Deitel & Deitel's C++ programming books are renowned for their clear explanations and practical examples, making them an excellent resource for beginners and experienced programmers alike.
Here's a simple code snippet demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Deitel Method of Teaching Programming
Interactive Learning
The Deitel approach emphasizes interactive learning, which significantly enhances the programming education experience. This method engages learners through hands-on practice, allowing them to immediately apply concepts they just learned. By writing code and solving problems in real-time, students receive instant feedback that is crucial for improving their coding skills.
This engagement fosters a deeper understanding of the material because learners are not passive recipients of knowledge. Instead, they actively participate in the learning process, which leads to better retention and application of programming concepts.
Structured Approach
Deitel's structured approach to teaching programming concepts is one of its core strengths. This method focuses on how to logically progress through programming topics, ensuring that students build on their prior knowledge. By organizing the learning material cohesively, Deitel helps guide students from basic concepts to more advanced topics with ease.
This logical flow is essential in programming. It prepares beginners by establishing a solid foundation before introducing them to complex programming constructs. As a result, students can feel confident as they move forward in their understanding of programming principles.
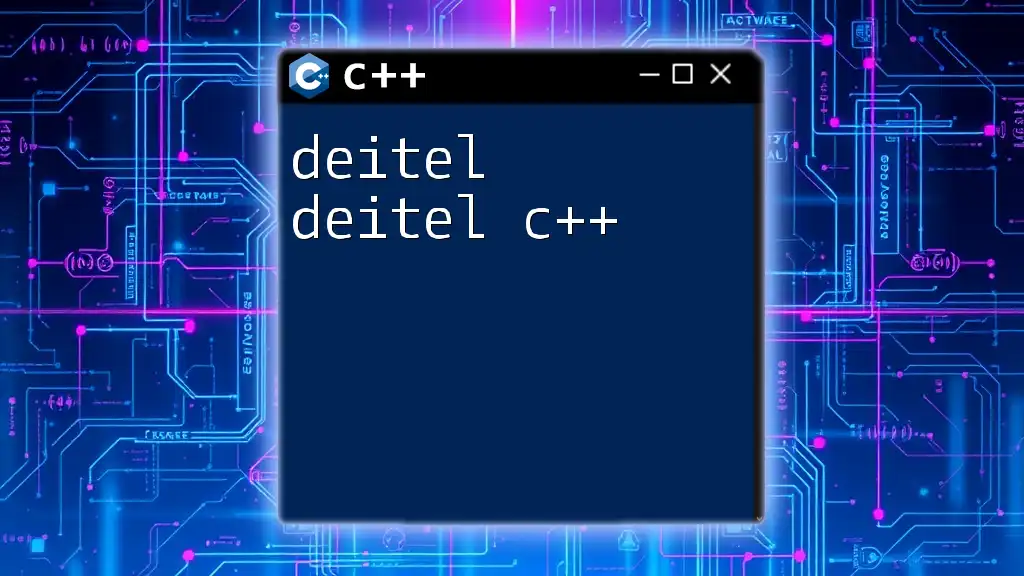
C++ How to Program Deitel: Key Features
Comprehensive Coverage of C++
Deitel's "C++ How to Program" book offers a thorough coverage of essential C++ topics. Readers will find comprehensive discussions on syntax, control structures, functions, classes, templates, and more. This expansive curriculum equips learners with everything they need to succeed as C++ programmers.
Moreover, the material explores both long-established practices and newer features introduced in modern C++. This broad coverage ensures that students are up-to-date and prepared for real-world programming challenges.
Real-World Applications
One of the hallmarks of Deitel's teaching philosophy is an emphasis on real-world applications. Deitel presents programming concepts through practical examples and projects, allowing learners to see how the theory translates to practical use. This connection to real-world scenarios not only provides motivation but also aids in understanding how to develop functional and effective programs.
For instance, students might work on projects that involve creating simple applications or games, enhancing their learning experience while gaining skills that are directly applicable in the technology industry.
