To write to a file in C++, you can use the `ofstream` class from the `<fstream>` library, which allows you to create a file and write data to it using the `<<` operator. Here's a code snippet demonstrating this:
#include <fstream>
int main() {
std::ofstream outputFile("example.txt");
outputFile << "Hello, world!" << std::endl;
outputFile.close();
return 0;
}
Understanding File Streams in C++
What Are File Streams?
File streams in C++ are objects that handle input and output operations associated with files. In file handling, we primarily use three types of streams:
- `ifstream`: Used for input file streams, allowing us to read data from files.
- `ofstream`: Useful for output file streams, allowing us to write data to files.
- `fstream`: A combination of both, enabling reading from and writing to files.
How to Include Necessary Libraries
To work with file streams, we need to include the `<fstream>` header in our C++ program. This library provides the necessary functionality for file operations. Including libraries is fundamental in C++, as they allow access to various functionalities:
#include <fstream>
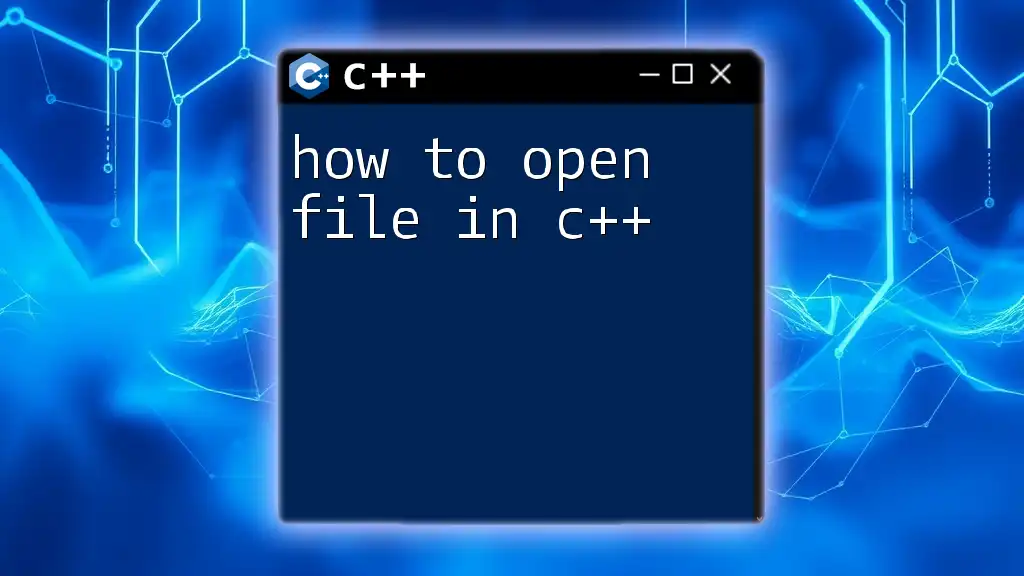
Writing to a File in C++
Opening a File
Before writing data to a file, it is essential to open it. When we open a file, we can specify different modes, such as:
- `ios::out`: This mode is used for output operations. If the file already exists, it will be overwritten.
- `ios::app`: This mode is for appending data to an existing file without overwriting its current content.
Here’s how you can open a file:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream outFile("example.txt", ios::out);
// Check if the file is open
if (!outFile) {
cerr << "Failed to open the file." << endl;
return 1;
}
// File operations go here
outFile.close();
return 0;
}
Example of Opening a File
In the example above, we try to open a file named `example.txt`. If the file is successfully opened, we can perform our write operations; otherwise, an error message will be displayed.
Writing Data to a File
Using the Insertion Operator (<<)
The simplest way to write data to a file is by using the insertion operator (`<<`). This method works similarly to how we output information to the console, but instead, we direct that output to our file.
Example of writing data to a file:
outFile << "Hello, World!" << endl;
outFile << "This is a line of text." << endl;
In the above code snippet, we demonstrate writing two lines to the file. Each line is followed by `endl`, which adds a newline character, ensuring our text appears on separate lines.
Using Member Functions
For more advanced file handling, we may use the `.write()` member function. This method is particularly useful for writing binary data.
Example of writing binary data to a file:
char data[] = "Binary Data";
outFile.write(data, sizeof(data));
In this example, we write a character array to the file, demonstrating the ability to handle raw binary data if needed.
