In C++, `cout` is used to output data to the console, and here is a simple example illustrating how to use it:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is `cout` in C++?
In C++, `cout` is an essential component used for outputting data to the console. It serves as a standard output stream, allowing programmers to convey messages, variable values, and results of computations directly to users. Understanding how to effectively use `cout` is crucial for anyone learning C++, as it provides a way to display information and debug code.
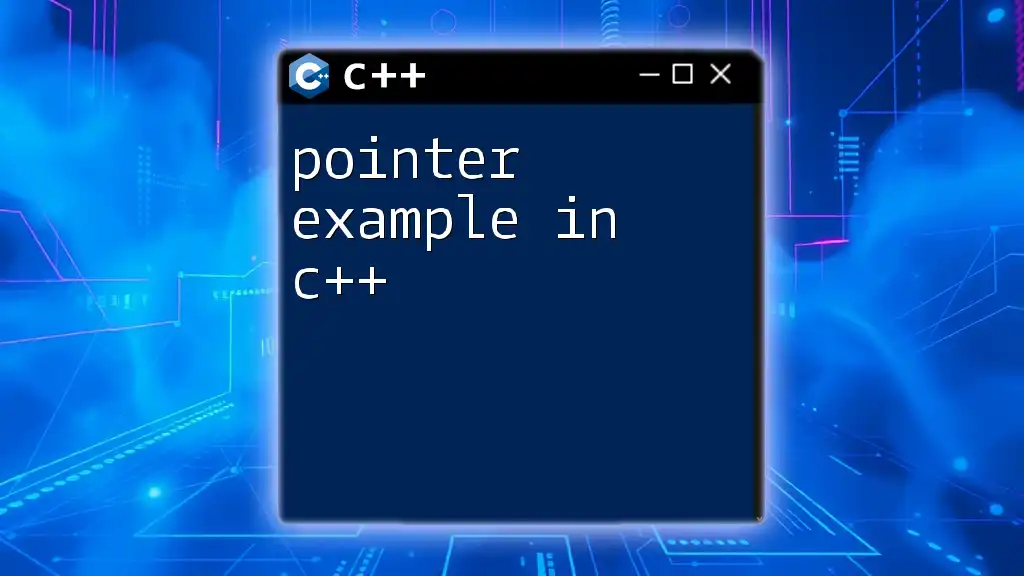
Importance of `cout` in C++ Programming
Learning `cout` is vital for a few reasons:
-
Feedback Mechanism: When developing applications, `cout` allows developers to receive immediate feedback from their code. This is especially useful for debugging, enabling them to verify the correctness of their program's logic and data.
-
User Interaction: `cout` facilitates direct interaction with the user, allowing them to see results and messages promptly.
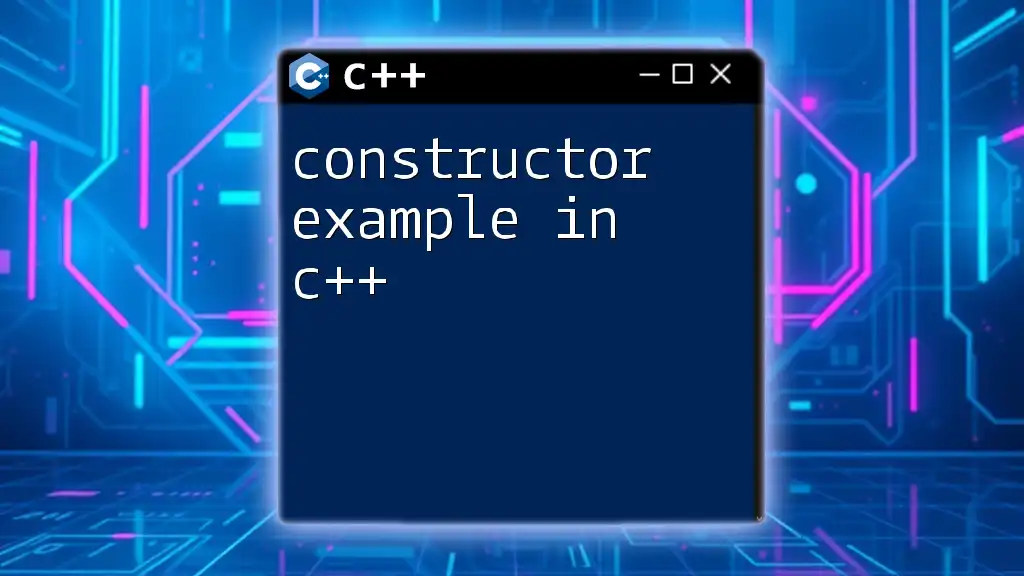
Understanding `cout`
What is a Stream?
