A virtual lab for C++ (cpp) allows users to experiment and practice C++ commands in a simulated environment, enhancing their coding skills through hands-on experience.
Here's a simple code snippet demonstrating the use of a virtual function in C++:
#include <iostream>
using namespace std;
class Base {
public:
virtual void display() {
cout << "Display Base Class" << endl;
}
};
class Derived : public Base {
public:
void display() override {
cout << "Display Derived Class" << endl;
}
};
int main() {
Base* bPtr; // Base class pointer
Derived dObj; // Derived class object
bPtr = &dObj; // Pointing to derived class object
bPtr->display(); // Calls Derived class display
return 0;
}
Introduction to Virtual Lab CPP
Virtual labs represent a revolutionary approach to learning programming, especially languages like C++. They provide an interactive and engaging environment where users can experiment with code in real-time. This is particularly beneficial in a fast-paced world where setting up a local development environment can be time-consuming.
Benefits of Using a Virtual Lab
Using a virtual lab for C++ offers numerous advantages:
- Accessibility: No need to install complex software on your computer. You can access your virtual lab from any device with an internet connection.
- Hands-on Experience without Setup: Instantly start writing and testing code, allowing you to focus on learning rather than tinkering with installations.
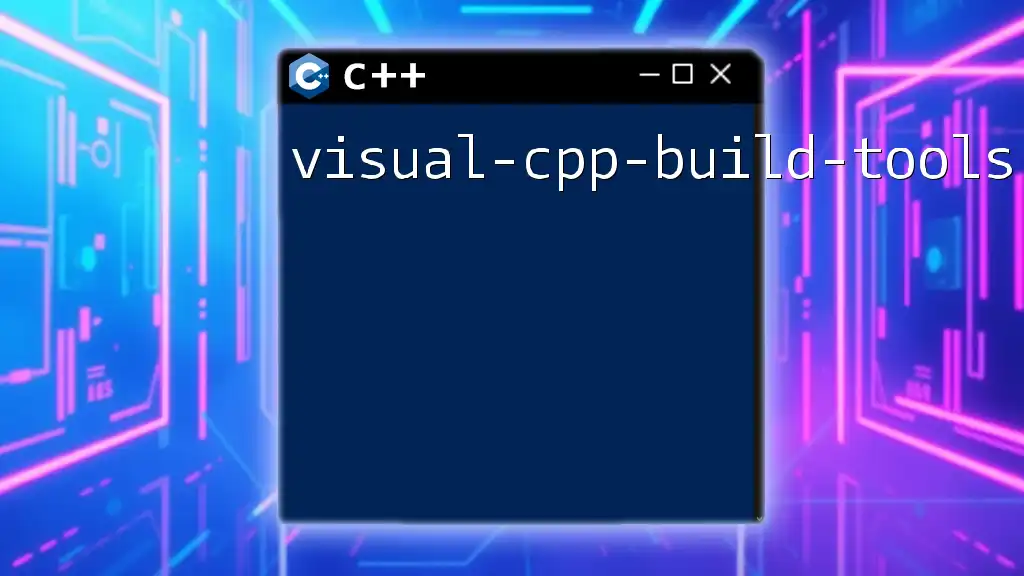
Understanding C++ Commands
What are C++ Commands?
C++ commands are the building blocks of C++ programming, allowing you to perform various operations within your code. These commands consist of specific syntax that instructs the computer on what actions to execute.
Commonly Used C++ Commands
Familiarizing yourself with essential C++ commands is pivotal for effective programming:
- Input/Output: The commands `cin` and `cout` are fundamental for interacting with users.
- Control Structures: Commands like `if`, `for`, and `while` enable you to create conditional logic and loops.
- Data Types: Knowing data types like `int`, `float`, and `char` is crucial for data manipulation.
