In C++, the `getline` function can be utilized to read a line of text from an input stream, allowing you to specify a custom delimiter to determine when to stop reading.
Here's a code snippet demonstrating the use of `getline` with a custom delimiter:
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter text (ends with ';'): ";
getline(std::cin, input, ';'); // ';' is the custom delimiter
std::cout << "You entered: " << input << std::endl;
return 0;
}
Understanding getline
What is the getline Function?
The `getline` function in C++ is a standard library function defined in the `<string>` header that allows you to read an entire line of text from an input stream. The primary use of `getline` is to read user input or data where line breaks are crucial, such as when processing text files or standard input. Its syntax is straightforward:
std::getline(std::istream& is, std::string& str);
In this syntax:
- `is` is the input stream (like `std::cin`).
- `str` is the `std::string` where the input will be stored.
Default Behavior
By default, `getline` reads characters from the input stream until it encounters a newline character (`\n`). This means that it effectively captures all characters up until the user presses the “Enter” key, allowing you to input strings that may contain spaces.
Here’s an example of the default behavior:
std::string text;
std::getline(std::cin, text);
std::cout << text;
In this case, if the user enters “Hello World”, the output will immediately display “Hello World” upon pressing Enter.

The Role of Delimiters
What is a Delimiter?
A delimiter is a character or set of characters that serves as a boundary in a sequence of data. In many programming scenarios, delimiters are used to separate values, such as in CSV (Comma-Separated Values) files, where commas act as delimiters between different items.
Using Custom Delimiters with getline
The `getline` function is versatile, allowing you to specify a custom delimiter. You can alter the default newline behavior by telling `getline` to stop reading when it encounters a specific character.
The syntax for using a custom delimiter is as follows:
std::getline(std::istream& is, std::string& str, char delim);
Example Code Snippet Using Delimiter
Here’s an example of how to use a specific delimiter (comma in this case):
std::string data;
std::getline(std::cin, data, ',');
std::cout << "Data: " << data;
In this code, `getline` will read input until it finds a comma, allowing you to effectively work with multi-part data entries.
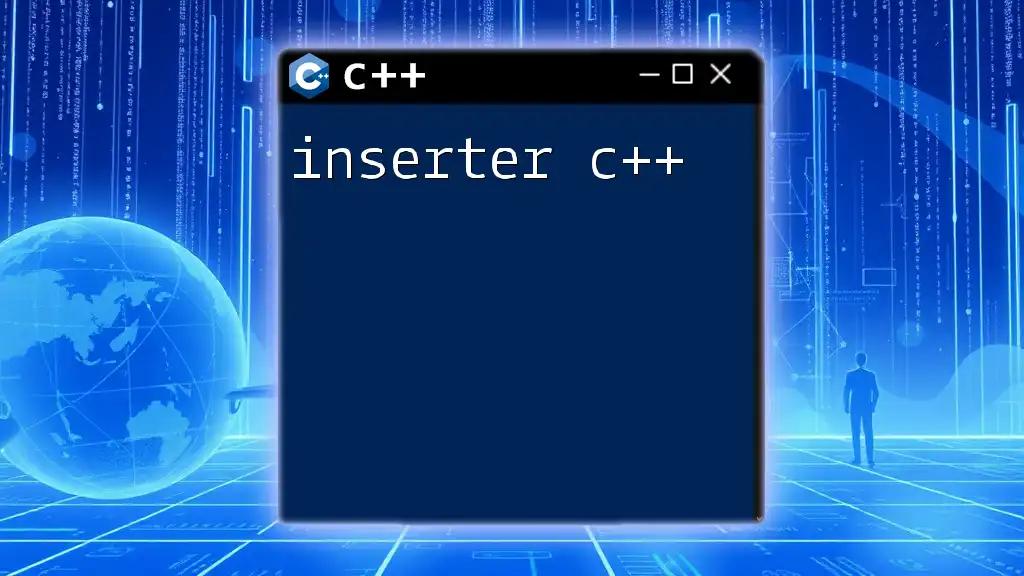
Practical Examples
Reading CSV Input
One common application of delimiters is reading CSV formatted input. CSV is widely used for storing tabular data, where each field is separated by a comma.
