To compile and run a C++ program using Code Runner in Visual Studio Code, you can simply open your `.cpp` file and use the shortcut (usually `Ctrl + Alt + N`) to execute the code. Here is a simple C++ program example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Code Runner
Installing Code Runner
To start leveraging Code Runner for compiling C++ code, the first step is to install the application. You can download Code Runner from the official website or your platform's app store. Before installation, ensure that your operating system meets the necessary requirements.
Follow these steps to install Code Runner:
- Download: Visit the official website and download the latest version compatible with your operating system.
- Install: Follow the on-screen instructions to complete the installation. For macOS users, drag the application to your Applications folder; Windows users will have standard installation prompts.
- Launch: After installation, open Code Runner to begin configuration.
Configuring Code Runner for C++
Before you can compile C++ code, you need to ensure that Code Runner is configured correctly for C++ development.
Setting Up C++ Compiler
The GNU Compiler Collection (GCC) is a popular choice for compiling C++ code. To configure it within Code Runner:
- Access Preferences: Open Code Runner and navigate to the settings/preferences menu.
- Select Compiler: Look for options related to languages or compilers. Ensure that the C++ compiler is set to GCC or any other compiler you wish to use.
- Path Configuration: Depending on your operating system, you might need to specify the path to the compiler's executable so that Code Runner can find it.
Customizing Preferences
Configuring user preferences can enhance your development experience. Consider the following:
- Theme and Appearance: Choose a light or dark theme based on your preference for visual comfort.
- Shortcuts: Familiarize yourself with keyboard shortcuts for faster coding. You can customize these shortcuts if needed using the preferences menu.
- Execution Commands: Set up execution commands specifically for C++ projects by specifying how you want your code to be run (e.g., `./your_program`).
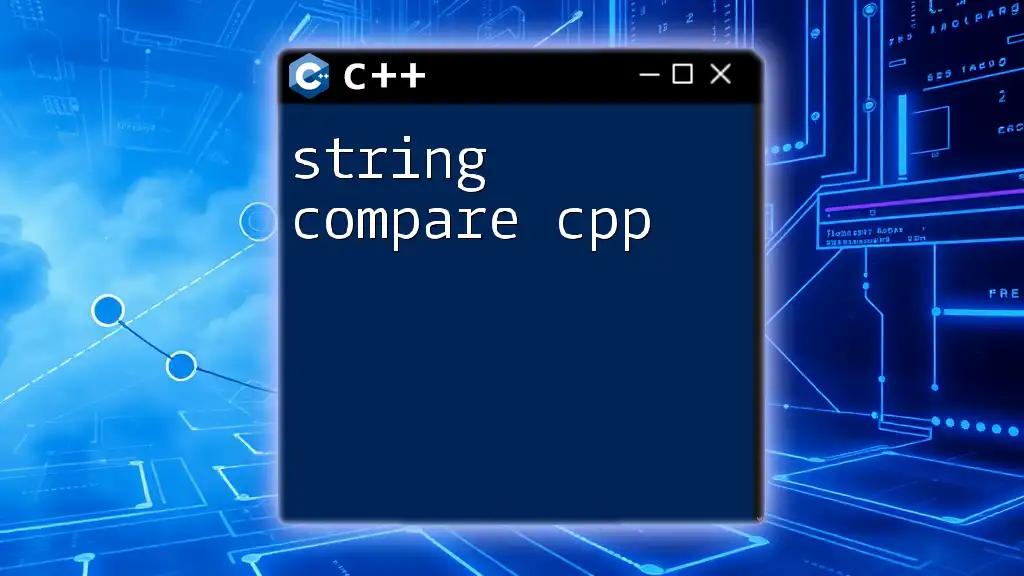
Writing Your First C++ Program
Creating a Simple C++ File
Within Code Runner, you can create and save C++ files easily. Begin by writing a simple program to familiarize yourself with the process.
Basic Structure of a C++ Program
Here’s the foundational structure of a C++ program to get you started:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example:
- `#include <iostream>`: This line allows you to use input and output streams.
- `int main() {...}`: The `main` function is where execution starts.
- `std::cout`: Outputs text to the console.
Saving Your C++ File
Make sure to save your C++ file with the correct extension `.cpp`. This helps Code Runner identify the file type and compile it appropriately. For project organization, consider using a dedicated folder structure such as `src` for source files and `bin` for compiled binaries.
