In C++, a virtual function allows derived classes to override a base class function, while a pure virtual function (declared by assigning 0) makes the base class abstract and requires derived classes to provide an implementation.
Here's a simple example demonstrating both:
#include <iostream>
class Base {
public:
virtual void virtualFunction() {
std::cout << "Base virtual function\n";
}
virtual void pureVirtualFunction() = 0; // Pure virtual function
};
class Derived : public Base {
public:
void virtualFunction() override {
std::cout << "Derived overridden virtual function\n";
}
void pureVirtualFunction() override {
std::cout << "Derived implementation of pure virtual function\n";
}
};
int main() {
Derived d;
d.virtualFunction();
d.pureVirtualFunction();
return 0;
}
Understanding Virtual Functions
What are Virtual Functions?
Virtual functions in C++ are member functions that you expect to override in derived classes. When a base class declares a function as virtual, C++ uses a mechanism known as dynamic dispatch to ensure that the correct function is called for an object, regardless of the type of reference (or pointer) used for the function call.
The purpose of virtual functions is to facilitate run-time polymorphism, allowing for more flexible and reusable code. They enable you to work with object references and pointers to base classes without needing to know the exact derived class type.
Example: Here's a basic illustration of virtual functions:
class Base {
public:
virtual void show() {
std::cout << "Base class show function called." << std::endl;
}
};
class Derived : public Base {
public:
void show() override { // Override the Base class version
std::cout << "Derived class show function called." << std::endl;
}
};
How Virtual Functions Work
When a class declares a virtual function, the compiler creates a virtual table (vtable) for that class. This table holds pointers to the virtual functions that the class can call. Each object of the class carries a pointer (known as the vptr) to the corresponding vtable.
Code Snippet: Here's how the vtable functions under the hood:
Base* b = new Derived(); // Base pointer referring to Derived object
b->show(); // Calls Derived::show due to dynamic dispatch
When `b->show()` is executed, C++ looks up the vtable for the `Derived` class, finds the correct function pointer, and invokes `Derived::show()` instead of `Base::show()`.
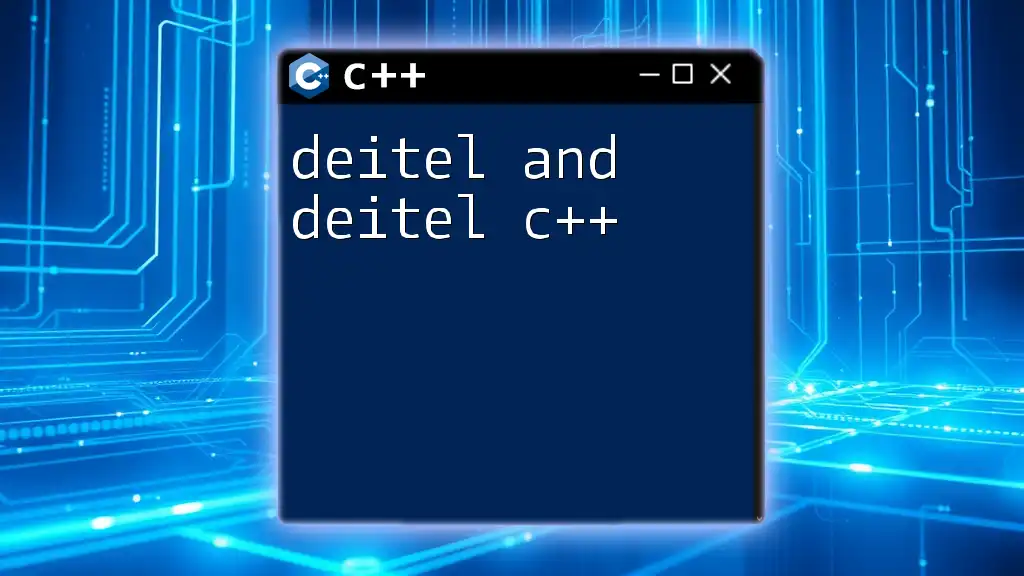
Exploring Pure Virtual Functions
What are Pure Virtual Functions?
A pure virtual function is a function declared in a base class that has no implementation in that class. It is specified by assigning `0` in its declaration. This makes the class an abstract class, meaning that it cannot be instantiated directly.