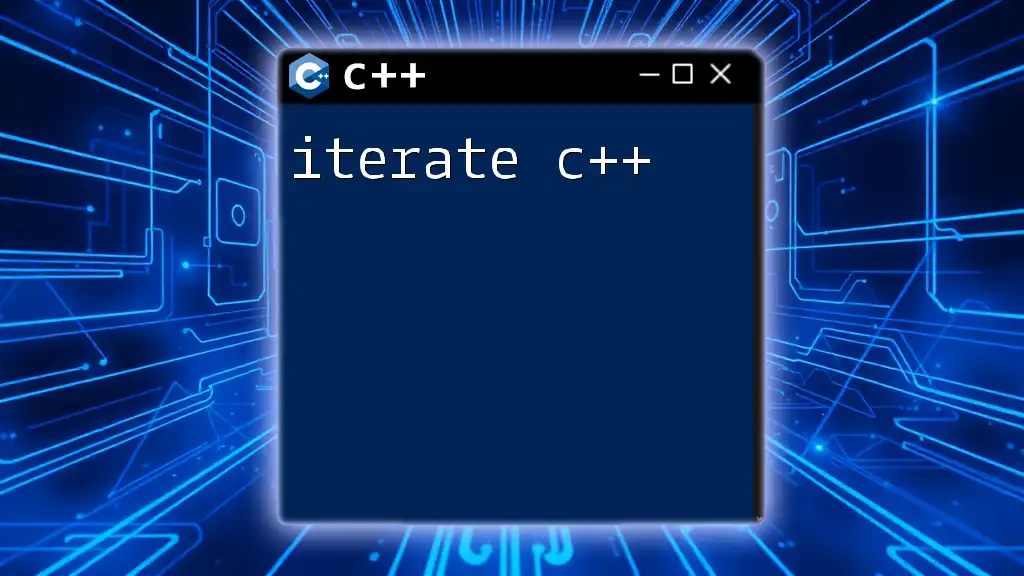
C++ Fundamentals from Deitel
Basic Syntax and Data Types
Understanding basic syntax and data types is crucial for any aspiring C++ programmer. In Deitel's teachings, students are introduced to the syntax rules that govern the language. For example, a simple program that outputs a number may look like this:
#include <iostream>
using namespace std;
int main() {
int number = 10;
cout << "The number is: " << number << endl;
return 0;
}
This example illustrates key concepts such as variable declaration, output using `cout`, and the importance of `return 0` to signify the successful execution of the program.
Data types are also explored in depth. By understanding types such as integers, floating points, characters, and strings, students grasp how to manage variables appropriately, prevent errors, and maintain clarity within their programs.
Control Structures
Control structures are essential for determining the flow of a program. Deitel explains how to implement conditional statements and loops effectively. For instance, a common control flow using an `if` statement might resemble the following:
int x = 20;
if (x >= 20) {
cout << "x is greater than or equal to 20" << endl;
}
Furthermore, looping constructs allow for repeated execution of code. A simple `for` loop is shown to further cement this idea:
for (int i = 0; i < 5; i++) {
cout << "Count: " << i << endl;
}
These control structures add dynamic functionality to programs, enabling developers to create more complex algorithms and solutions.
Functions and Modular Programming
Functions are fundamental to modular programming, allowing C++ programmers to encapsulate code into reusable blocks. This organization improves both readability and maintainability. Deitel promotes the use of functions with clear examples.
Consider the following example:
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3) << endl;
return 0;
}
Here, the `add` function takes two integers as arguments and returns their sum. This modular design allows for easy updates and debugging, as you can focus solely on specific sections of code without affecting the entire program.
