To compile a C++ program using the GCC (GNU Compiler Collection), you can use the following command in your terminal, replacing `filename.cpp` with the name of your source file.
g++ filename.cpp -o output
This command compiles `filename.cpp` and creates an executable named `output`.
Understanding C++ Compilation
Why Compile C++ Code?
Compiling C++ code is crucial as it transforms your human-readable source code into machine-executable files. This process enhances the performance and efficiency of your software. Compilers play a vital role by interpreting your code structure, checking for errors, and finally outputting an executable that can run on your operating system.
What is GCC?
GCC, or the GNU Compiler Collection, is an evolving collection of compilers for various programming languages, including C++. Known for its robustness and versatility, GCC has been a staple in the development community since its inception. Some features that make GCC a preferred choice include:
- Cross-platform capabilities: GCC runs on different operating systems, making it a versatile tool for developers.
- Open-source nature: This allows users to modify and contribute to the codebase, leading to continuous improvements.
- Comprehensive error reporting: GCC provides detailed error messages, making debugging easier.
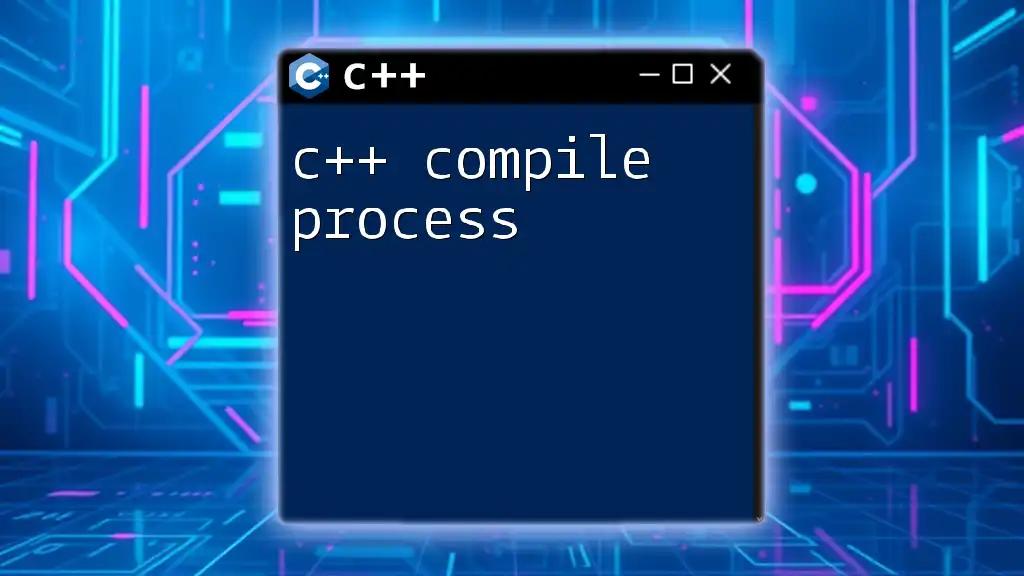
Setting Up GCC for C++
Installing GCC
Before you can compile C++ code with GCC, you need to ensure that it is installed on your system. Below are the instructions for various operating systems.
For Linux: You can install GCC using your package manager. For example, on Ubuntu or Debian-based systems, run:
sudo apt-get install g++
For macOS: If you're using Homebrew, the installation command is straightforward:
brew install gcc
For Windows: GCC can be installed via MinGW or Cygwin. For MinGW, you can download and install it from its official site, and during the installation, make sure to include the gcc component.
Verifying the Installation
After installation, you should confirm that GCC is correctly installed on your system. Open a terminal or command prompt and type:
g++ --version
This command will display the version number of GCC if it's installed correctly. Make sure to note down the version as it may be important for future compatibility.