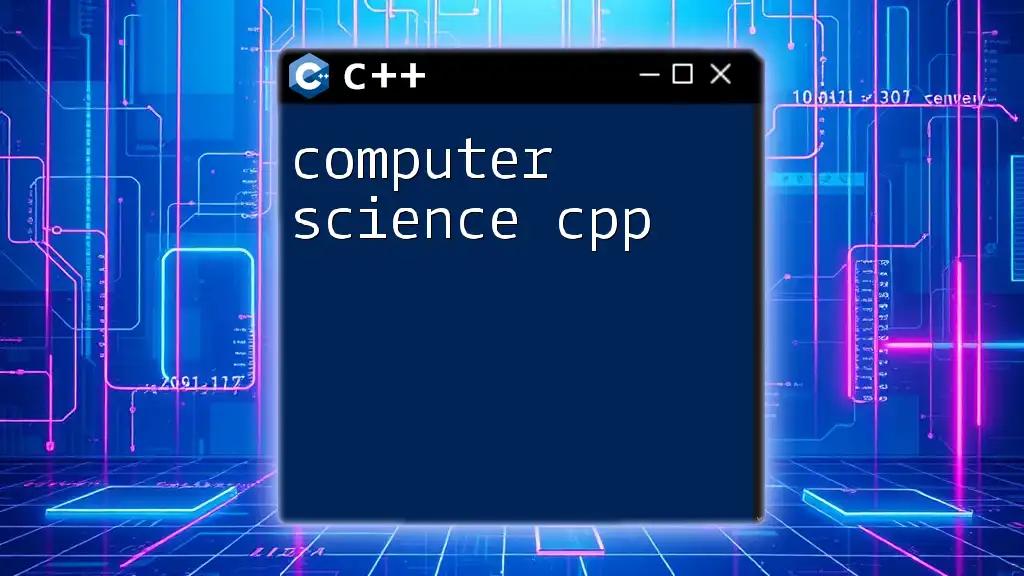
Compiling C++ Code in Code Runner
How to Compile
Compiling C++ code within Code Runner is simple and intuitive:
- With your C++ source file open, you can hit the play button (▶) typically located in the toolbar or use the keyboard shortcut (often Cmd + R on macOS or Ctrl + R on Windows).
- You should see compilation results and logs in the output console.
Understanding Compilation Errors
During the compilation process, you may encounter errors. Understanding how to troubleshoot these is essential for efficient coding.
Common Errors
-
Syntax Errors: This occurs when your code doesn’t follow C++ syntax rules. Common mistakes include missing semicolons or mismatched parentheses.
Example of a typical syntax error:
std::cout << "Error example" // Missing semicolon
-
Compilation Errors vs. Runtime Errors:
- Compilation Errors: These are errors detected when the code is converted into machine code. Fixing these errors ensures your code can compile.
- Runtime Errors: Occur during program execution and often relate to logic errors within the code.
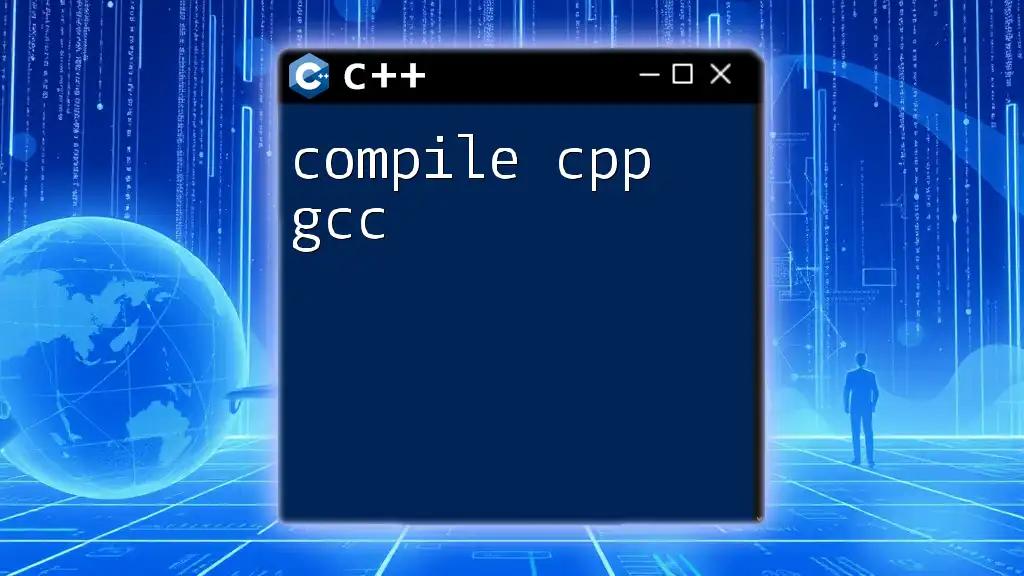
Running Your Compiled Program
Executing the Compiled Code
Once you have a successfully compiled C++ program, you can execute it directly from Code Runner. After compilation, simply click on the run button or use the designated shortcut again. You should see your program's output in the console.
