Decompiling C++ involves converting compiled machine code back into human-readable source code, typically using a decompiler tool, although the resulting code may not match the original perfectly.
// Example of using a decompiler
// Suppose you have a binary file 'program.exe', you can decompile it using:
// (This is a conceptual command; use actual tools like Ghidra or IDA Pro)
ghidra-headless program.exe -decompile
What is Decompiling?
Decompiling is the process of converting a compiled binary (a file containing machine code) back into a higher-level programming language. In the context of C++, this means reversing the compilation process to produce a representation that resembles the original source code. Its primary use is in reverse engineering, allowing developers to analyze code behavior or recover lost source code, which can be especially beneficial for debugging or understanding legacy code.
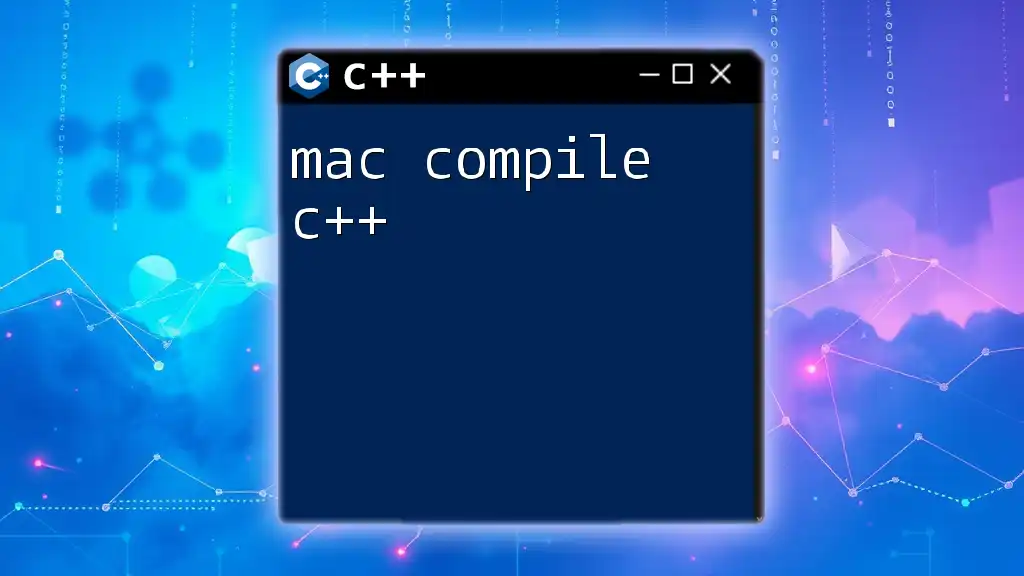
Why Use a C++ Decompiler?
Utilizing a C++ decompiler serves multiple purposes:
-
Analyzing Binaries: It helps in investigating compiled applications, allowing code analysis without access to the original source.
-
Recovering Lost Source Code: In instances where the original source code has been lost or corrupted, decompilation offers a pathway to retrieve usable code.
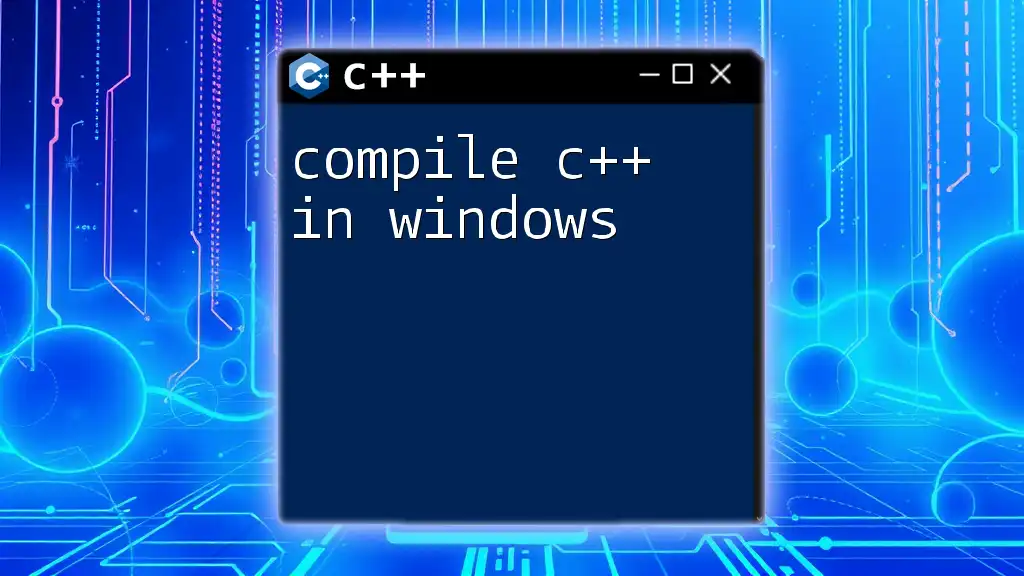
Understanding C++ Compilation
From Source Code to Binary
To appreciate decompilation, it's essential to grasp how C++ code is compiled. The process involves several stages:
-
Preprocessing: Handles directives such as `#include` and `#define`.
