Pseudocode serves as a high-level representation of algorithms, making it easier to convert logical steps into executable C++ code. Here’s an example of a simple pseudocode to find the sum of two numbers and its corresponding C++ implementation:
// Pseudocode:
// 1. Start
// 2. Initialize num1 and num2
// 3. Set sum = num1 + num2
// 4. Output sum
// 5. End
#include <iostream>
using namespace std;
int main() {
int num1 = 5; // Initialize num1
int num2 = 10; // Initialize num2
int sum = num1 + num2; // Calculate sum
cout << "Sum: " << sum << endl; // Output sum
return 0; // End
}
Understanding Pseudocode
What is Pseudocode?
Pseudocode is a high-level description of a computer programming algorithm. It uses the structure of programming languages without strict syntax rules, allowing developers to focus on the logic of the algorithm instead of the specifics of implementation. The main characteristics of pseudocode include:
- Simplicity: It’s easy to understand for both technical and non-technical audiences.
- Flexibility: There are no strict rules, which means it can be modified according to the needs of the programmer.
The benefits of using pseudocode include helping to clarify thoughts before diving into actual coding, allowing team members to discuss algorithms before committing to a programming language syntax, and providing a way to document the approach taken to solve a problem.
Example of Pseudocode:
START
SET total to 0
FOR each number in the list
ADD number to total
END FOR
PRINT total
END
Common Structures in Pseudocode
Sequential Execution
This is the simplest control structure where commands are executed in order from top to bottom.
Example in Pseudocode:
SET a to 10
SET b to 20
SET c to a + b
PRINT c
Visual representation shows that each line executes one after another without any interruptions.
Conditional Statements
Conditional statements allow the execution of certain blocks of code based on the evaluation of conditions.
Example of an If-Else Statement:
IF a > b THEN
PRINT "a is greater"
ELSE
PRINT "b is greater"
ENDIF
Flowcharts can be useful for visualizing the logic in the conditional statements, illustrating the decision-making process clearly.
Loops
Loops enable repeated execution of a block of code as long as a specified condition is met.
Examples:
- For Loop:
FOR i FROM 1 TO 10
PRINT i
END FOR
- While Loop:
WHILE the condition is true
DO something
END WHILE
These constructs can often be represented visually with flowcharts to illustrate the looping process and the exit conditions.
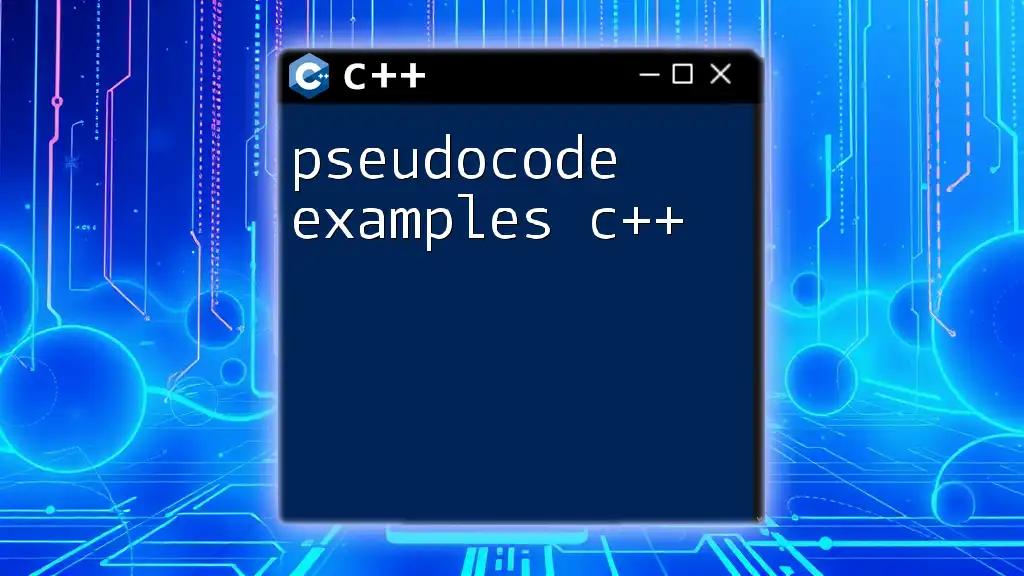
Translating Pseudocode to C++
Basic Syntax of C++
C++ serves as a powerful tool for translating pseudocode into executable code. The general structure of a simple C++ program typically includes:
#include <iostream>
using namespace std;
int main() {
// your code here
return 0;
}
When comparing the syntax of pseudocode to C++, you can see that pseudocode is less formal, allowing for flexibility in expression compared to the more rigid structure of C++.
