C++ is a powerful, high-level programming language used for various applications, while Visual C++ is an integrated development environment (IDE) provided by Microsoft that facilitates the development of C++ applications on Windows.
Here's a simple example of a C++ program using Visual C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is C++?
C++ is a powerful, high-level programming language that was developed in the early 1980s by Bjarne Stroustrup at Bell Labs. It was designed to provide low-level memory manipulation capabilities while supporting high-level object-oriented programming. One of the key features of C++ is its efficiency and flexibility, making it suitable for a wide range of applications—from system software to game development and enterprise applications.
C++ is often recognized for its rich set of features, including:
- Object-Oriented Programming (OOP), which allows developers to create reusable code.
- Standard Template Library (STL), which provides a vast array of data structures and algorithms.
- Memory Management, which gives developers direct control over memory allocation and deallocation.
Importance of C++ in Software Development
C++ holds a significant role in the field of software development due to its versatility. It is extensively used in various domains:
- System/Application Software: C++ is widely used in operating systems and key application software due to its performance efficiency.
- Game Development: Many game engines are developed using C++, allowing for high-performance graphics and real-time gameplay.
- High-Performance Applications: Industries that require real-time processing, such as finance or telecommunications, often rely on C++ due to its ability to manage hardware resources effectively.
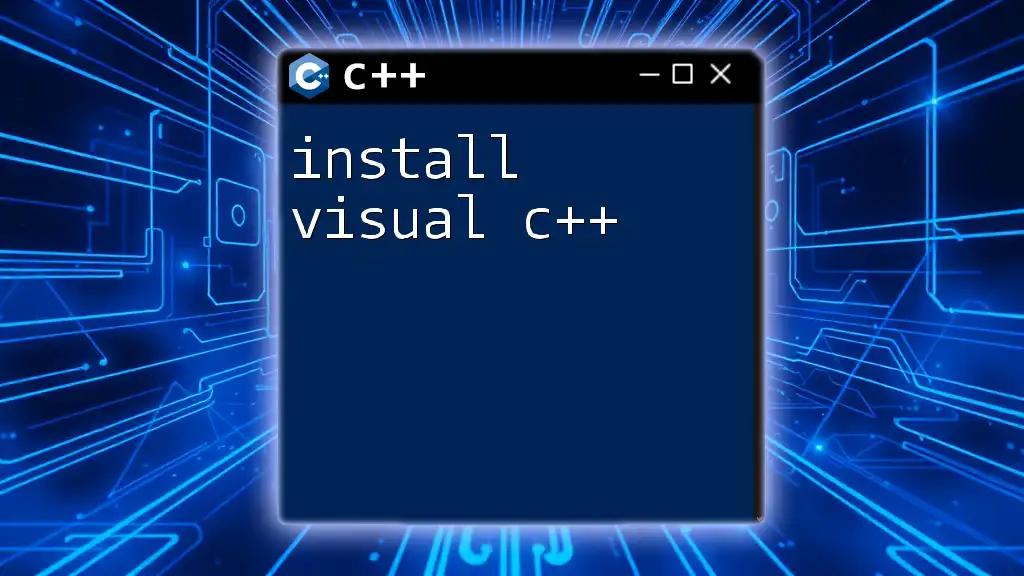
Visual C++: An Overview
What is Visual C++?
Visual C++ is Microsoft’s integrated development environment (IDE) designed for the C++ programming language. It offers a robust platform for developing Windows applications and includes a set of libraries that extend the capabilities of standard C++. Visual C++ allows developers to create high-performance applications with a graphical user interface (GUI) using Microsoft Foundation Classes (MFC).
Key Features of Visual C++
Visual C++ comes equipped with a multitude of features that streamline the development process:
- User-Friendly IDE: It provides a graphical interface that simplifies code writing and project management.
- Built-In Debugging Tools: Visual C++ includes powerful debugging tools that help developers identify and rectify issues effortlessly.
- Access to MFC: This set of classes enables developers to create Windows applications with a native feel, enhancing user experience.