In C++, a stream is a sequence of data elements that are made available for reading or writing. There are two primary types of streams:
-
Input Streams: Used for reading data from an input source (e.g., keyboard, file).
-
Output Streams: Used for sending data to an output destination (e.g., console, file).
The Role of `cout`
`cout` is an object of the `ostream` class, specifically defined in the `<iostream>` header. It is part of the C++ Standard Library and is often used together with the `std` namespace. Without understanding that `cout` belongs to this namespace, programmers may encounter errors while using it.
To use `cout` effectively, it's a common practice to include the line `using namespace std;`, which allows direct access to standard library features without prefixing them with `std::`.
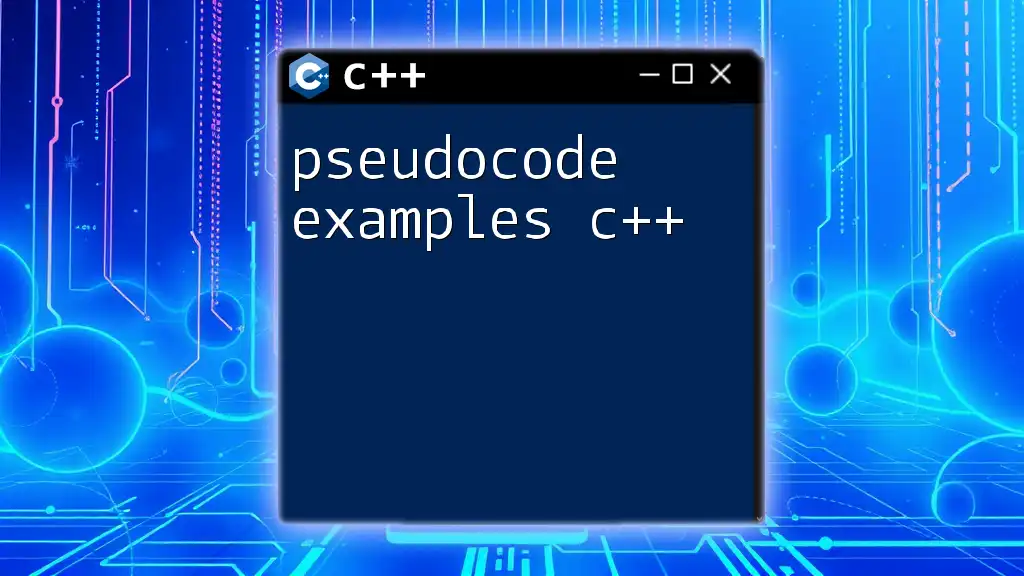
Basic Syntax of `cout`
Syntax Structure
The basic syntax of `cout` is straightforward:
std::cout << "message";
This line of code directs the string "message" to the standard output stream (typically the console).
Output Operators: The Arrow Operator (`<<`)
The left-shift operator (`<<`) is essential when working with `cout`. It enables the sending of data to the output stream.
For instance:
cout << "Hello, World!";
In this line, the string "Hello, World!" is passed to `cout` using the `<<` operator, resulting in the phrase being displayed on the console.
