirements, especially for custom structures.
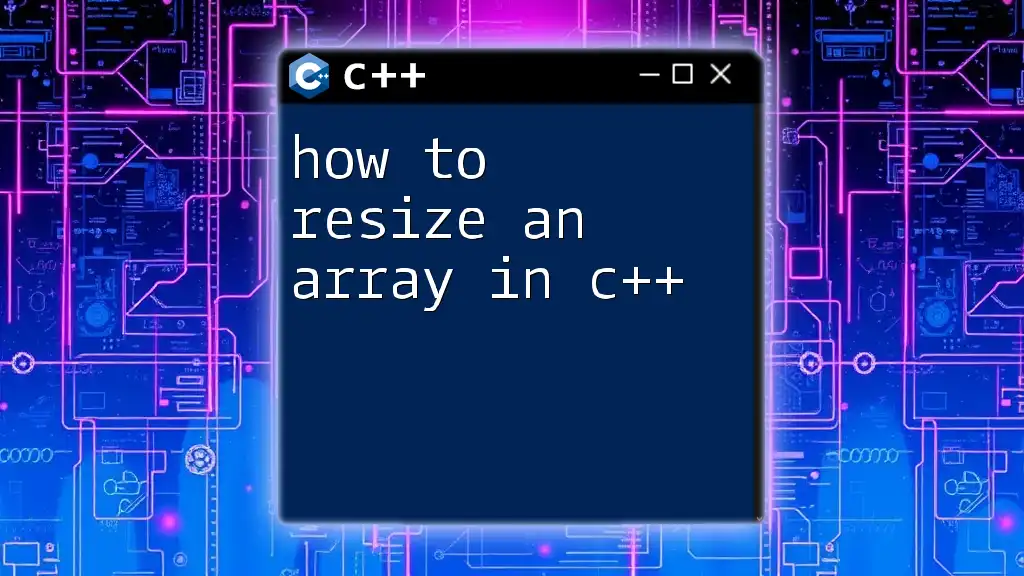
Resizing Dynamic Arrays
Why Resizing is Necessary
In practice, you often encounter situations where the initial size of a dynamic array becomes insufficient. Resizing is essential to adapt to new data, which static arrays cannot accommodate.
Techniques for Resizing
Manual Resizing
You can manually resize a dynamic array by allocating a new array with a larger size, copying the existing elements, and deleting the old array. Here’s a sample approach:
int* newArray = new int[newSize];
for (int i = 0; i < oldSize; ++i) {
newArray[i] = dynamicArray[i];
}
delete[] dynamicArray;
dynamicArray = newArray;
Explanation of the process:
- Allocate a new array with the desired size.
- Copy existing elements from the old array to the new one.
- Use `delete[]` to free the old memory, then point your original pointer to the new array.
Using the Standard Template Library (STL)
If manual resizing seems cumbersome, consider using `std::vector` from the Standard Template Library. `std::vector` simplifies array handling by automatically managing size and capacity. You can push back elements without worrying about memory management.
Here’s how you declare a dynamic vector:
#include <vector>
std::vector<int> dynamicVector;
dynamicVector.push_back(10); // Adding elements dynamically
When employing `std::vector`, C++ handles memory allocation and deallocation for you, reducing the complexity of your code.
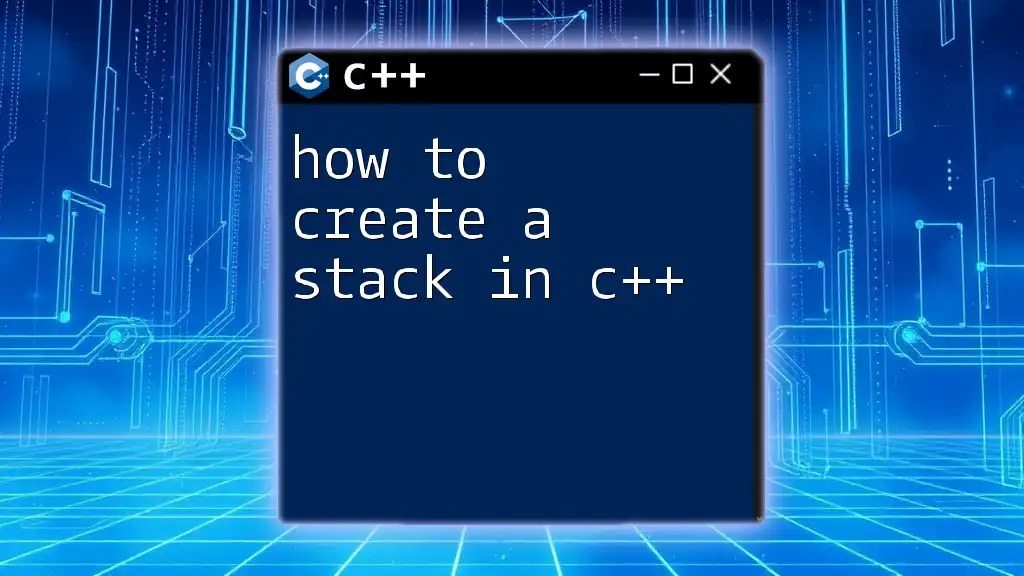
Common Mistakes and Troubleshooting
Memory Leaks
Memory leaks occur when dynamically allocated memory is not released, causing your program to consume more memory over time. Avoid common pitfalls by:
- Always using `delete[]` for dynamically allocated arrays.