Example: Here's how pure virtual functions are defined:
class AbstractBase {
public:
virtual void show() = 0; // Pure virtual function
};
class ConcreteDerived : public AbstractBase {
public:
void show() override { // Must override the pure virtual function
std::cout << "ConcreteDerived show function called." << std::endl;
}
};
Creating Abstract Classes
An abstract class serves as a blueprint for derived classes. It can contain both implemented and pure virtual functions. By defining at least one pure virtual function, a derived class is required to provide concrete implementations for all inherited pure virtual functions.
Example: This illustrates how to define an abstract class:
class Shape {
public:
virtual double area() = 0; // Pure virtual
virtual void draw() = 0; // Another pure virtual
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override {
return 3.14159 * radius * radius;
}
void draw() override {
std::cout << "Drawing Circle" << std::endl;
}
};
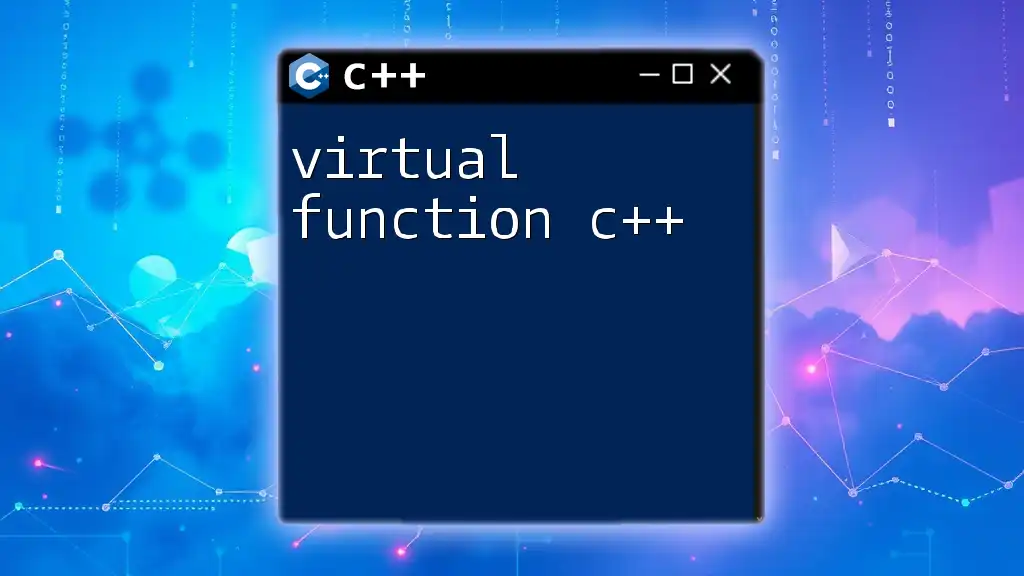
Virtual vs Pure Virtual: A Comparative Analysis
Key Differences
Feature | Virtual Function | Pure Virtual Function |
---|---|---|
Definition | A function with a default implementation in the base class | A function with no implementation in the base class |
Instantiation | Base class can be instantiated | Base class cannot be instantiated |
Purpose | For run-time polymorphism | Defines a contract for derived classes |
When to Use Virtual Functions
You can use virtual functions when you want to provide default behavior in the base class while allowing derived classes to override this behavior. For instance, consider a logging mechanism whereby the base class can provide a general logging method, but derived classes can specify detailed logs tailored to their needs.
Code Example:
class Logger {
public:
virtual void log(const std::string& message) {
std::cout << "Log: " << message << std::endl;
}
};
class ErrorLogger : public Logger {
public:
void log(const std::string& message) override {
std::cout << "Error: " << message << std::endl;
}
};
When to Use Pure Virtual Functions
Pure virtual functions are employed when you're designing a class to serve as an interface or base class and there is no meaningful default implementation available. They force derived classes to implement specific behavior.
