"Deitel C++" refers to a popular programming resource that emphasizes practical learning through concise examples and clear explanations, helping beginners quickly grasp essential C++ concepts.
Here’s an example of a simple C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Deitel’s C++?
Deitel’s C++ refers to a series of programming educational resources authored by the Deitel family, specifically Paul and Harvey Deitel. These resources have become fundamental in teaching computer programming concepts. The Deitel books focus on C++, which is one of the most widely utilized programming languages today, known for its versatility and power in developing applications ranging from games to complex system software.
The significance of C++ in programming cannot be overstated. It serves as the backbone for many software applications, and its features allow developers to write high-performance code. The Deitel approach leverages this language's capabilities to equip readers with practical skills necessary for real-world programming.
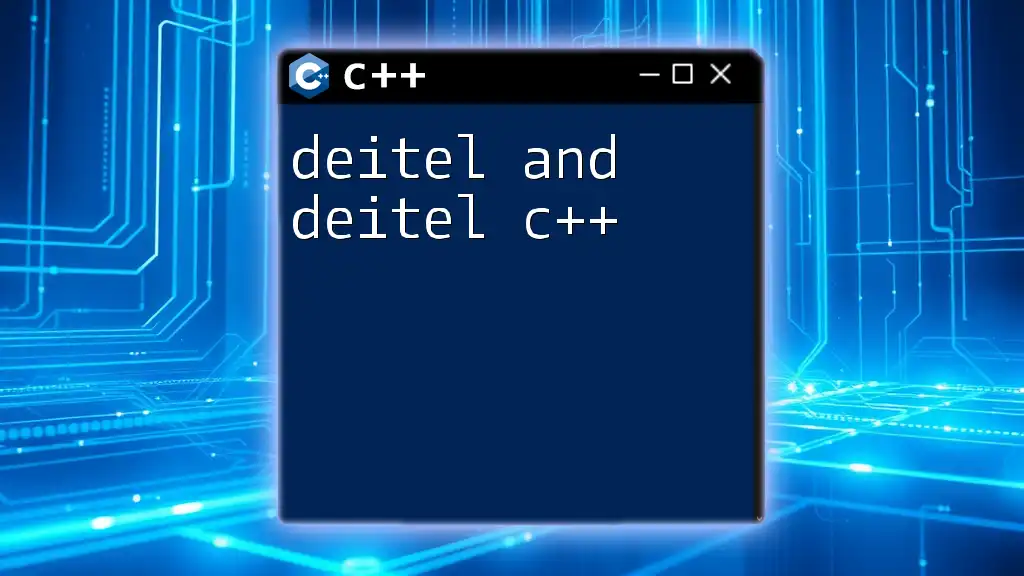
Why Choose Deitel for Learning C++?
One of the foremost reasons to choose Deitel for learning C++ is their structured learning approach. The Deitel books progressively introduce each concept, making it easier for beginners to grasp complex programming ideas. Rather than overwhelming learners with dense material, they hone in on step-by-step instruction that builds confidence.
Another vital aspect of Deitel’s method is the use of real-world examples. This practical approach helps students relate theoretical concepts to actual programming scenarios, reinforcing learning through application.
![Effortless Memory Management: Delete[] in C++ Explained](/images/posts/d/delete-cpp.webp)
Understanding C++ Basics
Overview of C++ Language
The C++ language is characterized by several key features that set it apart from other languages. Object-oriented programming (OOP) is one of the most significant features, enabling developers to create modular and maintainable code. Beyond OOP, C++ supports low-level memory manipulation, providing performance advantages not typically found in higher-level languages.
Getting Started with C++
To start programming in C++, you first need to set up your environment properly. Here are a few popular tools and IDEs you could consider:
- Visual Studio: A robust option for Windows users, offering extensive debugging and development features.
- Code::Blocks: A cross-platform IDE that’s simple yet effective for C++ development.
Basic Syntax and Structure
Writing your first C++ program is a vital step. The syntax may seem daunting initially, but it becomes intuitive with practice. Consider the following simple code snippet:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This code introduces some fundamental components:
- #include <iostream>: This line includes the standard input-output stream library.
