Pseudocode is a high-level description of an algorithm that outlines the logic of a C++ program in a simplified manner, making it easier to understand before actual coding begins.
Here's a simple example of pseudocode represented in C++ syntax for calculating the factorial of a number:
// Pseudocode for calculating factorial of a number
function factorial(n):
if n <= 1:
return 1
else:
return n * factorial(n - 1)
Understanding Pseudocode
What is Pseudocode?
Pseudocode is a high-level representation of algorithmic logic that allows programmers and non-programmers alike to convey complex ideas in a simple, human-readable format. It is not bound by the syntax rules of any specific programming language, making it a versatile tool for understanding and planning how code will execute.
Benefits of Using Pseudocode
Using pseudocode offers numerous advantages:
- Clarity: It simplifies complex programming ideas, allowing for better understanding and communication.
- Language Agnostic: Since pseudocode uses plain language and simplified expressions, it can be understood by anyone, regardless of their programming expertise.
- Focus on Logic: Encourages developers to concentrate on the algorithmic approach and logic before diving into the syntax-specific implementation of a programming language.
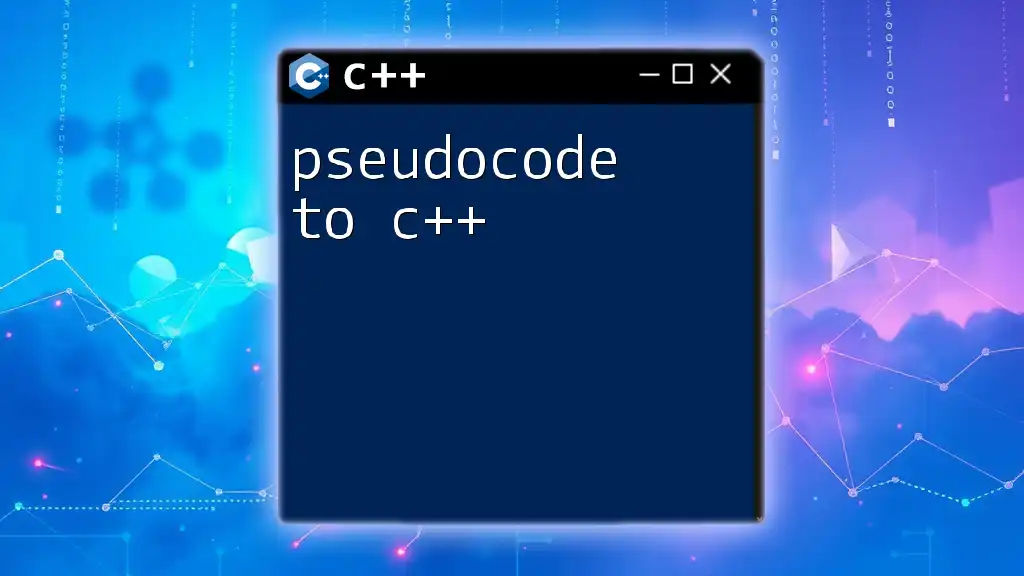
Transitioning from Pseudocode to C++
How Pseudocode Relates to C++
Pseudocode serves as a crucial stepping stone that bridges the gap between an idea and code implementation. It emphasizes the logical flow and steps required to solve a problem, which can then be translated into the specific constructs of a programming language like C++. Understanding this transition can significantly improve coding efficiency and accuracy.
Example Translation
Let’s examine a basic example to illustrate this transition. Consider the following pseudocode:
If the user's age is greater than 18 then
Print "You are an adult."
Else
Print "You are a minor."
This can be directly translated to C++ as:
if (age > 18) {
std::cout << "You are an adult.";
} else {
std::cout << "You are a minor.";
}
As you can see, the pseudocode clearly communicates the logic, which can be directly mapped to C++ syntax.