Consider the following snippet where we read multiple entries from standard input:
std::string line;
while (std::getline(std::cin, line, ',')) {
std::cout << "CSV Element: " << line << std::endl;
}
In this scenario, if the user inputs “John,Doe,25”, the program will print:
CSV Element: John
CSV Element: Doe
CSV Element: 25
Splitting Sentences into Words
You can also use space as a delimiter to read and split sentences into individual words. Here’s how you might do that:
std::string sentence = "Hello world from C++";
std::istringstream ss(sentence);
std::string word;
while (std::getline(ss, word, ' ')) {
std::cout << "Word: " << word << std::endl;
}
Each word in the sentence will be printed on a new line, as the `getline` function processes the string until it encounters the space character.
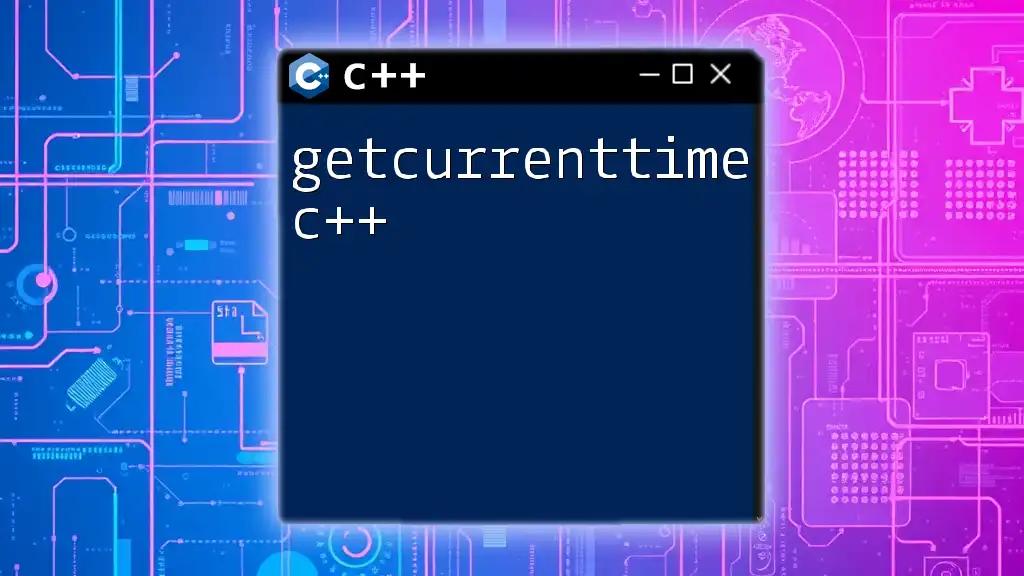
Advanced Techniques
Multiple Delimiters
In more complex scenarios, you might encounter the need to handle multiple delimiters. For instance, if you have a string where items are separated by both commas and semicolons, you can utilize regular expressions to tackle this:
// Include <regex>
std::string text = "word1,word2;word3";
std::regex delim("[,;]");
std::sregex_token_iterator it(text.begin(), text.end(), delim, -1);
std::sregex_token_iterator regEnd;
for (; it != regEnd; ++it) {
std::cout << "Word: " << *it << std::endl;
}
In this code snippet, both commas and semicolons function as delimiters, allowing you to effectively parse complex strings.
Error Handling and Input Validation
When using `getline`, implementing error handling and input validation is crucial. You want to ensure that the input received is valid and usable. Below is an example demonstrating how to handle empty input:
std::string input;
while (std::getline(std::cin, input)) {
if (input.empty()) {
std::cout << "Input cannot be empty. Try again." << std::endl;
} else {
std::cout << "You entered: " << input << std::endl;
break; // Exit loop on valid input
}
}
This loop will continue prompting the user for input until they enter a non-empty string.