- using namespace std;: This allows you to use names from the standard library without explicitly qualifying them.
- int main(): This is where the program execution begins.
In this snippet, the `cout` object is used to print "Hello, World!" to the console, demonstrating the basic structure of a C++ program.
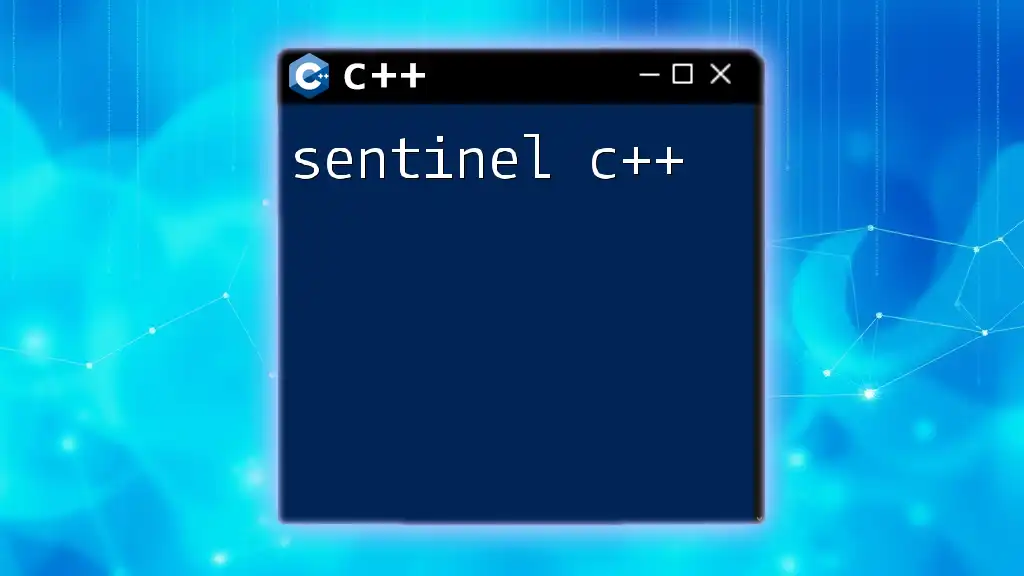
Core Concepts in C++
Variables and Data Types
In C++, variables are essential for storing data. Understanding how to declare and manipulate variables is crucial for programming effectively. C++ supports various data types:
- int: Integer type for whole numbers.
- float: Floating-point type for decimal numbers.
- char: Character type for single characters.
For example:
int age = 25;
float salary = 50000.50;
char grade = 'A';
Control Structures
Control structures guide the flow of the program.
Conditional Statements
C++ allows for conditional branching using if-else and switch statements. For instance, if you want to check if a number is positive or negative, you could use an if statement:
int number = -10;
if (number > 0) {
cout << "Positive" << endl;
} else {
cout << "Negative" << endl;
}
Looping Constructs
To perform repetitive tasks, C++ provides looping constructs like for, while, and do-while. Here’s an example using a `for` loop:
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
This structure will print the numbers 0 through 4 to the console.
