"Starting out with C++ 9th Edition introduces beginners to the foundational concepts and syntax of the language, empowering them to write simple programs quickly."
Here's a basic example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Your C++ Development Environment
Choosing the Right IDE
When starting out with C++ 9th edition, selecting the right Integrated Development Environment (IDE) is crucial. Popular choices include:
- Visual Studio: Known for its powerful debugging tools and productivity features. It's a great option for Windows users.
- Code::Blocks: Lightweight and easy to set up, making it a good choice for beginners. It's available on multiple platforms.
- CLion: A feature-rich IDE from JetBrains that supports various C++ standards and offers sophisticated code analysis.
After deciding on an IDE, follow the installation instructions provided on their official websites to get started.
Compilers and Build Systems
A compiler is a program that translates your C++ code into machine language that can be executed. Understanding how to set up a compiler is essential.
To install a compiler such as GCC (GNU Compiler Collection) or Clang, you can follow these steps:
- Windows: Install MinGW, which includes the GCC compiler. Download it from the MinGW website and follow the setup instructions.
- macOS: Use Homebrew and run the command `brew install gcc`.
- Linux: Most distributions come pre-installed with GCC; if not, you can typically install it via the package manager (e.g., `sudo apt install build-essential` for Ubuntu).
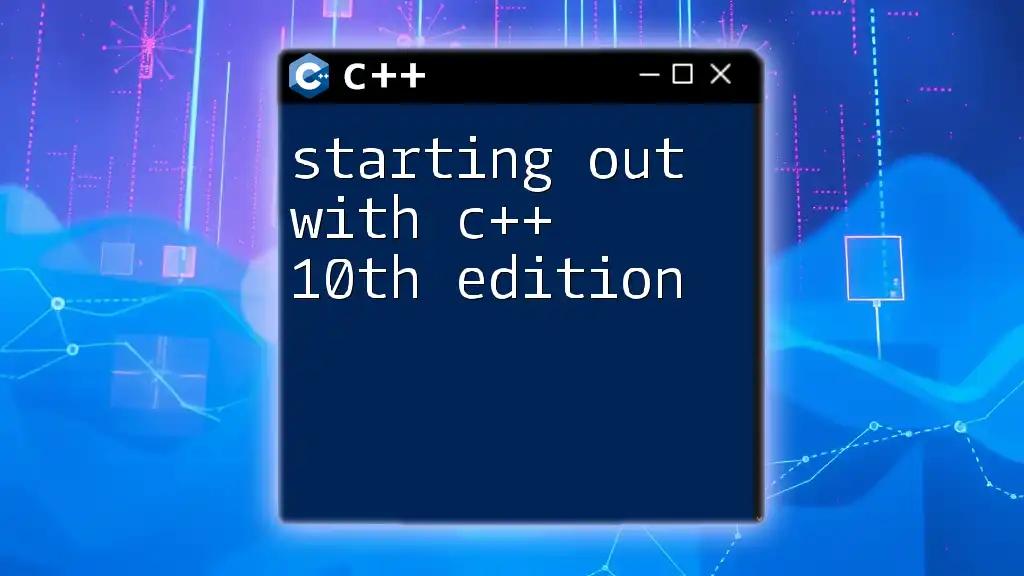
Core Concepts of C++
Variables and Data Types
Understanding variables is fundamental to programming. A variable is a storage location identified by a name, and it holds a value that can be changed during execution.
Example:
int age = 30;
double salary = 50000.50;
In this example, we declare an integer variable `age` and a double variable `salary`. The primary data types in C++ include:
- int: For integer values.
- float: For floating-point numbers.
- char: For single characters.
- bool: For boolean values (true/false).
Control Structures
Control structures enable you to dictate the flow of your program based on conditions or repetitions.
Conditional Statements
The `if`, `else if`, and `else` statements allow you to execute blocks of code based on specific conditions.