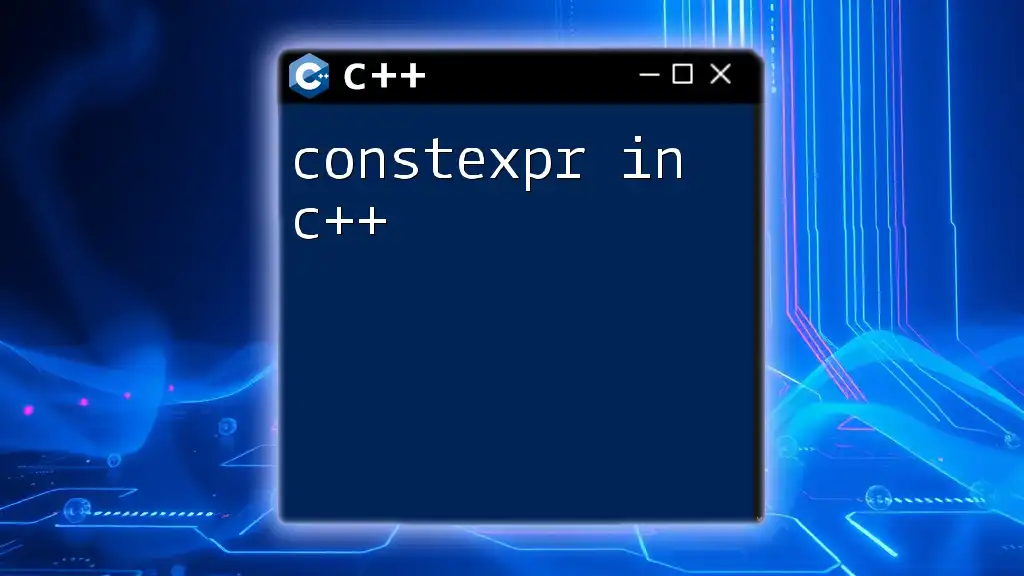
Simple Examples of `cout`
Example 1: Outputting a Simple String
Here's a basic example demonstrating how to use `cout` to display a string:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this program:
- We include the `<iostream>` header for `cout` to work.
- We use `cout` to output "Hello, World!" followed by `endl`, which adds a new line to the output and flushes the stream.
Example 2: Outputting Integer Values
Another example showcases how to output an integer variable alongside a string:
int number = 10;
cout << "The number is: " << number << endl;
This code outputs the string "The number is: " and then the value of `number`, resulting in the complete message being displayed.
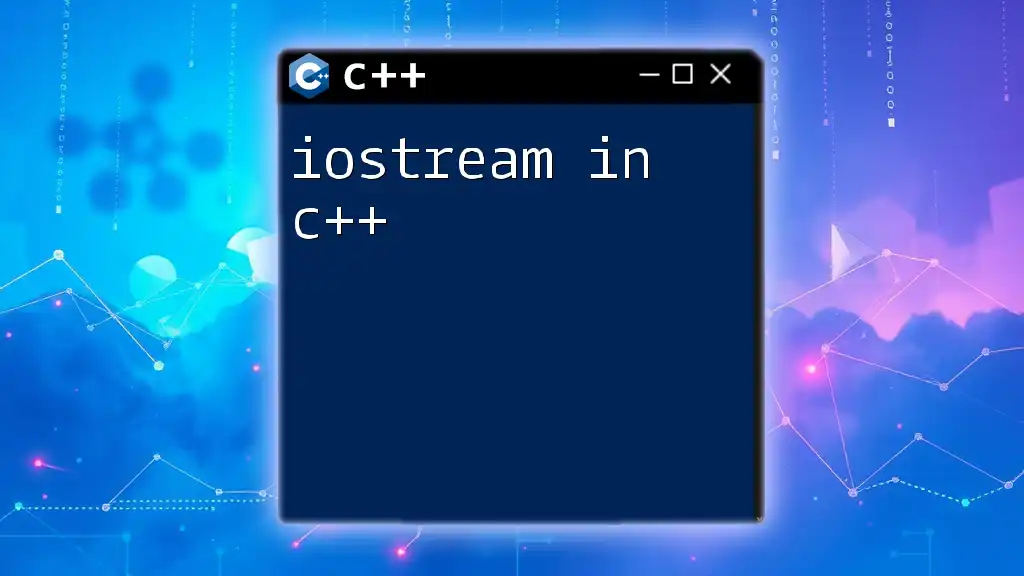
Formatting Output with `cout`
Using Escape Sequences
Escape sequences are special character sequences that allow for more versatile output formatting. Common escape sequences include:
- `\n` for newline
- `\t` for tab space
For example:
cout << "Hello,\nWorld!" << endl;
This command would beautify the output by placing "World!" on a new line.