Functions in C++
Functions are crucial for breaking down complex programs into manageable sections. They allow for code reusability and improved organization. A simple function could look like this:
void greet() {
cout << "Welcome to Deitel C++!" << endl;
}
You can call this function in your main program as follows:
int main() {
greet();
return 0;
}
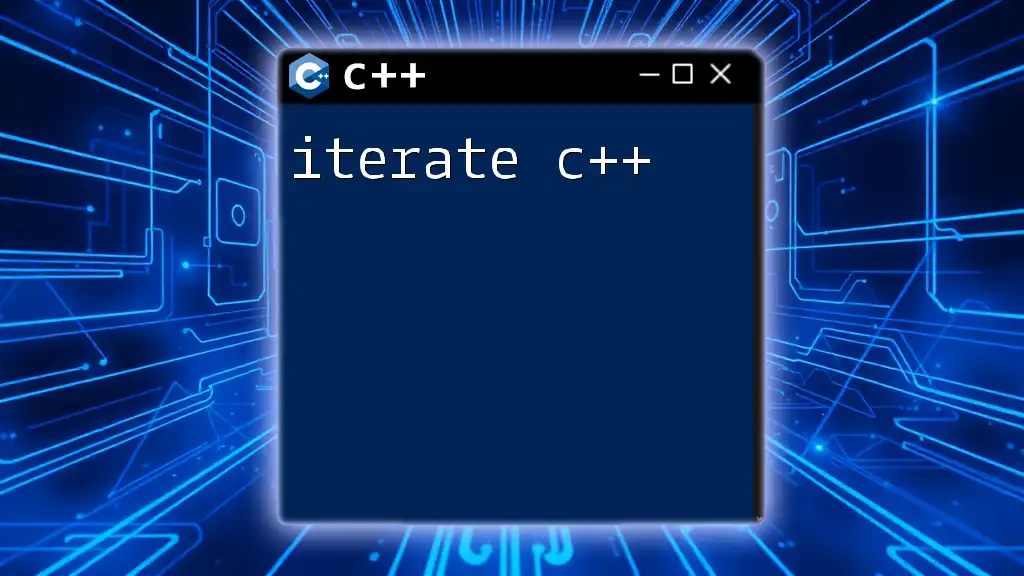
Object-Oriented Programming with C++
What is Object-Oriented Programming?
Object-oriented programming is a paradigm that uses "objects" to represent data and methods to manipulate that data. It emphasizes principles such as encapsulation, abstraction, inheritance, and polymorphism. These principles help create programs that are more intuitive and easier to manage.
Creating Classes and Objects
In C++, classes are the blueprint from which objects are created. Here’s how to define a simple class:
class Car {
public:
string brand;
void drive() {
cout << "Driving " << brand << endl;
}
};
In this class, `Car`, there is a public member variable `brand` and a method `drive()`. To create an object of the class and utilize it:
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.drive(); // Output: Driving Toyota
return 0;
}
Constructors and Destructors
Constructors are special functions called when an object is created, initializing the object's values. Destructors are invoked when an object is destroyed, cleaning up resources. Here’s an example:
class Book {
public:
Book() {
cout << "Book created!" << endl;
}
~Book() {
cout << "Book destroyed!" << endl;
}
};
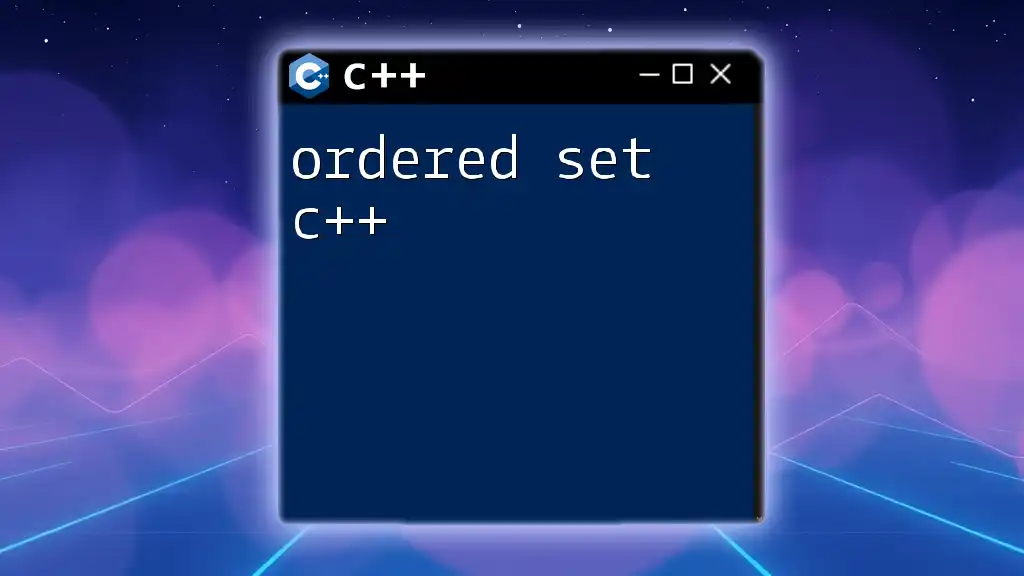
Advanced C++ Concepts
Templates and Generic Programming
Templates allow for the creation of functions and classes that work with any data type. Here is a simple function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
You can call this function with different types, such as:
cout << add<int>(5, 10); // Outputs: 15
cout << add<double>(5.5, 2.3); // Outputs: 7.8
Exception Handling
Handling errors gracefully is crucial in programming. C++ provides a structured way to manage exceptions using try, catch, and throw. Here’s how it works:
try {
throw runtime_error("An error occurred!");
} catch (runtime_error& e) {
cout << e.what() << endl; // Output: An error occurred!
