The "rknn tflite cpp" refers to using the RKNN Toolkit to convert TensorFlow Lite models for inference in C++ applications on Rockchip devices.
Here’s a simple example of how to load a TensorFlow Lite model using RKNN in C++:
#include "rknn_api.h"
// Load an RKNN model and prepare for inference
rknn_context ctx;
rknn_init_ctx(&ctx, "model.rknn");
Understanding RKNN and TFLite
What is RKNN?
RKNN, which stands for Renesas Kernel Neural Network, is a machine learning framework specifically designed for deploying neural networks on edge and IoT devices. Its primary purpose is to facilitate efficient inference within constrained environments, allowing for real-time decision-making and reducing the reliance on cloud-based processing. Some key features of RKNN include:
- Lightweight Architecture: Optimized for limited resources typical of edge devices.
- Ease of Integration: Works seamlessly with various hardware platforms.
- Supports Common Models: Inherits compatibility with diverse popular neural network models trained via frameworks like TensorFlow.
RKNN is particularly beneficial in applications such as smart cameras, smart appliances, and even in industrial automation where responsiveness and efficiency are critical.
What is TensorFlow Lite?
TensorFlow Lite (TFLite) is a lightweight version of TensorFlow that enables the deployment of machine learning models on mobile and edge devices. Its main advantages lie in the following areas:
- Speed: TFLite is designed for fast inference on mobile CPUs, GPUs, and DSPs, ensuring smooth performance.
- Small Binary Size: The lightweight design of TFLite enables it to fit well within the storage and memory constraints of smaller devices.
- Model Optimization Support: TFLite offers support for quantization, model pruning, and other techniques that reduce the size and increase the inference speed of machine learning models.
The TFLite model conversion process is crucial as it allows developers to convert their trained TensorFlow models into a more digestible format suited for embedded devices.
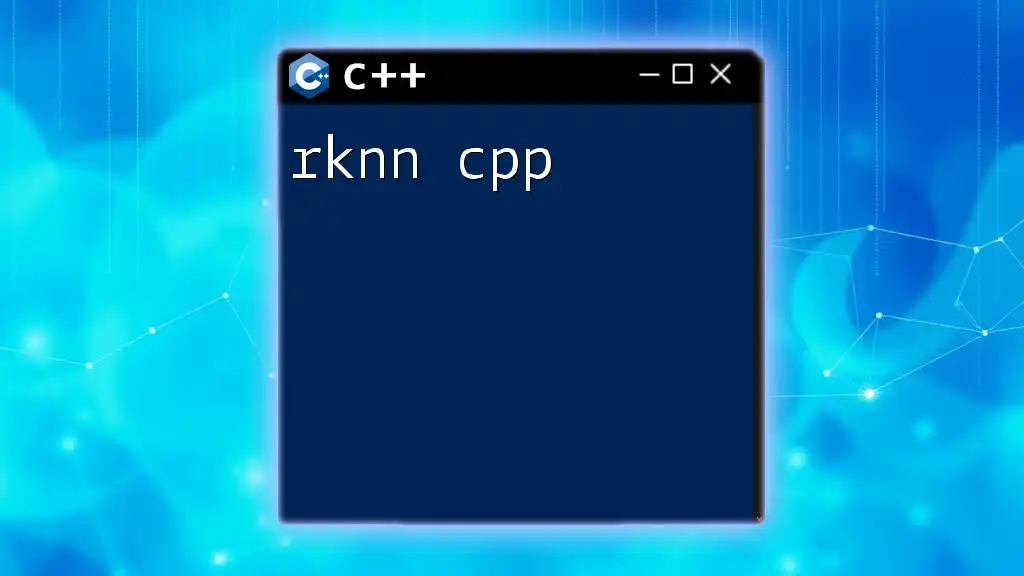
Setting Up Your Environment
Prerequisites
Before diving into RKNN and TFLite with C++, you need to ensure your development environment is properly set up. Here are the necessary software components:
- C++ Compiler: A compatible C++ compiler such as GCC or Clang.
- Integrated Development Environment (IDE): While you can use any text editor, IDEs like Visual Studio, CLion, or Eclipse can enhance your productivity with debugging features.