Code Example:
class Animal {
public:
virtual void makeSound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void makeSound() override {
std::cout << "Woof!" << std::endl;
}
};
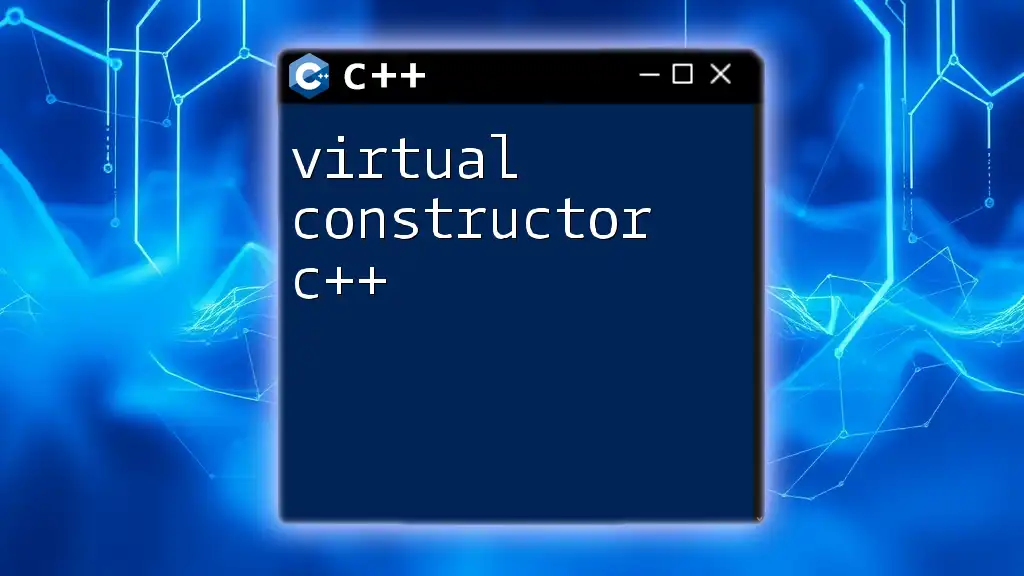
Implementing Virtual Functions in C++
Syntax and Structure
The syntax for declaring a virtual function is straightforward. Simply precede the function definition in the base class with the `virtual` keyword. Derived classes should use the `override` specifier to enhance readability and prevent issues.
Code Snippet:
class Base {
public:
virtual void display() {
std::cout << "Base display" << std::endl;
}
};
class Derived : public Base {
public:
void display() override {
std::cout << "Derived display" << std::endl;
}
};
Override and Final Specifiers
C++11 introduced the `override` and `final` keywords, enhancing the clarity and safety of code. The `override` keyword signals that the function is intended to override a base class virtual function, while `final` prevents any further overriding in derived classes.
Example:
class Base {
public:
virtual void show() final {
std::cout << "Final Base show" << std::endl;
}
};
class Derived : public Base {
public:
void show() override { // Error: cannot override final function
// ...
}
};
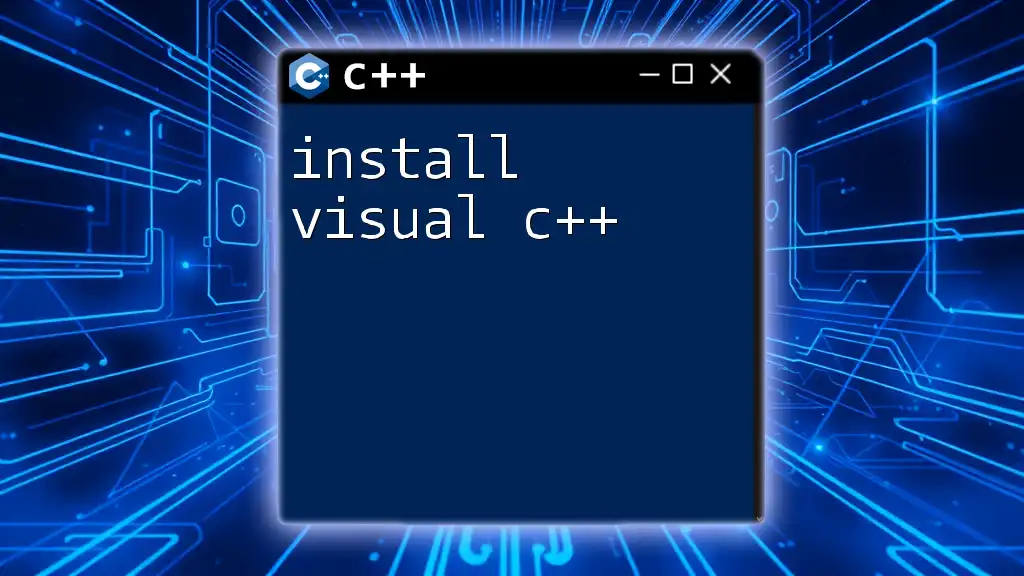
Practical Scenarios and Use Cases
Use Case 1: Implementing a Base Class for Different Shapes
Consider creating a `Shape` class to calculate areas, where specific shapes like circles or rectangles derive from it. Each shape can provide its respective area calculation.
