In C++, a `long double` is a floating-point data type that provides more precision and a larger range than a regular `double`, typically used for high-precision calculations.
Here’s a simple example:
#include<iostream>
#include<limits>
int main() {
long double ld = 3.14159265358979323846;
std::cout << "Value of long double: " << ld << std::endl;
std::cout << "Maximum long double: " << std::numeric_limits<long double>::max() << std::endl;
return 0;
}
What is a Long Double?
A long double is a floating-point data type in C++ that provides extended precision beyond that of the standard `double`. While a `double` typically offers about 15 to 17 decimal digits of precision, a `long double` can provide up to 18 to 19 decimal digits or more, depending on the compiler and system. This makes it ideal for calculations that require high precision, such as scientific computations, financial applications, and complex calculations involving many decimal places.
Differences between Float, Double, and Long Double
- float: This type typically uses 4 bytes and provides about 7 decimal digits of precision.
- double: This type typically uses 8 bytes and provides about 15 to 17 decimal digits of precision.
- long double: This type often uses 10, 12, or 16 bytes depending on the architecture, offering more than 18 decimal digits of precision.
The distinct advantage of the long double in C++ lies in its ability to handle very large or very small numbers with better accuracy than the other types.

Why Use Long Double?
In scenarios where precision is paramount, opting for a `long double` can prevent rounding errors that often arise with smaller floating-point types. Instances include:
- Scientific Computing: Fields like physics and chemistry require calculations that deal with very precise measurements.
- Financial Applications: Managing currency and economic calculations benefit from the increased precision to ensure accuracy.
- Data Analysis: When analyzing large datasets with intricate relationships, high precision aids in better statistical analysis.

Understanding the Long Double Data Type
Size and Precision of Long Double in C++
To understand how `long double` fits into the landscape of C++ data types, it is essential to examine its size and precision. The `sizeof(long double)` can yield different results based on the compiler being used.
Here’s a simple code example that demonstrates how to find the size and precision of `long double`:
#include <iostream>
#include <limits>
int main() {
std::cout << "Size of long double: " << sizeof(long double) << " bytes" << std::endl;
std::cout << "Precision of long double: " << std::numeric_limits<long double>::digits10 << " decimal digits" << std::endl;
return 0;
}
In this example, you can see how the `sizeof` operator retrieves the size of the `long double` datatype, and the `std::numeric_limits` class provides the number of decimal digits of precision.

How to Declare and Initialize Long Double
Declaring a Long Double Variable
Declaring a `long double` variable follows a straightforward syntax. Here's how you do it:
long double myVar = 3.14159265358979323846L;
The `L` suffix is crucial, as it indicates that the number should be treated as a `long double`, ensuring that the compiler handles it correctly without truncation.
Initializing Long Double
You can initialize a `long double` using various methods. Here are a couple of examples that highlight the correct initialization:
long double pi = 3.14159L; // Initialized with a constant
long double e = 2.71828182845904523536L; // Extended precision for e (Euler's number)
Notice again the `L` suffix, which is essential for ensuring the number retains its precision as `long double`.

Performing Operations with Long Double
Arithmetic Operations
Performing arithmetic operations with `long double` is similar to using other numeric types. Here’s how to do it:
long double a = 1.0L;
long double b = 2.0L;
std::cout << "Addition: " << (a + b) << std::endl;
std::cout << "Multiplication: " << (a * b) << std::endl;
This snippet performs basic arithmetic operations, and you can see how they work seamlessly with `long double` just like they do with other types.
Using Mathematical Functions
C++ provides a rich set of mathematical functions in the `<cmath>` library, which you can leverage with `long double`. For example, calculating the square root:
#include <cmath>
long double value = 9.0L;
std::cout << "Square root of 9: " << sqrt(value) << std::endl;
In this case, the `sqrt` function returns the square root of `value`, demonstrating the integration of `long double` with standard library mathematical functions.