Example:
if (age > 18) {
cout << "Adult";
} else {
cout << "Minor";
}
This code checks the value of `age` and prints "Adult" if the condition is met, or "Minor" otherwise.
Loops
Loops are essential for executing a block of code multiple times.
Using for loops allows you to repeat actions based on a condition.
Example:
for (int i = 0; i < 10; i++) {
cout << i;
}
This code snippet prints numbers from 0 to 9, incrementing by one each iteration.
Functions in C++
Functions are critical for modular programming. They allow you to encapsulate code into reusable blocks.
Example of Defining and Calling Functions:
void greet() {
cout << "Hello, World!";
}
You can call this function in your `main()` to display the greeting.
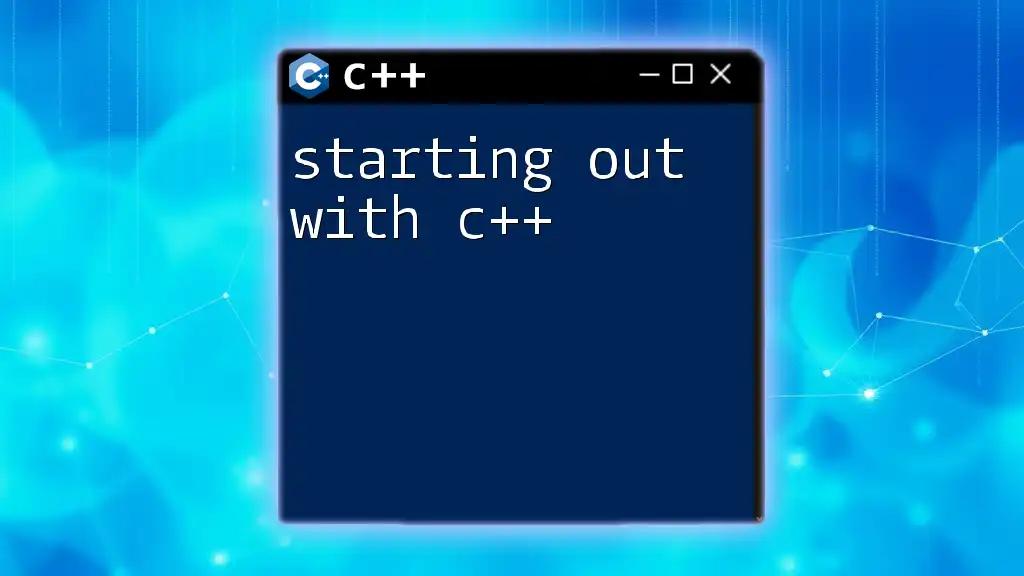
Object-Oriented Programming (OOP) in C++
Classes and Objects
Object-Oriented Programming allows for encapsulating data and functions within objects. A class acts as a blueprint for creating objects.
Example; Creating a Class:
class Car {
public:
string brand;
void honk() {
cout << "Beep beep!";
}
};
In this example, the `Car` class has a member variable `brand` and a method `honk()` that prints a message.
Inheritance and Polymorphism
Inheritance allows a class to inherit attributes and methods from another class, promoting code reusability.
Example of Inheritance:
class Vehicle {
public:
void start() { cout << "Vehicle started"; }
};
class Bike : public Vehicle {
public:
void honk() { cout << "Bike honked"; }
};
In the code above, `Bike` inherits from `Vehicle`, enabling it to access the `start()` method.
Encapsulation and Abstraction
Encapsulation refers to bundling data with methods that operate on that data, restricting direct access to some components. Abstraction simplifies complex systems by providing a limited interface, allowing users to interact easily without needing to understand all details.

Standard Template Library (STL)
Introduction to STL
The Standard Template Library is a powerful suite of pre-defined classes and functions. It includes templates for data structures, such as vectors and lists, which enhance flexibility with generic programming.