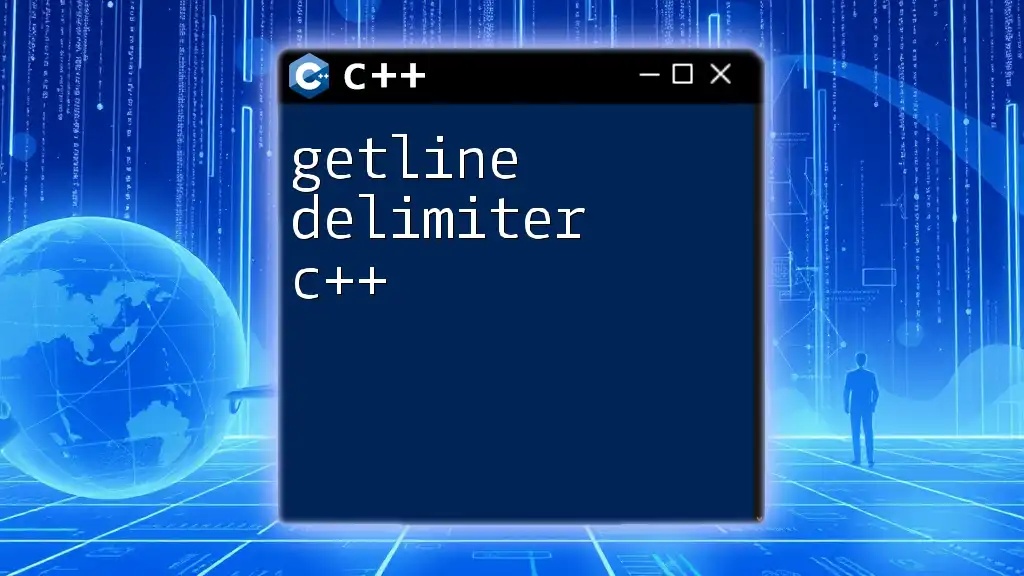
Object-Oriented Programming in C++
Classes and Objects
At the heart of C++ is its object-oriented programming (OOP) paradigm, emphasizing the use of classes and objects. Deitel effectively introduces students to these concepts, illustrating how they facilitate code organization and reusability.
For example, consider the following class definition:
class Car {
public:
void start() {
cout << "Car started." << endl;
}
};
int main() {
Car myCar;
myCar.start();
return 0;
}
In this example, `Car` is a class that defines a method called `start`. An object of the class is created in `main`, showcasing how to instantiate and interact with objects.
Inheritance and Polymorphism
Inheritance allows programmers to create new classes based on existing ones, promoting code reusability. Deitel explains how inheritance can be used to refine and extend existing code structures. For instance:
class Animal {
public:
virtual void sound() {
cout << "Animal makes a sound." << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Woof!" << endl;
}
};
In this snippet, `Dog` inherits from `Animal`, taking on its properties while redefining the `sound` method for its specific behavior. This leads to flexibility in code, enabling polymorphism—where a call to an overridden method can invoke a different implementation based on the object type.
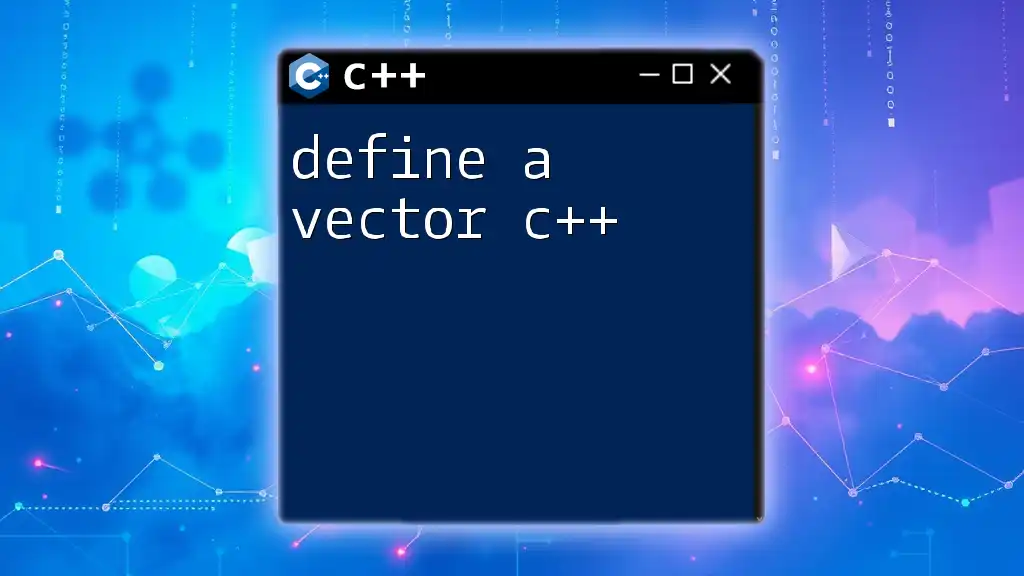
Debugging and Best Practices
Common C++ Errors and Solutions
Deitel also emphasizes the importance of debugging and understanding common errors. Students are taught to recognize and troubleshoot issues such as syntax errors, logical errors, and runtime errors. This knowledge is vital for developing robust applications.