Translating Conditional Statements
If-Else Statements
Translating an if-else statement from pseudocode to C++ follows a similar thought process but adheres to C++ syntax rules.
Pseudocode Example:
IF a > b THEN
PRINT "a is greater"
ELSE
PRINT "b is greater"
ENDIF
Corresponding C++ Code:
if (a > b) {
cout << "a is greater" << endl;
} else {
cout << "b is greater" << endl;
}
In this C++ example, the logic remains intact, but the syntax follows C++ conventions.
Switch Statements
Switch statements offer a way to execute different blocks of code based on the value of a variable. This is a useful alternative to multiple if-else statements.
Pseudocode Example:
SWITCH color
CASE "red":
PRINT "Red is selected"
CASE "green":
PRINT "Green is selected"
DEFAULT:
PRINT "Unknown color"
END SWITCH
Corresponding C++ Code:
switch (color) {
case "red":
cout << "Red is selected" << endl;
break;
case "green":
cout << "Green is selected" << endl;
break;
default:
cout << "Unknown color" << endl;
}
Translating Loops
For Loops
Pseudocode loops can easily be converted to C++ loops by following similar logic.
Pseudocode Example:
FOR i FROM 1 TO 10
PRINT i
END FOR
Corresponding C++ Code:
for (int i = 1; i <= 10; i++) {
cout << i << endl;
}
This translation accurately conveys the control structure while adhering to C++ syntax.
While Loops
While loops in pseudocode also directly translate to C++, though care should be taken to avoid infinite loops.
Pseudocode Example:
WHILE the condition is true
DO something
END WHILE
Corresponding C++ Code:
while (condition) {
// do something
}
It’s crucial to ensure that the condition eventually becomes false to allow the program to exit from the loop gracefully.

Functions in Pseudocode and C++
Defining Functions
Functions modularize code and promote reusability. Understanding how to translate them is vital for effective programming.
Pseudocode Example:
FUNCTION add(a, b)
RETURN a + b
END FUNCTION
Corresponding C++ Code:
int add(int a, int b) {
return a + b;
}
This example shows a direct relationship between the function's purpose in both pseudocode and C++.
Passing Parameters
Functions often require parameters, which can enhance flexibility.
Pseudocode Example:
FUNCTION multiply(x, y)
RETURN x * y
END FUNCTION
Corresponding C++ Code:
int multiply(int x, int y) {
return x * y;
}
Returning Values
Understanding how to return values is fundamental to function utility.
Pseudocode Example:
FUNCTION calculateArea(width, height)
RETURN width * height
END FUNCTION
Corresponding C++ Code:
int calculateArea(int width, int height) {
return width * height;
}
In both examples, the intention of the function remains clear and consistent through a simple translation process.
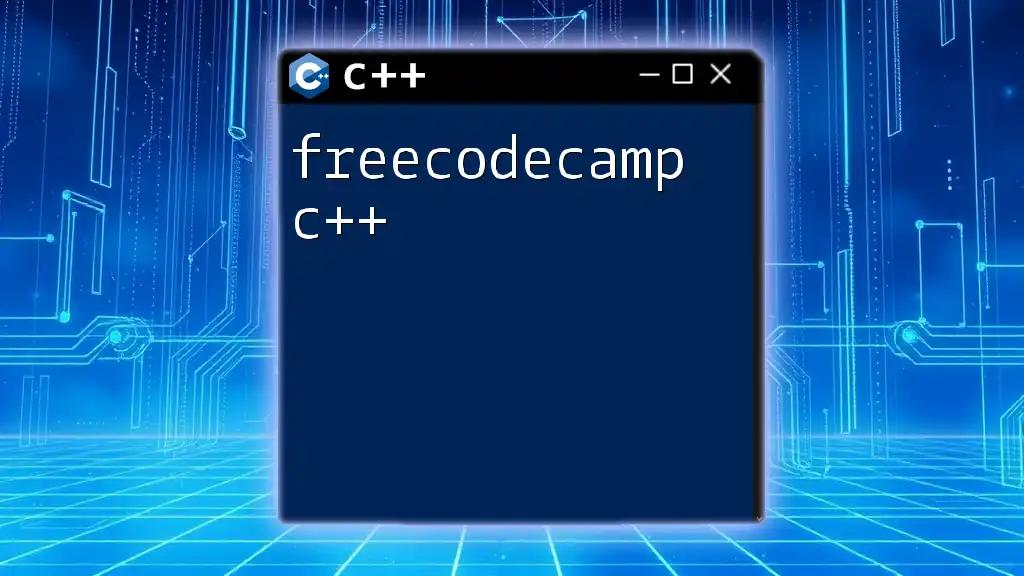
Working with Data Structures
Arrays
Pseudocode for Array Operations
Arrays provide a way to manage multiple data entries efficiently.