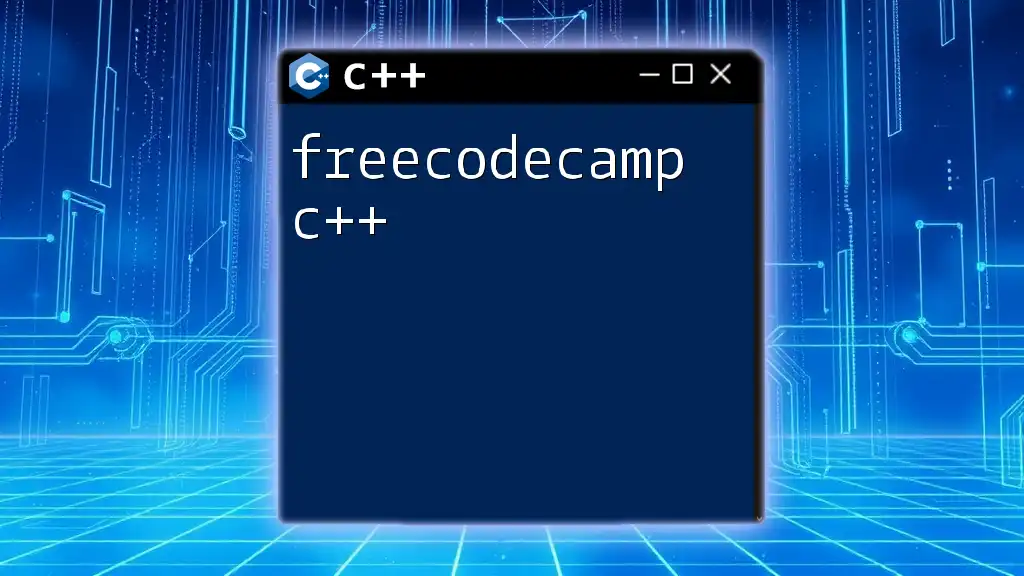
Writing Pseudocode: Best Practices
Simplify Your Approach
When crafting pseudocode, aim for conciseness and clarity. Avoid unnecessary complexity by using simple language. The objective is to formulate ideas that are easy to understand at a glance.
Consistency is Key
Consistency in your pseudocode enhances readability. Use identical naming conventions and maintain a uniform format across loops, conditionals, and functions. This fosters familiarity, making it easier for others to read and follow your logic.
Example of Good vs. Poor Pseudocode
Consider the following good example:
For each number in the list
If the number is even
Add it to the sum
In contrast, this poor example lacks clarity:
Loop through stuff and add things if they're even.
The good example clearly establishes the logic, while the poor example is vague and lacks structured detail.
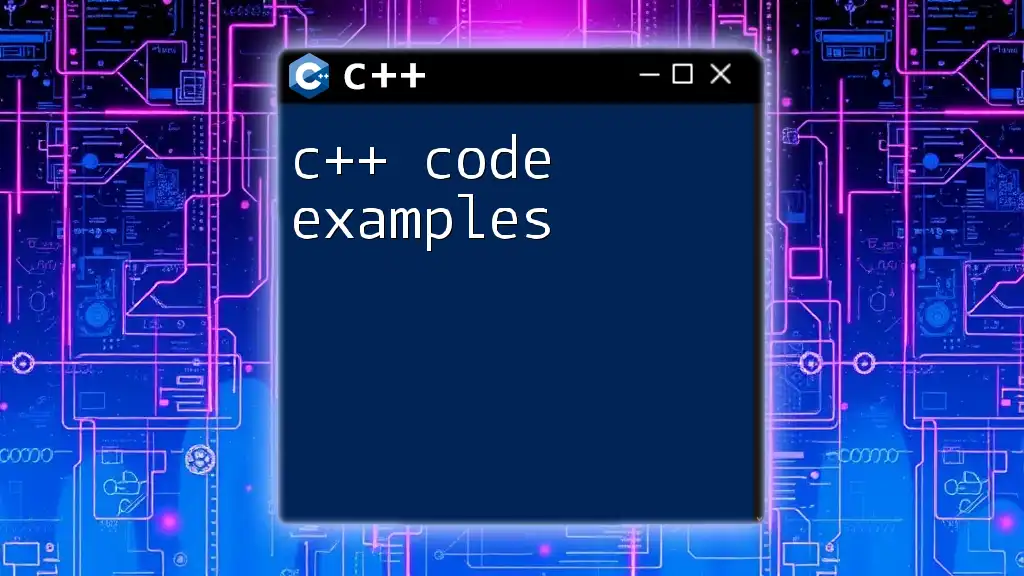
Common Patterns in Pseudocode
Control Structures
Understanding control structures is key in designing algorithms. Let's delve into two common types:
Conditionals
Conditionals allow you to execute different code paths based on specific conditions. A structure for a conditional in pseudocode may look like this:
If condition then
action
Else
alternative action
Loops
Loops enable repeated execution of code as long as a condition is true. The pseudocode structure for a loop can be represented as:
For each item in collection
action
Functions and Subroutines
Functions help to modularize your code, making it easier to manage and understand. A typical pseudocode structure for a function could be:
Function calculateSum(list)
Initialize sum to 0
For each number in list
Add number to sum
Return sum
This pseudocode clearly outlines the purpose of the function and the steps involved in achieving this goal.