Comparison Operations on Long Double
Using Relational Operators
Comparison operations using relational operators (`==`, `!=`, `<`, `>`, `<=`, `>=`) work just as they do with conventional floating-point types. However, caution is advised due to the potential for precision errors. Here’s an example:
long double x = 1.0L;
long double y = 1.0L;
if (x == y) {
std::cout << "x is equal to y." << std::endl;
}
This code checks if `x` and `y` are equal and will output the message if they are. It’s a simple yet effective demonstration of comparison operations.

Common Pitfalls with Long Double
Precision Issues and Rounding Errors
Despite the advantages of `long double`, it is crucial to remain aware of potential precision issues. For example:
long double a = 1.0L;
long double b = 1.0L - 1e-20L;
std::cout << "a == b: " << (a == b) << std::endl; // May not be true
In this example, even though the values of `a` and `b` seem very close, due to limitations in representation, they might not be treated as equal. Thus, caution is necessary when performing precision comparisons.
Platform Dependency of Long Double Size
Another consideration is that the size of `long double` can change based on the compiler and platform. For instance, under certain architecture, it may occupy 10 bytes, while other systems could allocate 16 bytes. It’s essential to consult the relevant documentation for your specific compiler to understand how `long double` is implemented on that platform.
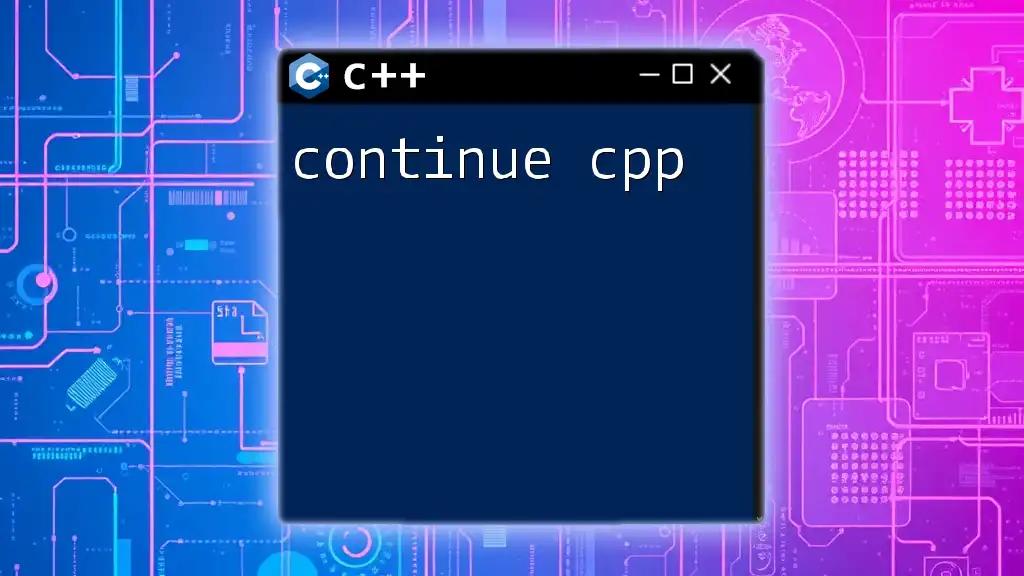
Conclusion
In summary, utilizing long double in C++ enables developers to perform calculations that demand higher precision. While it offers significant advantages, it’s essential to remain cognizant of the common pitfalls, such as precision errors and platform dependency. By understanding how to implement and handle `long double` appropriately, programmers can ensure accurate computations in their applications, whether dealing with scientific data or financial calculations.

Additional Resources
To deepen your understanding of `long double`, consider exploring the following resources:
- C++ documentation on floating-point types.
- Online courses focusing on advanced C++ programming.
- Books that cover numerical analysis and floating-point arithmetic in detail.
With this knowledge, you can effectively use `long double` in your C++ projects, ensuring the precision your applications require.