"Starting Out with C++ Early Objects 10th Edition" is a beginner-friendly resource that introduces fundamental programming concepts using C++ through practical examples and exercises to build a solid foundation.
Here’s a simple code snippet that demonstrates how to create and use a class in C++:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Calls the bark function
return 0;
}
Understanding C++ and Object-Oriented Programming
What Is C++?
C++ is a versatile, high-performance programming language that combines both low-level and high-level features. Its ability to interface closely with hardware while providing powerful abstraction makes it a preferred choice for system programming, game development, and real-time applications. Some of its key features include:
- Compiled Language: C++ is a compiled language, meaning the code is translated into machine-readable form, enhancing execution speed.
- Platform Independence: With the right compiler, C++ code can run on multiple platforms without modification.
- Efficiency: C++ is designed for performance, allowing fine-tuned control over system resources.
Introduction to Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming paradigm that uses "objects" to model real-world entities. The core concepts include:
- Encapsulation: Bundling data and methods that operate on the data within classes.
- Inheritance: Deriving new classes from existing classes, promoting code reuse.
- Polymorphism: Allowing methods to do different things based on the object invoking them, which simplifies code complexity.
- Abstraction: Hiding complex implementation details and exposing only the necessary features.
Learning C++ with an OOP approach helps you develop a more intuitive understanding of relationships in code, making your programs not only more manageable but also easier to follow.
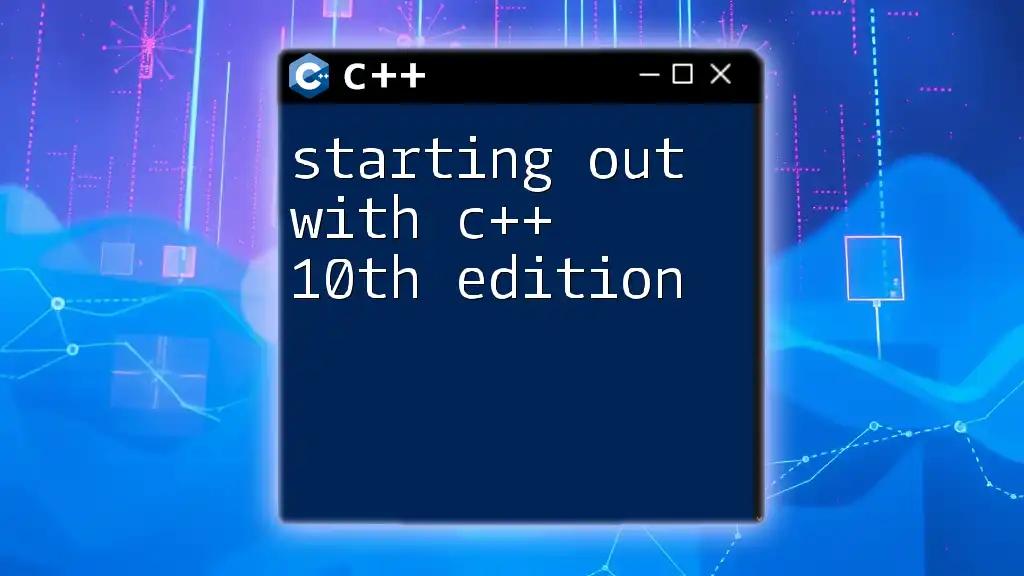
Getting Started with the Early Objects Approach
Overview of Early Objects
The early objects approach emphasizes the introduction of object-oriented principles at the beginning of your C++ journey. This method highlights the relationship between data and functionality, helping students grasp the concept of objects as cohesive units from the outset.
Benefits of Using Early Objects in C++
- Real-World Modeling: Encourages thinking about code in terms of objects that represent real-world items, improving design intuition.
- Simplified Learning Curve: By using objects early, beginners can focus on the practical application of programming concepts rather than theoretical constructs.
Setting Up Your Development Environment
To start coding in C++, you’ll need to set up a development environment. Here’s how to do it:
Required Tools
- Compilers: Choose a compiler like GCC (GNU Compiler Collection) or the Microsoft Visual C++ compiler.
- IDEs: Integrated Development Environments (IDEs) like Visual Studio, Code::Blocks, or Code::Lite streamline coding, compiling, and debugging processes.
Installation Instructions
- Download the Compiler: Visit the official sites to download the appropriate compiler for your operating system.
- Install an IDE: Follow the installation guide for your chosen IDE, ensuring compatibility with your compiler.
- Create Your First Project: Open your IDE and create a new project to start coding.