You should also install the RKNN SDK and TensorFlow Lite. Here’s a brief guide to setting that up:
- Install TensorFlow: You can install TensorFlow via pip using the following command:
pip install tensorflow
- Download RKNN SDK: Visit the official website of Renesas and follow the instructions to download the SDK relevant to your platform.
Setting Up Your Project
Once you have the necessary tools in place, create a new C++ project in your selected IDE. Organize your project structure effectively to maintain clarity:
- Create directories for source files, headers, and resources.
- Include the necessary headers for RKNN and TFLite at the top of your code files.
Example of headers you might include:
#include <rknn_api.h>
#include "tensorflow/lite/model.h"
#include "tensorflow/lite/interpreter.h"
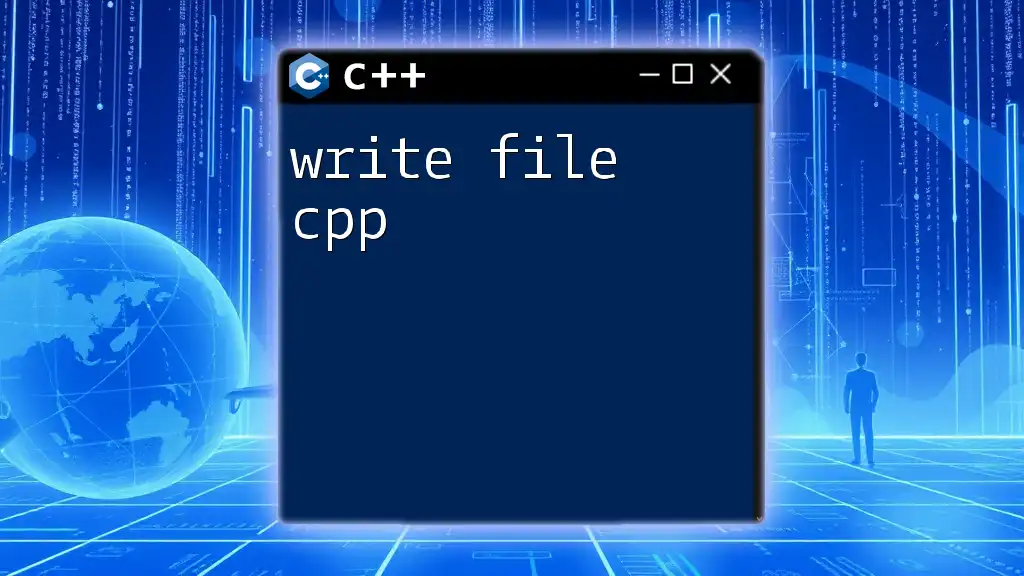
Converting a TensorFlow Model to TFLite
The Model Preparation Process
Before you can convert a TensorFlow model to TFLite, you need to make sure your model is ready. This involves:
- Training Your Model: Use TensorFlow to create and train your model, saving the checkpoints as required.
- Data Preprocessing: Ensure input data is prepared correctly for inference (normalizing, resizing, etc.).
Converting to TFLite
With your model prepared, the next step is conversion. You can convert your TensorFlow model to TFLite format using the TensorFlow Python API, as shown in the following snippet:
import tensorflow as tf
# Load the model
model = tf.keras.models.load_model('my_model.h5')
# Convert the model to TFLite
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
# Save the TFLite model
with open('my_model.tflite', 'wb') as f:
f.write(tflite_model)
Consider various conversion techniques, such as enabling optimization flags to improve the model's efficiency when running on edge devices.