Importance of Code Comments
Another key takeaway from Deitel’s teaching is the significance of code comments. Comments serve to clarify purpose and intent within code, making it easier for others to understand (or for the original author to revisit later) what each section is designed to accomplish. Effective commenting improves code readability, which is crucial in collaborative environments.
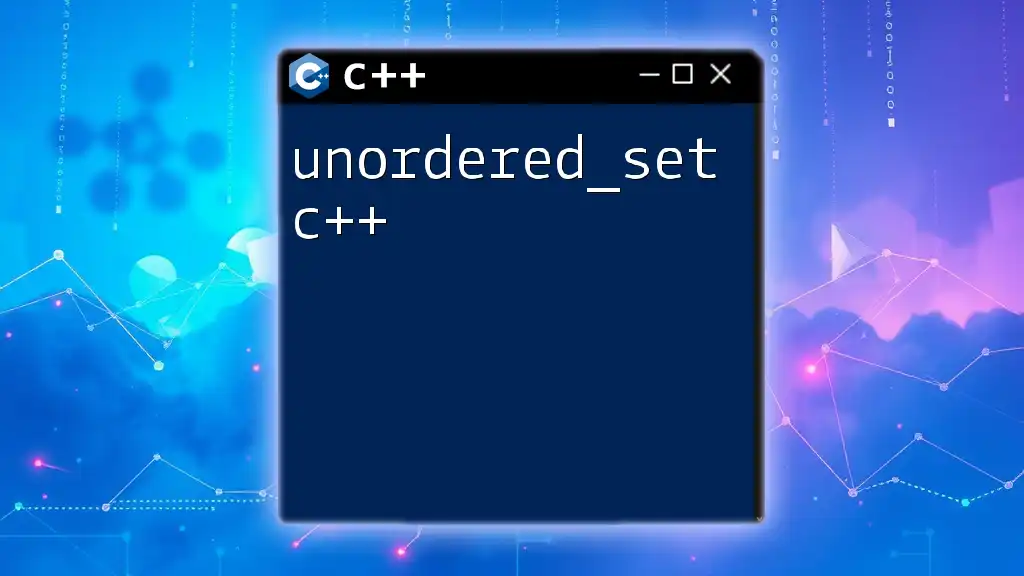
Resources for Learning C++ with Deitel
Books and eBooks
For learners eager to delve deeper into programming, Deitel's numerous published resources, including the “C++ How to Program” book, provide invaluable insights. These books cater to both beginners and experienced programmers, covering both foundational and emerging topics in C++.
Online Courses and Workshops
In addition to books, Deitel offers various online resources such as courses and workshops. Structured courses often provide a more disciplined learning environment, leading to comprehensive mastery of C++. These courses can also foster a sense of community among students, encouraging collaboration and networking.