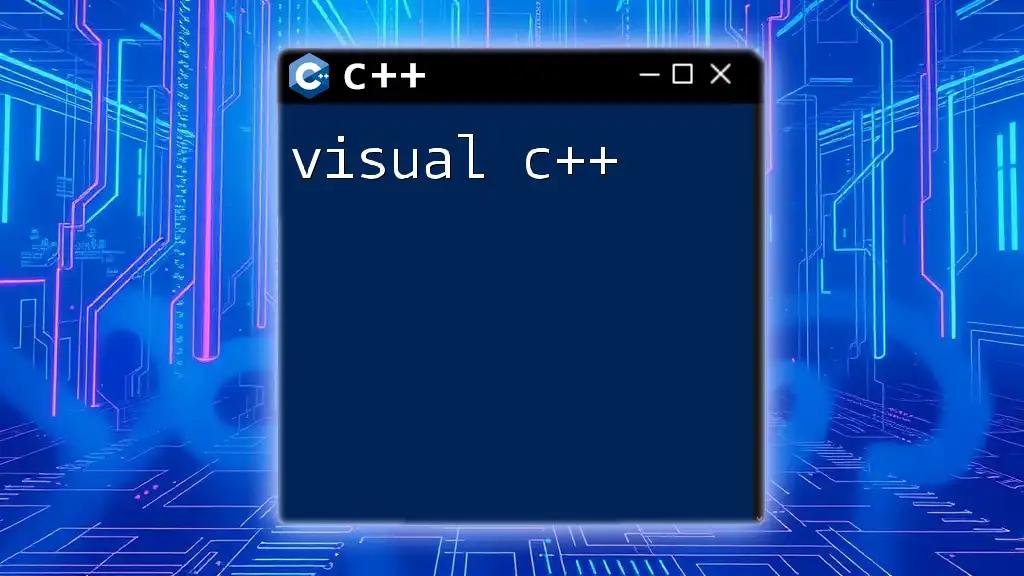
Getting Started with C++
Setting Up Your Development Environment
To kick off your journey with C++ in Visual C++, you’ll first need to set up your development environment:
- Download and install Visual Studio from the Microsoft website. During installation, ensure that you select the C++ development workload.
- Once installed, open Visual Studio and select “Create a New Project.”
Example: Creating a Simple Console Application
Creating your first project is straightforward:
- Choose “Console App” and click “Next.”
- Name your project and specify a location, then click “Create.”
- Visual Studio will set up the basic structure for you.
Understanding the Basics of C++
To navigate C++, you need to become familiar with its core concepts, including data types, variables, and control structures.
- Data Types: C++ offers several built-in data types, such as `int`, `char`, `float`, and `double`.
- Variables and Constants: You can declare variables to store data. Use `const` keyword for constants that shouldn’t change their value.
- Input and Output Operations: C++ utilizes the `iostream` library for input and output functions.
Example Code Snippet:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "You entered: " << num << endl;
return 0;
}
This simple program demonstrates basic input and output operations in C++.
- Control Structures: Use conditional statements such as `if`, `switch`, as well as loops like `for` and `while` to manage the flow of your program.
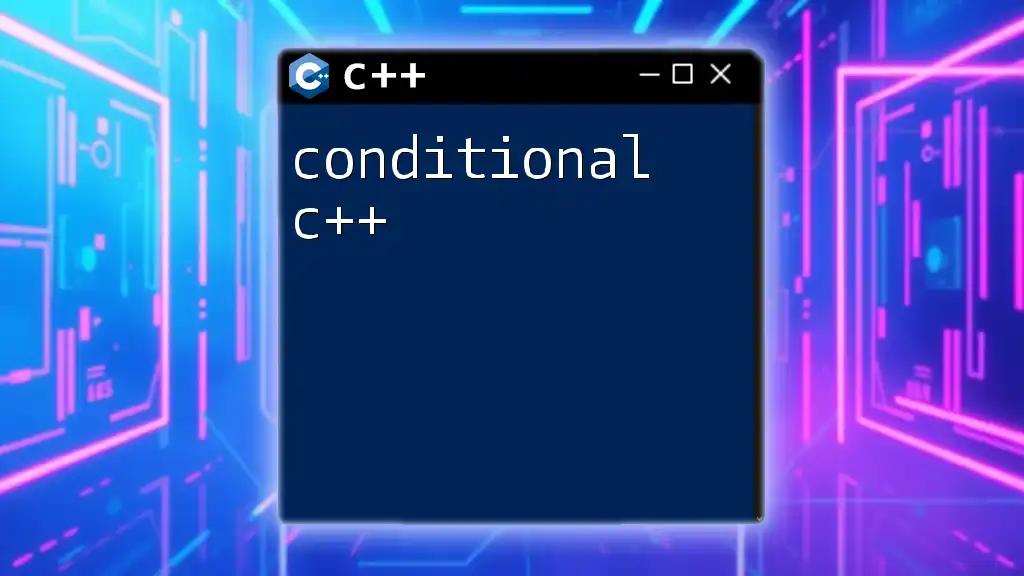
Differences Between C++ and Visual C++
Compiler and IDE
The primary distinction between plain C++ and Visual C++ lies in the compiler and the development environment. Visual C++ utilizes Microsoft's compiler, which can leverage Windows-specific features for optimized performance, while standard C++ can be compiled across various platforms.