- Keeping track of all dynamic memory allocations in your program to ensure they are properly freed.
Working with Pointers
Dynamic arrays involve pointers, and you must be careful with pointer arithmetic. Here are some safety measures:
- Always initialize pointers before use to avoid dereferencing null or unallocated pointers.
- Be cautious when accessing elements using indexes, ensuring you stay within the allocated memory bounds.
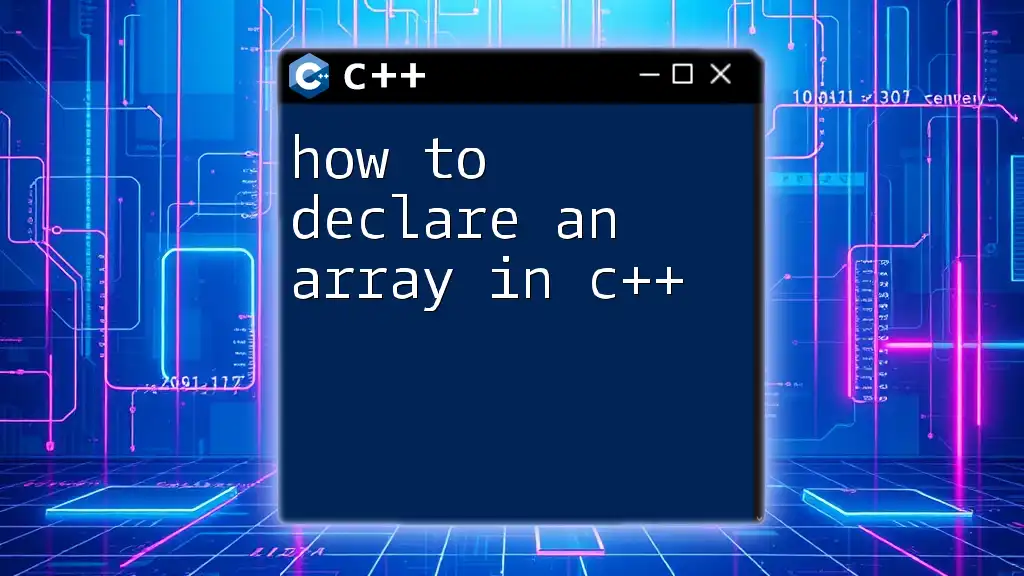
Conclusion
Creating a dynamic array in C++ offers flexibility needed in managing varying data sizes effectively. While the basic concepts of memory management may initially seem daunting, mastering dynamic arrays is invaluable for C++ programming.
With this knowledge, you're now prepared to use dynamic arrays confidently and can explore further features in C++, such as `std::vector`, to simplify your code. Happy coding!
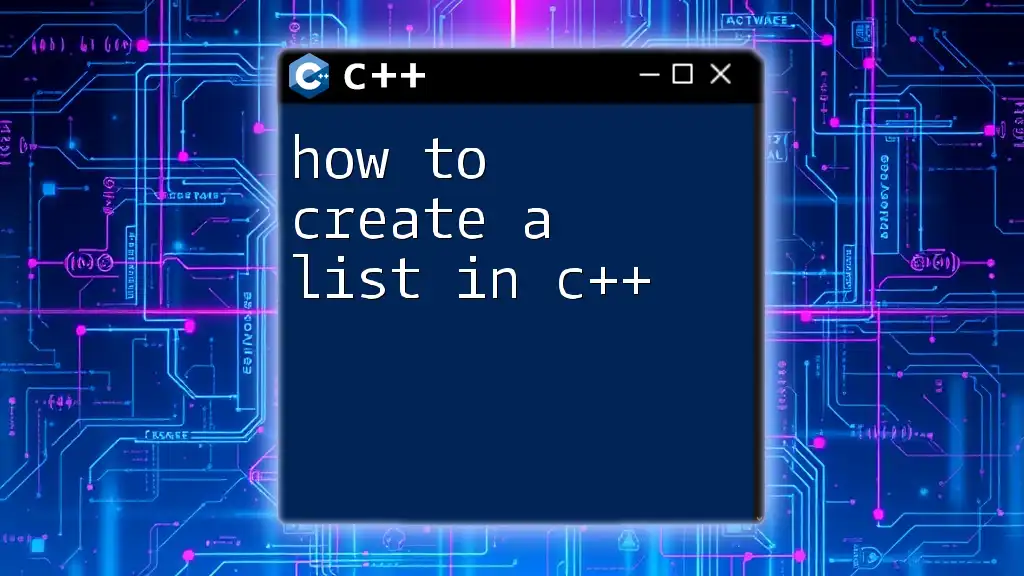
Additional Resources
For deeper insight into dynamic arrays and memory management approaches in C++, consider exploring the official C++ documentation or enrolling in specialized online courses.