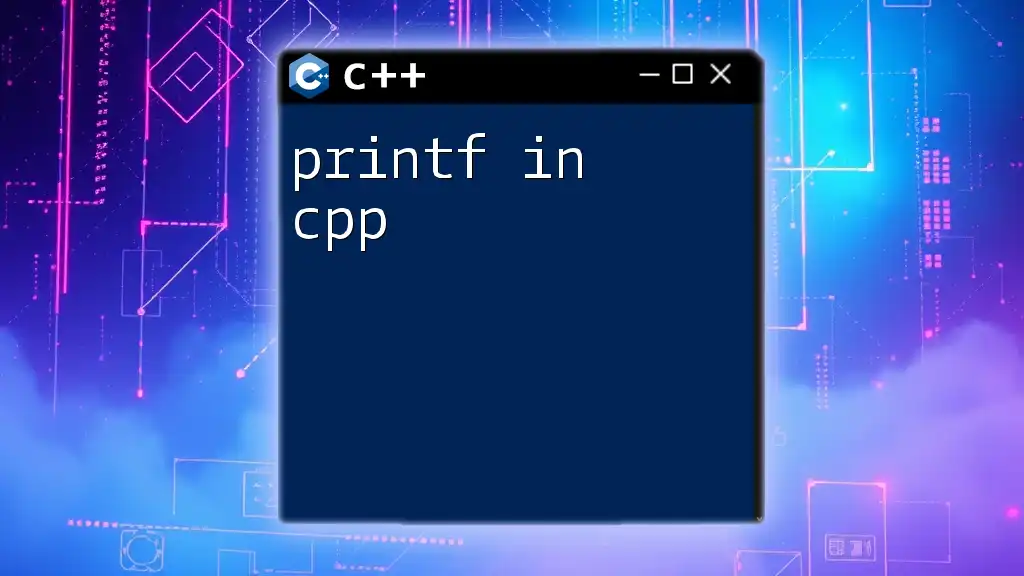
Integrating TFLite with RKNN in C++
Loading the TFLite Model
Now that you have converted your model to TFLite, the next step is to load this model into your C++ application. Utilize the TFLite C++ API to handle this task:
#include "tensorflow/lite/model.h"
// Load the model
std::unique_ptr<tflite::FlatBufferModel> model = tflite::FlatBufferModel::BuildFromFile("my_model.tflite");
if (!model) {
std::cerr << "Failed to load model" << std::endl;
return -1;
}
Running Inference with RKNN
The RKNN framework can then be employed to execute inference using the loaded model. Basic steps involved are initializing the RKNN context, setting inputs, running inference, and obtaining outputs:
// Create RKNN context
rknn_context ctx;
int ret = rknn_init(&ctx, ...);
if (ret < 0) {
std::cerr << "Failed to initialize RKNN context" << std::endl;
return ret;
}
// Set inputs for the model
rknn_inputs_set(ctx, ...);
// Run the model
rknn_run(ctx);
// Retrieve outputs
rknn_outputs_get(ctx, ...);
In this manner, developers can harness the power of both TFLite and RKNN, creating highly efficient applications suitable for a variety of edge computing scenarios.
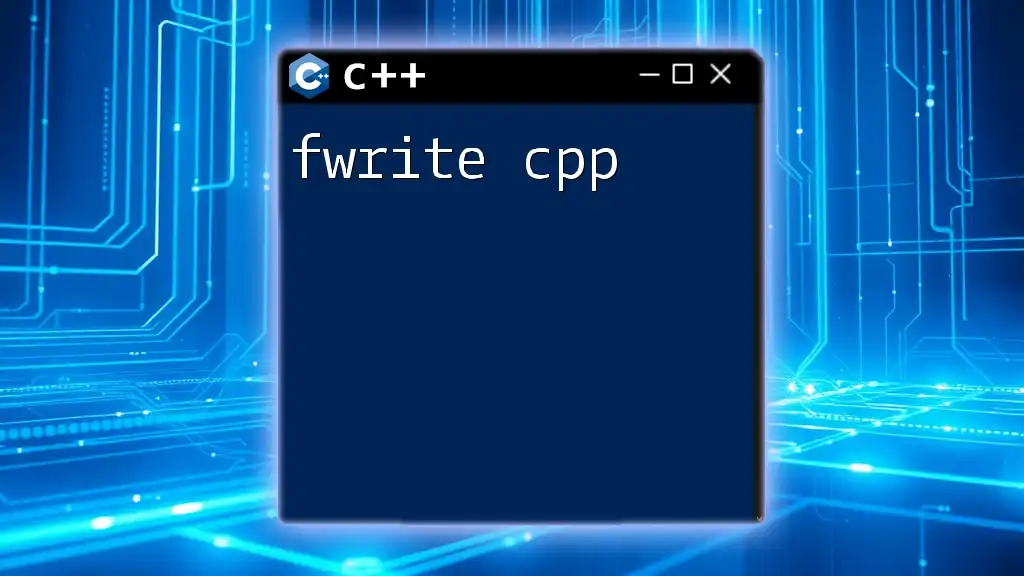
Common Issues and Troubleshooting
Debugging Challenges
As with any software engineering task, working with RKNN and TFLite in C++ can present challenges. Be vigilant for the following common pitfalls:
- Incompatible Model Formats: Ensure your TFLite model is generated according to the specifications expected by RKNN.