-
Compilation: Translates preprocessed code into assembly language.
-
Assembly: Converts assembly code into machine code (object files).
-
Linking: Combines object files into a single executable.
Key Terms and Concepts
-
Source Code: The human-readable code written by developers.
-
Object Code: The machine-readable output before final compilation.
-
Executable: The final product that the operating system can run.
-
Symbols: Identifiers like variable names and function names used in source code.
-
Debugging Information: Metadata embedded within executables that aid in debugging.
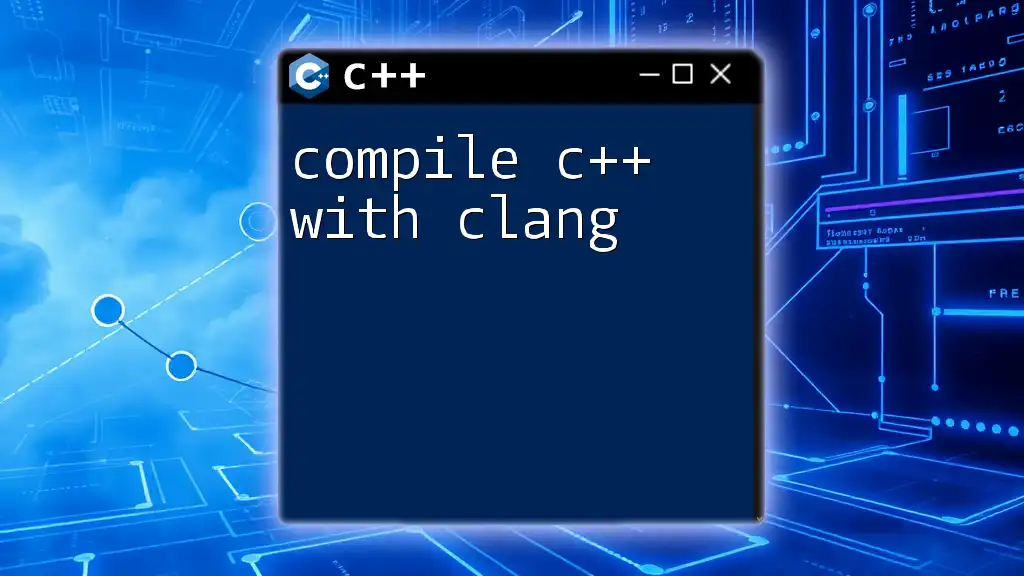
C++ Decompilers: An Overview
What is a C++ Decompiler?
A C++ decompiler is a specialized tool designed to analyze compiled C++ binaries and extract code that resembles the original source. Importantly, decompiling is distinct from disassembling, where disassemblers convert machine code into assembly language rather than a high-level language.
Popular C++ Decompilers
Several tools exist for C++ decompilation, each with unique features:
-
Ghidra: Developed by the NSA, Ghidra is a powerful and free tool used for reverse engineering. It features a modern interface and supports multiple file formats.
-
IDA Pro: A widely-used commercial decompiler that provides extensive features and an intuitive UI but can be quite expensive.
-
RetDec: An open-source decompiler that allows for web-based analysis of binaries as well as local usage, making it versatile.
Each of these tools has strengths and weaknesses, so your choice often depends on your specific needs and budget.
