To randomly shuffle elements in a C++ container, you can use the `std::shuffle` function along with a random number generator from the `<random>` header. Here's a concise code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <algorithm>
#include <random>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::random_device rd;
std::mt19937 g(rd());
std::shuffle(vec.begin(), vec.end(), g);
for (int n : vec) {
std::cout << n << ' ';
}
return 0;
}
Understanding Random Shuffle
What is Random Shuffle?
Random shuffling is the process of rearranging elements in a collection (like an array or a list) into a random order. This is crucial in many programming scenarios, especially where randomization is needed, such as:
- Card games: To ensure fairness by shuffling the deck.
- Shuffling playlists: Randomizing the order of songs so that listeners have a varied experience.
- Randomized algorithms: When working with algorithms that rely on random inputs to achieve their results.
The Importance of Randomness in Algorithms
Randomness plays a vital role in algorithm efficiency and result variability. In computer science, random algorithms can help in:
- Reducing computational time: Randomization often allows for faster solutions in problems like sorting and searching.
- Improving security: Systems that incorporate randomness are better equipped to handle cryptographic functions.
- Statistical sampling: Random shuffling ensures a representative sample from a dataset, which is crucial in statistics.
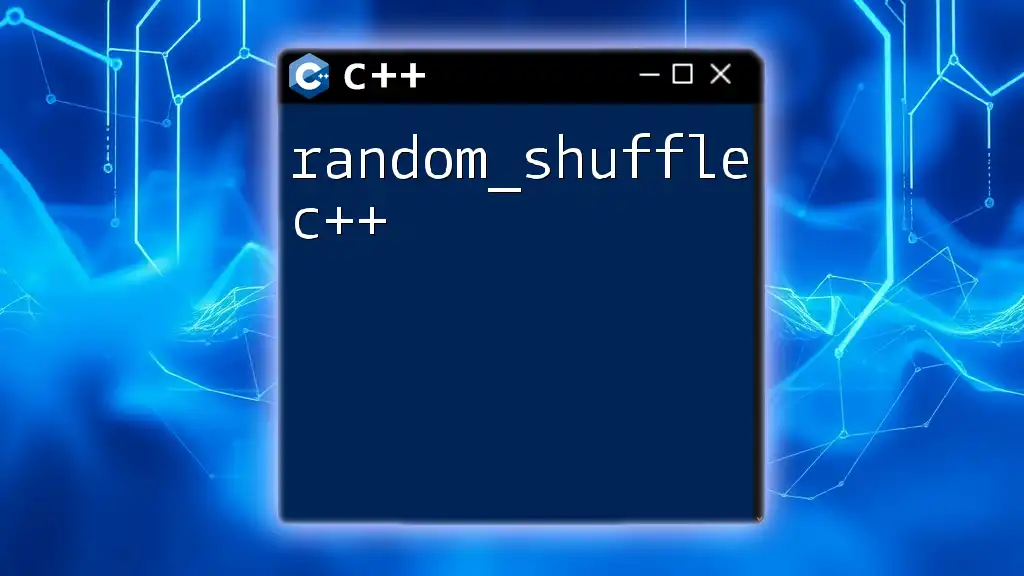
C++ Standard Library for Randomness
Introduction to `<algorithm>` and `<random>`
C++ provides a robust Standard Library that simplifies a variety of programming tasks, including randomization. The libraries `<algorithm>` and `<random>` are the cornerstones for implementing random shuffle.
Key Features of `<algorithm>`
The function `std::shuffle` from the `<algorithm>` library is particularly significant for random shuffling. This function allows you to shuffle the elements of a sequence in a highly efficient manner. Its power lies in the simplicity and effectiveness of the implementation.
Overview of `<random>`
The Random Number Generator (RNG)
To achieve randomness in your shuffle, you need a robust Random Number Generator (RNG), which is part of the `<random>` library. Various RNG engines are available, including:
- std::default_random_engine: A general-purpose engine that offers a balance of speed and randomness.
- std::mt19937: A high-quality RNG known as the Mersenne Twister, which is widely used due to its long period and uniform distribution.
