Post Title: Quick Guide to Using Matplotlib in C++
In C++, the `matplotlibcpp` library allows you to easily create visualizations similar to Python's Matplotlib with minimal code, enabling quick data plotting.
#include <matplotlibcpp.h>
namespace plt = matplotlibcpp;
int main() {
// Sample data
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 4, 9, 16, 25};
// Plotting the data
plt::plot(x, y);
plt::title("Sample Plot");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
Getting Started with Matplotlib in C++
Before you dive into using matplotlib cpp, it’s crucial to ensure that you have the right setup.
Prerequisites for Installing Matplotlib in C++
To utilize `matplotlib cpp`, you should have a good command of C++ and basic knowledge of data visualization concepts. Furthermore, ensure you have Python and its package management tool, pip, installed since matplotlib is fundamentally a Python library.
Setting Up Your Development Environment
Recommended IDEs for C++
While you can use any C++ IDE, a few popular options include:
- Visual Studio: Excellent for Windows users.
- CLion: A powerful cross-platform IDE.
- Code::Blocks: Lightweight and user-friendly.
Installing Python and Matplotlib Library
- Download and install Python from the official Python website. Choose the latest version.
- After installing Python, open your command line tool and execute:
pip install matplotlib
Installing matplotlibcpp
To begin using matplotlib in C++, you need to install `matplotlibcpp`, a header-only C++ wrapper for the matplotlib library.
Steps to Clone matplotlibcpp
Open your terminal and run:
git clone https://github.com/lava/matplotlib-cpp.git
Building with CMake
Make sure you have CMake installed. Inside the `matplotlib-cpp` directory, create a build directory:
mkdir build
cd build
cmake ..
make
This builds the library from source, making it available for use in your C++ projects.
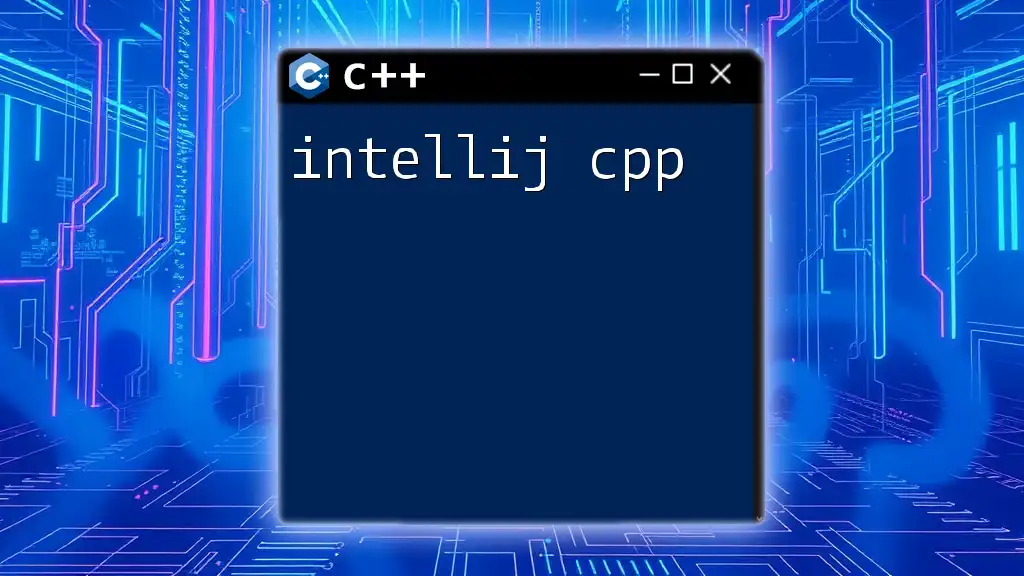
Basic Plotting with Matplotlibcpp
Once your setup is complete, you're ready to create plots.
Creating Your First Plot
Here is a simple example of generating a basic line plot:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 4, 9, 16, 25};
plt::plot(x, y);
plt::title("Basic Line Plot");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
In this code, we create two vectors representing the x and y coordinates, plot them, and then display the figure. Note how easily one can visualize data with just a few lines of code!