Using Vectors and Lists
Working with Vectors:
Vectors are dynamic arrays that resize automatically. They are preferred for their flexibility and ease of use.
Example of Vector Usage:
#include <vector>
using namespace std;
vector<int> numbers;
numbers.push_back(1);
numbers.push_back(2);
In this example, we create a vector called `numbers` and add two integers.
Difference Between Vectors and Lists
Vectors are stored in contiguous memory, making them faster for access, while lists allow for efficient insertions and deletions but have slower access times.
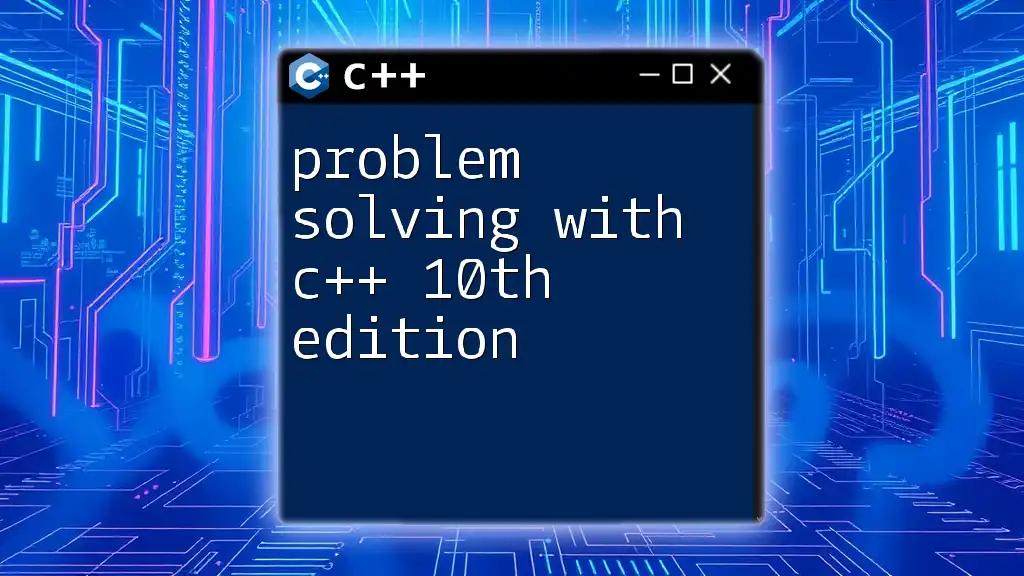
Error Handling in C++
Exceptions
Error handling is an integral part of robust programming. C++ uses exceptions to manage errors effectively.
Example of Using Try-Catch Blocks:
try {
// Code that may throw an exception
int result = 10 / 0; // Division by zero
} catch (const std::exception &e) {
cout << e.what(); // Handle the exception
}
In this example, if the division by zero occurs, the program does not crash. Instead, it goes to the "catch" block, where it can handle the error gracefully.