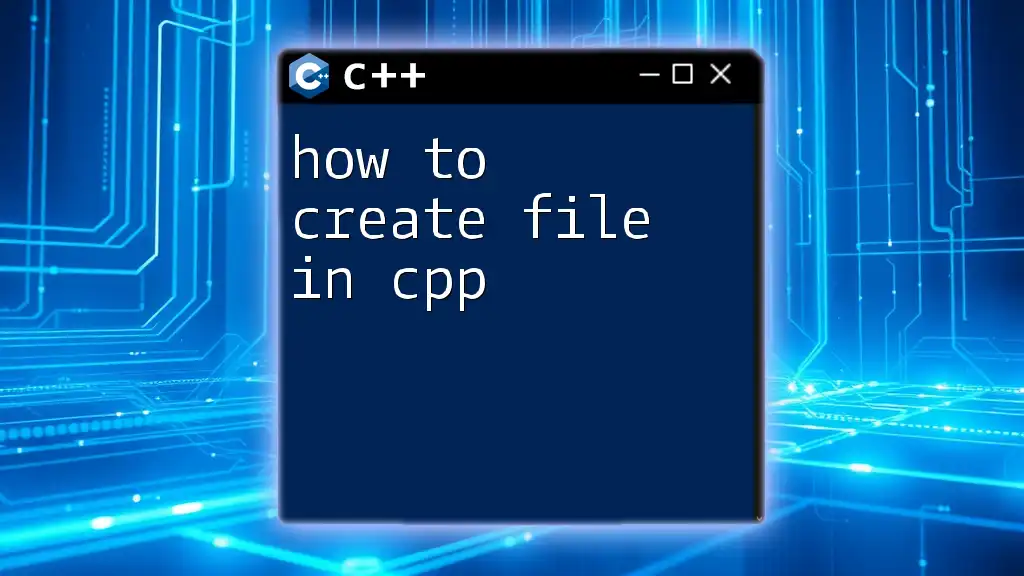
Implementing Random Shuffle in C++
Basic Setup
To start, you'll need a dataset to shuffle. The following code initializes a simple vector of integers:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
Using `std::shuffle`
The actual implementation of the shuffle using `std::shuffle` is straightforward. Below is an example demonstrating its use:
#include <algorithm>
#include <random>
#include <chrono>
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
// Random device for seeding
std::random_device rd;
std::mt19937 g(rd());
// Shuffle the vector
std::shuffle(nums.begin(), nums.end(), g);
for (int n : nums) {
std::cout << n << ' ';
}
return 0;
}
Explanation of the Code
-
Random Device for Seed: `std::random_device rd;` generates a non-deterministic random number to seed our RNG. This is essential for ensuring a unique shuffle every time you run the program.
-
Mersenne Twister: `std::mt19937 g(rd());` initializes the Mersenne Twister RNG with the seed, promoting high-quality randomness.
-
Shuffling: The `std::shuffle` function is called, taking the beginning and end of the vector along with the generator as arguments. This shuffles the elements in place and ensures the randomness is effectively distributed.
Custom Randomization
If you desire a deeper understanding or need special specifications, you may implement your own shuffling function. While not as efficient as the standard library functions, it can serve as a good exercise. Here's an example of a simple custom shuffle function:
void customShuffle(std::vector<int>& vec) {
for (int i = vec.size() - 1; i > 0; i--) {
int j = rand() % (i + 1);
std::swap(vec[i], vec[j]);
}
}
Note: Using custom shuffling emphasizes learning and understanding algorithms, but for most practical applications, it is advised to use `std::shuffle` for its efficiency and reliability.