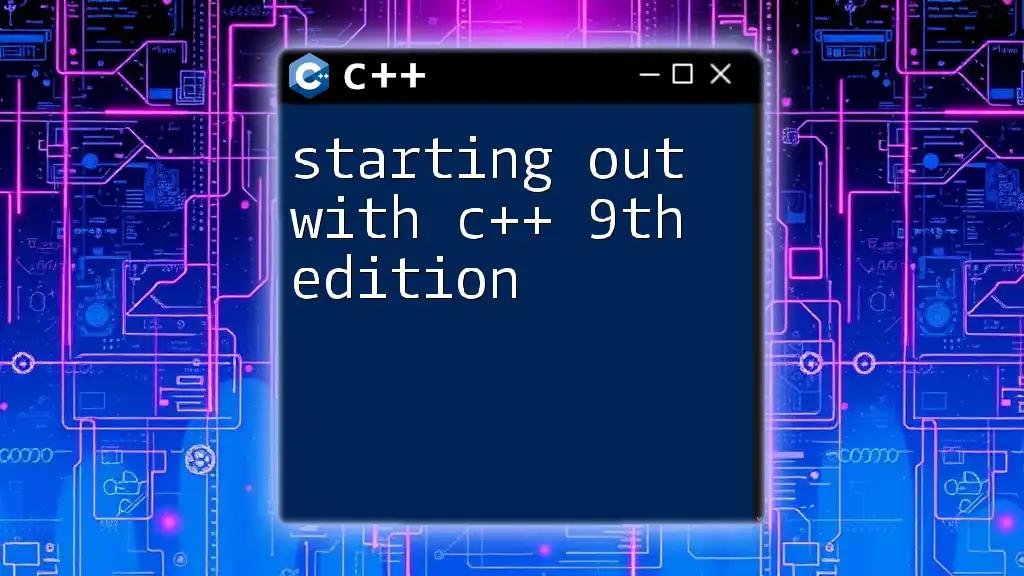
The Basics of C++ Syntax
Writing Your First C++ Program
Let’s create a simple program that prints "Hello, World!". This popular first step in programming exemplifies basic syntax and structure:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Explanation of Each Part
- `#include <iostream>`: This line includes the Input/Output stream library enabling the use of `cout` and `cin`.
- `using namespace std;`: Avoids prefixing standard functions with `std::`.
- `int main()`: Defines the main function where program execution begins.
- `cout << "Hello, World!" << endl;`: Outputs the string to the console.
- `return 0;`: Indicates that the program ran successfully.
Understanding Basic Syntax and Structure
Familiarizing yourself with the fundamental syntax of C++ is vital. Here are key components:
- Comments: Use `//` for single-line comments and `/* ... */` for multi-line comments.
- Variables and Data Types: C++ supports various data types such as `int`, `float`, `char`, and `bool`.
- Operators: Familiarize yourself with arithmetic, relational, and logical operators.
Control Structures
Decision-Making Statements
Control structures allow you to dictate the flow of your program. For example, using if-else statements lets you make decisions based on conditional expressions:
int number = 10;
if (number > 0) {
cout << "Positive Number" << endl;
} else {
cout << "Not a Positive Number" << endl;
}
Loops
Loops are essential for performing repetitive tasks. C++ supports several types of loops, including the `for` loop and `while` loop. Here’s a basic example of a `for` loop:
for (int i = 0; i < 5; i++) {
cout << "Iteration " << i << endl;
}
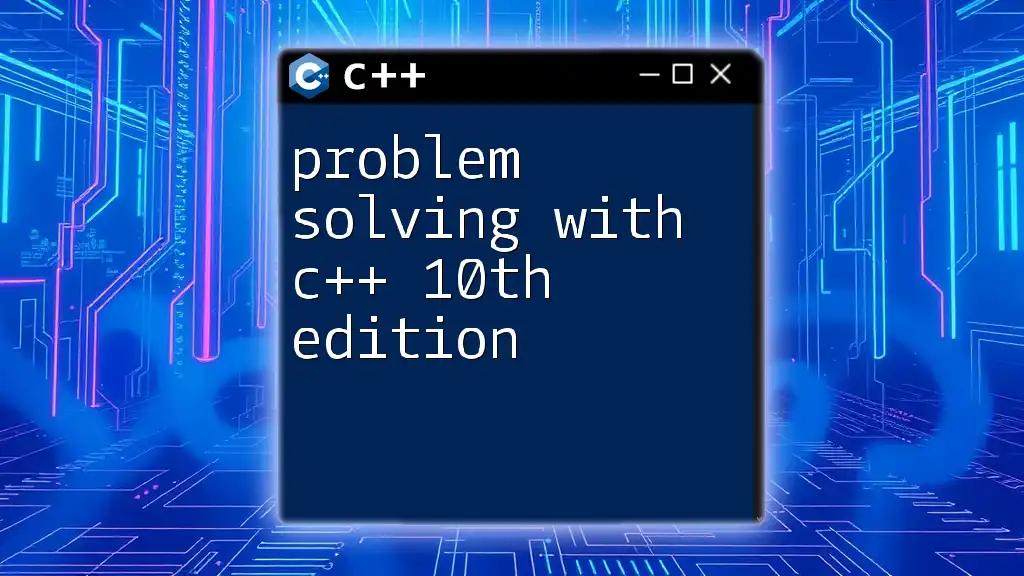
Diving Deeper: Object Creation and Manipulation
Defining and Using Classes
Classes are central to the object-oriented paradigm. They encapsulate attributes and methods. Here’s how to define a simple class:
class Car {
public:
string brand;
int year;
void display() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