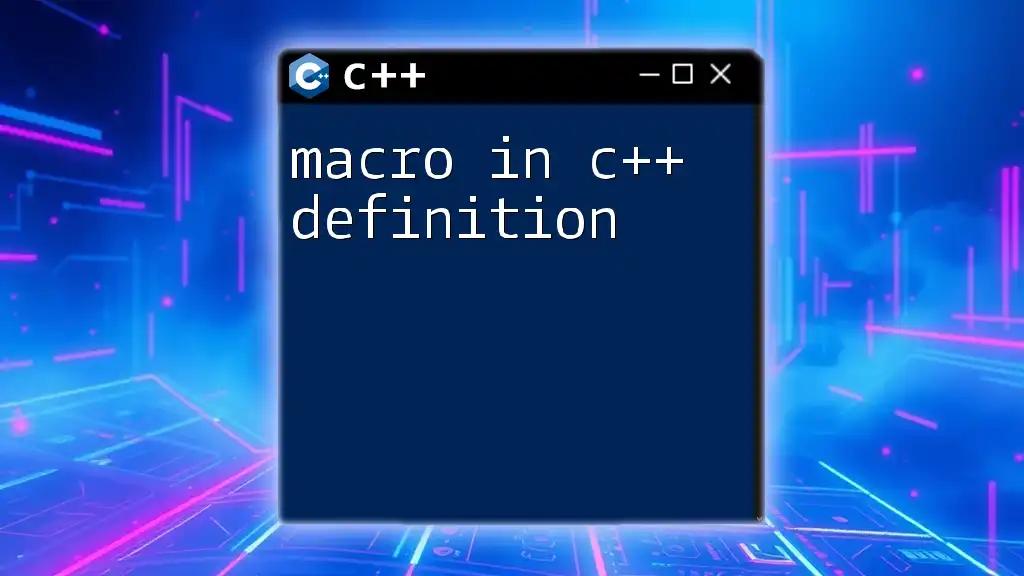
Best Practices for Writing C++ Code
Code Readability and Maintenance
When starting out with C++ 9th edition, writing clean code is vital. Use clear naming conventions, maintain consistent indentation, and adhere to a coherent coding style to enhance overall readability.
Commenting Your Code
Effective commenting is crucial for understanding complex code blocks. It helps both you and others interpret your intentions in the code.
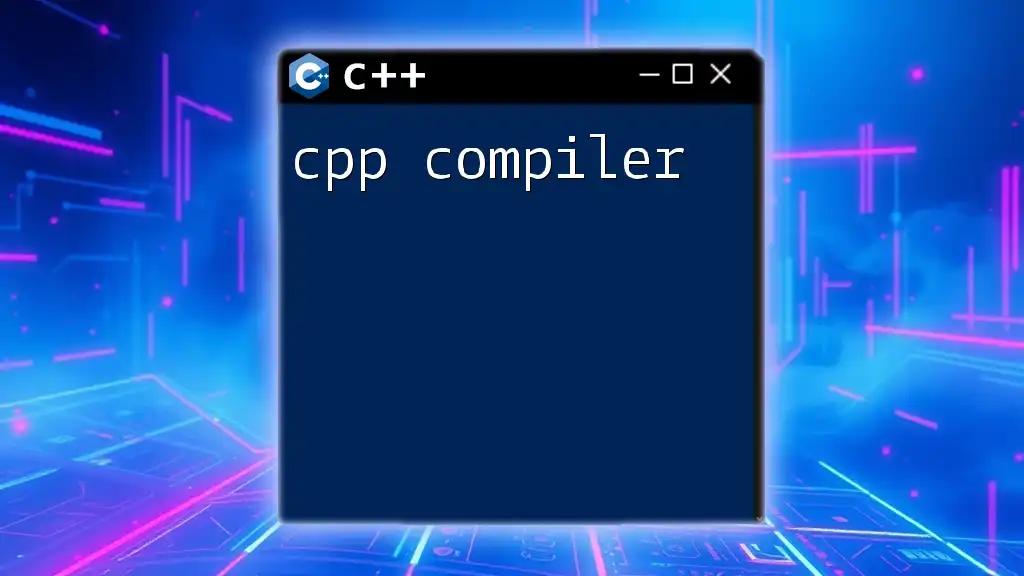
Conclusion
Throughout this journey of starting out with C++ 9th edition, we have explored essential concepts from setting up your development environment to advanced topics like OOP and exception handling. Mastering these foundations will empower you to progress in your C++ programming skillset.