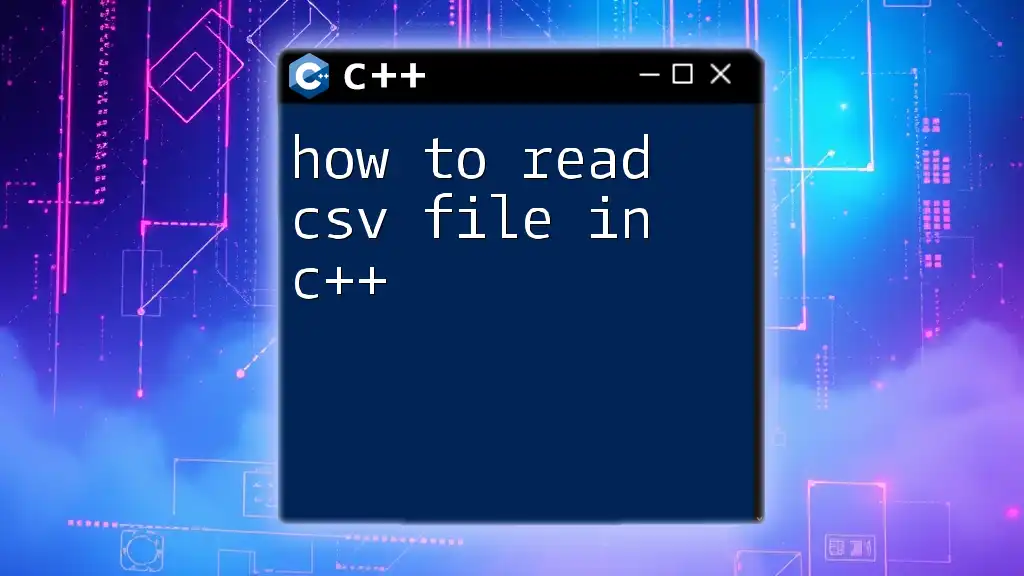
Testing and Verifying Randomness
Ensuring Fairness in Randomness
After shuffling, you may want to ensure that the shuffling is fair and exhibits randomness. One approach is to analyze the distribution of shuffled outcomes over multiple iterations.
It's crucial to consider statistical methods, such as the Chi-square test, to assess the uniformity of your shuffled array over time. Visualizing distributions can also offer insight into the effectiveness of your shuffle.
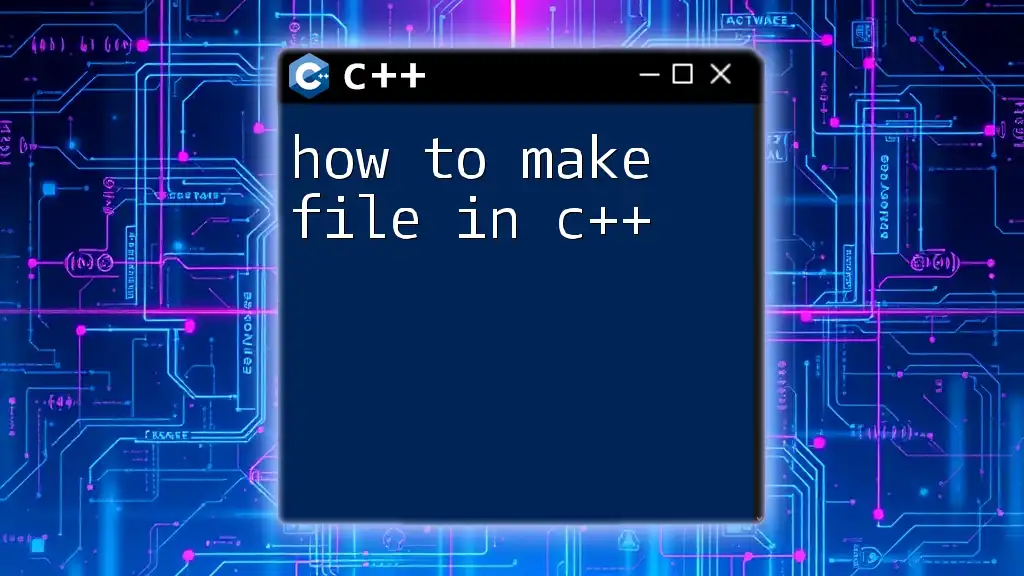
Conclusion
In summary, understanding how to random shuffle in C++ opens up new possibilities for various applications, from gaming to statistical algorithms. The `std::shuffle` function, in conjunction with `<random>`, provides a powerful, efficient way to randomize elements. Experimenting with different shuffling techniques can deepen your knowledge of C++ and enrich your programming skill set.