To create an object of the `Car` class and use it:
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2022;
myCar.display();
return 0;
}
Utilizing Constructors and Destructors
Constructors are special member functions invoked when an object is created. A destructor is used to clean up before the object is destroyed. Here’s an example of both:
class Book {
public:
string title;
Book(string t) : title(t) {
cout << "Book created: " << title << endl;
}
~Book() {
cout << "Book destroyed: " << title << endl;
}
};
int main() {
Book myBook("The Great Gatsby");
return 0;
}
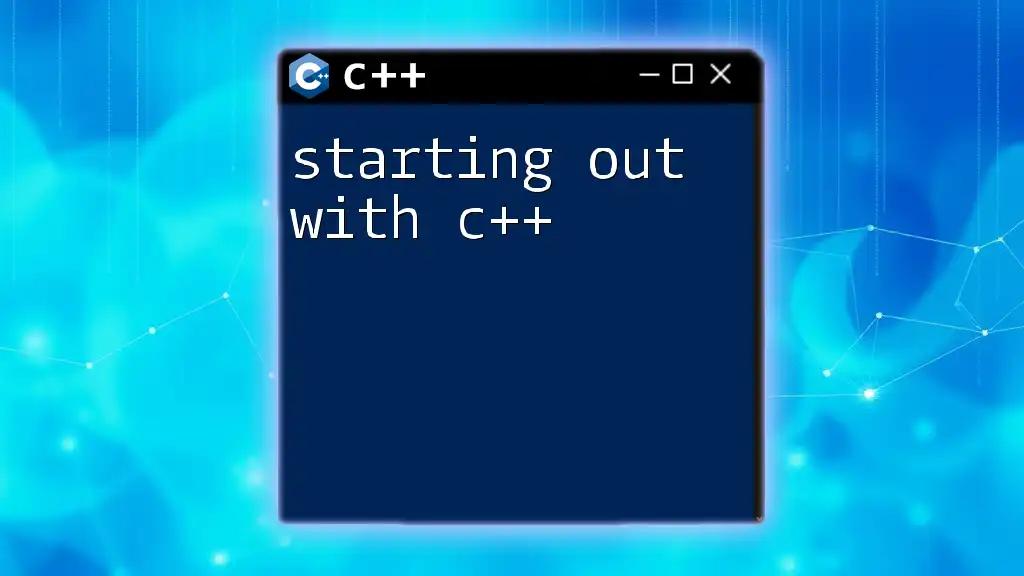
Working with Object-Oriented Principles
Inheritance
Inheritance allows one class (the derived class) to inherit properties and behaviors from another class (the base class). Here’s an example showcasing inheritance:
class Vehicle {
public:
void drive() {
cout << "Driving a vehicle." << endl;
}
};
class Car : public Vehicle {
public:
void horn() {
cout << "Car is honking!" << endl;
}
};
int main() {
Car myCar;
myCar.drive(); // Inherited method
myCar.horn(); // Car's own method
return 0;
}
Polymorphism
Polymorphism enables methods to execute in different ways based on the object type. This is primarily achieved through function overloading and overriding.
class Shape {
public:
virtual void draw() {
cout << "Drawing a shape." << endl;
}
};
class Circle : public Shape {
public:
void draw() override {
cout << "Drawing a circle." << endl;
}
};
int main() {
Shape* s;
Circle c;
s = &c;
s->draw(); // Calls Circle's draw() due to polymorphism
return 0;
}

Best Practices in C++ Programming
Writing Clean and Maintainable Code
Code readability is crucial for collaboration and maintenance. Use meaningful identifiers and comments where necessary, ensuring that your code is easy to understand for yourself and others.
Debugging Strategies
Debugging is an essential skill for programmers. Utilizing IDE tools such as breakpoints, watch windows, and integrated debuggers can significantly enhance your debugging experience.