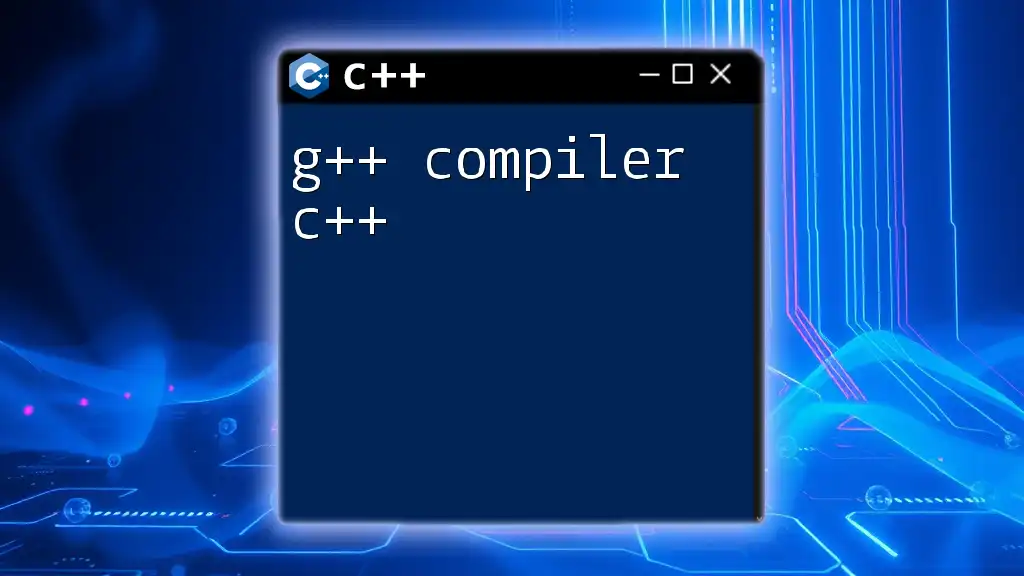
Getting Started with C++ Decompilation
Setting Up Your Environment
Before decompiling a binary, you need to set up an appropriate environment. The essential steps include:
-
Download a C++ Decompiler: Choose one from the previously mentioned options and download it.
-
Installation: Follow the installation instructions specific to the tool. This usually involves running an installer or extracting files.
Example Setup
Let’s say you choose Ghidra for its free access and feature set. The installation process and initial setup may look like this:
- Download the Ghidra package from its official website.
- Extract the downloaded file to your preferred directory.
- Launch Ghidra and set up your workspace, which provides a project area for your decompilation work.
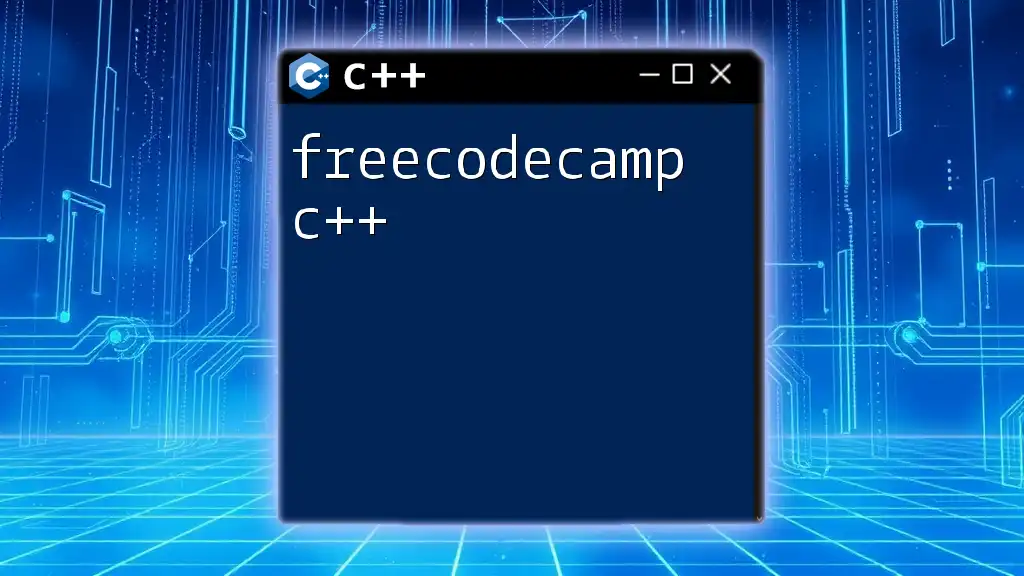
Decompilation Process Step-by-Step
Choosing Your Target Binary
Identifying the right binary for decompilation is crucial. Consider these points:
-
Executable Type: Make sure the file is indeed a compiled C++ application (.exe, .dll for Windows, or similar formats for Linux).
-
Versioning: Older binaries may yield better results as they might not employ modern obfuscation techniques.
Using a C++ Decompiler
Once the environment is set up, load the binary into the decompiler. The typical steps involve:
-
Load the Binary: Utilize the “File” menu to open the target executable.