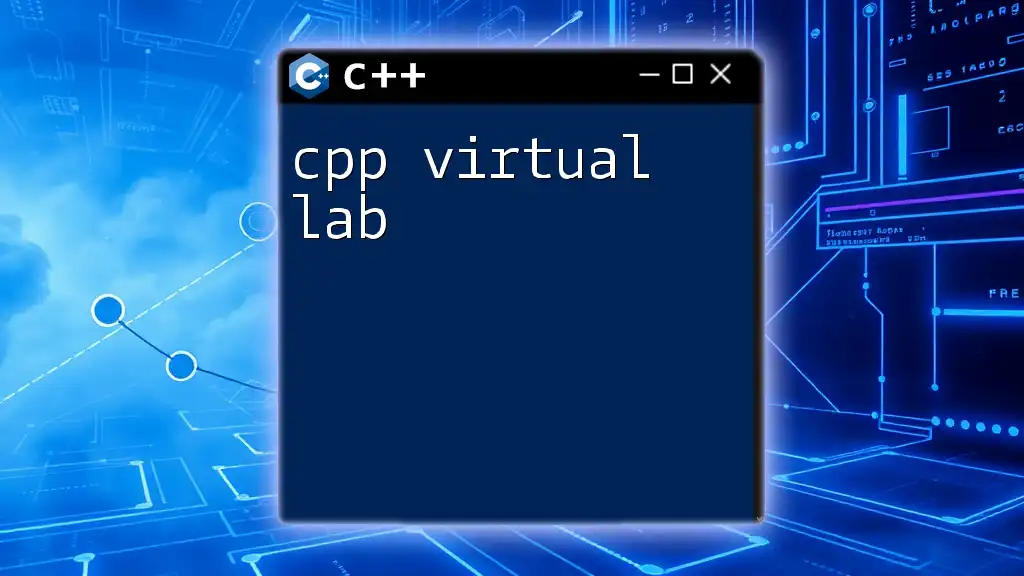
Setting Up Your Virtual Lab Environment
Choosing a Virtual Lab
When selecting a virtual lab, it's helpful to know a few popular options:
- Replit: Offers collaboration features and supports multiple languages, making it versatile.
- CodeChef: Primarily focused on competitive programming, it provides an excellent environment for tests and practice.
Creating an Account and Starting Your First Project
Setting up your virtual lab is straightforward. Here’s an overview of the process:
- Visit your chosen virtual lab's website and create a free account.
- Navigate to the project section to start your first C++ project.
- Explore the interface, including how to write, run, and debug your code.
Using Pre-built Templates and Examples
Most virtual labs feature templates to help you get started quickly:
- Find Templates: Explore the template library or community contributions.
- Example Projects: Use existing projects to understand how to structure your own code and apply best practices.
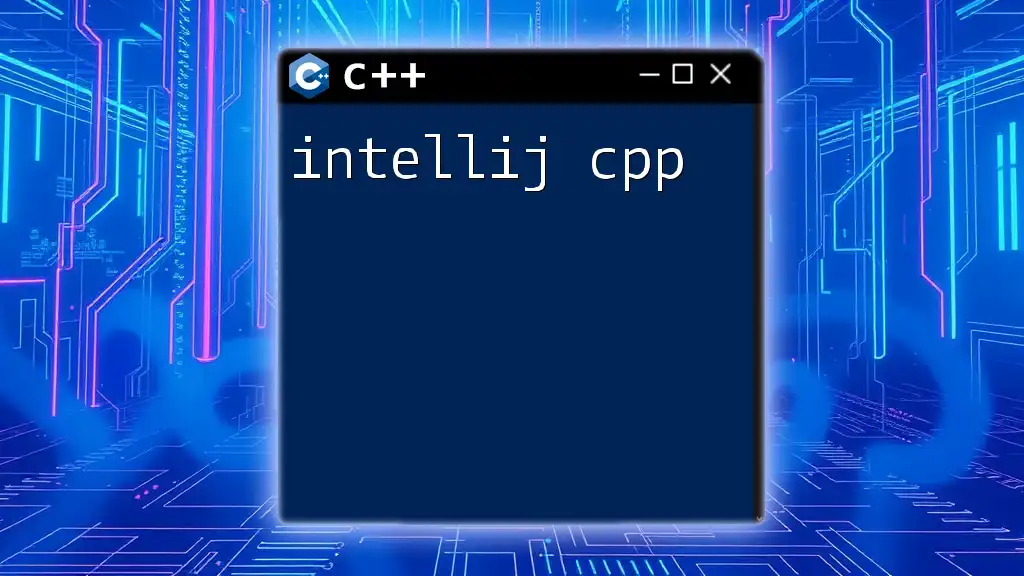
Writing and Testing C++ Code in the Virtual Lab
Creating Your First C++ Program
Let’s write a simple “Hello, World!” program to get you started. This classic example illustrates the basic structure of a C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!";
return 0;
}
In this snippet:
- `#include <iostream>` includes the standard input-output library.
