In C++, color codes can be used to change the text color of console output on supported terminals, for example, using ANSI escape codes:
#include <iostream>
int main() {
std::cout << "\033[31mThis text is red!\033[0m" << std::endl; // Red text
return 0;
}
The Basics of Color Output in C++
What is Console Color Coding?
Console color coding refers to the ability to change the color of text and background in terminal applications. This feature significantly enhances the readability and visual appeal of console output. By integrating color, developers can highlight important notifications or distinguish error messages, making it easier for users to read and react to information.
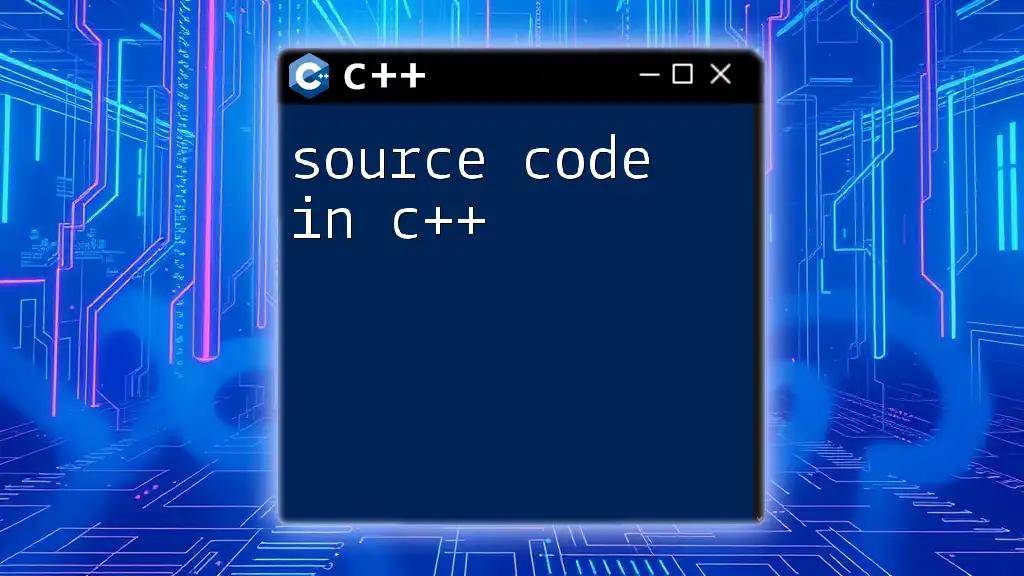
Color Codes in C++
Using ANSI Color Codes
C++ programs can utilize ANSI escape codes to add color to console output. ANSI escape codes start with an escape character followed by a sequence of characters that define the desired formatting.
A fundamental structure for an ANSI code looks like this:
std::cout << "\033[1;31mThis is red text\033[0m" << std::endl;
In this example:
- `\033` initiates the escape sequence.
- `[1;31m` specifies the text style (bold) and color (red).
- `\033[0m` resets the color to default after displaying the text.
Understanding Different Color Attributes
When manipulating colors in C++, it's essential to differentiate between foreground and background colors. Foreground colors refer to the text color, while background colors apply to the area behind the text.
The overall syntax for setting a color is:
\033[<style>;<foreground>;<background>m
Common ANSI Color Codes
Here are a few basic color codes you can use:
- Text Colors (Foreground):
- `30` - Black
- `31` - Red
- `32` - Green
- `33` - Yellow
- `34` - Blue
- `35` - Magenta
- `36` - Cyan
- `37` - White
- Background Colors:
- `40` - Black
- `41` - Red
- `42` - Green
- `43` - Yellow
- `44` - Blue
- `45` - Magenta
- `46` - Cyan
- `47` - White
Implementing Color Changes in C++
Creating Helper Functions for Color Output
To simplify color usage in your C++ programs, creating a helper function is advantageous. Below is an example function that allows you to easily set text color:
void setColor(int textColor, int bgColor) {
std::cout << "\033[" << textColor << ";" << bgColor << "m";
}
This function takes in two parameters defining the text and background colors.
