Starting out with C++ involves understanding the basic syntax and structure of the language, which is essential for writing efficient programs.
Here’s a simple "Hello, World!" example in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Your C++ Environment
Choosing a C++ Compiler
To get started with C++, you first need a C++ compiler. A compiler translates your C++ code into executable programs, making it essential for building applications. Popular compilers include:
- GCC (GNU Compiler Collection): Widely used in UNIX-like systems and supports multiple programming languages.
- Clang: Known for its fast compilation and detailed error messages, making it a great choice for beginners.
- MSVC (Microsoft Visual C++): A powerful option for Windows users.
To install a compiler, follow the respective documentation for your operating system, ensuring you set the environment variables correctly for easy command-line usage.
Integrated Development Environments (IDEs)
Using an Integrated Development Environment (IDE) simplifies C++ programming by providing features like code highlighting, debugging, and compilation tools. Here are some recommended IDEs:
- Code::Blocks: A free, open-source IDE that supports multiple compilers and is ideal for beginners.
- Visual Studio: A comprehensive IDE with advanced debugging features, particularly suited for Windows development.
- CLion: A commercial IDE with smart code assistance and built-in tools to boost productivity.
Setting up your first C++ project typically involves creating a new project in your chosen IDE, configuring the build settings, and adding your source files.
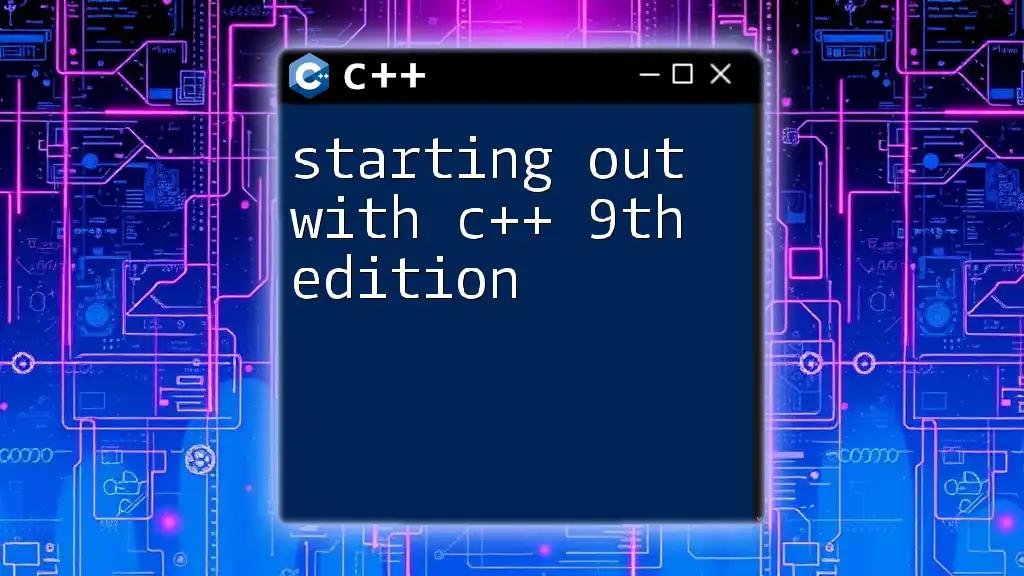
Understanding the Basics of C++
C++ Syntax and Structure
C++ syntax is crucial to understand as it dictates how your code is structured. A basic C++ program follows this outline:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This simple example showcases how to include a library, declare the main function, and print text to the console. Key components include:
- `#include <iostream>`: This directive includes the Input/Output stream library, allowing you to use standard I/O operations.
- `using namespace std;`: Simplifies access to standard library elements.
- `int main()`: The main function where program execution starts.
- `cout`: Outputs text to the console.
Data Types and Variables
C++ supports various fundamental data types, such as:
- int: Integer values
- float: Floating-point numbers
- char: Single characters
- bool: Boolean values (true/false)
Declaring a variable involves specifying the data type followed by the variable name. For instance:
int age = 30;
float salary = 50000.5;
In this example, `age` is an integer set to 30, while `salary` is a float initialized to 50000.5. Understanding data types helps you choose the right type for your variables based on the data they will hold.