- `using namespace std;` allows you to use standard features without prefixing them.
- `int main()` signifies the start of your program.
Compiling and Running Your Code
Executing your code in a virtual lab is typically just a click away:
- Run Your Code: Look for a “Run” or “Execute” button in the virtual lab interface. The output should display below your code.
- Understanding Errors: If your code doesn’t compile, the lab will display error messages. Pay attention to line numbers and syntax – they guide you toward fixing issues.
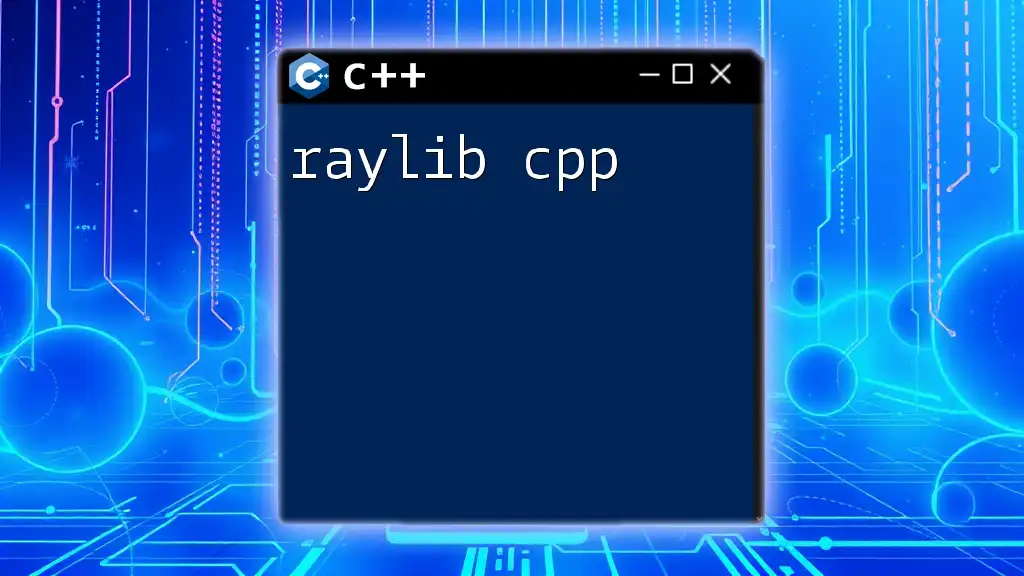
Advanced Features of Virtual Lab CPP
Using Libraries and Frameworks
C++ libraries can significantly enhance your programming capabilities. Let’s look at how to utilize the Standard Template Library (STL):
#include <vector>
#include <iostream>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
cout << num << " ";
}
return 0;
}
This example illustrates how to create a dynamic array using `vector`, showcasing the convenience offered by libraries in C++ programming.
Collaborative Features
Modern virtual labs often come equipped with tools for collaboration. This strengthens learning through teamwork:
- Working Together: You can invite peers to edit your code in real-time, making it easier to tackle projects as a group.
- Understanding Version Control: Familiarize yourself with version control basics to keep track of changes and revert to previous versions if needed.