Using the Helper Function
To utilize the `setColor` function effectively, you can call it before your text output:
setColor(31, 40); // Set text to red and background to black
std::cout << "This text is red on a black background." << std::endl;
setColor(0, 0); // Reset to default colors
This allows for easy and reusable color settings throughout your program.
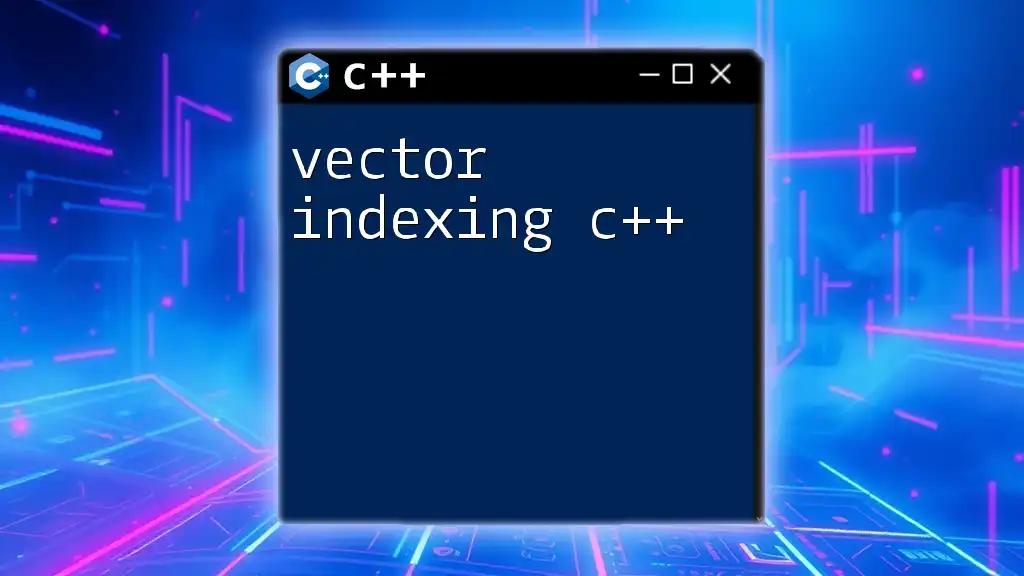
Advanced Color Techniques
Customizing Color with RGB Values (Windows)
While ANSI codes are beneficial for many platforms, Windows provides an additional way to change console text colors using the Windows API. This method allows developers to customize colors further with RGB values, though it requires utilizing Windows-specific functions.
For instance, you can use the following code snippet to change text color in a Windows console application:
#include <windows.h>
void setColor(int colorId) {
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), colorId);
}
Here, you can pass an integer that combines foreground and background color attributes, allowing for a wide range of colors.