-
Initial Analysis: Allow the decompiler to perform a preliminary analysis of the binary to identify functions and structures.
-
Navigate the UI: Familiarize yourself with the tool’s interface. In Ghidra, you’ll see function lists, and control flow graphs which represent how the binary functions.
Navigating Decompiled Code
Once the analysis is complete, you can explore the decompiled output. Decompiled code might not be perfectly readable but generally follows recognizable syntax.
For example, the following snippet may represent a decompiled function from the binary:
// Decompiled example function
int add(int a, int b) {
return a + b;
}
This example shows how the original functionality is preserved, enabling further analysis or reconstruction.
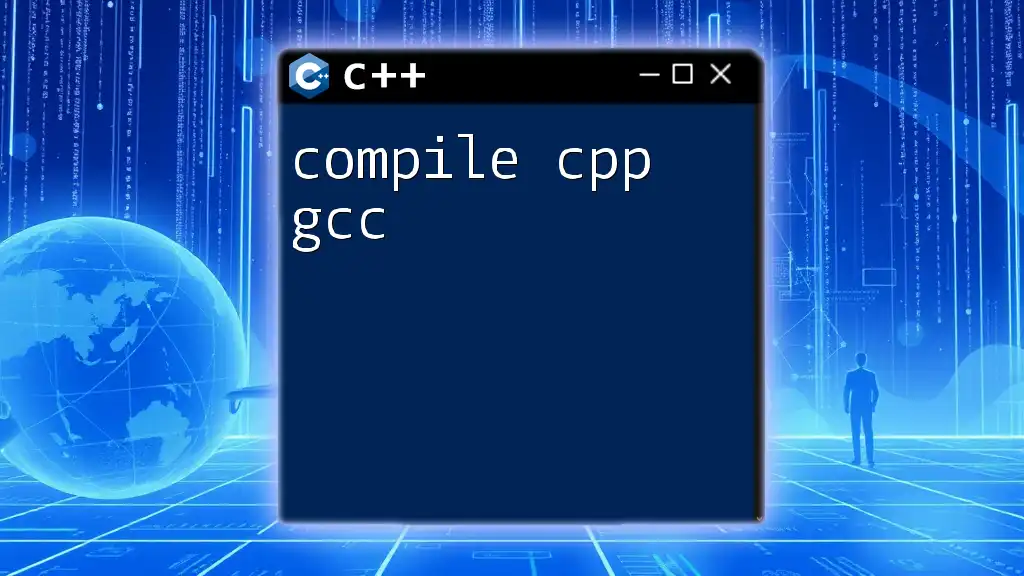
Interpreting Decompiled Output
Understanding Decompiled Code
In your decompiled output, you'll encounter various familiar C++ structures, including classes, functions, and conditionals. Understanding these structures and reconstructing the original logic often requires programming knowledge.
Common Challenges in Decompilation
The decompilation process is not without its challenges. Here are some typical issues:
-
Obfuscation: Many binaries use techniques to make reverse engineering more difficult, such as renaming variables and concealing logic.
-
Loss of Type Information: Compilers often optimize code, which can lead to missing type information in the decompiled output, making it hard to understand.
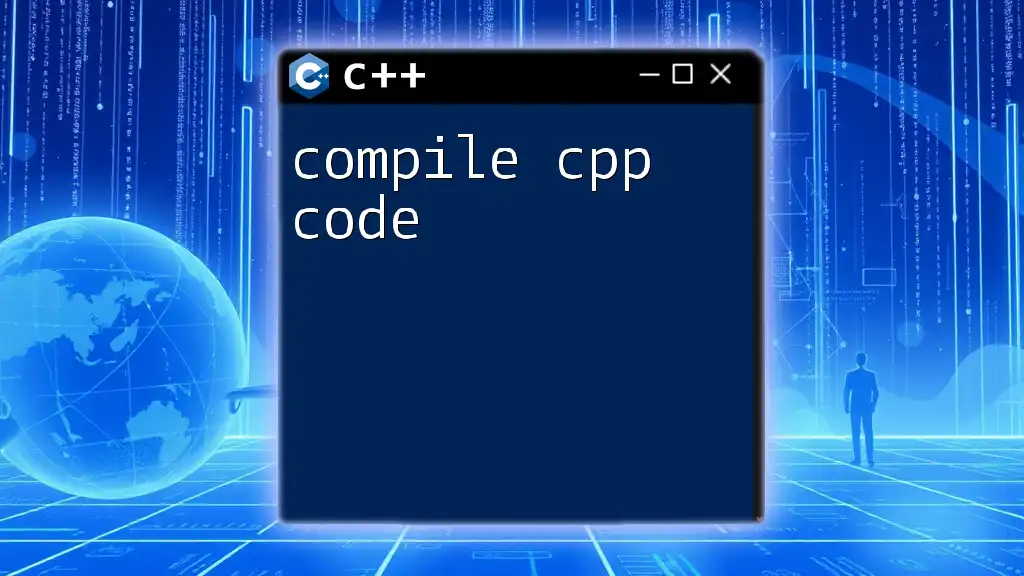
Advanced Techniques in Decompilation
Using Annotations and Comments
To improve the readability of decompiled code, leverage annotations:
-
Adding Comments: Use the decompiler’s commenting feature to provide explanations and insights about the code structure.