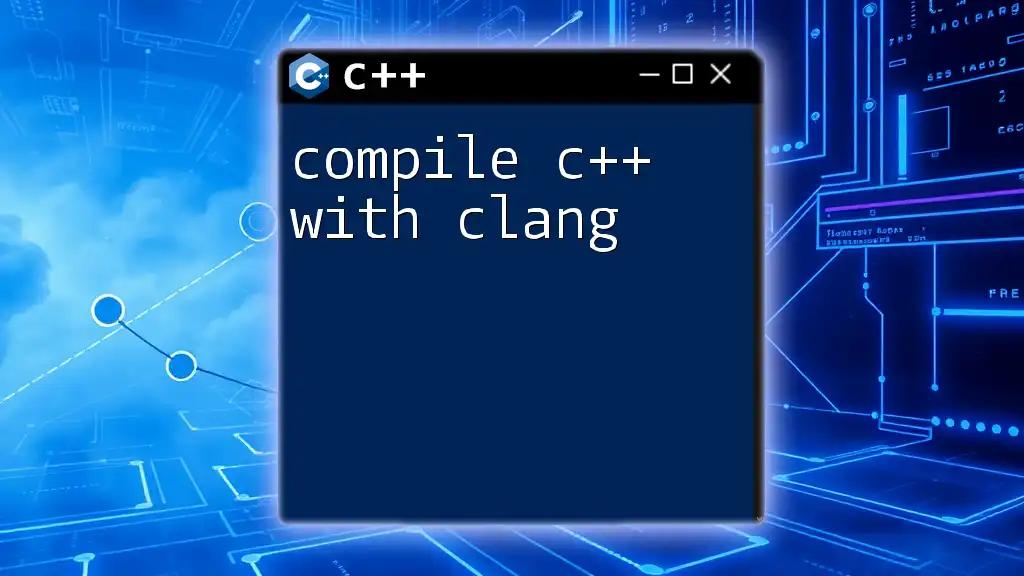
Using GCC to Compile C++
The Basic Compilation Command
To compile C++ code using GCC, you'll use a straightforward command structure:
g++ <source-file>.cpp -o <output-file>
- `<source-file>.cpp` is the name of your C++ file.
- `<output-file>` is the desired name for your compiled executable.
Compiling a Simple C++ Program
Let's consider a simple "Hello, World!" program. Create a file named `hello.cpp` and add the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this program, navigate to the directory containing `hello.cpp` and run the command:
g++ hello.cpp -o hello
Upon successful compilation, you can execute your program by running:
./hello
You should see the output: Hello, World!
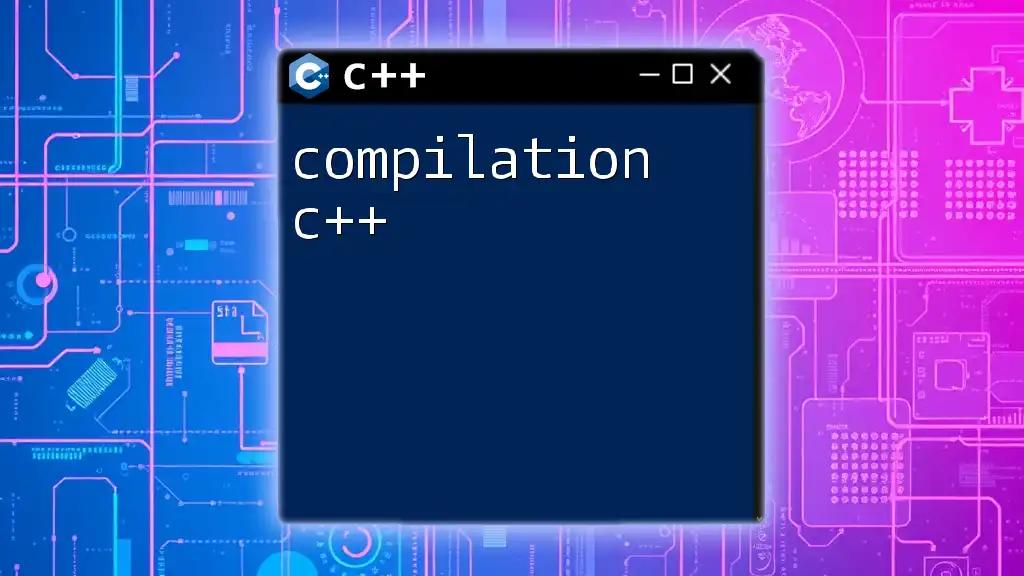
Advanced Compilation Options with GCC
Compiler Flags and Options
GCC offers numerous flags that enhance the compilation process. Here are some of the most commonly used flags:
- `-g`: This option generates debug information, aiding you during the debugging process.