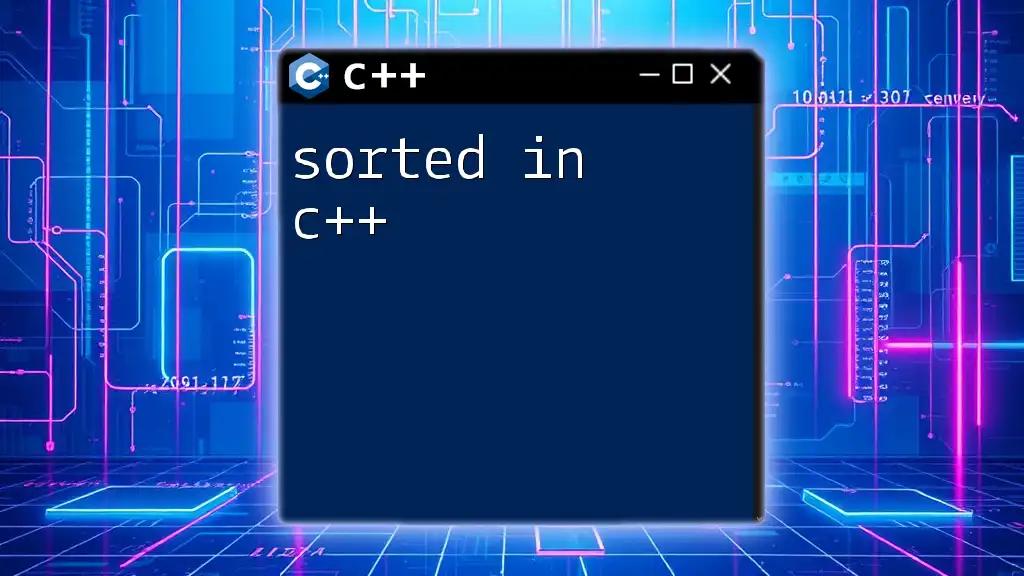
Best Practices for Color Coding
Using Color Sparingly
While color can enhance the user experience, too much color can lead to visual clutter. It's essential to use color judiciously. Highlighting key messages or differentiating sections is beneficial; however, using colors excessively may confuse or overwhelm users. For instance, employing a single color for error messages can be effective, rather than mixing multiple colors for different outputs.
Accessibility Considerations
Ensuring your color choices are accessible to a wider audience, including those with visual impairments, is crucial. A good practice is to use high-contrast colors and to combine color with other formatting options, such as text styles (bold or underline). Various online tools are available that can help assess the color contrast and accessibility standards.
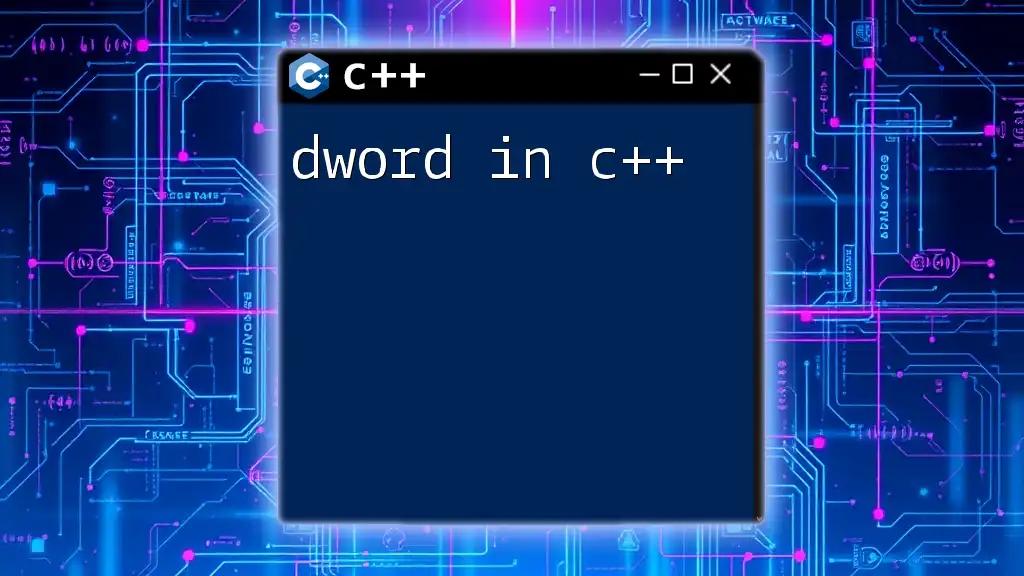
Conclusion
In conclusion, the color code in C++ is a powerful feature that can significantly enhance the user experience of console applications. From using simple ANSI escape codes to advanced Windows implementations, developers have a wealth of options for customizing text output. It's essential to experiment with these color codes while keeping in mind best practices and accessibility considerations to create effective and user-friendly applications.

Additional Resources
To further explore the use of color codes in C++, consider diving into relevant books, online documentation, and community forums. Engaging with the programming community will not only broaden your understanding but also inspire you to use color coding creatively in your projects.