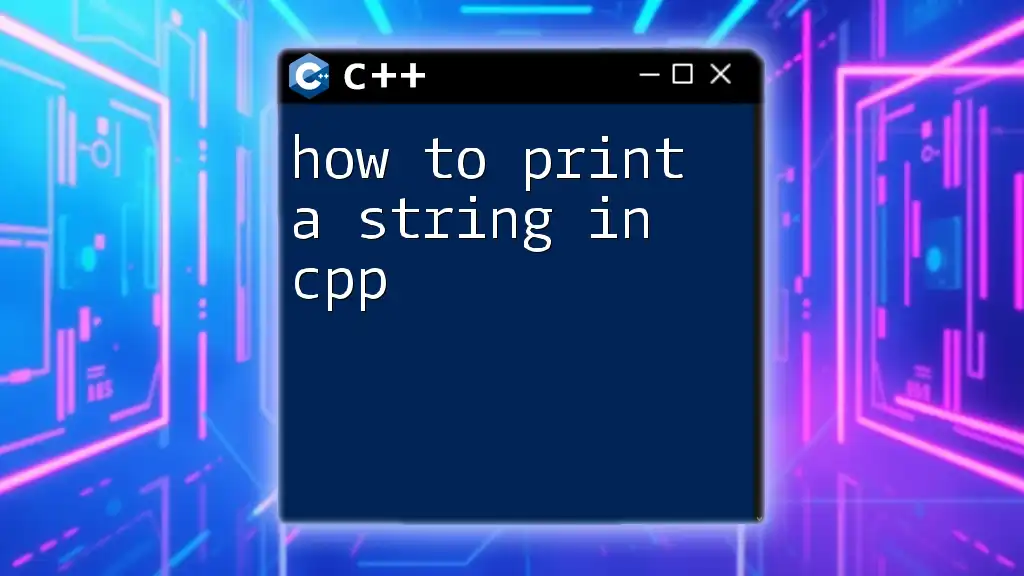
Further Reading
- For more detailed insights, refer to the official C++ documentation on [C++ algorithm library](https://en.cppreference.com/w/cpp/algorithm).
- Explore books like "The C++ Standard Library" by Nicolai M. Josuttis for a deeper understanding of C++ utilities and methodologies.
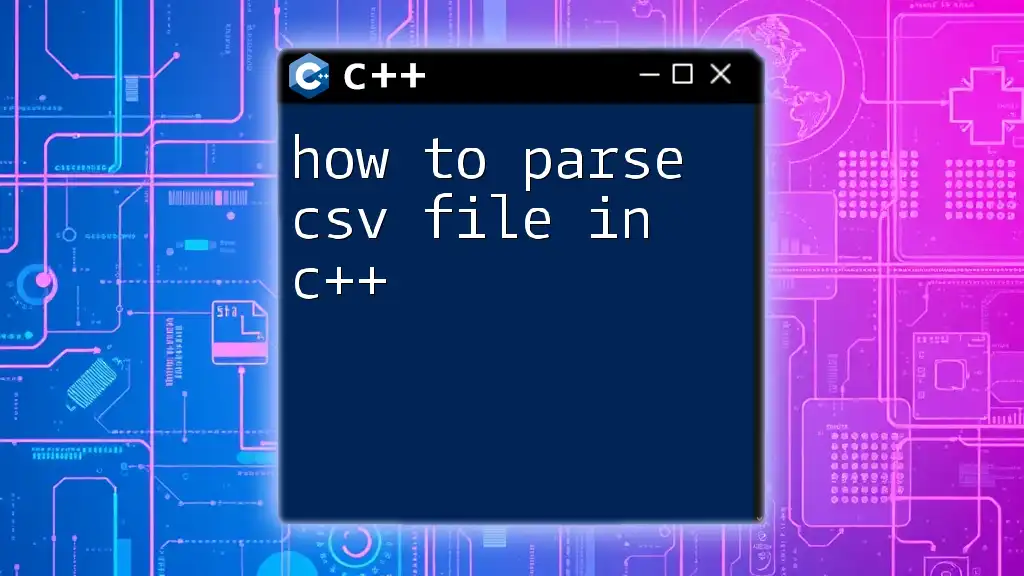
Call to Action
Try implementing shuffling techniques in your own C++ projects! Experiment with both `std::shuffle` and your custom algorithms, and don’t hesitate to share your challenges or successes in the world of randomization!