Source code in C++ refers to the written instructions that define how a program operates, typically saved in files with a `.cpp` extension and compiled to create executable applications.
Here’s a simple example demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and its Functionality
C++ is a powerful programming language that combines the efficiency and performance of low-level programming with the flexibility and ease of high-level programming. Designed with the goals of providing better memory management, object-oriented programming features, and system-level access, C++ is widely used in software development, game development, and high-performance applications. Its importance lies in its ability to provide developers with tools that allow for efficient computation and system-level programming.
What is Source Code?
Source code refers to the collection of code written by programmers in a programming language, such as C++. It is the foundation for building software applications and is compiled into machine code that the computer can execute. The process of transforming C++ source code into executable programs involves a compiler, which translates human-readable commands into machine-friendly language. Understanding source code in C++ is crucial for developers who wish to create efficient, effective applications.
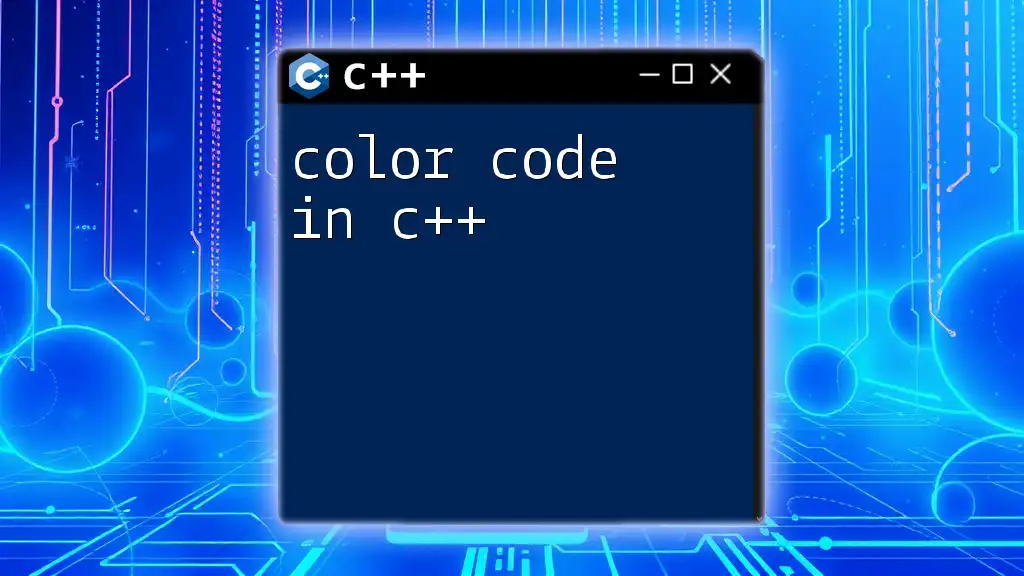
The Structure of a C++ Program Source Code
Elements of C++ Source Code
Headers and Libraries play a pivotal role in C++. They allow developers to include pre-defined functions and classes that facilitate programming. For instance, including the standard input-output library would look like this:
#include <iostream>
The main function is the starting point of every C++ program. It signals where the program begins execution. Here’s the basic structure of the `main()` function:
int main() {
// code goes here
return 0;
}
Inside the `main()` function, programmers write declarations and statements. This includes defining variables, constants, and determining their data types. For example, you might declare an integer variable like this:
int age = 30;
Control Structures in C++ Source Code
Conditional Statements