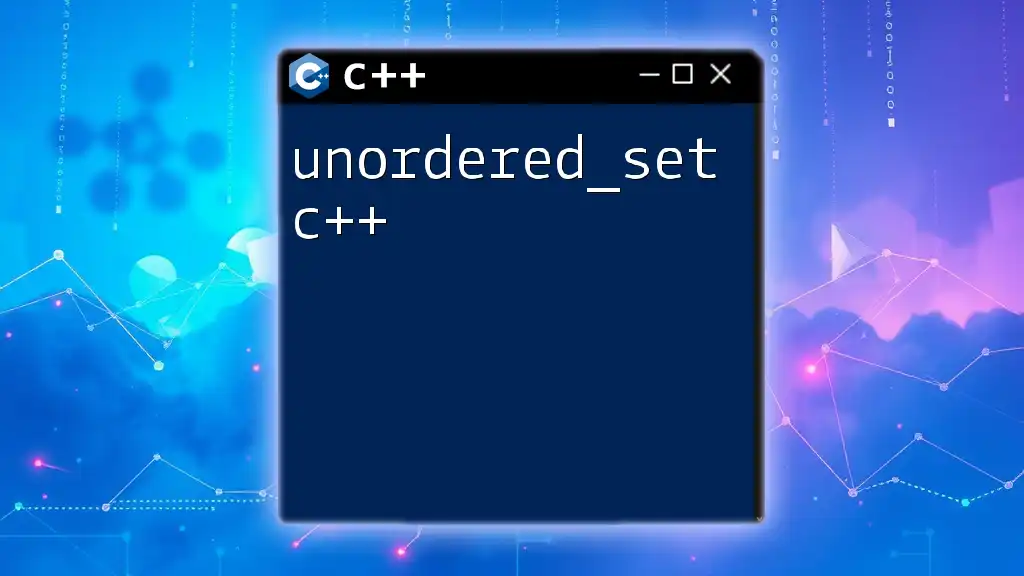
Practical Examples of Pseudocode in C++
Example 1: Basic Algorithms
Pseudocode for Finding the Maximum
To find the maximum value in a list of numbers, you might write:
Function findMax(numbers)
Initialize max to the first number
For each number in numbers
If number > max then
max = number
Return max
With this pseudocode as a guide, the corresponding C++ implementation would be:
int findMax(const std::vector<int>& numbers) {
int max = numbers[0];
for (int number : numbers) {
if (number > max) {
max = number;
}
}
return max;
}
Example 2: Sorting Algorithms
Pseudocode for Bubble Sort
If you are implementing a sorting algorithm, like Bubble Sort, your pseudocode may look like this:
Function bubbleSort(array)
For i from 0 to length(array) - 1
For j from 0 to length(array) - i - 1
If array[j] > array[j + 1]
Swap(array[j], array[j + 1])
The corresponding C++ code would be:
void bubbleSort(std::vector<int>& array) {
int n = array.size();
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (array[j] > array[j + 1]) {
std::swap(array[j], array[j + 1]);
}
}
}
}
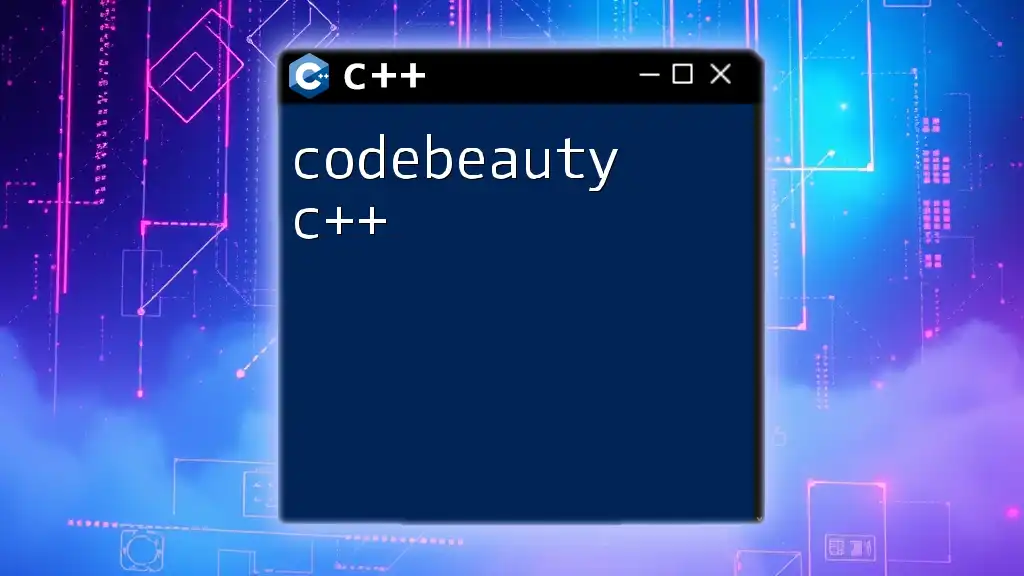
Conclusion
Recap of Key Points