-
Creating Annotations: Highlight key sections of code with annotations to aid understanding for yourself or future users.
Manual Adjustment and Reconstruction
Sometimes, the decompiler output may require tweaks to resemble the original source better. Here’s how to manually enhance code:
A potential original function:
void processItems(std::vector<int>& items) {
for(auto& item : items) {
item *= 2; // Doubling each item
}
}
In your decompiled output, the logic might be scattered. You may need to adjust it manually for clarity by reintroducing variable names and structures that were stripped away during compilation.
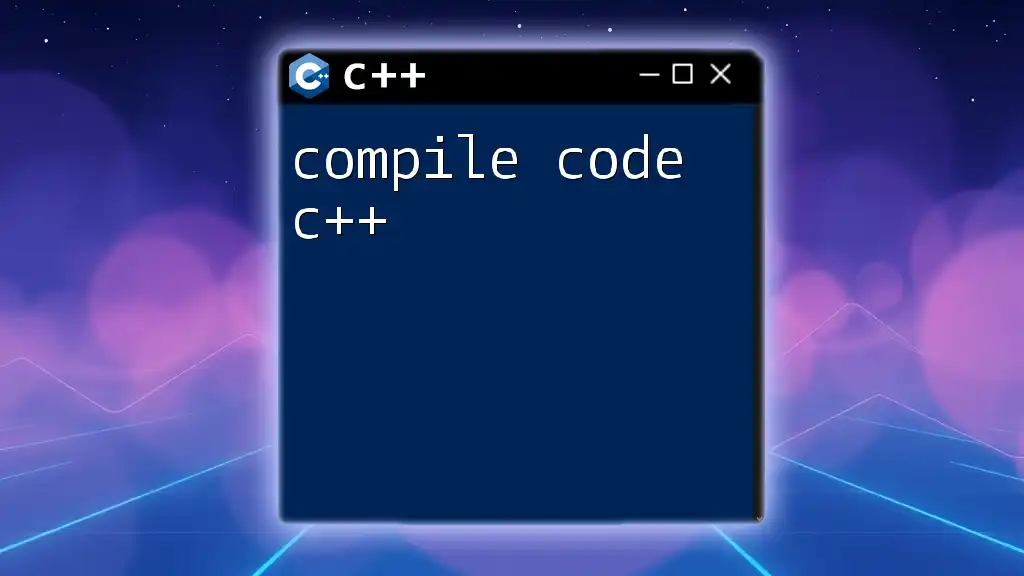
Ethical Considerations
Legal Aspects of Decompiling Software
It's vital to understand the legal ramifications of decompiling software. Generally, the legality varies based on:
-
Copyright Laws: In many jurisdictions, decompiling code without permission may constitute copyright infringement.
-
Notable Legal Cases: Familiarize yourself with landmark cases that shape current laws in this field. These can provide insight into acceptable practices.
Responsible Use of Decompiled Code
While decompilation can be a powerful tool, using it ethically is critical. Keep the following points in mind:
-
Follow Guidelines: Use decompiled code for educational purposes or security analysis only.
-
Attribution: If you share any modifications or insights derived from decompiled code, ensure you give appropriate credit and respect the original authors' rights.