C++ allows the use of conditional statements like `if`, `else if`, and `else` to control the flow of the program. This capability enables developers to execute code based on certain conditions. Here's how you might use an if statement:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
Loops
Loops in C++ provide a way to execute a block of code repeatedly as long as a specified condition holds true. The most commonly used loops include the `for`, `while`, and `do-while` loops. A typical `for` loop may look like this:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
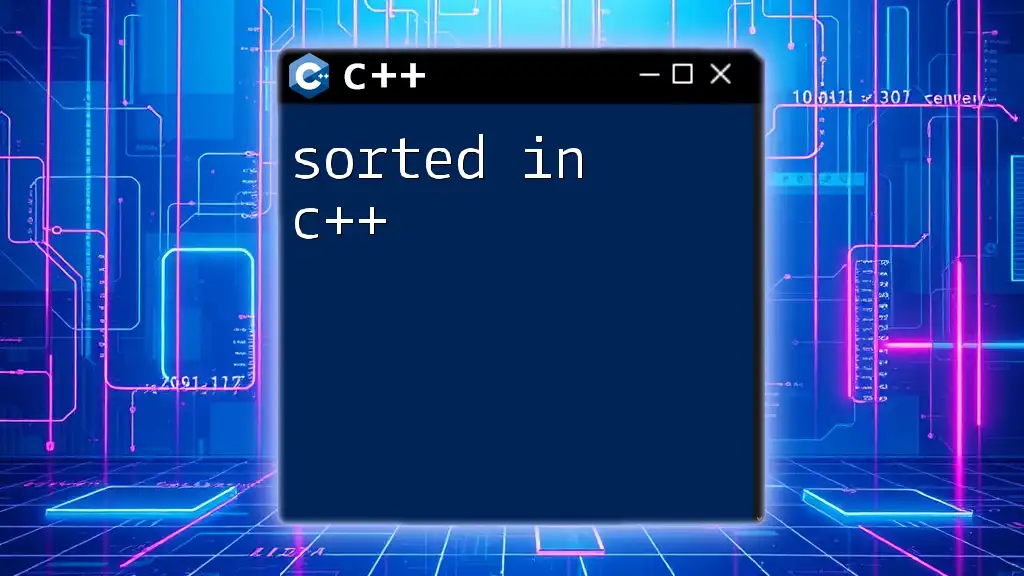
Writing Efficient C++ Source Code
Code Organization and Readability
Writing efficient C++ source code involves prioritizing organization and readability. Comments are essential for describing complex parts of your code, helping others (or even yourself) understand your intentions later. It's also crucial to use meaningful variable names. For instance:
// Calculate the area of a circle
double radius = 5;
double area = 3.14 * radius * radius;
Best Practices in C++ Source Code
To ensure clarity and maintainability, developers should adhere to best practices such as consistent formatting and indentation, avoiding redundant code, and employing functions. Using functions not only enhances clarity but also promotes code reuse. Here’s a simple example of a function that calculates the area of a circle:
double calculateArea(double r) {
return 3.14 * r * r;
}
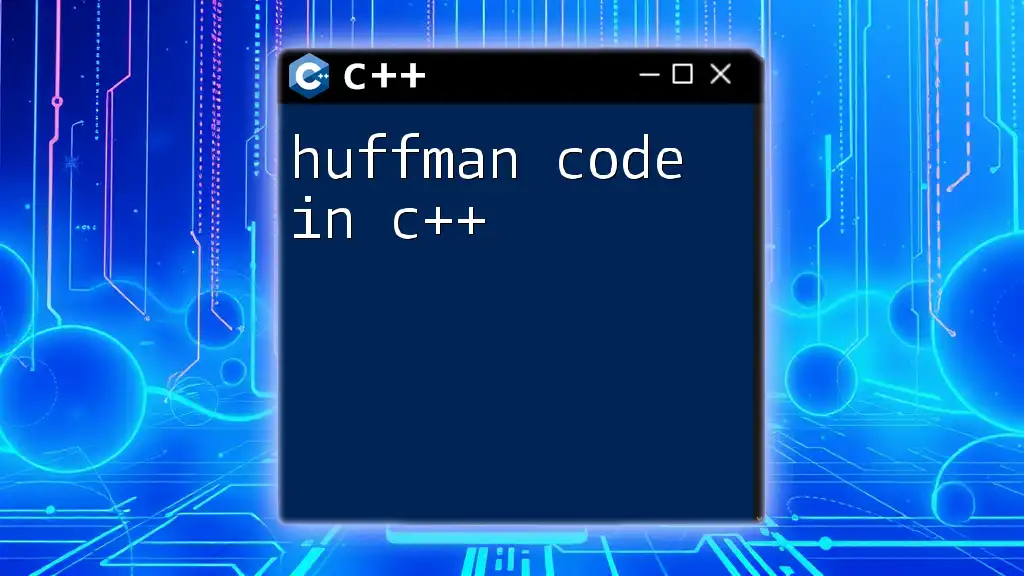
Debugging and Testing C++ Source Code
Common Errors and How to Fix Them
When writing source code in C++, developers will inevitably encounter errors. It’s vital to understand the distinction between syntax errors (which prevent code from compiling) and logical errors (which allow code to compile but produce wrong results). Using debugging tools, such as integrated development environments (IDEs) with built-in debuggers, can facilitate troubleshooting.
Testing Your C++ Code
Testing is a critical aspect of software development. Unit testing allows developers to validate specific functionalities within their code. For example, here’s how one might test a simple function:
assert(calculateArea(5) == 78.5);
This assertion checks that the function correctly computes the area for a circle with a radius of 5.