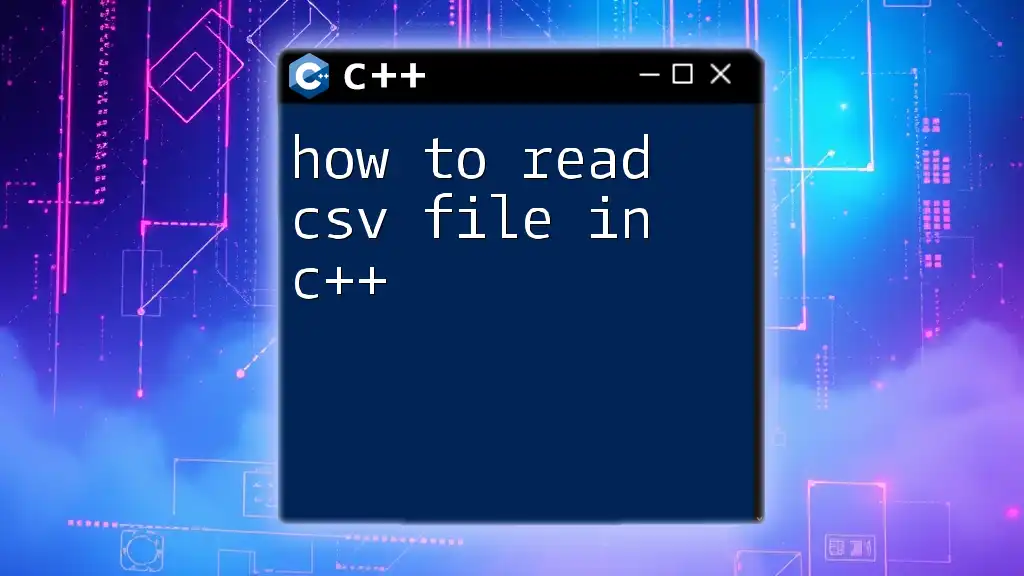
Appending to a File
How to Append Data
When we need to add new information to an existing file, we open it in append mode using `ios::app`. This approach ensures that current data remains intact while new data is added at the end.
ofstream outFile("example.txt", ios::app);
outFile << "This text will be appended." << endl;
outFile.close();
In this case, if we run the program multiple times, the appended line will keep getting added at the bottom of `example.txt`, preserving prior contents.
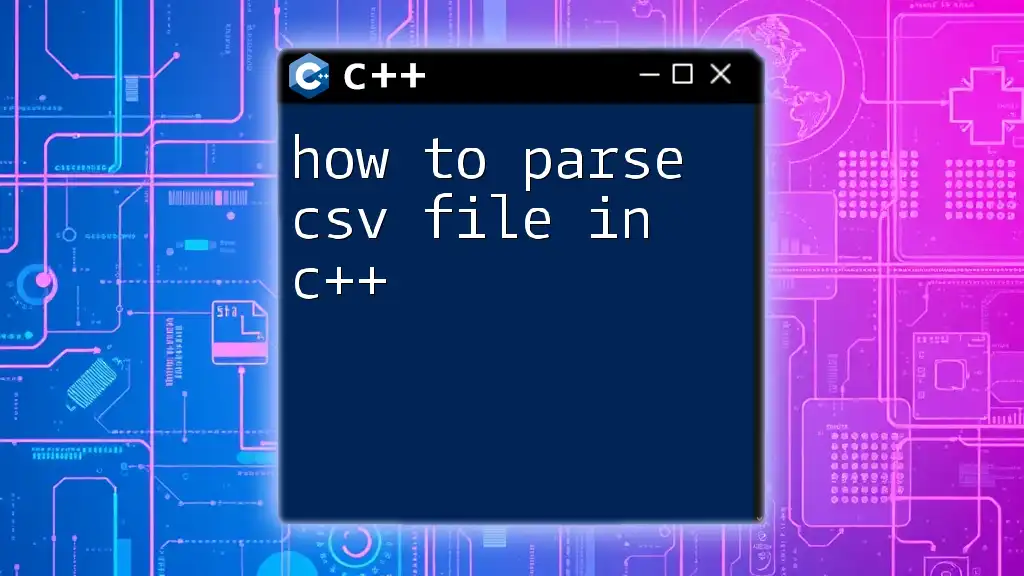
Closing a File
Why Closing a File Is Important
Once we finish our file operations, it is crucial to close the file. This action releases system resources and ensures that all data is correctly written and saved.
Neglecting to close a file may lead to resource leaks or even cause data loss. Always remember to close your file after completing your operations.