Code Snippet:
class Shape {
public:
virtual double area() = 0; // Pure virtual function
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override {
return 3.14159 * radius * radius;
}
};
Use Case 2: Designing a Plugin System
In systems requiring extensibility, abstract classes with pure virtual functions can define interfaces for plugins. This allows clients to create plugins while hiding specific implementations.
Example:
class Plugin {
public:
virtual void execute() = 0; // Pure virtual function
};
class MyPlugin : public Plugin {
public:
void execute() override {
std::cout << "Executing MyPlugin." << std::endl;
}
};
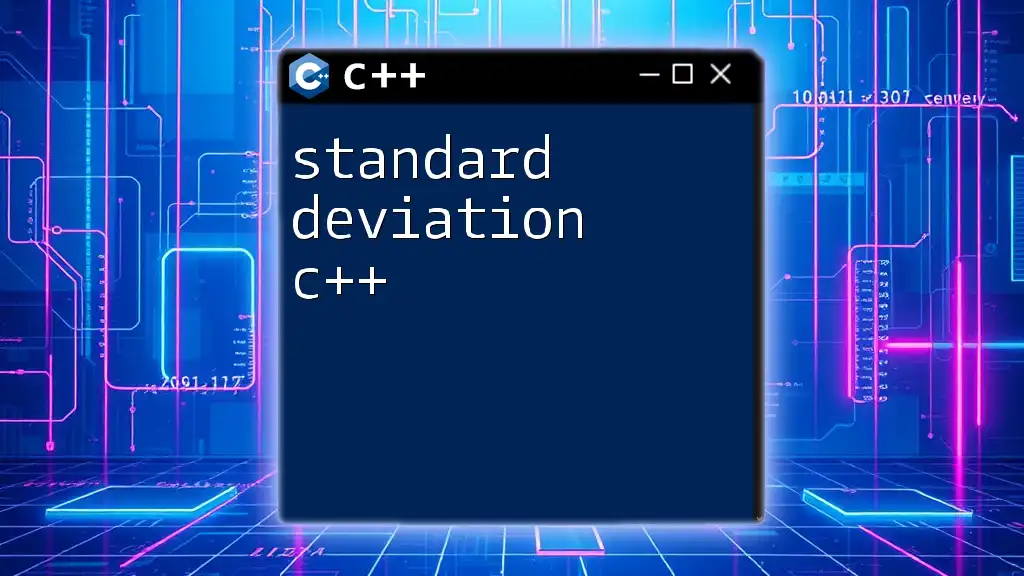
Best Practices
Key Considerations in Using Virtual and Pure Virtual Functions
-
Always declare destructors as virtual in base classes to ensure proper cleanup of derived classes:
class Base { public: virtual ~Base() {} };
-
Be mindful of performance impacts when using virtual functions, as they introduce a slight overhead due to the indirection of vtable lookups.
-
Ensure derived classes implement all pure virtual functions of the base class, or they will remain abstract.