Manipulators in C++
Manipulators are functions in C++ that can change the format of the output. Popular manipulators include:
- `endl`: Moves the cursor to a new line and flushes the output buffer.
- `setw`: Sets the width for the next output field.
- `fixed` and `setprecision`: Controls the output format of floating-point numbers.
An example demonstrating the use of a manipulator:
#include <iomanip>
cout << setw(10) << number << endl;
This line formats the output value by aligning it to the right within a 10-character width space.
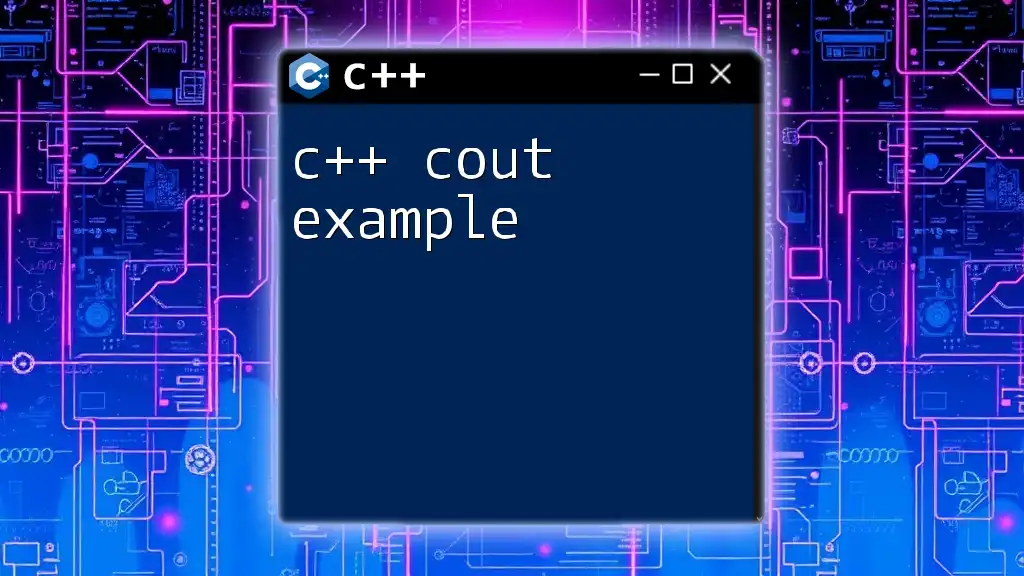
Advanced Usage of `cout`
Outputting Multiple Values
With `cout`, you can easily output multiple values in a single line using multiple `<<` operators. Here's an example:
float pi = 3.14;
cout << "Value of pi: " << pi << ", Just a number: " << 5 << endl;
This prints both the string "Value of pi:" and its respective float value, along with another number, all formatted in one statement.
Stream Manipulation
C++ offers several ways to control the format of the output further. You can change the precision of floating-point numbers or manipulate the output structure. Here's how you can utilize `setprecision` and `fixed`:
cout << fixed << setprecision(2) << pi << endl;
This example mentions that when we want to display `pi`, it will show two decimal places as "3.14".