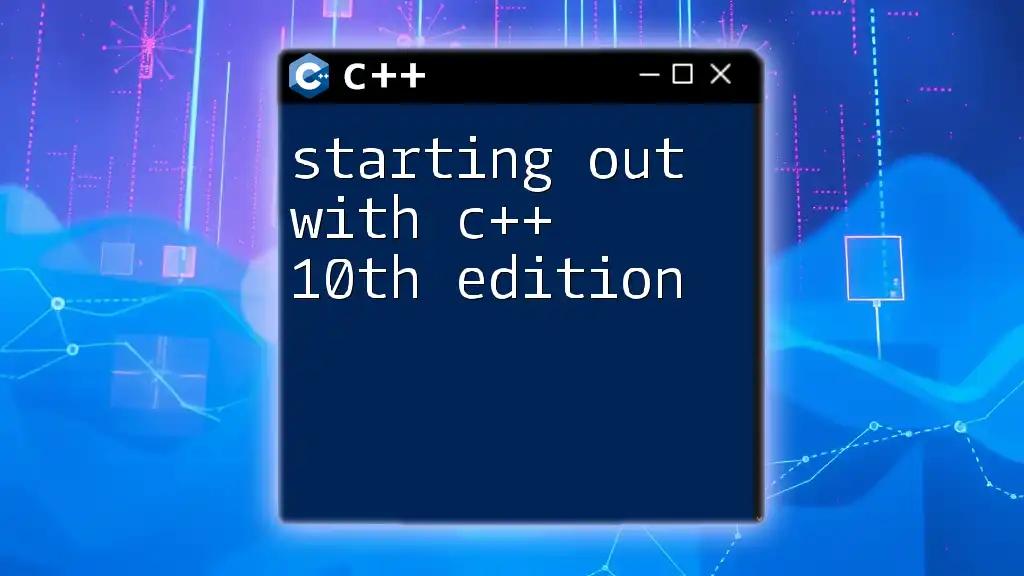
Control Structures in C++
Conditional Statements
Control structures direct the flow of your program based on conditions. Conditional statements, such as `if`, `else if`, and `else`, allow your program to take different actions based on different conditions. For instance:
if (age >= 18) {
cout << "You are an adult." << endl;
} else {
cout << "You are not an adult." << endl;
}
In this example, if `age` is 18 or older, the program outputs "You are an adult." Otherwise, it states otherwise. Good practice involves ensuring all conditions are mutually exclusive to avoid logical errors.
Switch Case Statement
The switch case statement is an alternative to multiple `if-else` conditions and can improve code readability. It's particularly useful when evaluating a single expression for multiple possible outcomes.
switch (day) {
case 1:
cout << "Monday" << endl;
break;
case 2:
cout << "Tuesday" << endl;
break;
default:
cout << "Not a valid day" << endl;
}
Here, depending on the value of `day`, the program will print the corresponding day of the week.
Loops in C++
Loops allow repeating instructions until a condition is met. C++ offers three types: for, while, and do-while loops.
- For Loop: Ideal when you know how many iterations you want to perform.
int sum = 0;
for (int i = 1; i <= 5; i++) {
sum += i; // Accumulate sum from 1 to 5
}
cout << "Sum: " << sum << endl;
- While Loop: Useful when the iterations are dependent on a condition, such as waiting for user input.
int num;
while (num != 0) {
cout << "Enter a number (0 to exit): ";
cin >> num;
}
- Do-While Loop: Similar to the while loop, but it guarantees at least one execution of the code block.

Working with Functions
Defining and Calling Functions
Functions in C++ are self-contained blocks of code designed to perform a specific task. They promote code reuse and organization.
To define a function:
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
Calling this function can be done as follows:
int result = add(5, 3);
cout << "The result is: " << result << endl;
Function Overloading
C++ allows multiple functions to have the same name as long as their parameter lists differ. This is known as function overloading. For instance:
int add(int a, int b) {
return a + b;
}
float add(float a, float b) {
return a + b;
}
Here, the `add` function can work with both integers and floats, enhancing flexibility.