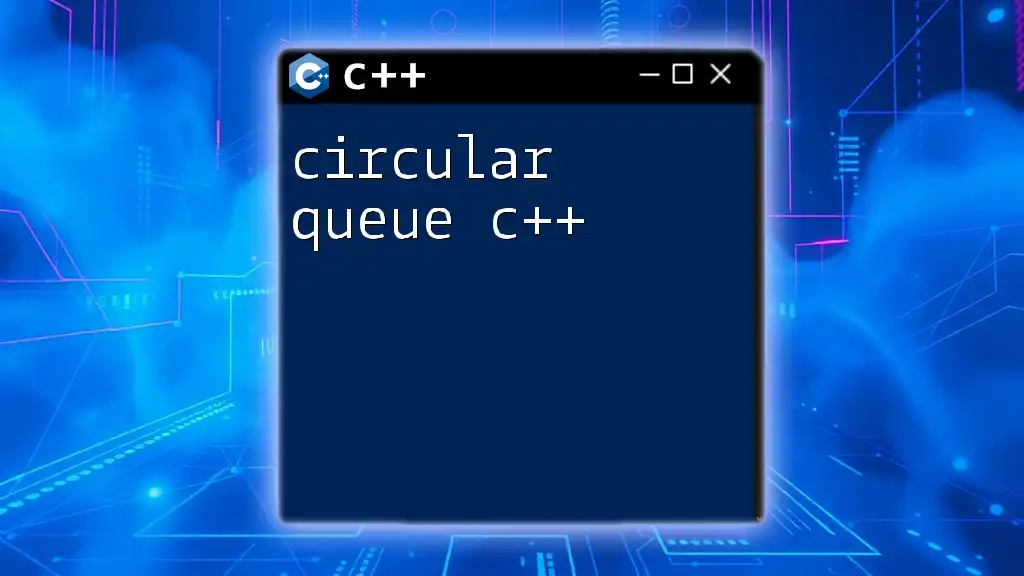
Common Pitfalls
Mistakes to Avoid
-
Forgetting to declare destructors as virtual can lead to resource leaks when derived class objects are deleted through base class pointers.
-
Incorrectly overriding functions without the `override` specifier can mask errors, leading to unexpected behavior.
-
Undefined behavior when attempting to call a pure virtual function outside a derived class context can lead to runtime exceptions, so ensure proper type usage.
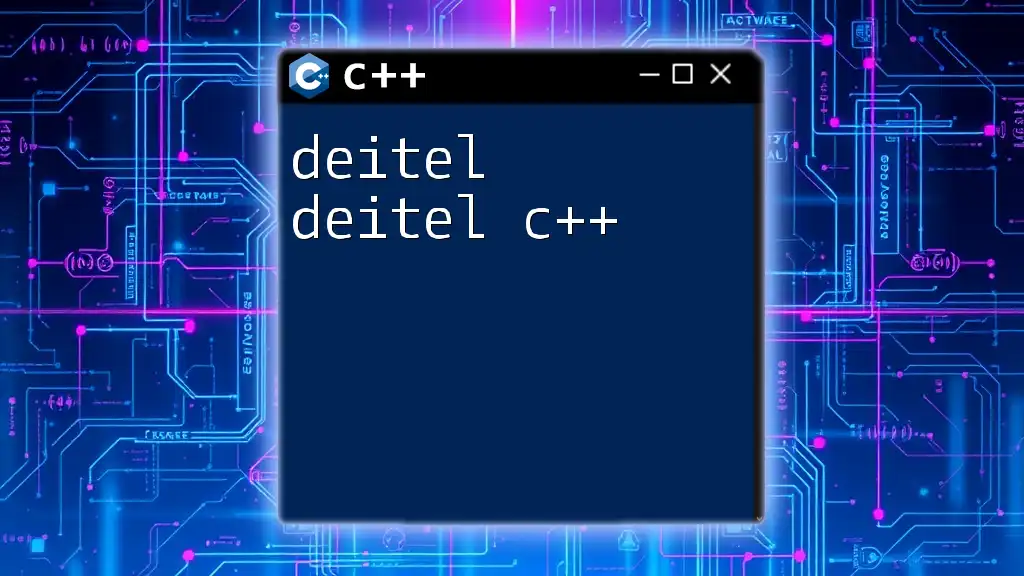
Conclusion
Mastering the concepts of virtual and pure virtual C++ functions is crucial for effective polymorphic design. These features promote code reusability and flexibility while enabling developers to manage complex systems through abstraction.
To fully harness the capabilities of these functions, practice creating diverse class hierarchies and implementing various scenarios that necessitate polymorphism. With time and experience, you'll find that virtual functions and pure virtual functions will become indispensable tools in your C++ programming toolkit.