Redirecting Input and Output
Code Runner also allows you to manage file input and output. For example, to run your program using input from a file and redirect output to another file, you can use command line redirection syntax.
./your_program < input.txt > output.txt
In the above command:
- `< input.txt` redirects the contents of `input.txt` as input to your program.
- `> output.txt` sends the output generated by your program to `output.txt`.
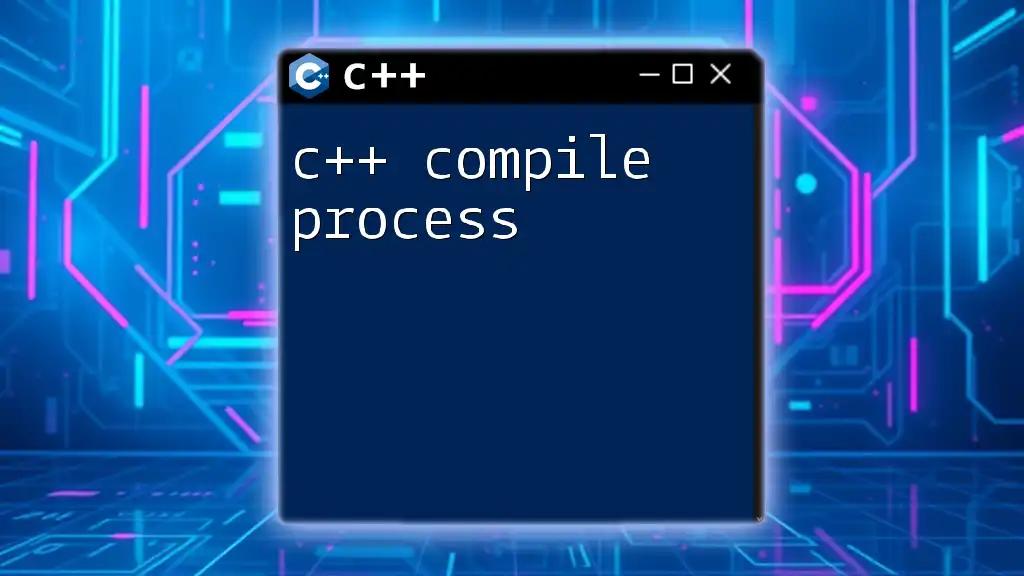
Advanced Features of Code Runner
Debugging Support
Debugging is a critical aspect of programming. Code Runner supports debugging C++ applications effectively, allowing you to find and correct issues in your code.
You can set breakpoints—specific points in your code where execution will pause—so you can inspect variables or evaluate expressions at runtime. Observing variable values while stepping through the code provides valuable insight into the program’s behavior.
Custom Build Commands
For more complex projects, you can create custom build commands:
- Define specific commands in preferences to handle unique compilation processes, such as linking libraries or passing specific flags to the compiler.
- Integration with version control systems, like Git, can be facilitated through such commands, ensuring your code maintains version history seamlessly.