- Resource Limitations: Running a heavy model may exceed your device's memory or processing capabilities. Optimize your model accordingly.
Additionally, using logging and debugging tools integrated into your IDE can greatly simplify tracking down issues.
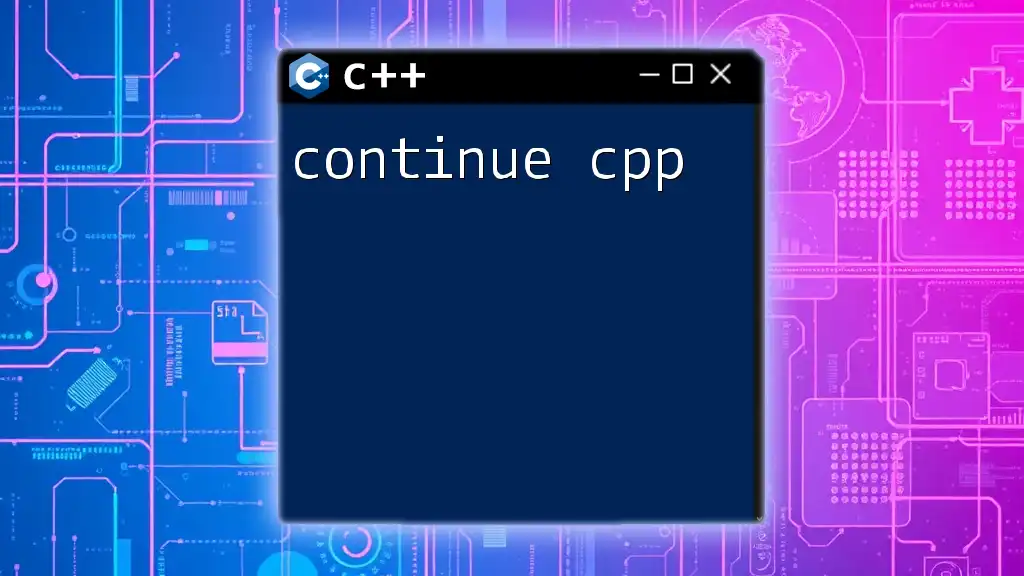
Advanced Topics
Model Optimization Techniques
Optimizing your model is essential for improving performance, especially when deploying on resource-constrained devices. Techniques to consider include:
- Quantization: Reduces model size and increases inference speed.
- Pruning: Involves removing non-essential neurons or weights.
- Layer Fusion: Merging layers can lead to fewer operations during inference.
Implementing these optimizations can significantly enhance performance without sacrificing accuracy.
Hardware Acceleration
Many modern devices come equipped with hardware acceleration options. Leveraging this capability can lead to massively improved inference times. To use hardware acceleration with RKNN, ensure:
- You are using a compatible device that supports GPU or TPU processing.
- Proper API calls are made in your code to direct the inference tasks to the correct hardware unit.
This can be configured within RKNN’s API while initializing the context and setting up the model.