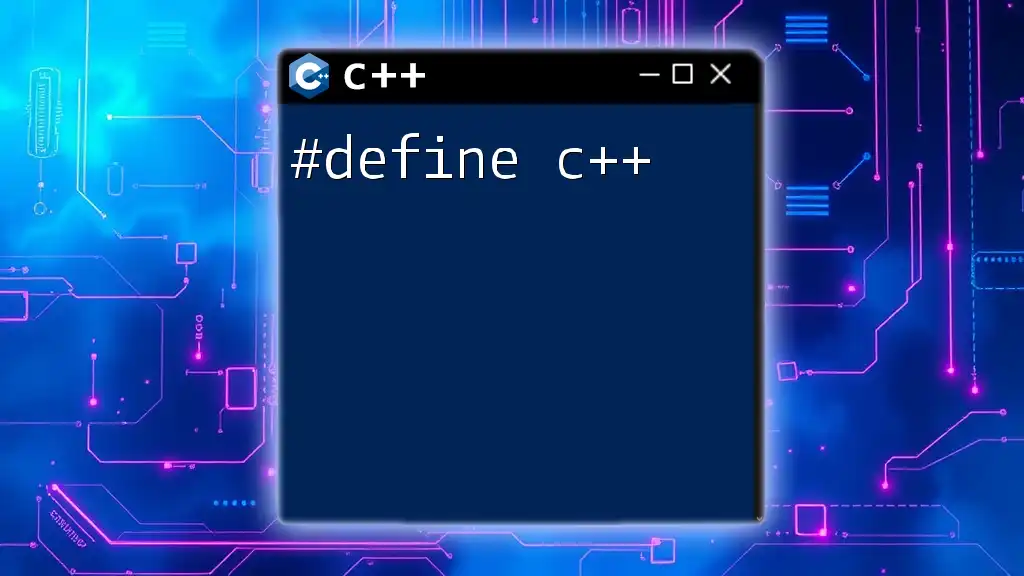
Conclusion
In summary, to decompile C++ code requires a blend of tools, knowledge of the compilation process, and understanding of the legal landscape. By leveraging C++ decompilers effectively, you can gain valuable insights into binary applications, recover lost source code, and analyze existing binaries. Remember to always follow ethical guidelines and best practices to respect the rights of original creators.
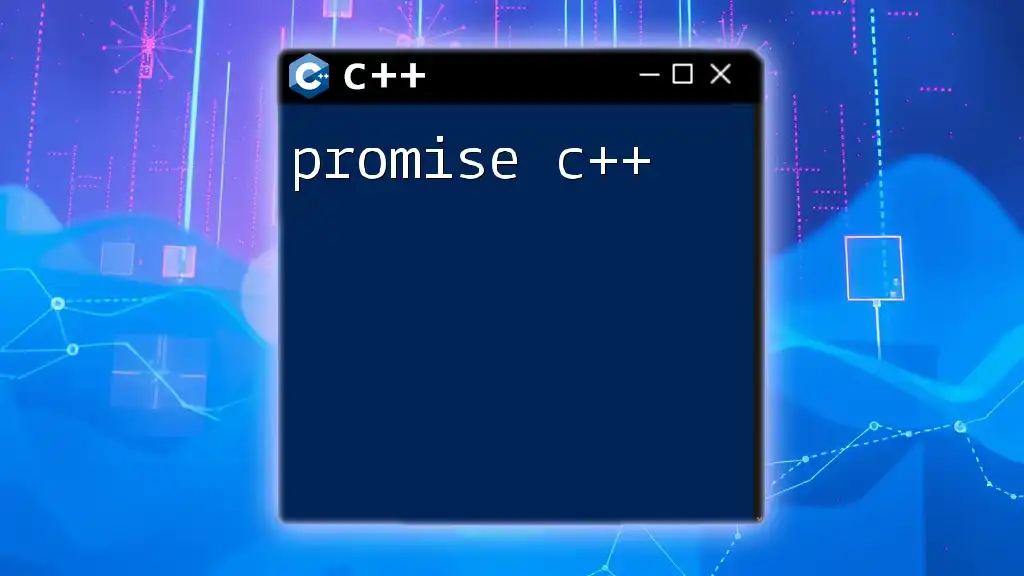
Frequently Asked Questions (FAQs)
Can I decompile any C++ executable?
Not necessarily. Legally, you should only decompile software you own or have explicit permission to analyze. Additionally, compiled code that is highly optimized or obfuscated may yield less intelligible results.
What are the limitations of C++ decompilers?
While decompilers can produce readable code, they cannot guarantee accuracy due to optimizations done during compilation. Furthermore, certain constructs may be irreversible, leading to challenges in interpretation.
Is decompiling legal in my country?
Legality varies. Many countries allow decompilation for personal and educational use, but distribute or use decompiled material without permission may infringe on copyright laws. Always consult legal advice specific to your jurisdiction.