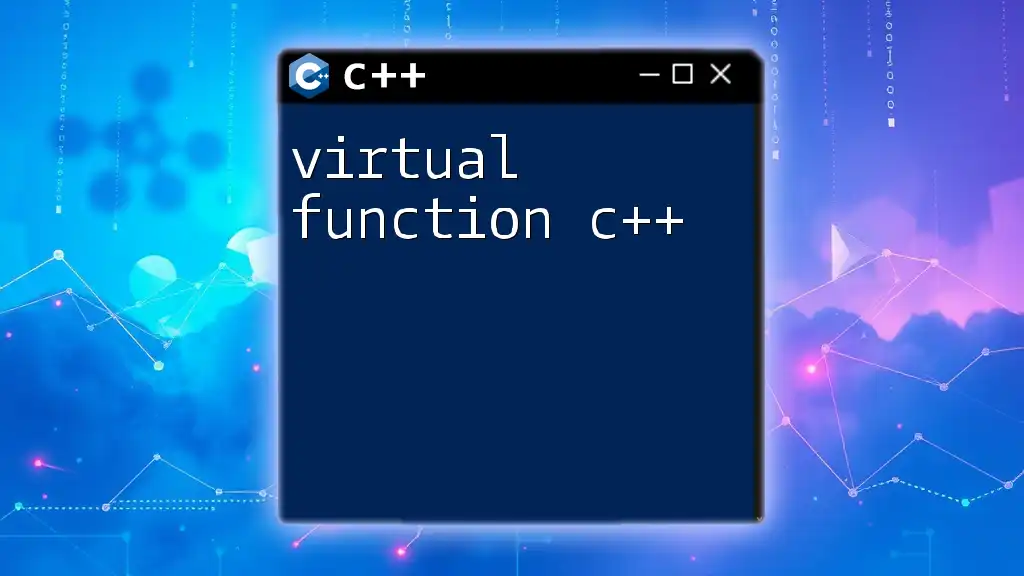
Troubleshooting Common Issues
Compilation Errors
Compilation errors can be frustrating but are an essential part of learning. Common pitfalls include:
- Syntax Errors: Mistyped commands or incorrect punctuation can halt compilation. Review your code carefully.
- Typographical Errors: Ensure all identifiers are spelled correctly and consistently.
Runtime Errors
Once your code compiles, you may encounter runtime errors. These errors occur while the program is running and can sometimes be challenging to diagnose:
- Common Issues: Unhandled exceptions, such as accessing out-of-bound elements in arrays, can cause your program to crash.
- Debugging Strategies: Use print statements to monitor variable values and program flow, helping you identify where the issue lies.
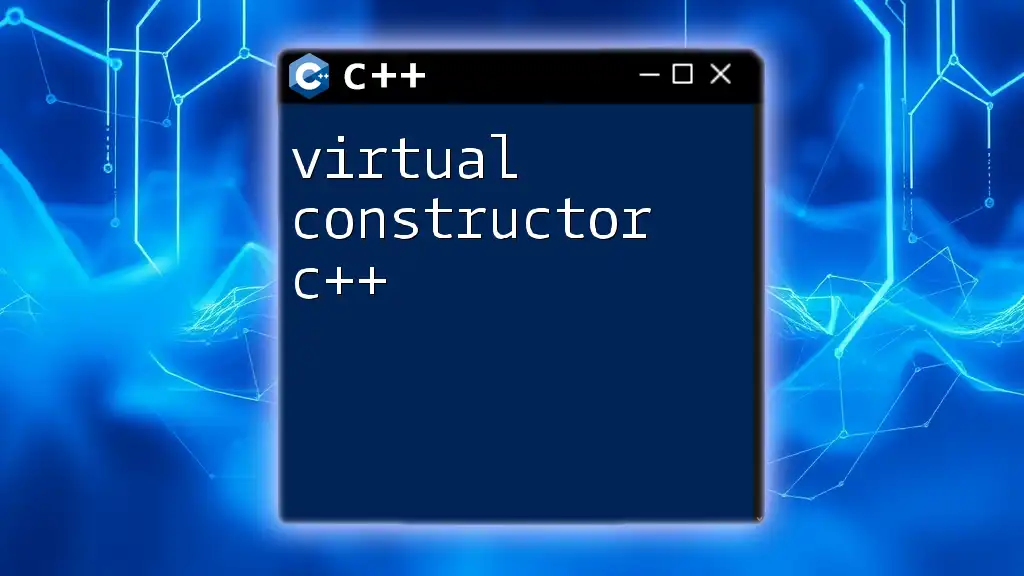
Best Practices for Using Virtual Labs
Documenting Your Code
Proper documentation elevates your code’s readability. Always strive to include comments that explain what your code does. A well-documented snippet might look like this:
#include <iostream>
using namespace std;
// This function adds two numbers and returns the result
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3);
return 0;
}
Comments provide context, making it easier for others (and future you) to understand your thought process.
Version Control and Backup
Maximize your coding efficiency by maintaining good operational habits:
- Saving Your Work: Regularly save your projects to avoid losing significant progress.
- Managing Multiple Projects: Organize your projects logically in folders, and name files descriptively to navigate easily.