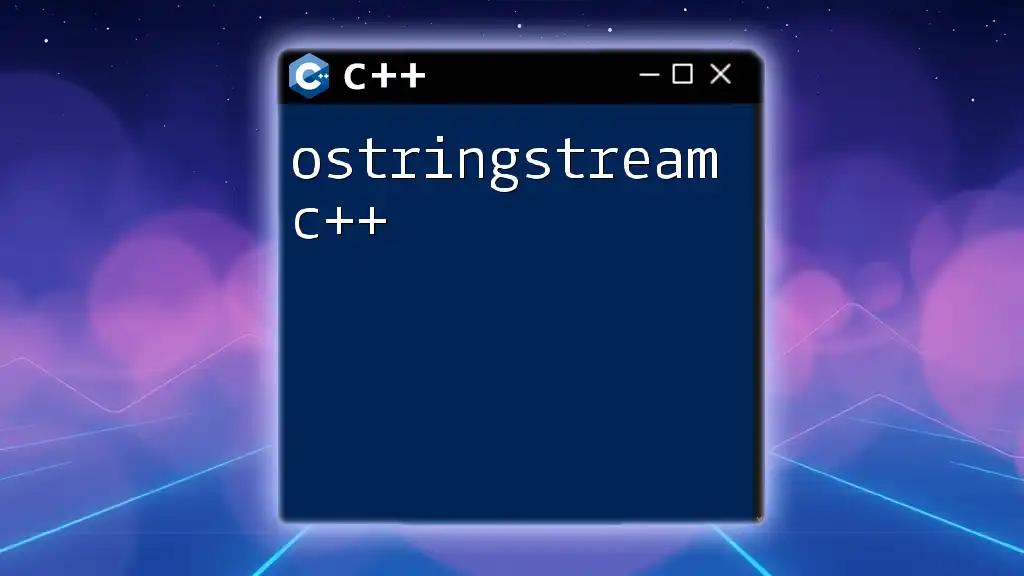
Introduction to Object-Oriented Programming (OOP) in C++
What is OOP?
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of "objects" that can contain both data and methods. OOP promotes the principles of encapsulation, inheritance, and polymorphism, leading to better organization and reuse of code.
Creating Classes and Objects
In C++, classes define blueprints for creating objects. A class encapsulates data for the object and methods for manipulating that data.
Example of defining a class:
class Car {
public:
void drive() {
cout << "Car is driving!" << endl;
}
};
// Creating an object of Car class
Car myCar;
myCar.drive();
In this example, the `Car` class has a method named `drive`. When you create an object (`myCar`) from this class and call `drive()`, it executes the associated method.
Constructors and Destructors
Constructors are special functions that are called when an object is created, initializing it to a valid state. Conversely, destructors are called when an object is destroyed, allowing for cleanup processes.
Example:
class Person {
public:
Person() {
cout << "Constructor called!" << endl; // Constructing the object
}
~Person() {
cout << "Destructor called!" << endl; // Cleaning up
}
};
// Usage
Person p; // Constructor is called upon creation
In this instance, when an object of type `Person` is instantiated, the constructor initializes it, and when it goes out of scope, the destructor is invoked.

Conclusion
Starting out with C++ is all about mastering its foundational concepts, such as data types, control structures, functions, and object-oriented programming principles. Learning these fundamentals paves the way for tackling more complex topics and real-world applications.
Next Steps
To deepen your C++ knowledge, consider exploring advanced topics such as templates, exception handling, and the Standard Template Library (STL). Engaging with community resources, online courses, and practice projects will enrich your learning journey and lead to greater confidence and skill in C++. Keep coding and enjoy your C++ adventure!

Additional Resources
Familiarize yourself with useful C++ libraries like STL, and explore the vast array of documentation available. Joining communities such as Reddit or Discord groups for C++ learners will provide ongoing support and foster collaborative learning, allowing you to connect with fellow programmers as you progress.