To print a variable in C++, you can use the `cout` stream in combination with the insertion operator (`<<`) to output the variable to the console.
#include <iostream>
using namespace std;
int main() {
int myVariable = 10;
cout << myVariable << endl;
return 0;
}
Understanding Variables in C++
What is a Variable?
A variable in programming acts as a storage container for data. It allows developers to store values that may change over time, facilitating easier data management and manipulation in software applications. Variables can represent a wide range of data types, such as numbers, characters, and even more complex structures like arrays or classes.
Types of Variables in C++
C++ primarily distinguishes between two types of variables:
-
Primitive Types: These include basic data types such as `int`, `float`, `char`, and `double`, which are built into C++.
-
User-defined Types: These are data types created by the user, including structures (`struct`), classes (`class`), and arrays. They allow for more complex data representations that align with the needs of the programmer.

Basics of Printing in C++
The Standard Output Stream
In C++, the `std::cout` object is used to send output to the console. It is part of the C++ standard library, specifically included in the `<iostream>` header file. This standard output stream is crucial for visually inspecting variable values and debugging programs.
Syntax for Printing Variables
The fundamental syntax for using `std::cout` involves using the stream insertion operator (`<<`) followed by the variable you wish to print. Here's a simple example:
#include <iostream>
int main() {
int myVar = 10;
std::cout << myVar;
return 0;
}
In this code snippet, the variable `myVar`, which holds the integer value `10`, is printed to the standard output.

Printing Different Data Types
Printing Integer Variables
When you need to print an integer variable, you can do so directly with `std::cout`. For instance:
int number = 42;
std::cout << "The number is: " << number << std::endl;
In this example, `42` will be output to the console alongside the preceding message.
Printing Floating-Point Variables
To print floating-point variables, the process remains the same. Here’s how it looks:
float pi = 3.14;
std::cout << "The value of pi is: " << pi << std::endl;
This line will output `The value of pi is: 3.14`, showcasing how you can output variables of type `float`.
Printing Character Variables
Printing characters is performed similarly:
char letter = 'A';
std::cout << "The first letter is: " << letter << std::endl;
This code will display `The first letter is: A` in the console.
Printing String Variables
For printing strings, you can use `std::string` from the `<string>` header:
#include <string>
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
The output here will simply read `Hello, World!`.
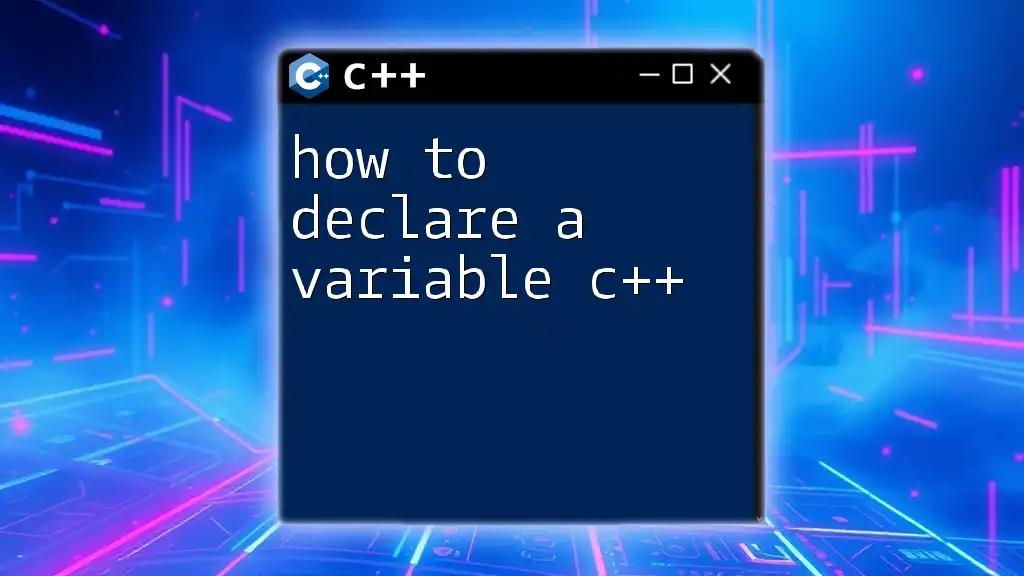
Formatting Output
Using `std::endl`
Utilizing `std::endl` adds a new line and flushes the output buffer efficiently. This is vital when you need your output to appear on separate lines for clarity:
std::cout << "Line 1" << std::endl;
std::cout << "Line 2" << std::endl;
Using Escape Sequences
Escape sequences can improve formatting significantly. Here are a couple of common ones:
- `\n` for a new line.
- `\t` for a tab space.
Example of formatting with escape sequences:
std::cout << "Value:\t" << number << "\n\n";
This will produce output that is neatly organized and easier to read.

Combining Multiple Variables in Output
Using the Stream Insertion Operator
You can combine multiple variables in a single output line using the stream insertion operator:
std::cout << "Coordinates: (" << x << ", " << y << ")" << std::endl;
This example is effective for displaying data such as x and y coordinates in a single print statement.
Using String Concatenation
Another approach is to concatenate strings. C++ allows for this through the `+` operator:
std::string name = "John";
std::cout << "Hello, " + name + "!" << std::endl;
This line will output `Hello, John!`, effectively combining the greeting message with the variable.

Best Practices for Printing Variables
Choosing Meaningful Messages
Meaningful messages help make your output clear and intuitive. Instead of simply outputting variable values, provide context. For example, instead of:
std::cout << myVar;
Consider enhancing it:
std::cout << "The current value of myVar is: " << myVar << std::endl;
Minimizing Clutter in Output
It’s essential to maintain readability in your output. Avoid overwhelming the console with too much information. If outputting several variables is necessary, consider wrapping related outputs in functions to simplify your main logic.

Debugging with Printed Variables
Using Print Statements for Debugging
Print statements are an invaluable debugging tool. They allow you to track the state of variables at different stages of your program execution. For instance:
std::cout << "Debug: myVar = " << myVar << std::endl;
This approach can help identify any issues by checking the value of `myVar` during runtime.
Identifying Common Mistakes
To avoid common output-related bugs:
- Ensure the correct variable type is printed. For example, printing an integer variable as a string may confuse the output.
- Watch for errors like forgetting to include `#include <iostream>`, which would lead to compilation errors.

Conclusion
Mastering how to print a variable in C++ is crucial for effective programming, debugging, and data representation. By familiarizing yourself with various data types, formatting options, and best practices, you can significantly enhance your ability to create clear and meaningful console outputs. Whether you're a beginner or experienced programmer, honing this skill is essential for effective C++ programming.

Further Learning Resources
Recommended Books and Tutorials
Consider exploring comprehensive C++ programming books or online tutorials that offer deeper insights and additional examples.
Online Coding Platforms for Practice
Engage with platforms like LeetCode or Codecademy to practice and refine your skills in using print statements and other C++ functionalities actively.
By continuing your learning journey, you'll become increasingly proficient in outputting variables and mastering C++.