}
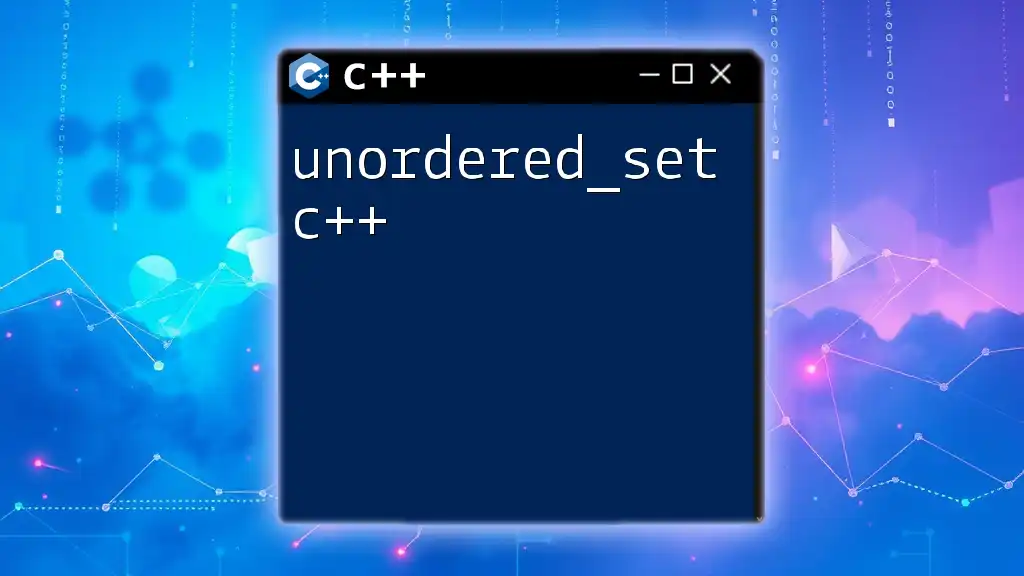
Practical Examples and Case Studies
Building a Simple C++ Application
Creating a simple calculator application is a great way to understand the concepts learned. Here’s a brief outline of steps you would take:
- Define basic operations (addition, subtraction, multiplication, division).
- Implement a user interface that accepts input.
- Use loops to allow for multiple calculations until the user decides to exit.
Real-world Applications of C++
C++ is extensively used across various industries. In gaming, developers leverage its performance for graphics rendering. In finance, C++ aids in creating high-frequency trading applications. Its versatility makes it a top choice for system software and performance-critical applications.