Customizing the Plot
To make your plots more informative, you can add titles, labels, and legends. Here’s an example:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 4, 9, 16, 25};
plt::plot(x, y, "r-"); // Red line
plt::title("Customized Line Plot");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::legend({"y = x^2"});
plt::show();
return 0;
}
Here, `"r-"` specifies a red solid line. Adding a legend provides clarity to what the plot represents.
Saving Plots to File
You might want to save your plots for later use. Here’s how to save in various formats like PNG or PDF:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 4, 9, 16, 25};
plt::plot(x, y);
plt::title("Saved Plot");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::save("plot.png"); // Saving as PNG
plt::show();
return 0;
}
In this example, use `plt::save("plot.png")` to save your plots directly to a file.
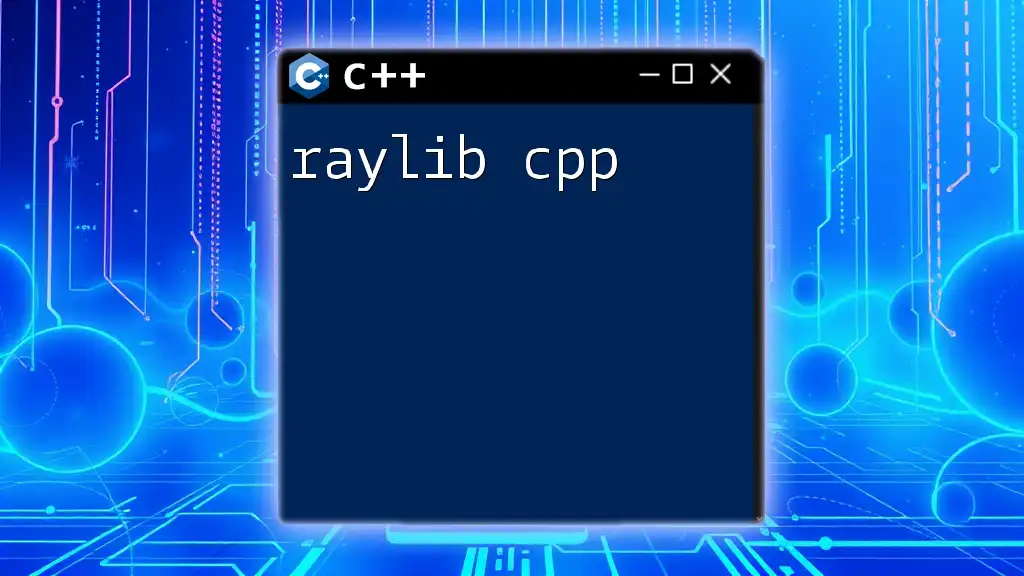
Advanced Plotting Techniques
Once you're comfortable with basic plots, you can explore more advanced techniques.
Scatter Plots
Scatter plots are beneficial for observing correlations between datasets. Here’s how to create one:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 3, 2, 5, 4};
plt::scatter(x, y);
plt::title("Scatter Plot Example");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
This code produces a scatter plot illustrating how certain values relate to one another.
Histograms
Histograms are essential for showing the distribution of data:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> data = {1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5};
plt::hist(data, 5); // 5 bins
plt::title("Histogram Example");
plt::xlabel("Data Values");
plt::ylabel("Frequency");
plt::show();
return 0;
}
This code will generate a histogram divided into five bins, allowing you to observe the distribution frequencies of your dataset.
Subplots and Layouts
Creating multiple plots in one figure is possible through subplots. This is handy for comparative analysis:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
plt::subplot(2, 1, 1); // 2 rows, 1 column, first plot
plt::plot({1, 2, 3}, {1, 4, 9});
plt::title("First Plot");
plt::subplot(2, 1, 2); // 2 rows, 1 column, second plot
plt::scatter({1, 2, 3}, {10, 20, 30});
plt::title("Second Plot");
plt::show();
return 0;
}
This example demonstrates how to effectively position multiple plots for better visual comparison.