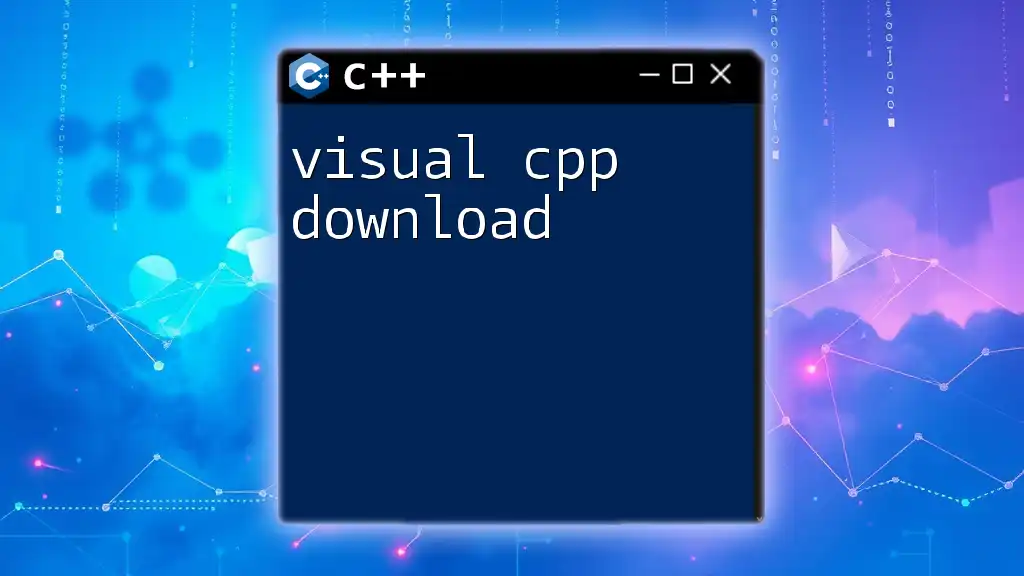
Conclusion
Virtual labs are a powerful tool in mastering C++. They not only provide an environment for experimentation but also allow you to learn efficiently and effectively. By taking advantage of the features offered in virtual labs, collaborating with peers, and adhering to best practices, you can advance your skills significantly.
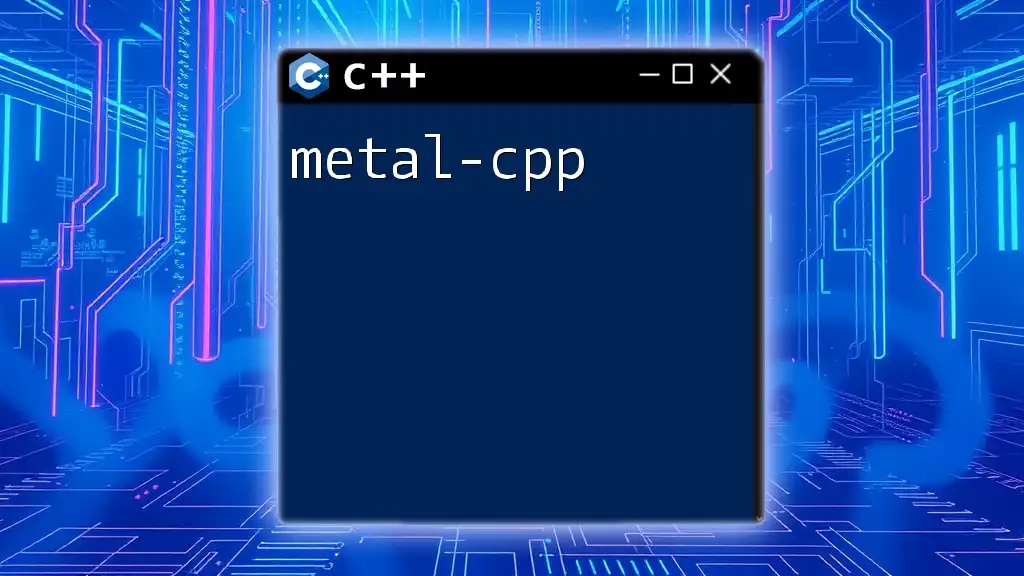
Additional Resources
To further enhance your learning, consider exploring recommended courses, books, and online communities dedicated to C++. Engaging with these resources will solidify your understanding and become part of a supportive programming network.