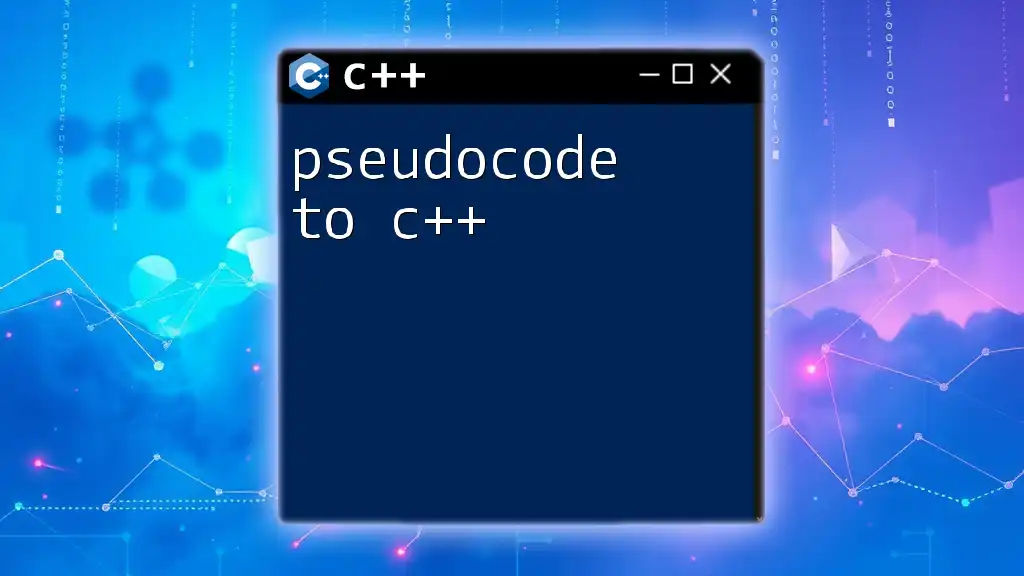
Practical Examples of C++ Source Code
Building a Simple C++ Program
To illustrate the construction of source code in C++, let's create a simple calculator program. This example will prompt the user for two numbers and then calculate and display their sum. Below is a complete code example:
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two numbers: ";
cin >> a >> b;
cout << "Sum: " << (a + b) << endl;
return 0;
}
This straightforward implementation demonstrates basic input/output concepts alongside arithmetic operations in C++.
Advanced C++ Source Code Techniques
Object-Oriented Programming in C++
C++ supports object-oriented programming (OOP), which organizes code around objects rather than actions. This paradigm allows for increased modularity and reusability. Here’s an example of a simple class that represents a rectangle:
class Rectangle {
public:
int width, height;
int area() {
return width * height;
}
};
In this example, the `Rectangle` class has two attributes, `width` and `height`, and a method `area()` that computes the area of the rectangle, showcasing the principles of encapsulation in OOP.
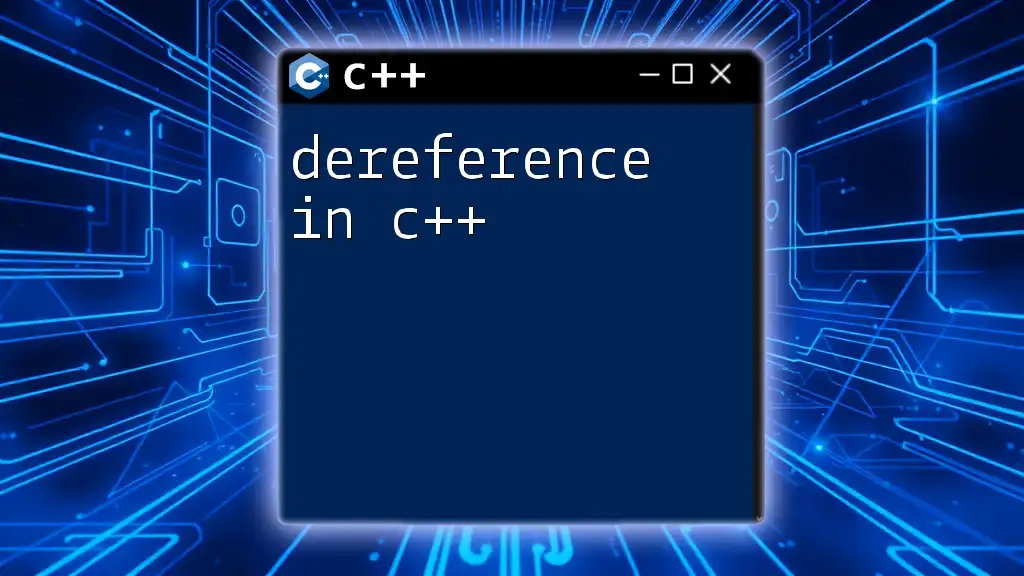
Conclusion
Understanding source code in C++ is foundational for anyone aspiring to be a proficient programmer. From grasping the structure of C++ programs to effectively writing, debugging, and testing code, mastering these fundamentals opens the door to building complex, real-world applications.
As you continue your coding journey, remember to explore C++’s vast possibilities and consider diving deeper into its advanced features. Numerous resources, including books, online courses, and programming communities, are available to help you on your path to becoming a C++ expert. Embrace the challenges of programming with excitement and curiosity!