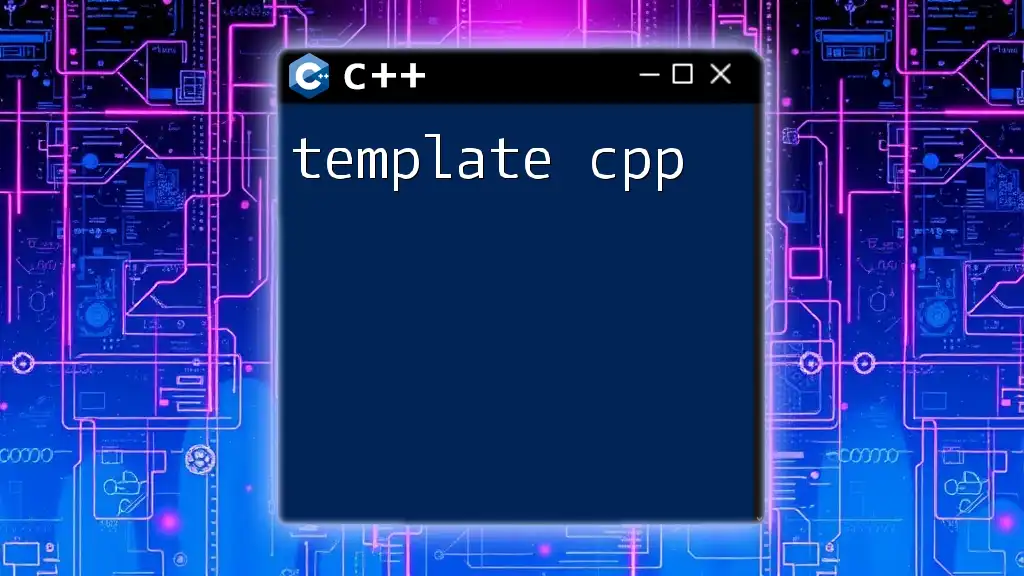
Integrating Matplotlib with Data Sources
Reading Data from CSV Files
Integrating `matplotlib cpp` with data from external sources can vastly improve analysis. Here's a simple way to read data from a CSV file:
#include <fstream>
#include <sstream>
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
void readCSV(const std::string &filename, std::vector<double> &x, std::vector<double> &y) {
std::ifstream file(filename);
std::string line;
while (std::getline(file, line)) {
std::stringstream ss(line);
double x_val, y_val;
ss >> x_val;
ss.ignore(1); // Ignore comma
ss >> y_val;
x.push_back(x_val);
y.push_back(y_val);
}
}
int main() {
std::vector<double> x, y;
readCSV("data.csv", x, y);
plt::plot(x, y);
plt::title("Data from CSV");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
This code snippet shows how to read data from a formatted CSV file into vectors that can then be plotted.
Using External Libraries
Using libraries such as Armadillo can optimize data handling. This is especially beneficial for large datasets.
#include <armadillo>
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
arma::mat data;
data.load("data.txt"); // Load data into Armadillo matrix
plt::plot(data.col(0), data.col(1)); // First column vs second column
plt::title("Data from Armadillo");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
Using Armadillo allows for robust numerical operations for the dataset before visualization.