Libraries and Frameworks
When it comes to libraries, C++ makes use of the Standard Template Library (STL) for generic programming, while Visual C++ offers access to MFC, which simplifies GUI programming on Windows. Each library serves distinct purposes, and knowledge of when to use them is essential for effective development.
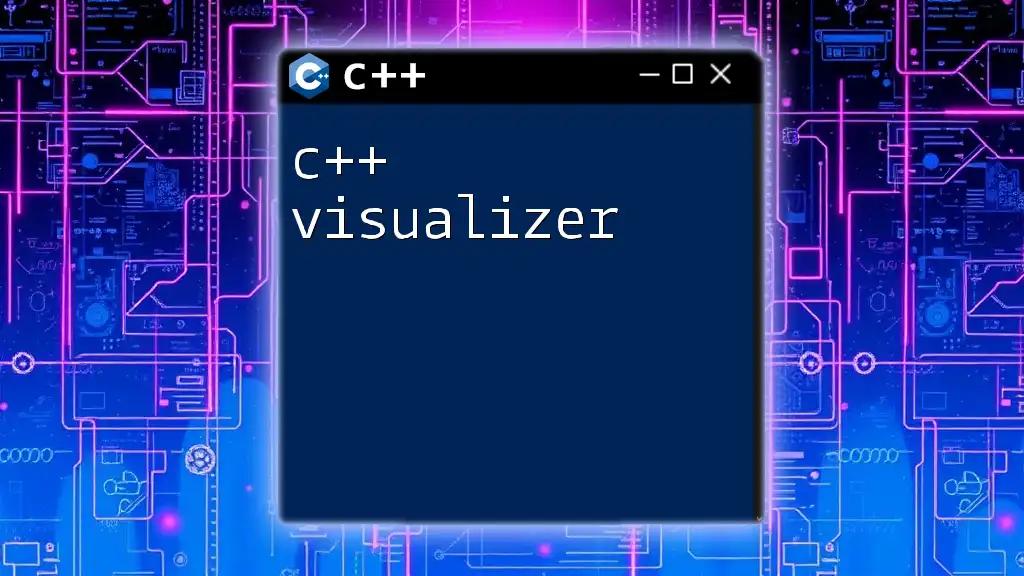
Advanced C++ Concepts in Visual C++
Object-Oriented Programming (OOP)
C++ is inherently built around object-oriented programming principles, making it essential for developers to understand OOP concepts such as encapsulation, inheritance, polymorphism, and abstraction.
Example: Creating a Class
Creating a class in C++ is simple:
class Animal {
public:
void speak() { cout << "Animal speaks!" << endl; }
};
int main() {
Animal myAnimal;
myAnimal.speak();
return 0;
}
This example outlines how to define a class and create an object that invokes a method.
Exception Handling
Exception handling is crucial for building resilient applications. By utilizing the `try-catch` blocks, developers can ensure that errors do not crash the application.
Example Code Snippet
try {
// Code that may throw an exception
} catch (const std::exception& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
This snippet demonstrates how to catch and handle exceptions effectively.