Pseudocode is a vital tool in the programming landscape, serving as a roadmap for algorithm development. It allows for clear communication and planning before moving to the coding stage. By emphasizing clarity and consistency, you can enhance both the design and implementation of your C++ programs.
Encouragement to Practice
We encourage you to start experimenting with writing your own pseudocode. Begin with simple problems and gradually increase complexity. Practice converting your pseudocode into actual C++ code to reinforce your understanding of the concepts discussed.
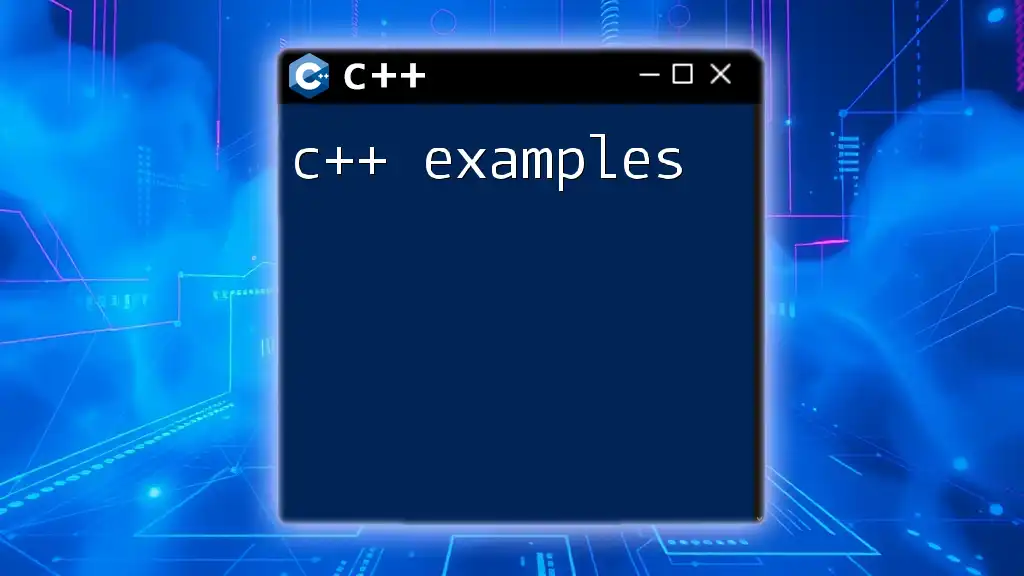
Further Readings and Resources
Recommended Books and Websites
There are numerous resources available that delve deeper into pseudocode and C++. For comprehensive learning, consider exploring well-known programming literature and online guides that will provide additional insights.
Online Courses and Tutorials
Platforms like Coursera and Udemy offer a variety of courses on C++ programming, often incorporating pseudocode to simplify learning. Engaging with these courses can greatly enhance your command of both algorithm design and C++ syntax.