Example Pseudocode:
SET arr TO [1, 2, 3, 4, 5]
PRINT arr[0]
C++ Implementation of Arrays
Translating the pseudocode example into C++ looks like this:
int arr[] = {1, 2, 3, 4, 5};
cout << arr[0] << endl;
This example demonstrates initializing an array and accessing an element, mirroring the pseudocode structure.
Structures (Structs)
Pseudocode for Structs
Structs allow grouping related properties.
Pseudocode Example:
STRUCT Person
STRING name
INT age
END STRUCT
C++ Implementation of Structs
Translating that to C++ would look like this:
struct Person {
string name;
int age;
};
Accessing struct members in C++ remains straightforward:
Person p;
p.name = "Alice";
p.age = 30;
This maintains the structure while applying C++ syntax.
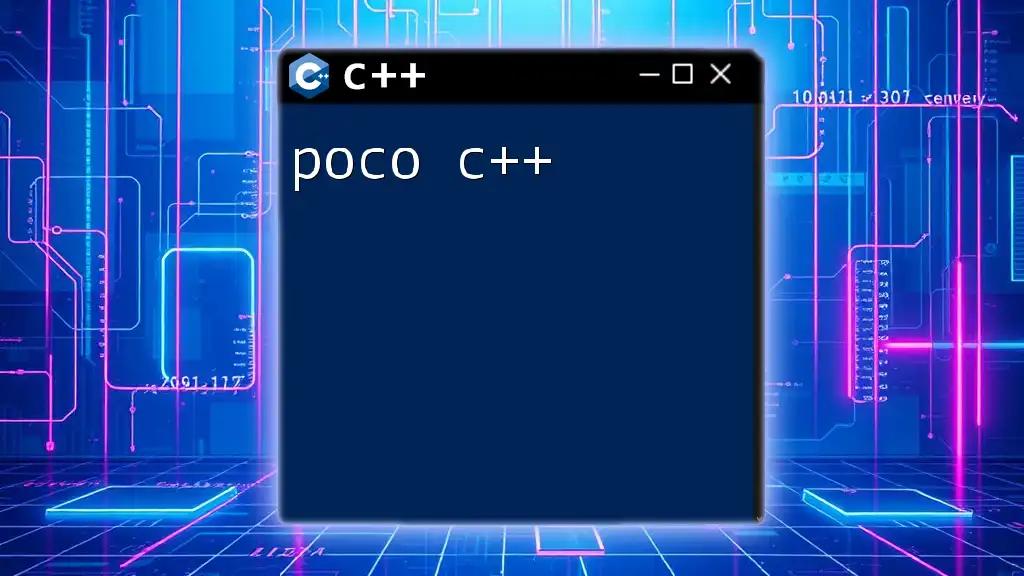
Best Practices for Translating Pseudocode to C++
Keeping It Simple
When translating pseudocode to C++, always aim for simplicity. Overly complex implementations can lead to errors and confusion. Avoid adding unnecessary layers of logic; keep things clear and straightforward.
Commenting and Documentation
One of the keys to maintaining code readability is effective commenting. Comments should explain the why of a piece of code rather than the what since the code itself should be clear enough to convey the latter.
Best Practices for Writing Comments:
- Use a consistent style.
- Write meaningful comments that add context to complex code sections.
Example:
// Calculate the area of a rectangle
int area = calculateArea(width, height);
Testing and Debugging
Testing your code is critical. Use various tools and methodologies such as unit tests and debugging tools to ensure your C++ implementation behaves as expected.
It's advisable to incrementally test your code as you convert pseudocode, which helps localize errors effectively.