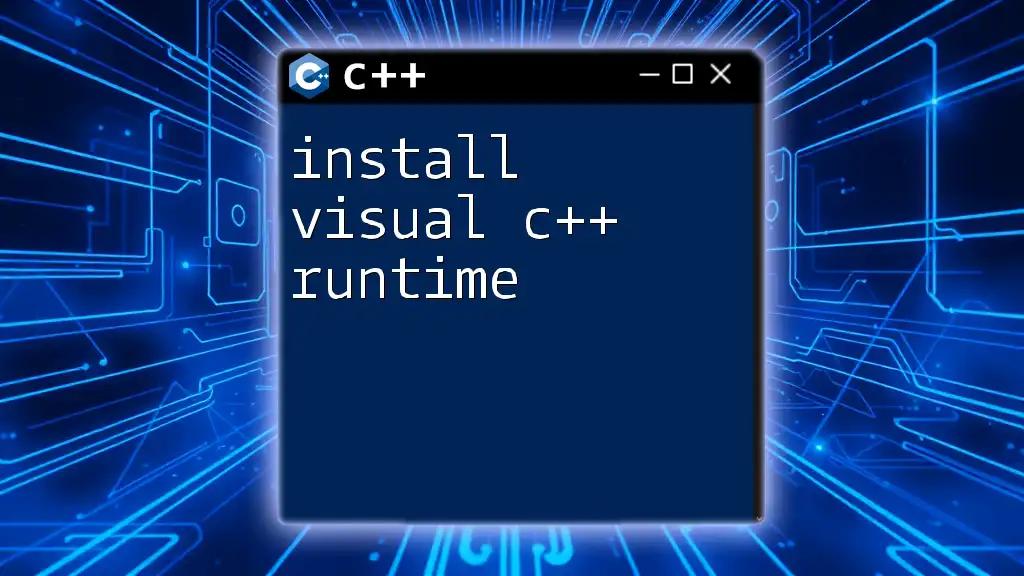
Debugging and Testing in Visual C++
Using Built-In Debugging Tools
Visual C++ provides a comprehensive suite of debugging features. From setting breakpoints to stepping through code, it simplifies the debugging process. Developers can inspect variable values and call stacks, aiding in identifying logical errors during execution.
Unit Testing in Visual C++
Unit testing is an essential practice to ensure the reliability of code. Visual C++ supports various testing frameworks, enabling developers to write tests that verify the functionality of individual components.
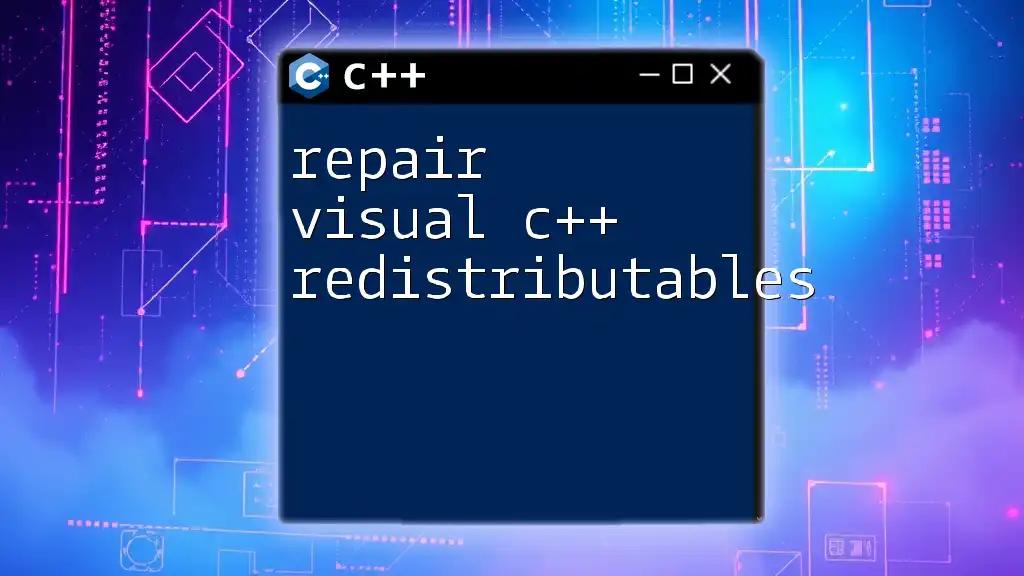
Best Practices for C++ Programming in Visual C++
Code Organization
Structured code organization is vital for maintainability. Adopting modular approaches using header and source files, alongside meaningful naming conventions and commenting, enhances code readability.
Performance Optimization Techniques
C++ provides numerous tools for performance optimization. It’s crucial to avoid common pitfalls such as memory leaks and unnecessary object copies. Employing profiling tools allows developers to analyze performance metrics and optimize their applications accordingly.