- `-Wall`: By enabling all compiler warning messages, you can catch potential issues early.
- `-O2`: This optimization flag improves the code performance while keeping the compilation time relatively short.
Example of Using Flags
When compiling your "Hello, World!" program with these flags, your command will look like this:
g++ -g -Wall -O2 hello.cpp -o hello
Each flag serves a specific purpose. For example, enabling `-Wall` will alert you to any potential issues, which is especially helpful for beginners.
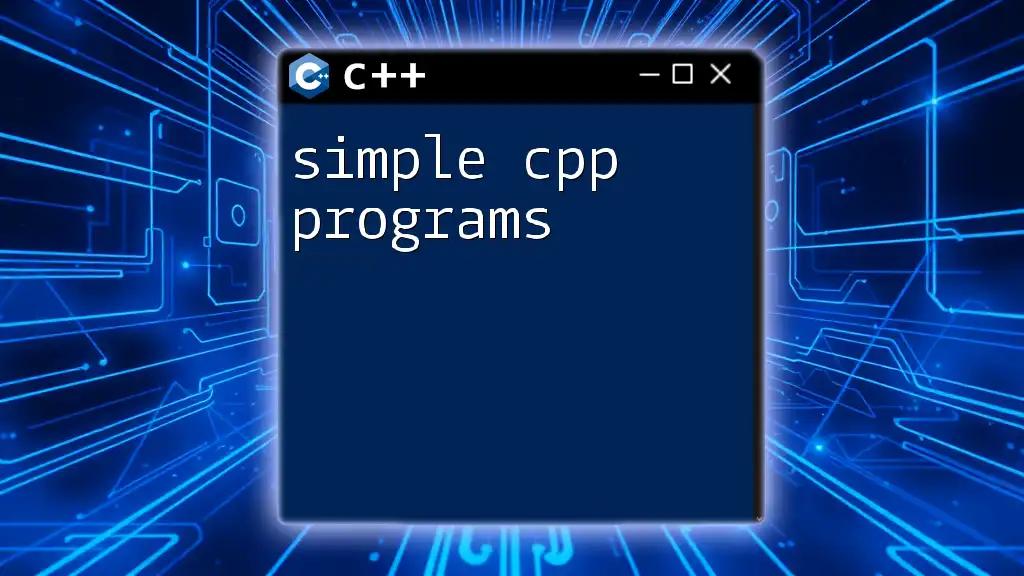
Error Handling and Debugging
Common Compilation Errors
Compiling C++ code is not always straightforward. Here are some common errors you might encounter:
- Syntax errors: Issues with code structure (e.g., missing semicolons).
- File not found: Occurs if the specified source file does not exist.
- Reference errors: This can happen when using undeclared variables.
When you encounter an error, GCC will provide a message indicating where the issue lies, allowing you to quickly troubleshoot it.
Debugging C++ Code with GCC
For those who need to investigate runtime issues, using GDB (GNU Debugger) can be invaluable. After compiling with the `-g` flag, you can start GDB with your compiled program like this:
gdb hello
Once inside GDB, you can set breakpoints and step through the code with commands such as:
- `break <function_name>`: Set a breakpoint at a specified function.
- `run`: Start the program.
- `next`: Execute the next line of code.