Code Example for Closing a File
After operations, simply call the `close()` method:
outFile.close();
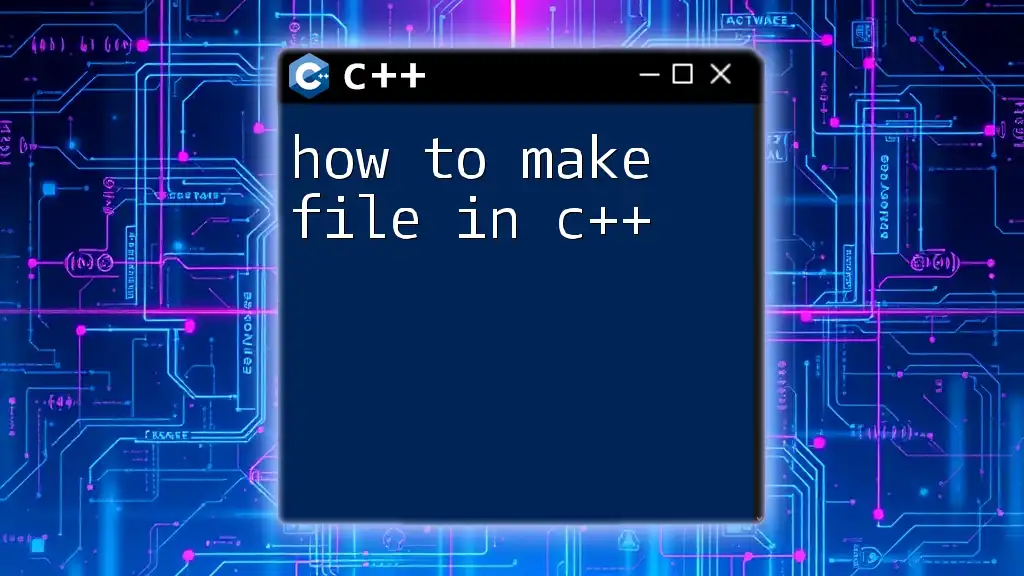
Handling Errors When Writing to Files
Checking for Errors
Error handling is vital in programming, particularly when dealing with file operations, as failures may occur for various reasons (permissions, non-existing paths, etc.). It's essential to implement error-checking mechanisms.
Example:
if (outFile.fail()) {
cerr << "Error writing to file." << endl;
}
In this snippet, we check whether any failure occurred during our write operations. If so, an appropriate error message is displayed.
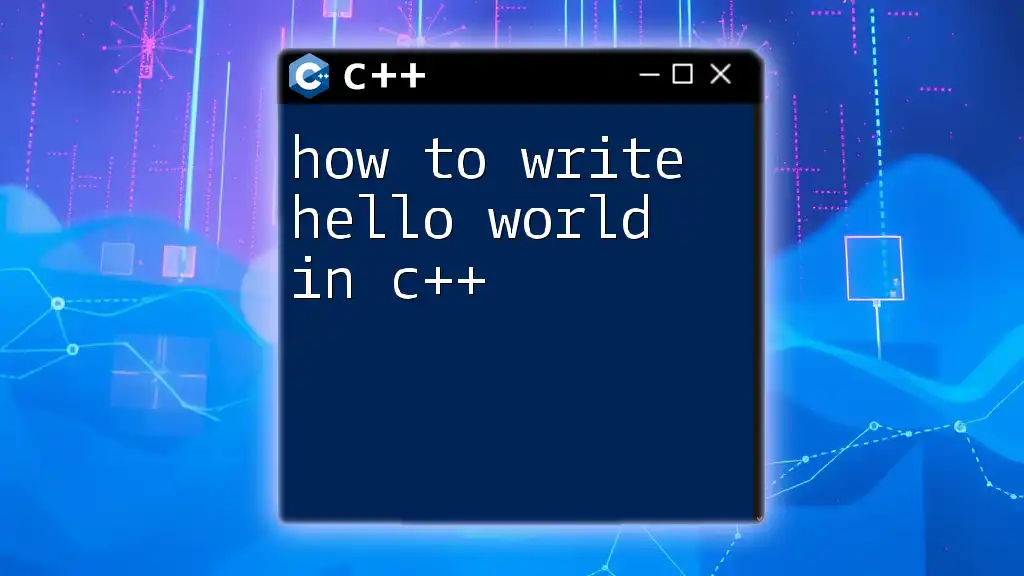
Examples of Writing to a File in C++
Writing Multiple Lines
If you need to write multiple lines efficiently, using loops can simplify the task. Here’s an example:
for (int i = 0; i < 5; i++) {
outFile << "Line " << i + 1 << endl;
}
Here, the loop writes five lines to the file, sequentially labeling them.
Writing Different Data Types
You can write various data types—like integers, strings, and floats—to a file seamlessly. Here’s how it looks in practice:
int age = 25;
float height = 5.9;
string name = "Alice";
outFile << "Name: " << name << ", Age: " << age << ", Height: " << height << endl;
This example illustrates writing different data types in a single line, showcasing the flexibility of C++ file writing capabilities.