// Example of a simple test
assert(calculateArea(5, 10) == 50);
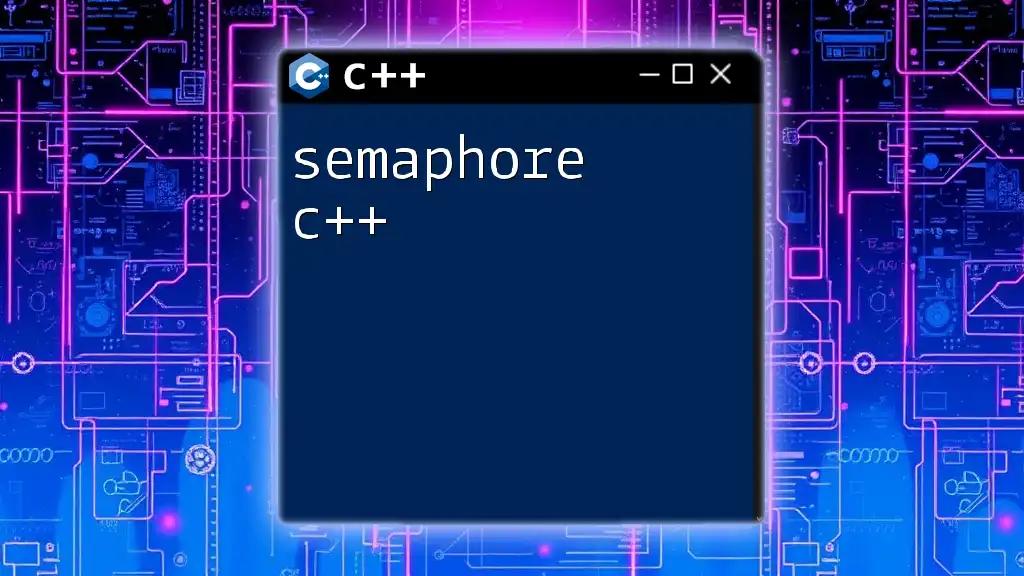
Conclusion
This guide outlined how to transition from pseudocode to C++, focusing on conditional statements, loops, functions, and data structures. The essence of translating pseudocode to C++ lies in understanding the underlying logic and maintaining clarity during the conversion process. Practice is the key—start converting more pseudocode examples into C++ to gain confidence and proficiency in the language!
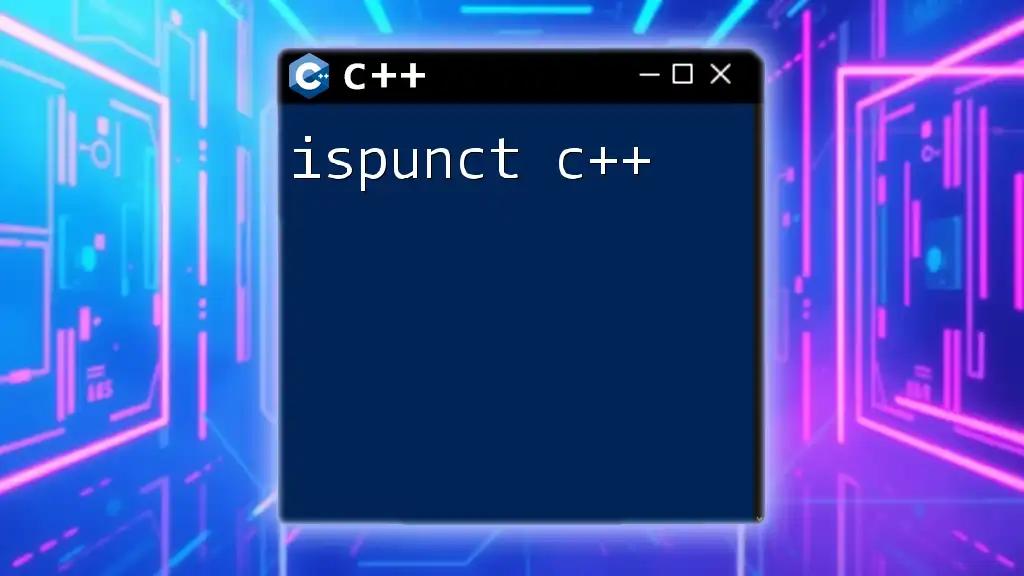
Additional Resources
To enhance your understanding and skills, consider utilizing recommended books, online courses, and community forums. Engaging with other passionate programmers can offer support and additional insights into both pseudocode and C++.