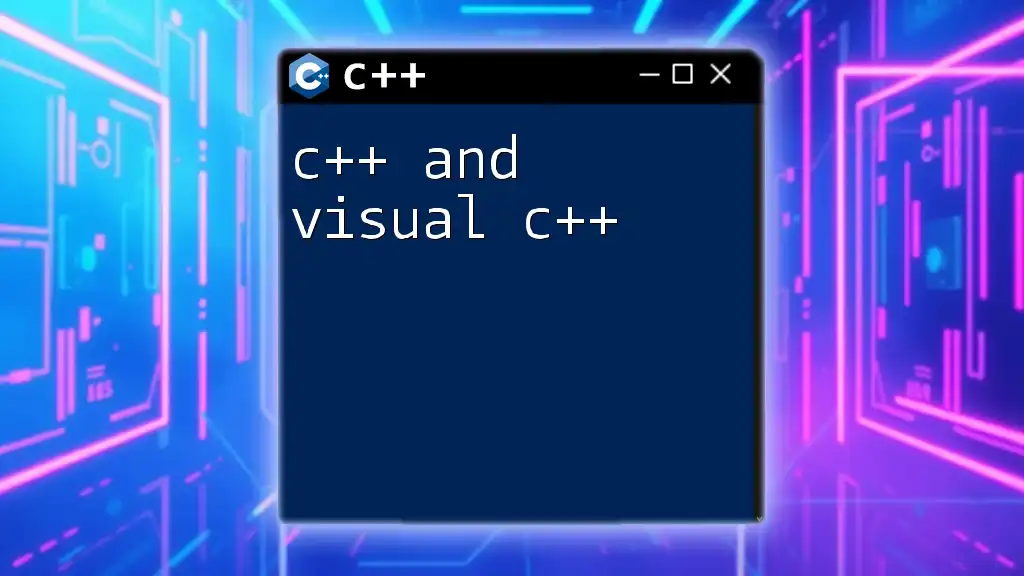
Additional Resources
For further learning, consider exploring recommended books and online courses, along with valuable C++ documentation available through resources like Stack Overflow and GitHub.
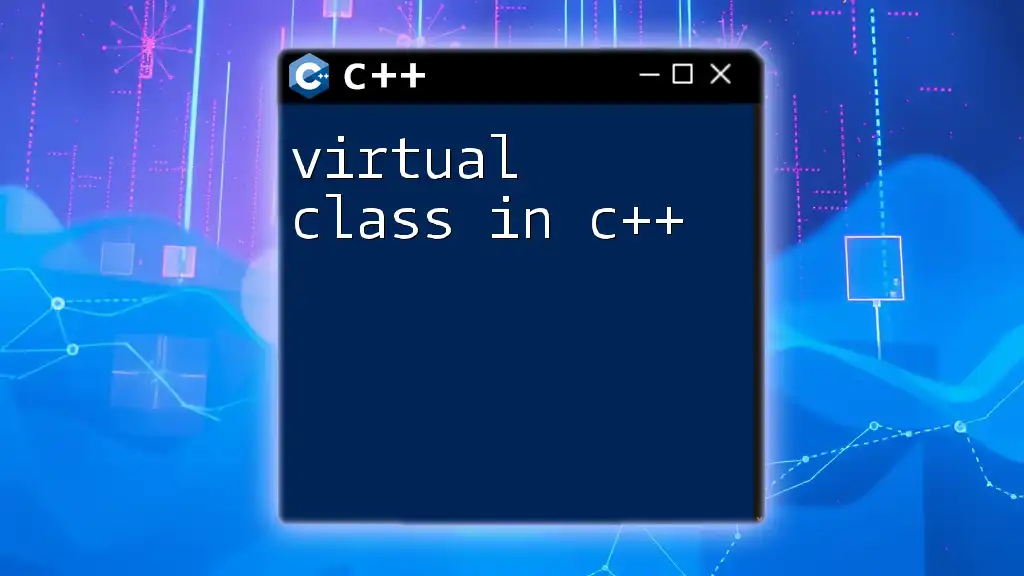
Call to Action
Challenge yourself by implementing a few of the demonstrated concepts in your projects. Share your experiences, examples, or any questions you have, and join our community dedicated to mastering efficient C++ programming!