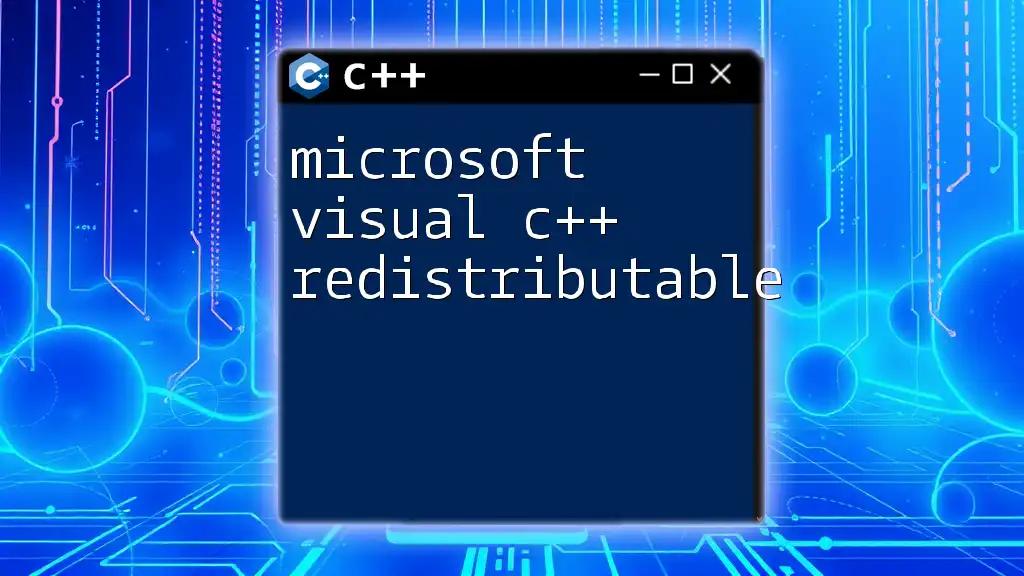
Additional Resources
To continue your learning path, consider exploring books dedicated to C++, online courses, and tutorials that dive deeper into the specifics of the language. Engaging with community forums can also be incredibly helpful for getting answers to your questions and participating in discussions.
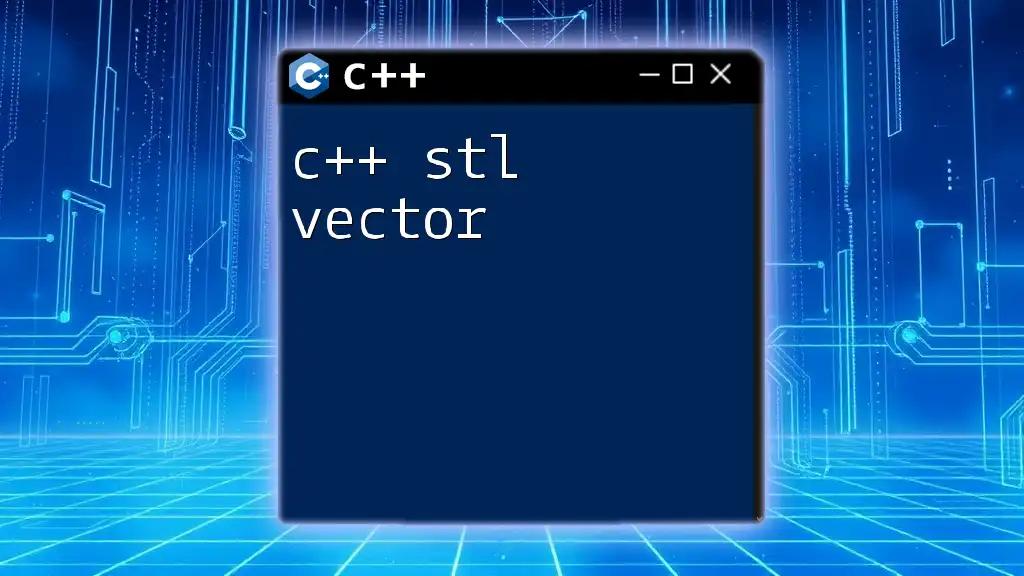
Call to Action
I encourage you to start practicing C++ by taking on small projects or challenges. The more you code, the more proficient you will become. Embrace the learning process, and remember that every programmer was once a beginner!