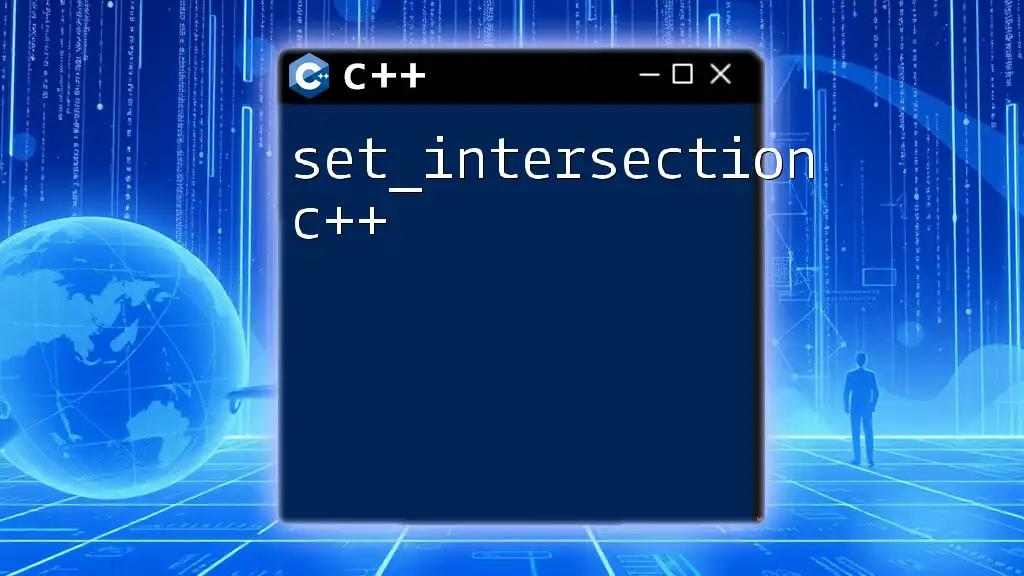
Best Practices
Choosing the Right Delimiter
Choosing an effective delimiter is essential for the proper parsing of your data. When dealing with user input, select a character that does not appear in the data itself unless necessary. For instance, if your input data could potentially contain commas, consider using less common characters as delimiters.
Avoiding Common Pitfalls
When working with delimiters, one common pitfall is not handling edge cases, such as empty input or input containing only delimiters. It’s crucial to account for these scenarios to avoid unexpected behavior in your program.
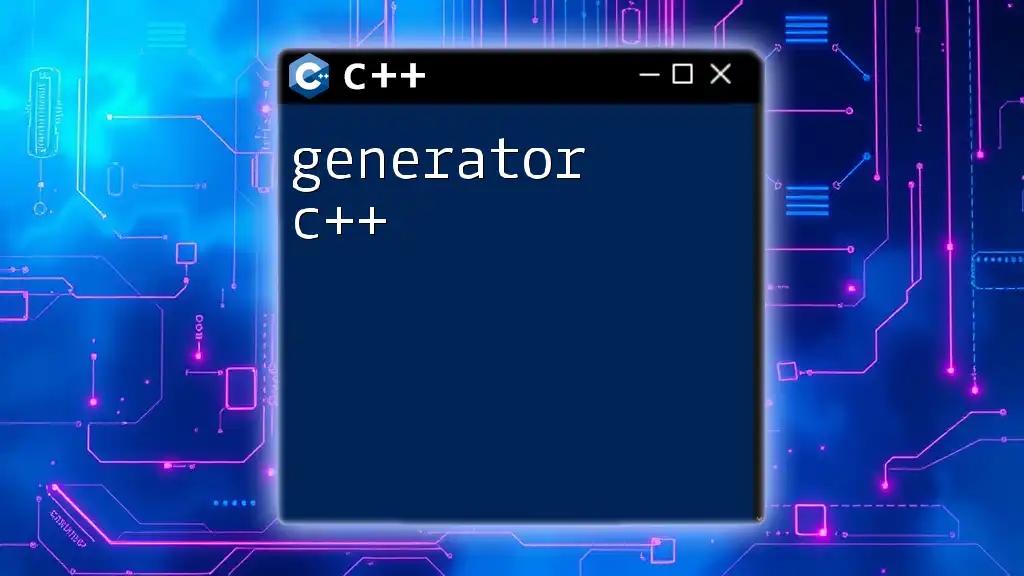
Conclusion
By mastering the use of the `getline` function along with custom delimiters in C++, you can significantly enhance your data input and parsing capabilities. Understanding how to read, split, and validate user input is invaluable for building efficient and user-friendly applications. The flexibility of `getline` combined with proper delimiter management allows you to handle various real-world scenarios effectively. As you continue to learn and apply C++, stay curious about the different ways you can manipulate strings and data flow in your programs. For further enhancements in your skills, delve into official documentation or explore specialized C++ learning resources.