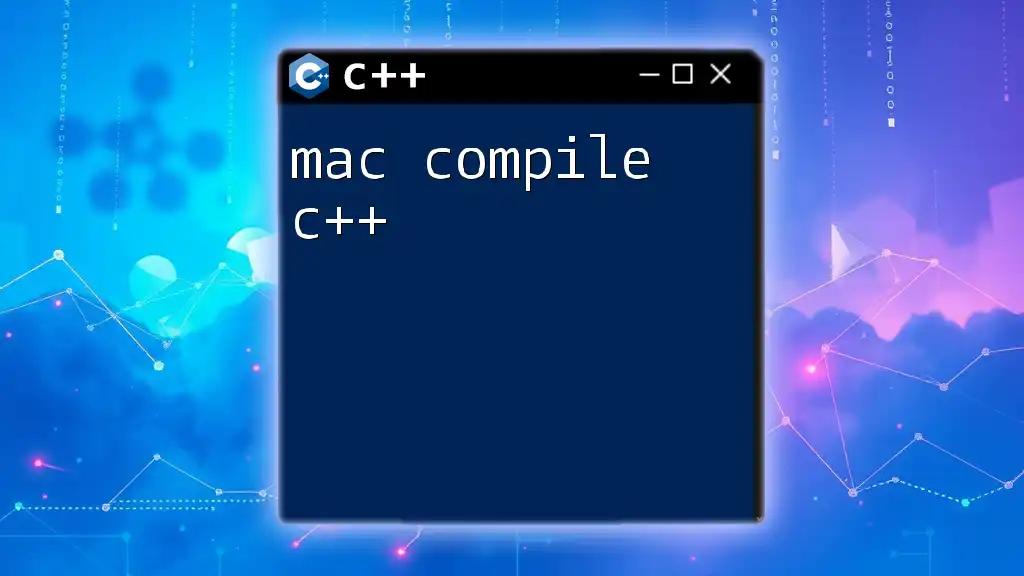
Best Practices for C++ Development with Code Runner
Code Organization Tips
Efficient code organization is vital for ease of maintenance. Use a consistent directory structure for projects and clearly comment on your code to improve readability. This practice makes it easier for you and others to understand the intent behind different sections.
Performance Optimization
Prior to compiling, examine your code for performance optimization. Leverage algorithms and data structures that enhance execution speed and efficiency. Testing and profiling can significantly reduce compile time and runtime.
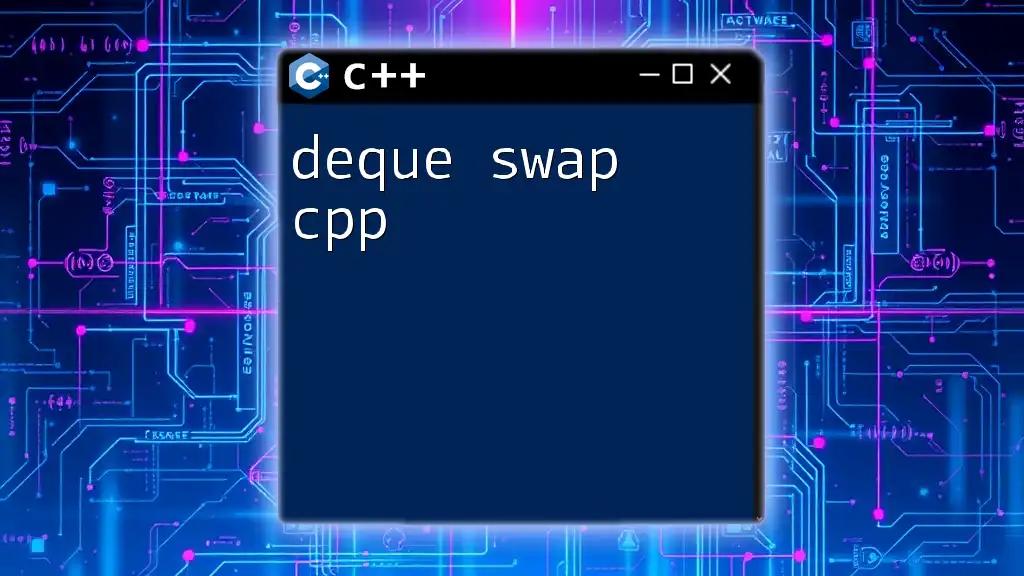
Troubleshooting Common Issues
Compilation or configuration issues may arise. Some common problems include:
- Configuration Problems: If Code Runner fails to compile, verify that the correct compiler path is specified in settings.
- File Not Found Errors: Ensure that all paths to include files or libraries are correct to avoid compilation failures.