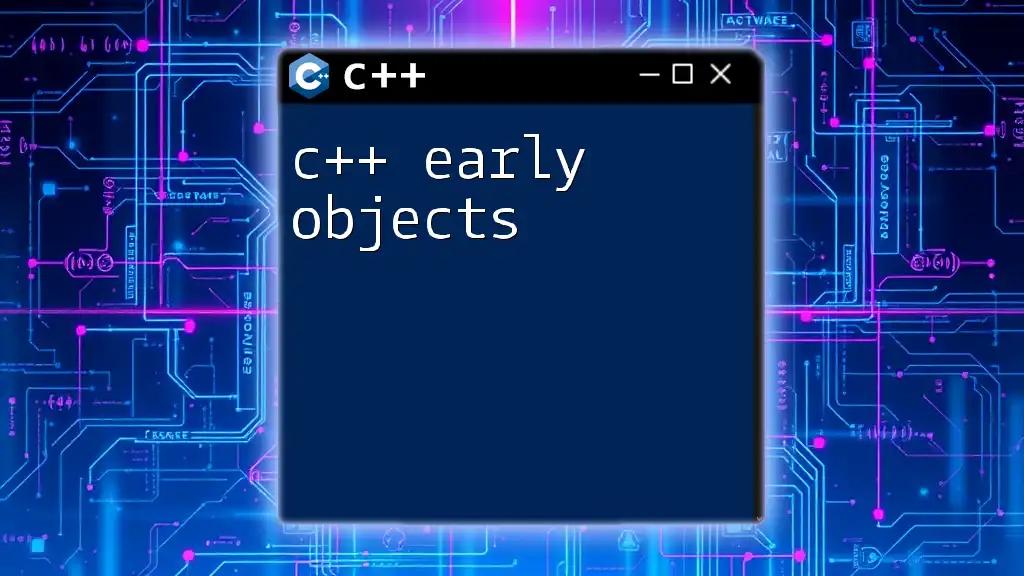
Resources for Continued Learning
Once you have grasped the fundamentals, explore further resources to deepen your understanding. Recommended materials include:
- Books: "C++ Primer" and "Effective C++" are great companions for advanced comprehension.
- Online Courses: Websites like Coursera and edX offer C++ courses tailored for various skill levels.
- Communities: Engage in forums and communities like Stack Overflow or Reddit, where you can ask questions and exchange knowledge with fellow C++ enthusiasts.
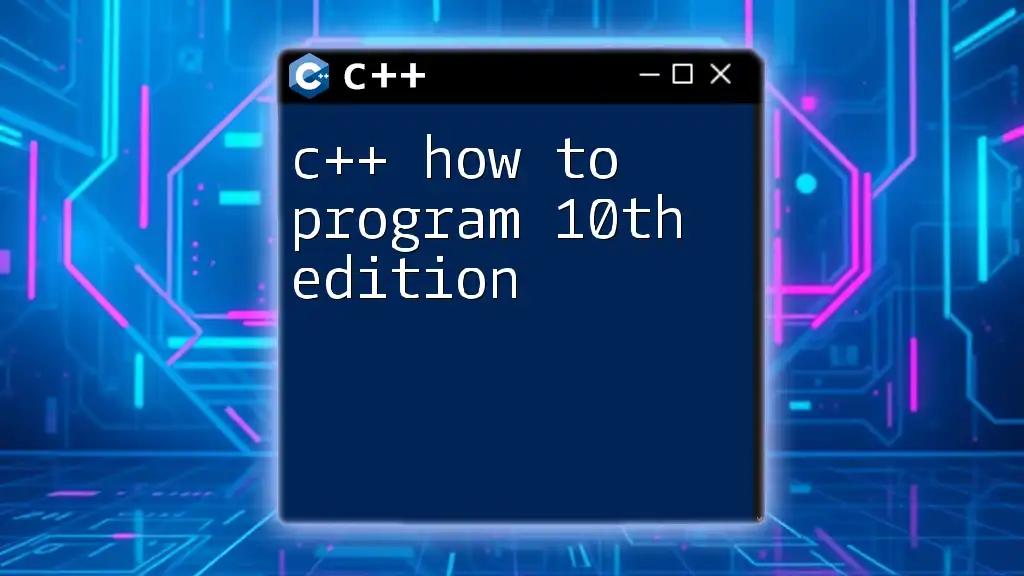
Conclusion
Starting out with C++ using the Early Objects approach equips you with the foundational understanding necessary to tackle real-world problems through programming. This guide provides a structured pathway to mastering C++, emphasizing key concepts and hands-on examples. Remember, practice is crucial; don’t hesitate to experiment with the concepts learned here. Welcome to the dynamic world of C++ programming!