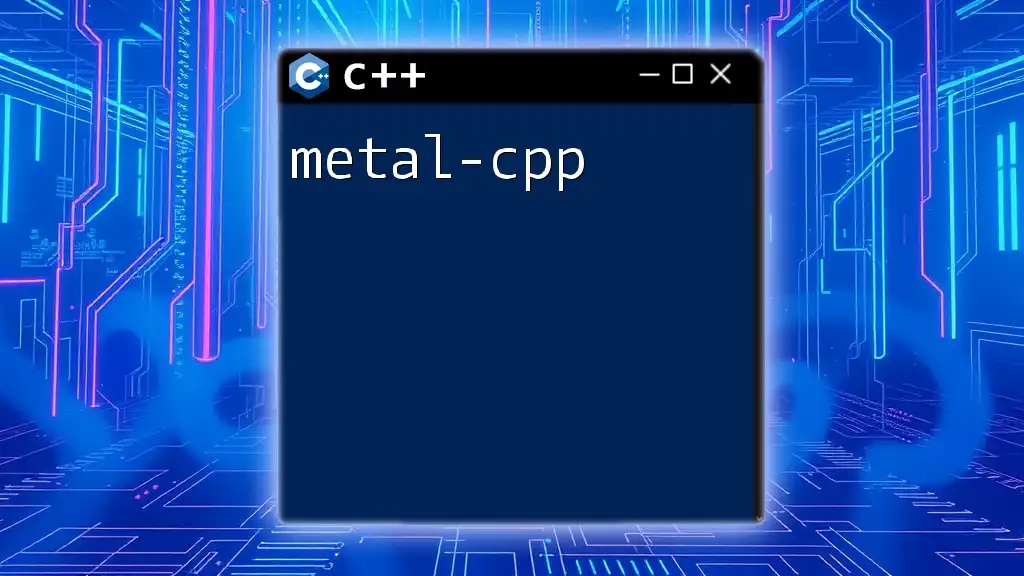
Customizing Aesthetics in Matplotlibcpp
Modifying Line Styles and Colors
Customizing plot appearance can enhance presentation. Here's how to change line styles and colors:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 4, 9, 16, 25};
plt::plot(x, y, "b--"); // Blue dashed line
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
The `"b--"` notation designates a blue dashed line. Such customizations enhance the visualization's clarity and appeal.
Annotations and Tooltips
Annotations provide context, adding value to your visualizations. Here’s how you might add annotations:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 4, 9, 16, 25};
plt::plot(x, y);
plt::annotate("Maximum", {4, 16}, {0, 1}); // Annotations
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
In this example, the `plt::annotate` function provides significant contextual information directly on the plot.
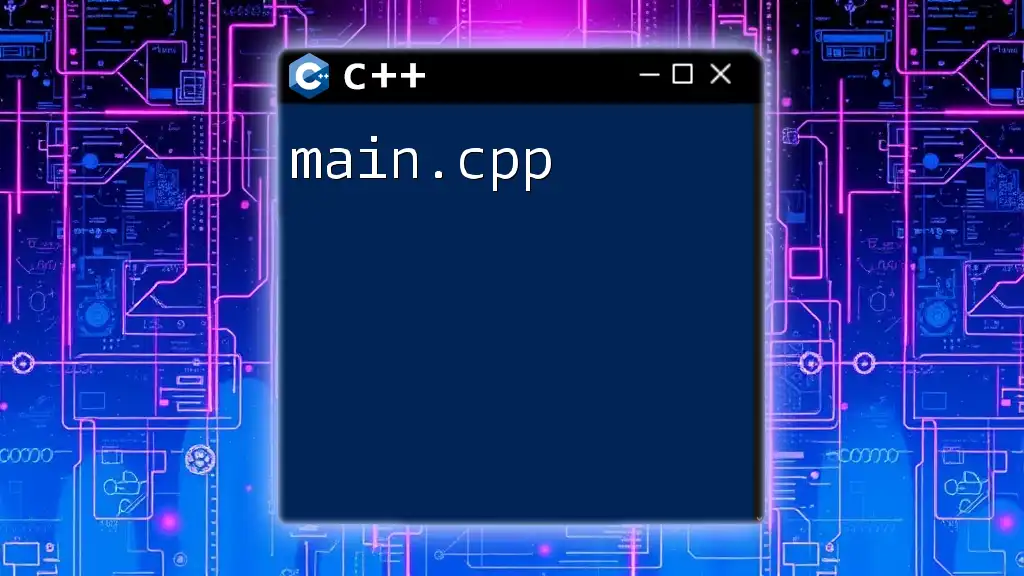
Common Pitfalls and Troubleshooting
Issues with Plot Rendering
Rendering issues may occur if the display back-end is not set correctly. Ensure that the backend is compatible with your operating system or try another backend if necessary. For instance, try switching to GTK or QT if you encounter issues.