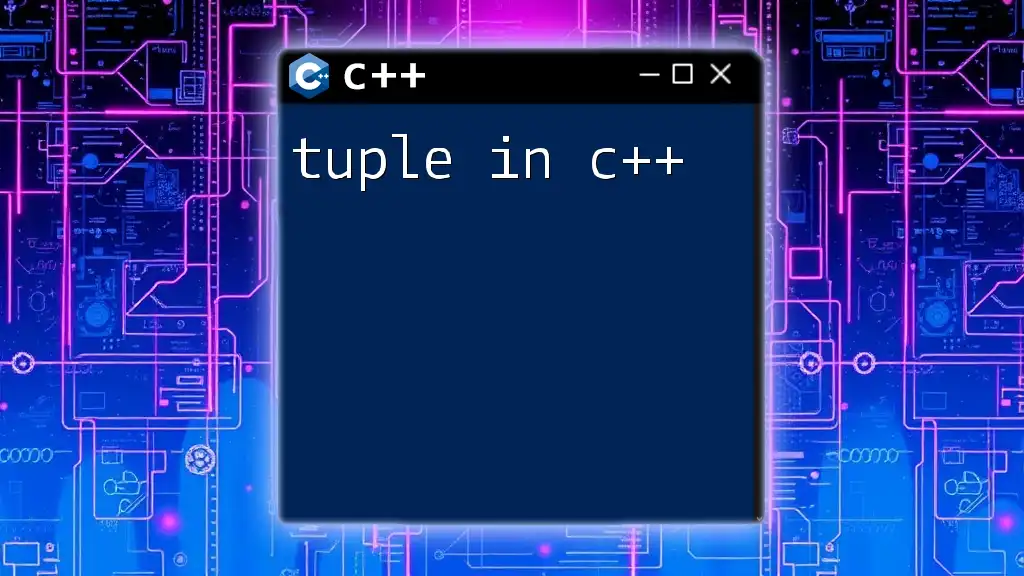
Common Mistakes to Avoid
Not Including Required Headers
Failing to include the `<iostream>` header can lead to compilation errors, as C++ will not recognize `cout`.
Forgetting the Namespace
When the `std` namespace is omitted, the compiler may also return an error stating that `cout` is undefined. Always remember to include `using namespace std;` or use `std::cout` directly.
Misusing the Output Operator
A frequent mistake is to misuse the `<<` operator, as shown in the following incorrect code:
cout << "Incorrect usage" >> endl; // Incorrect operator
The above code will result in a compilation error since `>>` is the wrong operator for output.
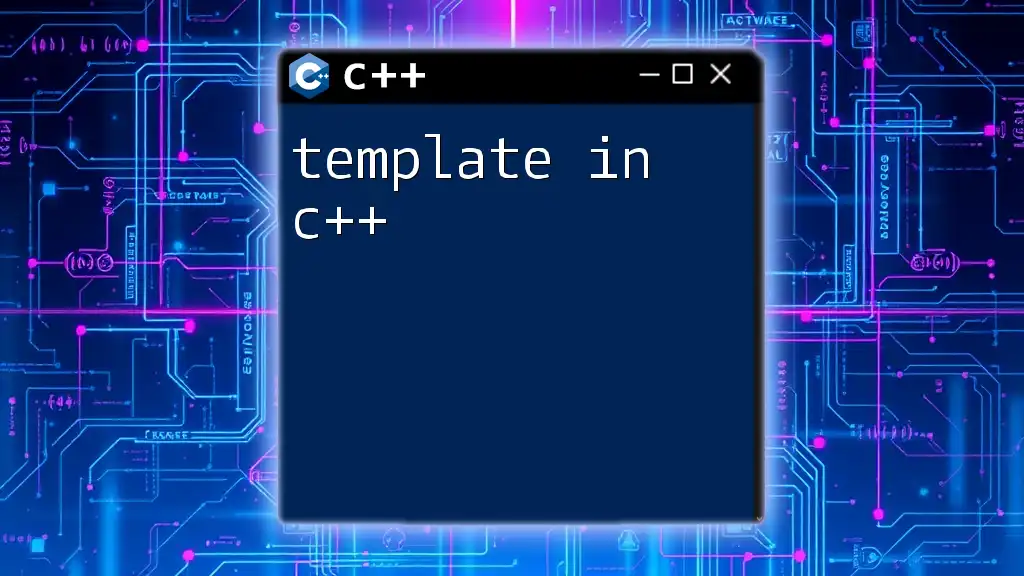
Summary of Key Points
The ability to use `cout` for displaying output in C++ is a fundamental skill that new programmers must acquire. From simple strings to formatted numerical output, mastering `cout` ensures that developers can effectively communicate information to users and diagnose their code through display features.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Encouraging Practice
To truly grasp the functionality of `cout`, it’s crucial to practice by implementing various examples and formatting options discussed in this guide. Explore further by using `cout` to transform your C++ coding experience.