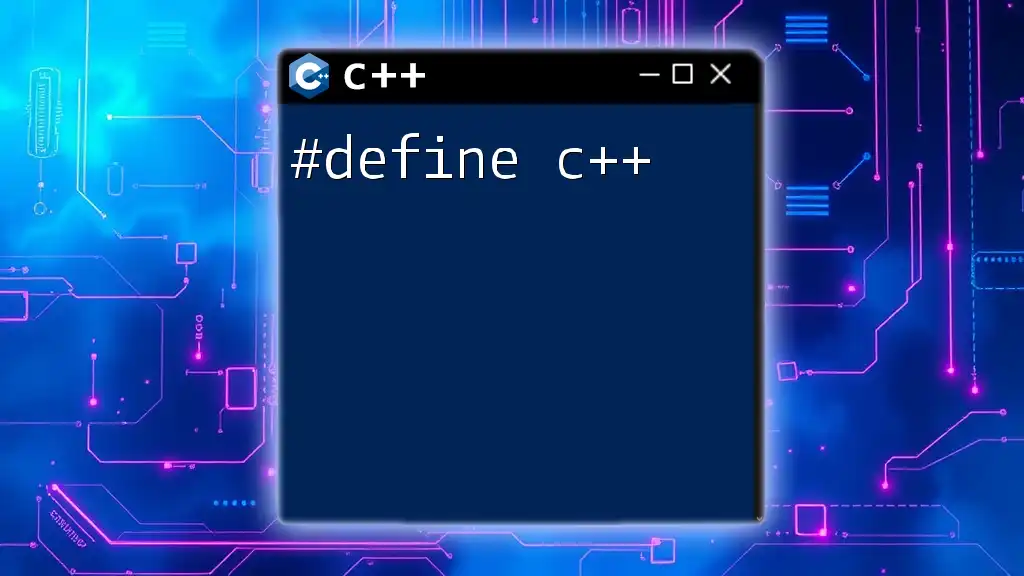
Resources for Further Learning
Deitel’s Books and Online Resources
For comprehensive learning, the Deitel C++ series is a must-read. Titles like "C++ How to Program" are essential for a deep dive into programming concepts.
Online Courses and Tutorials
To supplement your reading, consider online platforms. Websites like Coursera, Udacity, and Codecademy offer structured C++ courses that cater to all learning levels, providing quizzes and practical exercises to reinforce knowledge.
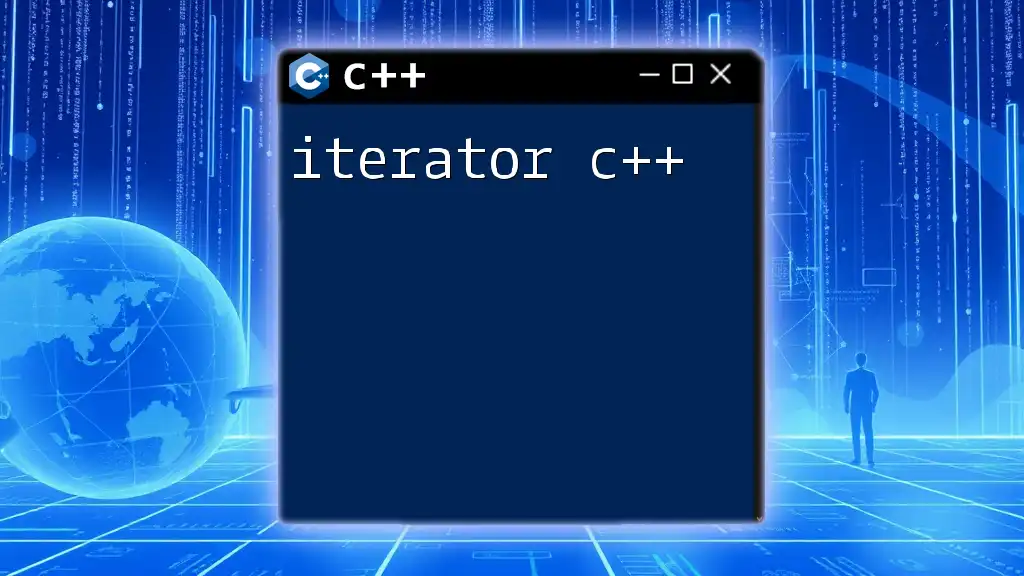
Conclusion
Investing time in learning C++ opens doors to numerous career opportunities, allowing you to engage in cutting-edge technology and software development. The skills acquired through studying Deitel’s resources will enable you to craft efficient, high-quality code.
By embracing the world of C++ programming, you're not just learning a skill; you're preparing for a future in a field brimming with possibilities. Dive into Deitel's C++ materials and embark on your programming journey today!