Performance Optimization
When working with large datasets, performance can be affected. To solve this, consider using data compression or filtering techniques to reduce the amount of data processed at once. Additionally, leverage multi-threading wherever applicable, particularly during data retrieval or processing stages.
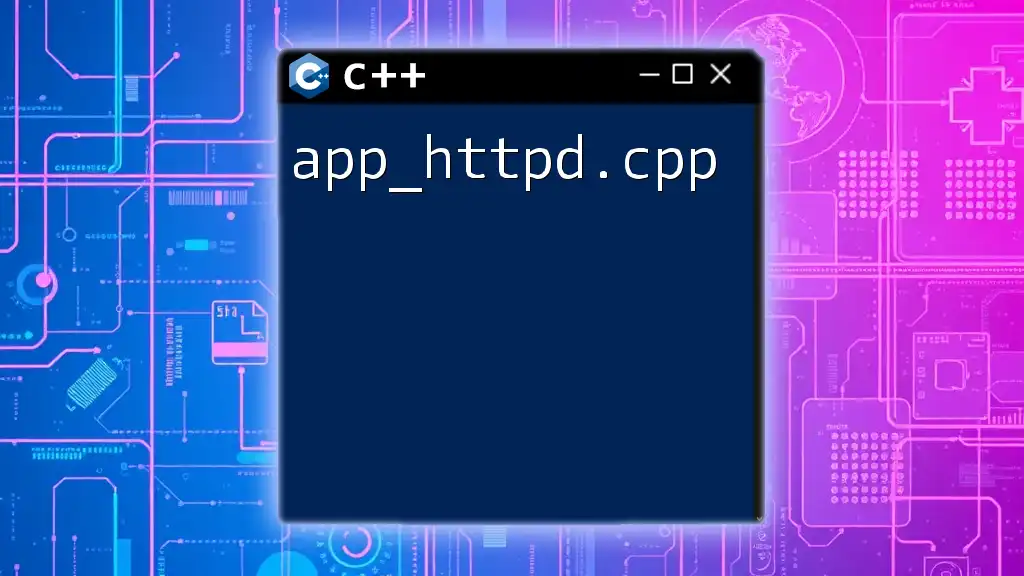
Best Practices for Data Visualization in C++
Choosing the Right Types of Visuals
Choosing the right type of visualization is crucial for data interpretation. Keep in mind:
- Use line plots for trends.
- Scatter plots are ideal for showing relationships.
- Histograms are good for distribution representation.
Maintaining Code Readability
It’s important that your C++ code remains clear and concise. Use meaningful variable names and appropriate comments to explain complex sections of your code. Structuring your code into functions, as demonstrated in previous sections, also aids readability.
Documenting Your Visualizations
Finally, documenting your visualizations is paramount. This means not only commenting in the code but also maintaining clear documentation outside the code for easy reference. Explain the data source, the type of analysis performed, and interpretations drawn from the visualizations.