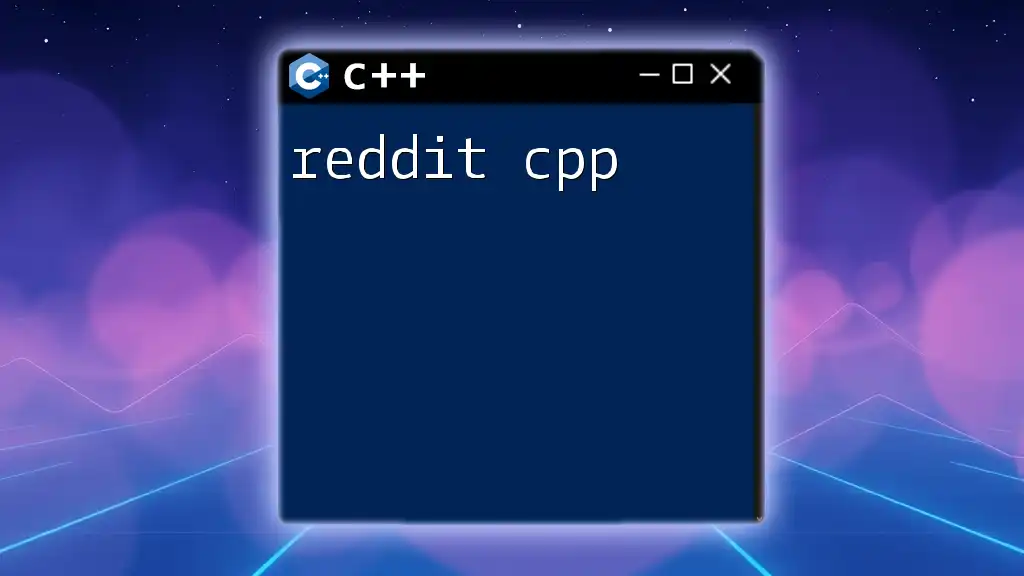
Conclusion
Understanding the intricacies of RKNN and TFLite in conjunction with C++ allows developers to significantly boost their machine learning applications, particularly in edge computing scenarios. With the detailed processes and code snippets outlined throughout this guide, you are well-equipped to explore these frameworks further. Dive into experimentation and enhance your skills, and don’t hesitate to reach out for more guidance to expedite your learning journey!
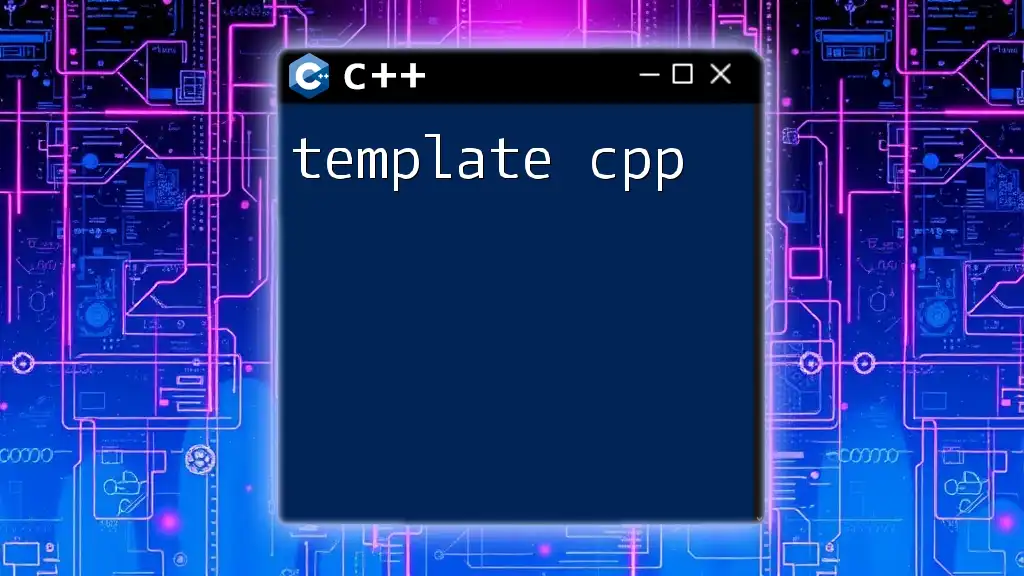
Additional Resources
For further development and insights in machine learning, consider those recommended resources:
- Official RKNN documentation
- TensorFlow Lite official documentation
- Online courses on machine learning and C++ development
By staying updated with the latest in technology and continuous practice, you can make substantial strides in your expertise with rknn tflite cpp!