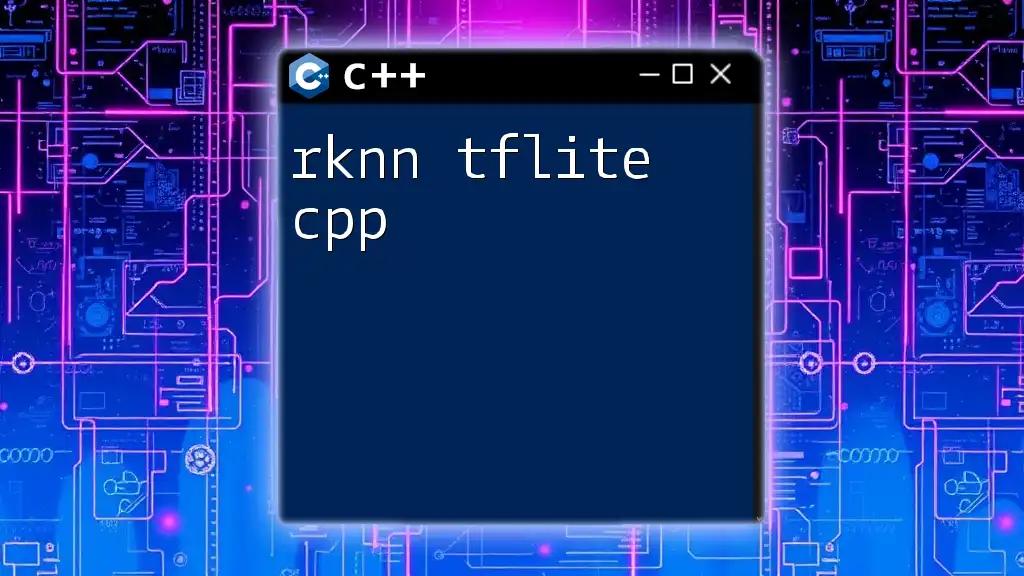
Conclusion
Efficiently compiling C++ code using Code Runner enhances your programming workflow, making it easier to test and debug applications. By understanding how to set up, compile, and run your C++ code, you can focus more on writing efficient algorithms and less on managing compilation intricacies. Jump into learning C++ further to unlock your programming potential and enhance your skills.
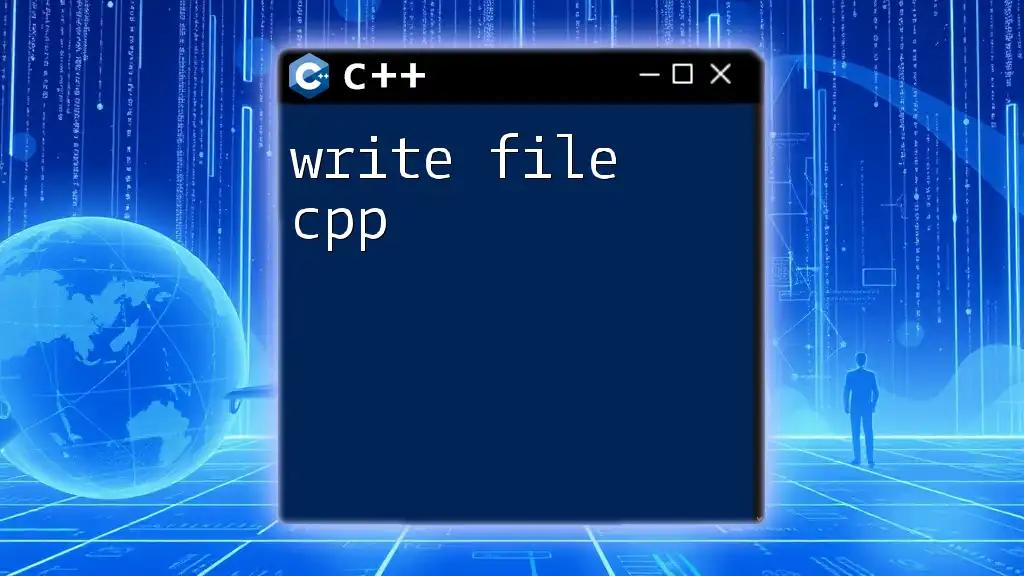
Resources
For additional assistance and advanced learning, you can explore the following resources:
- Official documentation for Code Runner and C++
- Recommended C++ programming books and online courses
- Online communities and forums where you can seek help and share knowledge on C++ development.
This guide should equip you with the foundational skills to leverage Code Runner for efficient C++ development. Happy coding!