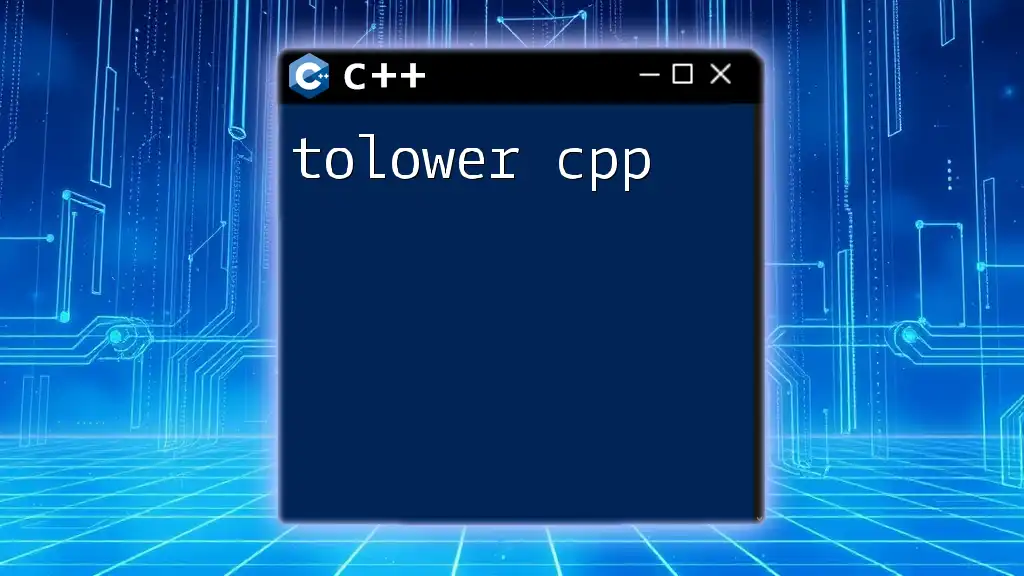
Conclusion
As you can see, utilizing matplotlib cpp opens the door to powerful data visualization directly within C++. Mastering this tool will enhance your analytical capabilities and enable more effective storytelling through your data.
The Future of Matplotlib in C++
With continual updates in both C++ and Python libraries, the potential applications of matplotlib cpp remain vast and largely untapped. Continuous practice and trend awareness will ensure you harness these advancements effectively.
Encouraging Continuous Learning and Practice
Stay curious and always explore new features and techniques within matplotlib cpp. Engage with communities, attend workshops, and share your findings. This will not only solidify your understanding but also keep you engaged with the ever-evolving tech landscape.
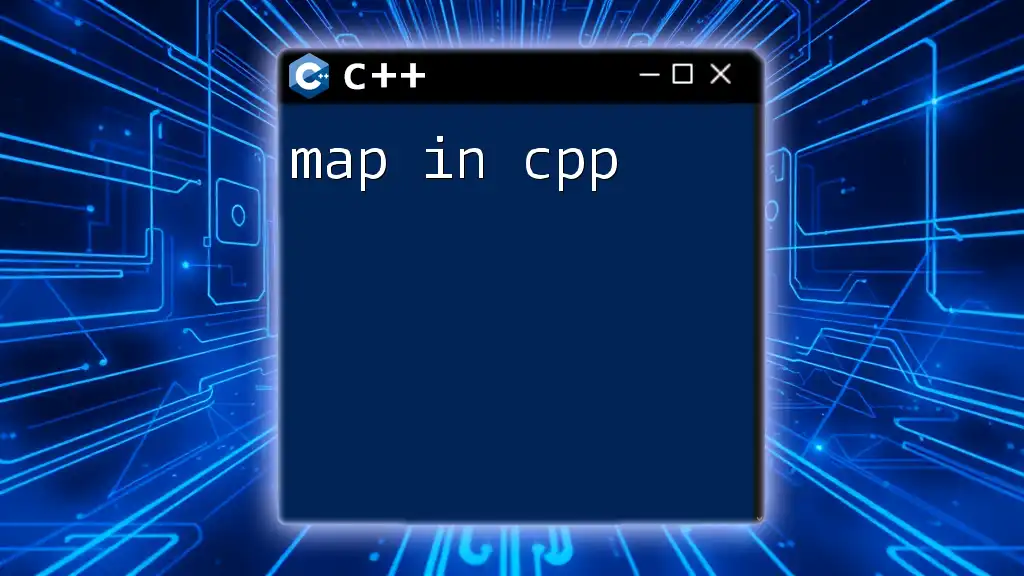
Additional Resources
Books and Online Tutorials
Consider referencing the official [Matplotlib documentation](https://matplotlib.org/) and C++ programming guides. Online platforms such as Coursera, Udacity, or even YouTube can also provide valuable resources and tutorials on both C++ and data visualization concepts.
Community and Forums
Engaging with communities on platforms such as Stack Overflow or Reddit's r/cpp can be invaluable for troubleshooting and growing your understanding of matplotlib cpp.