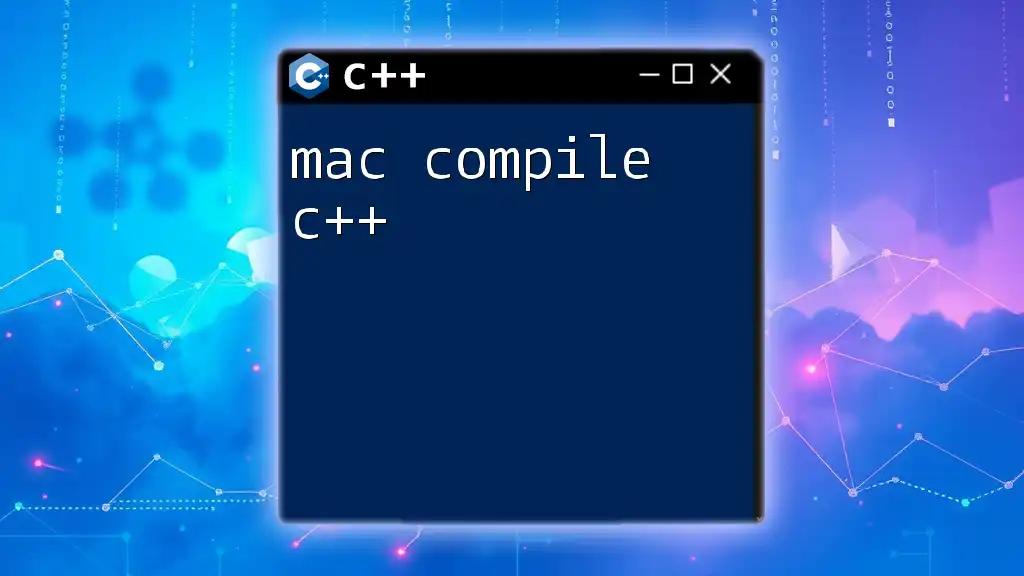
Making Executables with GCC
Cross-Platform Compilation
Compiling C++ code for different platforms requires understanding how linking works. You can create platform-agnostic code by avoiding system-specific calls and using static or dynamic linking to share libraries.
Creating Makefiles for Larger Projects
For larger projects that include multiple source files, using a Makefile simplifies the compilation process. A basic Makefile might look like this:
all: program
program: file1.o file2.o
g++ -o program file1.o file2.o
%.o: %.cpp
g++ -c $<
In this Makefile:
- The `all` target builds the project.
- The `program` target defines how to link object files into an executable.
- The `%.o: %.cpp` pattern defines how to compile `.cpp` files into object files.
To use this Makefile, simply run:
make
This command will automatically compile all necessary files based on the dependency structure you set.
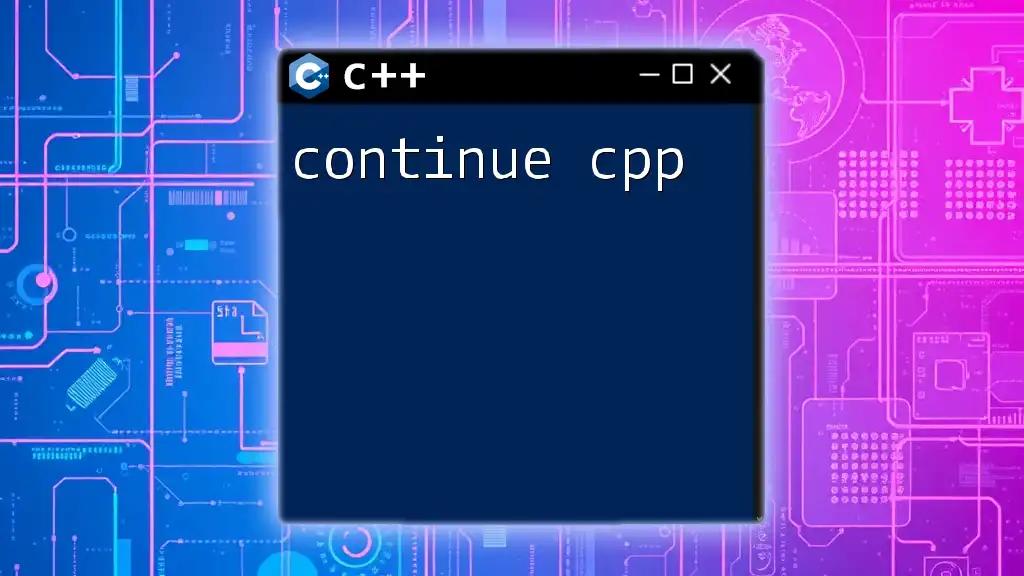
Conclusion
Understanding how to compile C++ code with GCC is foundational for any software developer working in C++. The information provided here should empower you to compile simple programs, tackle advanced features, and debug effectively. Regular practice will further deepen your understanding and proficiency with GCC. Don’t hesitate to explore additional resources and engage with the community for further learning.