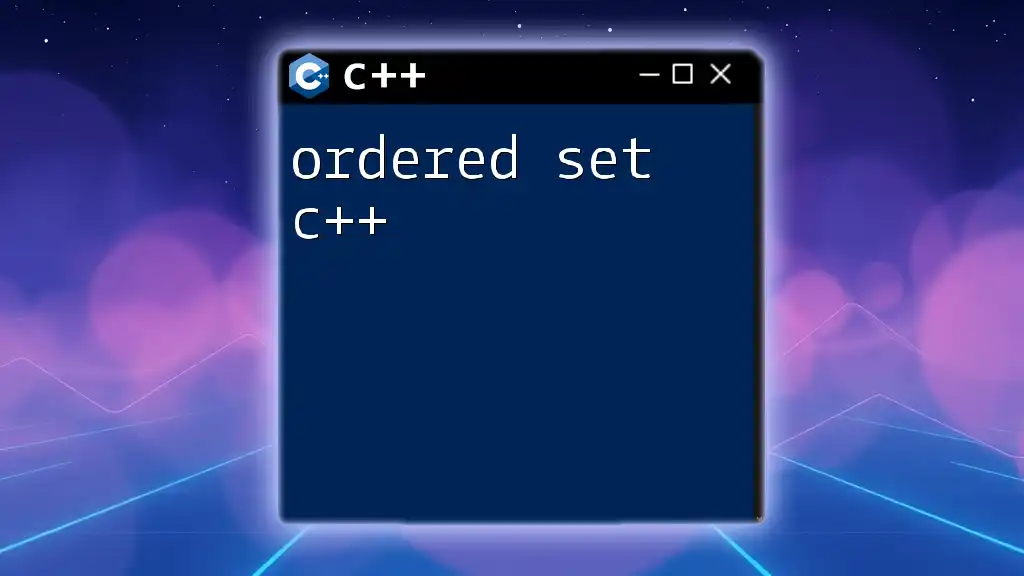
Conclusion
Through the Deitel and Deitel C++ learning model, students gain access to a wealth of information presented in an interactive, structured manner. From mastering the fundamentals of C++ syntax and data types to exploring advanced OOP concepts, learners benefit from a well-rounded education designed to prepare them for real-world programming challenges.
By engaging with Deitel's resources, aspiring programmers can embark on their journey of learning C++, honing their skills and building a solid foundation for future coding projects. Whether through books or online courses, there is ample opportunity to develop expertise in this invaluable programming language. Start your journey in learning C++ today!
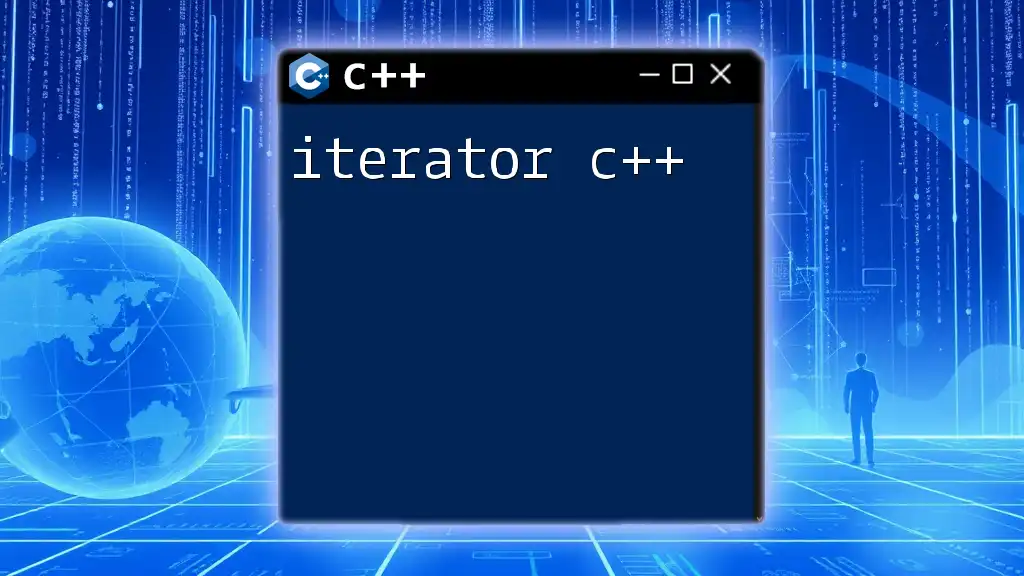
Additional Resources
Be sure to explore the official Deitel website for more materials and community support forums where you can ask questions and share your learning experiences with others!