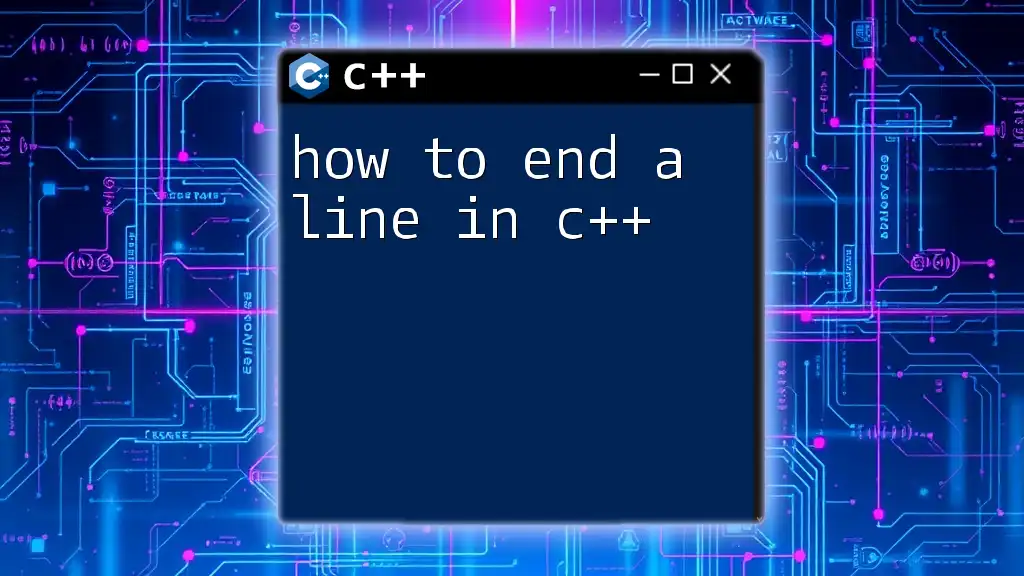
Best Practices for Writing to Files in C++
Using RAII (Resource Acquisition Is Initialization)
A best practice in managing file streams is employing the RAII principle. By utilizing constructors and destructors, files will automatically close when they go out of scope, significantly reducing the risk of resource leaks.
For example:
void writeFile() {
ofstream outFile("example.txt");
// File operations
} // outFile is automatically closed here
Always Check If Your File Is Open
Whenever you open a file, it's good practice to check if the operation was successful. Failing to do so might lead to unexpected behavior in your program.
Example:
if (!outFile) {
cerr << "Error opening file!" << endl;
}
This ensures that you are aware of any issues from the start, making debugging simpler.
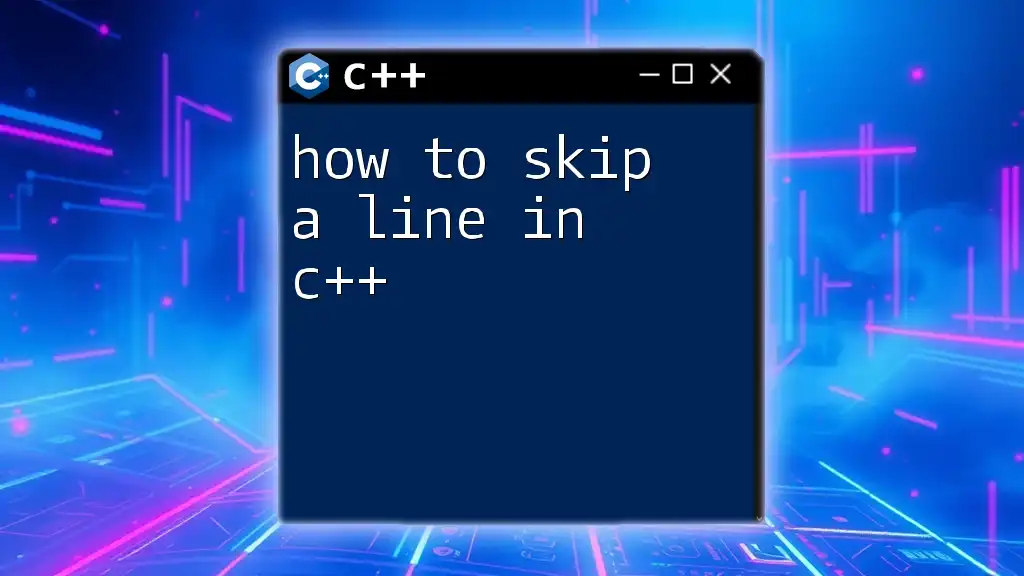
Conclusion
Learning how to write in a file in C++ is an essential skill for any developer. This guide covers the crucial aspects of file handling, including opening, writing, appending, and closing files properly. By following the provided examples and best practices, you'll develop robust and efficient file-handling techniques in your C++ projects. Always make sure to implement error handling to catch any potential issues and improve the reliability of your code. Now, you’re equipped to explore file writing in your applications!