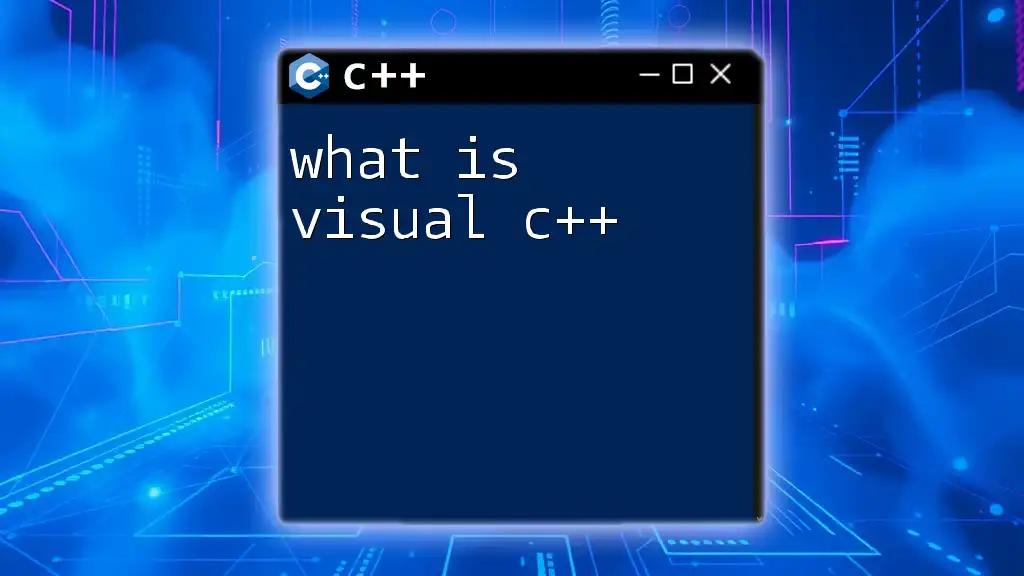
Conclusion
In summary, mastering C++ and Visual C++ provides a strong foundation for any software developer. The integration of C++ with Visual Studio's features expands the horizons of what can be achieved in application development.
As you embark on your journey with C++, explore further with advanced resources and tutorials. Consider enrolling in specialized courses to gain hands-on experience and deepen your understanding. The world of C++ programming awaits, full of possibilities to create efficient, high-quality software.
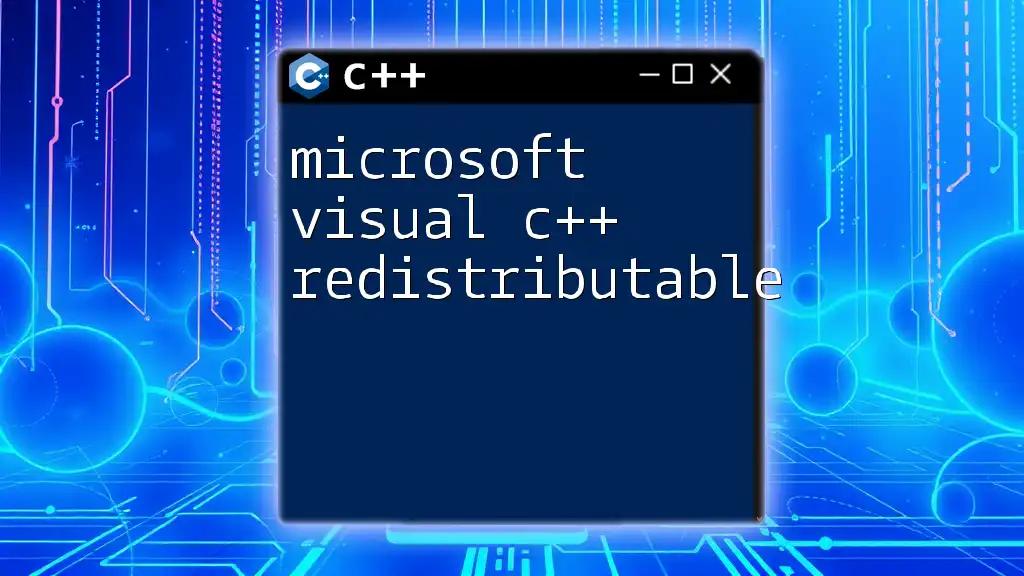
Additional Resources
To continue your learning, refer to foundational books, official documentation, and online communities. Engaging with fellow developers will bolster your skills and open new opportunities in the realm of C++ programming.